Spring & Validations: Supporting Both Infrastructure and Business Logic
Where to put validation rules, how to preserve domain purity, and how Spring helps enforce both technical and business constraints. As stated in Spring’s documentation: "There are pros and cons for considering validation as business logic, and Spring offers a design for validation and data binding that does not exclude either one of them. Specifically, validation should not be tied to the web tier and should be easy to localize, and it should be possible to plug in any available validator. Considering these concerns, Spring provides a Validator contract that is both basic and eminently usable in every layer of an application." So let's break this down. Validation in Spring: Business Logic or Infrastructure Concern, Where should validations live? In the documentation it's mentioned that there are pros and cons for considering validation as business logic. So should it be part of the domain model? Or should it remain in the infrastructure? Let's explore the decision by a Domain-Driven Design approach, and also explore the flexibility that Spring offers. Pros of Considering Validation as Business Logic (i.e., part of the Domain Model): By taking part of the domain model as an example: "The ENTITY is responsible for maintaining its own invariants and enforcing its own rules" What we can take away is: Always Follows the Business Rules: Rules like “a ticket can't be sold after the event starts” are always checked. Protects Invariants: The model will never enter in an invalid state. Reusable Logic: Whether the model is invoked through API, batch jobs, etc. public class Event { private final UUID id; private final String name; private final LocalDateTime startTime; private final int capacity; private int ticketsSold; public Event(UUID id, String name, LocalDateTime startTime, int capacity) { this.id = id; this.name = name; this.startTime = startTime; this.capacity = capacity; this.ticketsSold = 0; } // Always Follows the Business Rules public boolean canSellTicket() { return LocalDateTime.now().isBefore(startTime); } // Protects Invariants public void sellTicket(int quantity) { if (!canSellTicket()) { throw new IllegalStateException("Ticket sales are closed: event has already started."); } if (ticketsSold + quantity > capacity) { throw new IllegalStateException("Not enough tickets available."); } ticketsSold += quantity; } public UUID getId() { return id; } public int getRemainingTickets() { return capacity - ticketsSold; } // Other getters/setters } But, as good as it sounds there are still some very important cons to be considered, which makes us reconsider. Cons of Considering Validation as Business Logic One of the most important cons is overloading the domain model with all kinds of checks, business & technical. This can lead to: Hard to Read & Maintain Hard to Reuse Rules: You can’t reuse single rules (like “name must not be blank”) without instantiating the whole model. Too Strict for Some Use Cases: Partial data (e.g., drafts events) can’t be created because the model expects full, valid input. public class Event { private final UUID id; private final String name; private final LocalDateTime startTime; private final int capacity; private int ticketsSold; public Event(UUID id, String name, LocalDateTime startTime, int capacity) { // ❌ 1. Too Much Logic in One Place if (name == null || name.isBlank()) { throw new IllegalArgumentException("Name cannot be blank"); } if (!name.matches("[A-Za-z0-9 ]+")) { throw new IllegalArgumentException("Event name contains invalid characters"); } if (name.length() > 100) { throw new IllegalArgumentException("Name too long"); } if (capacity < 1 || capacity > 10000) { throw new IllegalArgumentException("Capacity must be between 1 and 10000"); } if (startTime.isBefore(LocalDateTime.now())) { throw new IllegalArgumentException("Start time must be in the future"); } this.id = id; this.name = name; this.startTime = startTime; this.capacity = capacity; this.ticketsSold = 0; } // ❌ 2. Hard to Reuse Rules public static boolean isValidName(String name) { return name != null && !name.isBlank() && name.length()
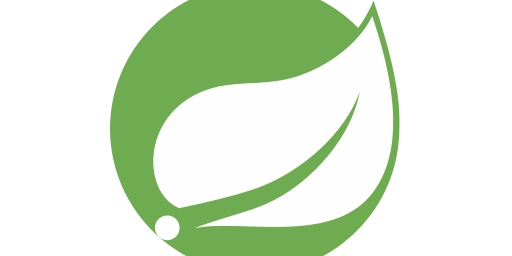
Where to put validation rules, how to preserve domain purity, and how Spring helps enforce both technical and business constraints.
As stated in Spring’s documentation:
"There are pros and cons for considering validation as business logic, and Spring offers a design for validation and data binding that does not exclude either one of them. Specifically, validation should not be tied to the web tier and should be easy to localize, and it should be possible to plug in any available validator. Considering these concerns, Spring provides a Validator contract that is both basic and eminently usable in every layer of an application."
So let's break this down.
Validation in Spring: Business Logic or Infrastructure Concern, Where should validations live?
In the documentation it's mentioned that there are pros and cons for considering validation as business logic. So should it be part of the domain model? Or should it remain in the infrastructure?
Let's explore the decision by a Domain-Driven Design approach, and also explore the flexibility that Spring offers.
Pros of Considering Validation as Business Logic (i.e., part of the Domain Model):
By taking part of the domain model as an example:
"The ENTITY is responsible for maintaining its own invariants and enforcing its own rules"
What we can take away is:
Always Follows the Business Rules: Rules like “a ticket can't be sold after the event starts” are always checked.
Protects Invariants: The model will never enter in an invalid state.
Reusable Logic: Whether the model is invoked through API, batch jobs, etc.
public class Event {
private final UUID id;
private final String name;
private final LocalDateTime startTime;
private final int capacity;
private int ticketsSold;
public Event(UUID id, String name, LocalDateTime startTime, int capacity) {
this.id = id;
this.name = name;
this.startTime = startTime;
this.capacity = capacity;
this.ticketsSold = 0;
}
// Always Follows the Business Rules
public boolean canSellTicket() {
return LocalDateTime.now().isBefore(startTime);
}
// Protects Invariants
public void sellTicket(int quantity) {
if (!canSellTicket()) {
throw new IllegalStateException("Ticket sales are closed: event has already started.");
}
if (ticketsSold + quantity > capacity) {
throw new IllegalStateException("Not enough tickets available.");
}
ticketsSold += quantity;
}
public UUID getId() {
return id;
}
public int getRemainingTickets() {
return capacity - ticketsSold;
}
// Other getters/setters
}
But, as good as it sounds there are still some very important cons to be considered, which makes us reconsider.
Cons of Considering Validation as Business Logic
One of the most important cons is overloading the domain model with all kinds of checks, business & technical. This can lead to:
Hard to Read & Maintain
Hard to Reuse Rules: You can’t reuse single rules (like “name must not be blank”) without instantiating the whole model.
Too Strict for Some Use Cases: Partial data (e.g., drafts events) can’t be created because the model expects full, valid input.
public class Event {
private final UUID id;
private final String name;
private final LocalDateTime startTime;
private final int capacity;
private int ticketsSold;
public Event(UUID id, String name, LocalDateTime startTime, int capacity) {
// ❌ 1. Too Much Logic in One Place
if (name == null || name.isBlank()) {
throw new IllegalArgumentException("Name cannot be blank");
}
if (!name.matches("[A-Za-z0-9 ]+")) {
throw new IllegalArgumentException("Event name contains invalid characters");
}
if (name.length() > 100) {
throw new IllegalArgumentException("Name too long");
}
if (capacity < 1 || capacity > 10000) {
throw new IllegalArgumentException("Capacity must be between 1 and 10000");
}
if (startTime.isBefore(LocalDateTime.now())) {
throw new IllegalArgumentException("Start time must be in the future");
}
this.id = id;
this.name = name;
this.startTime = startTime;
this.capacity = capacity;
this.ticketsSold = 0;
}
// ❌ 2. Hard to Reuse Rules
public static boolean isValidName(String name) {
return name != null && !name.isBlank() && name.length() <= 100;
}
// ❌ 3. Too Strict for Some Use Cases
public static Event createDraft(UUID id, String name) {
// Constructor expects full, valid input — can't easily create drafts
return new Event(id, name, LocalDateTime.now().plusDays(1), 100);
}
// Other getters/setters
}
Treating Validations Through Spring
One way to avoid overloading the model with technical checks on the input, is to assign that responsibility to the infrastructure layer (they could also be configured on the persistence layer but that can lead to orchestration issues). Spring through Bean Validation makes it possible to handle such cases on that layer:
Overloading the model:
public Event(String name, int capacity, LocalDateTime startTime) {
if (name == null || name.isBlank()) {
throw new IllegalArgumentException("Name cannot be blank");
}
if (capacity < 1 || capacity > 10000) {
throw new IllegalArgumentException("Capacity must be between 1 and 10000");
}
if (startTime.isBefore(LocalDateTime.now())) {
throw new IllegalArgumentException("Start time must be in the future");
}
}
public class CreateEventRequest {
@NotBlank
@Size(max = 100)
private String name;
@Min(1)
@Max(10000)
private int capacity;
@NotNull
@Future
private LocalDateTime startTime;
// getters and setters
}
In controller:
@PostMapping("/events")
public ResponseEntity> create(@Valid @RequestBody CreateEventRequest request) {
Event event = new Event(request.getName(), request.getCapacity(), request.getStartTime());
...
}
Under the Hood
Spring Boot autoconfigures a Validator behind the scenes which in turn inspects the fields of the request object (if they have configs like @NotNull, @Size, etc.) and apply the validation rules before the method implementation is executed.
But sometimes the infrastructure might be bypassed, and the application layer might be invoked directly. This could lead to technical and unwanted business errors, so how does Spring make it possible to handle this?
Validation by Using Spring’s Validator Interface
The Spring org.springframework.validation.Validator implementations are flexible enough to be considered as technical components, or as an orchestrator for parts of the domain model by making use of Specifications or Domain-Services.
By creating injectable implementations of the Validator, they can be used in the application layer. The example below will treat input & business logic validations (i.e., using Domain-Services)
FlightBookingCommand {
Long userId = 123L;
Long flightId = 42L;
}
Input Validator
Purpose: Validate the format, structure, and presence of data.
@Override
public void validate(Object target, Errors errors) {
FlightBookingCommand cmd = (FlightBookingCommand) target;
if (cmd.getUserId() == null) {
errors.rejectValue("userId", "userId.missing", "User ID is required.");
}
if (cmd.getFlightId() == null || cmd.getFlightId() <= 0) {
errors.rejectValue("flightId", "flightId.invalid", "Flight ID must be a positive number.");
}
}
Domain Validator
@Override
public void validate(Object target, Errors errors) {
FlightBookingCommand cmd = (FlightBookingCommand) target;
if (!creditService.hasEnoughCredits(cmd.getUserId(), cmd.getFlightId())) {
errors.reject("credits.low", "User does not have enough credits.");
}
if (flightService.isFlightFull(cmd.getFlightId())) {
errors.reject("flight.full", "Flight is already fully booked.");
}
}
@Service
public class FlightBookingService {
// DI
public void bookFlight(FlightBookingCommand command) {
// STEP 1: Input validation
Errors inputErrors = new BeanPropertyBindingResult(command, "command");
inputValidator.validate(command, inputErrors);
if (inputErrors.hasErrors()) {
throw new ValidationException("Input is invalid", inputErrors);
}
// STEP 2: Domain validation (same object, different rules)
Errors domainErrors = new BeanPropertyBindingResult(command, "command");
domainValidator.validate(command, domainErrors);
if (domainErrors.hasErrors()) {
throw new ValidationException("Business rule violated", domainErrors);
}
// STEP 3: Proceed with booking
Flight flight = flightRepository.findById(command.getFlightId()).orElseThrow();
Booking booking = new Booking(command.getUserId(), flight);
bookingRepository.save(booking);
}
}
Example Response
{
"errors": [
"User ID is required.",
"Flight is already fully booked."
]
}
Validation isn’t just about checking data — it’s also about design decisions, and Spring’s flexibility gives us the freedom to decide where and how they should be.