What is @Component and How to Use It in Spring
What is @Component? @Component is a stereotype annotation from the Spring framework that allows you to mark your classes as Spring components. These classes will be managed by Spring and will be available in the Spring IoC container, so they can be injected into other classes (also managed by Spring) through the @Autowired annotation. Use this annotation when you need to create an application component that does not deal with business logic, or when it does not fit into web, service, or repository layers. It's a more generic type. If you're not sure which stereotype to use, @Component is a good choice. Why use @Component? It marks your class for Spring to auto-detect and register it into the Spring IoC container. It facilitates component injection and dependency management. It makes it easier to create more flexible classes due to the inversion of control. You can add properties to your component and manage these properties from an external configuration, allowing you to change values without recompiling and redeploying your application. How to use @Component This annotation is simple to use. Define a class with @Component: @Component public class MyComponent { public void doSomething() { System.out.println("Doing something..."); } } Now your bean is already managed by Spring (if it's been scanned by Spring; check the @ComponentScan article to understand how this works). It will be available for injection with @Autowired like this: @Service public class MyService { private final MyComponent myComponent; @Autowired public MyService(MyComponent myComponent) { this.myComponent = myComponent; } public void performAction() { myComponent.doSomething(); } } In the example above, MyService uses @Autowired to inject MyComponent. @Component is an important Spring tool that helps create and manage parts of your program. It's useful when you need to connect different parts of your code. Use @Component when you're unsure if your class should be a controller, service, or repository. If you like this topic, make sure to follow me. I’ll be explaining more about Spring annotations! Stay tuned! Willian Moya (@WillianFMoya) / X (twitter.com) Willian Ferreira Moya | LinkedIn
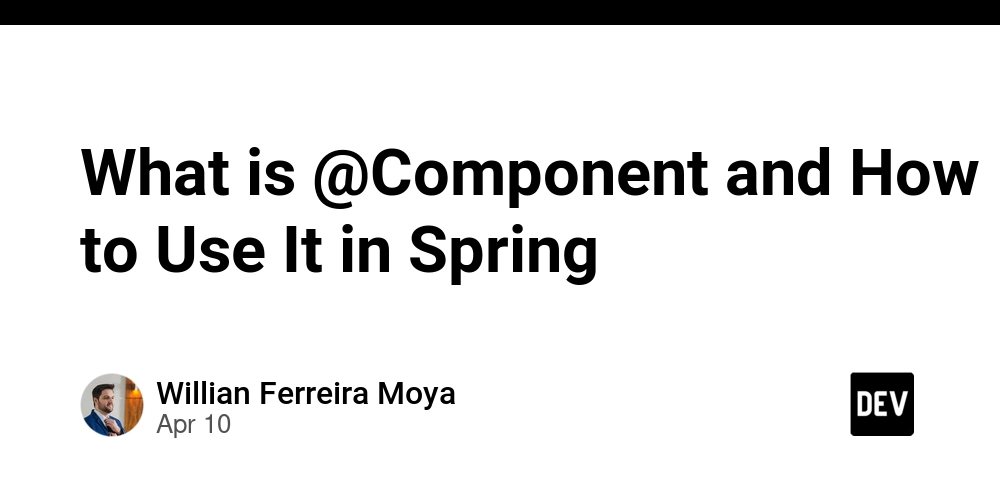
What is @Component
?
@Component
is a stereotype annotation from the Spring framework that allows you to mark your classes as Spring components. These classes will be managed by Spring and will be available in the Spring IoC container, so they can be injected into other classes (also managed by Spring) through the @Autowired
annotation.
Use this annotation when you need to create an application component that does not deal with business logic, or when it does not fit into web, service, or repository layers. It's a more generic type. If you're not sure which stereotype to use, @Component
is a good choice.
Why use @Component
?
- It marks your class for Spring to auto-detect and register it into the Spring IoC container.
- It facilitates component injection and dependency management.
- It makes it easier to create more flexible classes due to the inversion of control. You can add properties to your component and manage these properties from an external configuration, allowing you to change values without recompiling and redeploying your application.
How to use @Component
This annotation is simple to use. Define a class with @Component
:
@Component
public class MyComponent {
public void doSomething() {
System.out.println("Doing something...");
}
}
Now your bean is already managed by Spring (if it's been scanned by Spring; check the @ComponentScan
article to understand how this works). It will be available for injection with @Autowired
like this:
@Service
public class MyService {
private final MyComponent myComponent;
@Autowired
public MyService(MyComponent myComponent) {
this.myComponent = myComponent;
}
public void performAction() {
myComponent.doSomething();
}
}
In the example above, MyService
uses @Autowired
to inject MyComponent
.
@Component
is an important Spring tool that helps create and manage parts of your program. It's useful when you need to connect different parts of your code. Use @Component
when you're unsure if your class should be a controller, service, or repository.
If you like this topic, make sure to follow me. I’ll be explaining more about Spring annotations! Stay tuned!