Understanding Java Garbage Collection
If you've spent any time coding in Java, you've probably encountered, or at least heard, about the mysterious process called Garbage Collection (GC). At first glance, it might seem complicated or even magical, but in reality, it's a powerful feature designed to simplify your life as a developer. So, what exactly is garbage collection? In simple terms, garbage collection in Java is an automatic memory management process. Imagine you're constantly creating objects in your application, which occupy space in your memory (specifically, the heap memory). Eventually, some of these objects are no longer needed or used, they become "garbage." If left unchecked, these unnecessary objects clutter your memory, leading to slower performance and potential memory leaks. That's where garbage collection steps in. It automatically identifies and removes objects that are no longer reachable or needed by your application, freeing up valuable memory resources. Essentially, Java's garbage collector is like an efficient janitor, quietly working behind the scenes to keep your application's memory clean and tidy. How does Java determine what's garbage? Java uses the concept of object reachability. An object becomes eligible for garbage collection when there are no references pointing to it, meaning it's inaccessible and won't be used again by the program. Once Java identifies these unreachable objects, it frees up their occupied memory. Different Java Garbage Collection Algorithms Java doesn't rely on just one method. Instead, it employs several algorithms: Mark-and-Sweep: Java scans and "marks" live objects that are reachable. Objects not marked are considered garbage and subsequently "swept" or cleaned away. Generational Garbage Collection: Objects are categorized into "Young" and "Old" generations. Typically, short-lived objects reside in the Young generation, which gets cleaned up more frequently. Longer-lived objects reside in the Old generation, where garbage collection occurs less frequently, optimizing overall performance. Garbage-First (G1): Designed to handle large heaps and high-performance applications efficiently, G1 segments the heap into regions and prioritizes cleaning regions with the most garbage first, thus optimizing both performance and efficiency. Can you influence garbage collection? While Java manages garbage collection automatically, you do have some control. You can fine-tune garbage collection behavior with JVM parameters such as -Xmx and -Xms, which set the maximum and initial heap size, respectively. Additionally, methods like System.gc() can suggest to Java that it's a good time to perform garbage collection. However, Java still decides whether or not to act immediately on such requests. Why should you care? Understanding garbage collection isn't just academic—it's crucial for building efficient and high-performance Java applications. By grasping the basics and knowing how to optimize your code to better accommodate garbage collection, you can significantly enhance your application's responsiveness, stability, and scalability. In summary, Java's garbage collection is a sophisticated yet approachable process. It quietly works in the background, ensuring your application's memory stays clean and efficient, allowing you to focus on developing great software without worrying excessively about memory management.
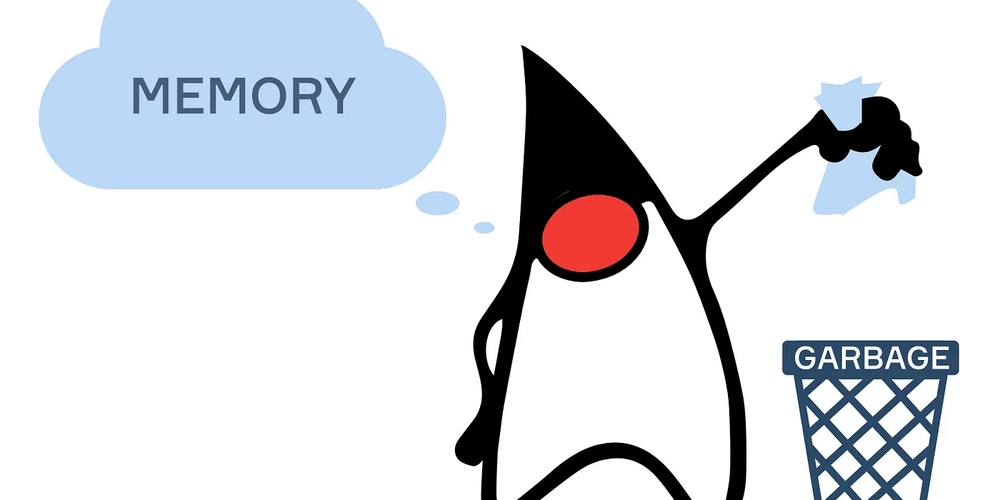
If you've spent any time coding in Java, you've probably encountered, or at least heard, about the mysterious process called Garbage Collection (GC). At first glance, it might seem complicated or even magical, but in reality, it's a powerful feature designed to simplify your life as a developer.
So, what exactly is garbage collection?
In simple terms, garbage collection in Java is an automatic memory management process. Imagine you're constantly creating objects in your application, which occupy space in your memory (specifically, the heap memory). Eventually, some of these objects are no longer needed or used, they become "garbage." If left unchecked, these unnecessary objects clutter your memory, leading to slower performance and potential memory leaks.
That's where garbage collection steps in. It automatically identifies and removes objects that are no longer reachable or needed by your application, freeing up valuable memory resources. Essentially, Java's garbage collector is like an efficient janitor, quietly working behind the scenes to keep your application's memory clean and tidy.
How does Java determine what's garbage?
Java uses the concept of object reachability. An object becomes eligible for garbage collection when there are no references pointing to it, meaning it's inaccessible and won't be used again by the program. Once Java identifies these unreachable objects, it frees up their occupied memory.
Different Java Garbage Collection Algorithms
Java doesn't rely on just one method. Instead, it employs several algorithms:
Mark-and-Sweep: Java scans and "marks" live objects that are reachable. Objects not marked are considered garbage and subsequently "swept" or cleaned away.
Generational Garbage Collection: Objects are categorized into "Young" and "Old" generations. Typically, short-lived objects reside in the Young generation, which gets cleaned up more frequently. Longer-lived objects reside in the Old generation, where garbage collection occurs less frequently, optimizing overall performance.
Garbage-First (G1): Designed to handle large heaps and high-performance applications efficiently, G1 segments the heap into regions and prioritizes cleaning regions with the most garbage first, thus optimizing both performance and efficiency.
Can you influence garbage collection?
While Java manages garbage collection automatically, you do have some control. You can fine-tune garbage collection behavior with JVM parameters such as -Xmx
and -Xms
, which set the maximum and initial heap size, respectively. Additionally, methods like System.gc()
can suggest to Java that it's a good time to perform garbage collection. However, Java still decides whether or not to act immediately on such requests.
Why should you care?
Understanding garbage collection isn't just academic—it's crucial for building efficient and high-performance Java applications. By grasping the basics and knowing how to optimize your code to better accommodate garbage collection, you can significantly enhance your application's responsiveness, stability, and scalability.
In summary, Java's garbage collection is a sophisticated yet approachable process. It quietly works in the background, ensuring your application's memory stays clean and efficient, allowing you to focus on developing great software without worrying excessively about memory management.