Typed Arrays and ArrayBuffer Deep Dive
Typed Arrays and ArrayBuffer Deep Dive Introduction JavaScript, as a dynamically typed and high-level programming language, abstracts many complexities away from developers. However, as applications grow in size and performance requirements increase, the need for more efficient data manipulation techniques is paramount. This leads us to the realm of Typed Arrays and ArrayBuffer—powerful structures designed for high-performance manipulation of binary data. Historical Context The introduction of Typed Arrays in JavaScript came with the ECMAScript 2015 (ES6) specification, but the roots can be traced back to the need for efficient data manipulation in web applications. Before Typed Arrays, JavaScript primarily relied on standard Arrays, which are high-level and lack performance efficiency when dealing with binary data. This led to increased interest in handling raw binary data, especially with the burgeoning growth of web APIs that interact with binary formats, such as WebGL (for graphics rendering) and web storage. ArrayBuffer: The Foundation of Typed Arrays ArrayBuffer is a generic, fixed-length raw binary data buffer. You can think of it as a block of memory that stores data of any type. The ArrayBuffer itself does not provide methods or properties directly for reading or writing its contents; instead, it must be associated with a Typed Array or a DataView object. // Creating an ArrayBuffer of 16 bytes const buffer = new ArrayBuffer(16); console.log(buffer.byteLength); // 16 Typed Arrays Typed Arrays are special kinds of array-like objects that provide a mechanism for accessing and manipulating the raw binary data stored in an ArrayBuffer; they offer a more efficient way to work with binary data than standard JavaScript arrays. JavaScript provides several Typed Array types, including: Int8Array Uint8Array Uint8ClampedArray Int16Array Uint16Array Int32Array Uint32Array Float32Array Float64Array BigInt64Array BigUint64Array Each Typed Array type points to the underlying ArrayBuffer but ensures correct handling of data type-specific views. // Create a Uint8Array from the ArrayBuffer const intArray = new Uint8Array(buffer); intArray[0] = 42; intArray[1] = 255; console.log(intArray[0]); // 42 console.log(intArray[1]); // 255 Advanced Access and Manipulation Dynamic Sizing of Typed Arrays Typed Arrays can be resized using the underlying ArrayBuffer. While you cannot change the size of a Typed Array once created, you can create a new Typed Array with a different length that references the same ArrayBuffer. const smallArray = new Uint8Array(2); smallArray[0] = 2; smallArray[1] = 4; // Create a larger view of the same ArrayBuffer const largerArray = new Uint8Array(smallArray.buffer, 0, 4); largerArray[2] = 5; // Can manipulate newly created console.log(smallArray); // Uint8Array(2) [ 2, 4 ] console.log(largerArray); // Uint8Array(4) [ 2, 4, 5, 0 ] Edge Cases and Performance Considerations Memory Management It is crucial to understand that Typed Arrays and ArrayBuffer do not automatically free memory when no longer required. Proper cleanup is needed to prevent memory leaks. In a Node.js environment or other long-running applications, managing memory efficiently becomes vital, as growth in memory usage can lead to performance degradation. Performance Impact Typed Arrays are significantly faster than regular JavaScript arrays when working with numeric data. This advantage arises from two core aspects: Contiguous Memory Layout: Typed Arrays allocate memory in bulk (a contiguous block), which is optimal for CPU caches. Type Consistency: Unlike regular JavaScript types that dynamically change, Typed Arrays enforce a fixed type—this uniformity allows for lower CPU overhead during operations. Performance optimizations can include using Uint8Array (or other Typed Arrays) for intensive numeric operations instead of BigInt or floating-point types when extreme precision is not needed. Real-World Use Cases WebGL: Graphics applications leverage Typed Arrays to manipulate pixel data efficiently for rendering images. Using Uint8Array for color values enables developers to mesh textures effectively and enhance performance when working with shaders. Audio Processing: Libraries such as Web Audio API utilize Float32Array for audio buffers, allowing for rapid manipulation of audio data streams. Data Streaming: Applications such as video players use Typed Arrays to decode and manipulate streaming data, reducing lag and buffering times. Comparing with Alternative Approaches Prior to Typed Arrays, the most efficient way of handling binary data in JavaScript was through the use of Array or custom implementations. However, neither of these approaches provided the necessary performance efficiency or ease of use. Comparing the structures: Standard Arrays: Pros: E
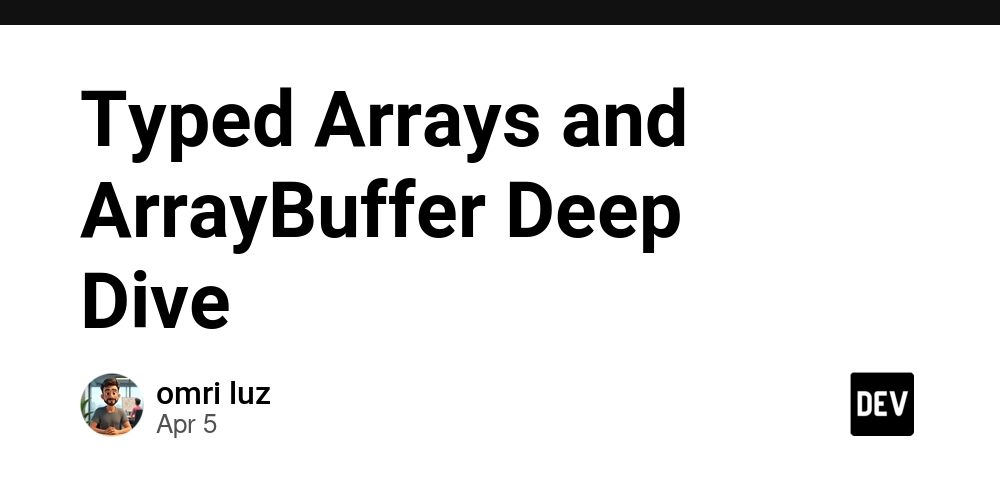
Typed Arrays and ArrayBuffer Deep Dive
Introduction
JavaScript, as a dynamically typed and high-level programming language, abstracts many complexities away from developers. However, as applications grow in size and performance requirements increase, the need for more efficient data manipulation techniques is paramount. This leads us to the realm of Typed Arrays and ArrayBuffer—powerful structures designed for high-performance manipulation of binary data.
Historical Context
The introduction of Typed Arrays in JavaScript came with the ECMAScript 2015 (ES6) specification, but the roots can be traced back to the need for efficient data manipulation in web applications. Before Typed Arrays, JavaScript primarily relied on standard Arrays, which are high-level and lack performance efficiency when dealing with binary data. This led to increased interest in handling raw binary data, especially with the burgeoning growth of web APIs that interact with binary formats, such as WebGL (for graphics rendering) and web storage.
ArrayBuffer: The Foundation of Typed Arrays
ArrayBuffer is a generic, fixed-length raw binary data buffer. You can think of it as a block of memory that stores data of any type. The ArrayBuffer itself does not provide methods or properties directly for reading or writing its contents; instead, it must be associated with a Typed Array or a DataView object.
// Creating an ArrayBuffer of 16 bytes
const buffer = new ArrayBuffer(16);
console.log(buffer.byteLength); // 16
Typed Arrays
Typed Arrays are special kinds of array-like objects that provide a mechanism for accessing and manipulating the raw binary data stored in an ArrayBuffer; they offer a more efficient way to work with binary data than standard JavaScript arrays. JavaScript provides several Typed Array types, including:
Int8Array
Uint8Array
Uint8ClampedArray
Int16Array
Uint16Array
Int32Array
Uint32Array
Float32Array
Float64Array
BigInt64Array
BigUint64Array
Each Typed Array type points to the underlying ArrayBuffer but ensures correct handling of data type-specific views.
// Create a Uint8Array from the ArrayBuffer
const intArray = new Uint8Array(buffer);
intArray[0] = 42;
intArray[1] = 255;
console.log(intArray[0]); // 42
console.log(intArray[1]); // 255
Advanced Access and Manipulation
Dynamic Sizing of Typed Arrays
Typed Arrays can be resized using the underlying ArrayBuffer. While you cannot change the size of a Typed Array once created, you can create a new Typed Array with a different length that references the same ArrayBuffer.
const smallArray = new Uint8Array(2);
smallArray[0] = 2;
smallArray[1] = 4;
// Create a larger view of the same ArrayBuffer
const largerArray = new Uint8Array(smallArray.buffer, 0, 4);
largerArray[2] = 5; // Can manipulate newly created
console.log(smallArray); // Uint8Array(2) [ 2, 4 ]
console.log(largerArray); // Uint8Array(4) [ 2, 4, 5, 0 ]
Edge Cases and Performance Considerations
Memory Management
It is crucial to understand that Typed Arrays and ArrayBuffer do not automatically free memory when no longer required. Proper cleanup is needed to prevent memory leaks. In a Node.js environment or other long-running applications, managing memory efficiently becomes vital, as growth in memory usage can lead to performance degradation.
Performance Impact
Typed Arrays are significantly faster than regular JavaScript arrays when working with numeric data. This advantage arises from two core aspects:
- Contiguous Memory Layout: Typed Arrays allocate memory in bulk (a contiguous block), which is optimal for CPU caches.
- Type Consistency: Unlike regular JavaScript types that dynamically change, Typed Arrays enforce a fixed type—this uniformity allows for lower CPU overhead during operations.
Performance optimizations can include using Uint8Array (or other Typed Arrays) for intensive numeric operations instead of BigInt or floating-point types when extreme precision is not needed.
Real-World Use Cases
WebGL: Graphics applications leverage Typed Arrays to manipulate pixel data efficiently for rendering images. Using Uint8Array for color values enables developers to mesh textures effectively and enhance performance when working with shaders.
Audio Processing: Libraries such as Web Audio API utilize Float32Array for audio buffers, allowing for rapid manipulation of audio data streams.
Data Streaming: Applications such as video players use Typed Arrays to decode and manipulate streaming data, reducing lag and buffering times.
Comparing with Alternative Approaches
Prior to Typed Arrays, the most efficient way of handling binary data in JavaScript was through the use of Array
or custom implementations. However, neither of these approaches provided the necessary performance efficiency or ease of use. Comparing the structures:
-
Standard Arrays:
- Pros: Easy to use, flexible, handles mixed types.
- Cons: Slower for binary and numeric data due to dynamic typing and lack of contiguous memory.
-
Typed Arrays:
- Pros: Fast, efficient, and type-safe.
- Cons: More rigid; dealing with mixed data types requires either manually managing multiple Typed Arrays or supplementing an additional abstraction layer.
Advanced Debugging Techniques and Pitfalls
Debugging binary data can be non-trivial given that the contents may not be readily mappable to human-readable formats. Some suggested strategies include:
- Using DataView: This provides a more flexible interface, allowing you to read different types from the same ArrayBuffer without needing a specific Typed Array.
const view = new DataView(buffer);
view.setInt8(0, 42);
console.log(view.getInt8(0)); // 42
Checking Endianness: When manipulating a DataView, especially when involving network protocols or file formats, being aware of the endianness of data is crucial.
Use of Console: Console methods like
console.table()
can help visually represent the data contained in Typed Arrays.
Conclusion
Typed Arrays and ArrayBuffer represent a pivotal enhancement in the JavaScript ecosystem. They provide an advanced toolkit for developers aiming for high-performance applications that manipulate binary data. By embracing these constructs, developers can optimize their applications, reduce memory overhead, and enhance performance—critical factors in modern web development.
References
- Mozilla Developer Network – Typed Arrays Documentation
- ECMAScript Specification – Typed Array
- WebGL – Typed Arrays Introduction
The above documentation serves as an extensive resource for gaining deeper insights and engaging with advanced features and modifications of Typed Arrays and ArrayBuffer.