Tracking
Imagine if you can automate an alerting system that whenever a user deletes a resource on the AWS cloud you want to send message to specific people. Well, we are going to create one using two AWS services, AWS Lambda and CloudTrail. The main parts of this article: 1- About AWS Services 2- Technical Part (Python code) 3- Conclusion About AWS Services 1- AWS Lambda: AWS Lambda is a compute service that runs your code in response to events and automatically manages the compute resources, making it the fastest way to turn an idea into a modern, production, serverless applications. 2- AWS CloudTrail: AWS CloudTrail is an AWS service that helps you enable operational and risk auditing, governance, and compliance of your AWS account. Actions taken by a user, role, or an AWS service are recorded as events in CloudTrail. Events include actions taken in the AWS Management Console, AWS Command Line Interface, and AWS SDKs and APIs. Architecture overview Before running the Lambda function, make sure CloudTrail logging is enabled. Go to the CloudTrail console, navigate to the Trails section, and create a trail if one doesn't already exist. Ensure that the trail is configured to deliver logs to an S3 bucket, as the Lambda function reads CloudTrail logs directly from S3. Also make sure the Lambda function has the correct IAM role permissions to interact with S3 bucket. import json import boto3 import gzip import io def get_deleted_events(records): deletion_events = [] for record in records: if record.get("eventName") and "Delete" in record["eventName"]: deletion_events.append(record) return deletion_events def lambda_handler(event, context): s3_client = boto3.client("s3") for record in event["Records"]: bucket_name = record["s3"]["bucket"]["name"] object_key = record["s3"]["object"]["key"] response = s3_client.get_object(Bucket=bucket_name, Key=object_key) with gzip.GzipFile(fileobj=io.BytesIO(response["Body"].read()), mode='rb') as file: cloudtrail_logs = json.loads(file.read().decode("utf-8")) deletion_events = get_deleted_events(cloudtrail_logs.get("Records", [])) if deletion_events: print(f"Found {len(deletion_events)} deletion event(s):") for event in deletion_events: print(json.dumps(event, indent=2)) else: print("No deletion events found in this log file.") return {"statusCode": 200, "body": "Processed CloudTrail logs."} Now in order to test this we need to input the following object: { "Records": [ { "s3": { "bucket": { "name": "" }, "object": { "key": "" } } } ] } Now in order to test, I will delete a Lambda function that I don't need it. And then take the latest Cloudtrail log from S3. Great, the Lambda function successfully detected the deletion event. To further enhance this solution, you can update the code to process multiple S3 objects in one run, or consider leveraging AWS-native services like AWS Config, AWS CloudTrail Lake, or AWS Security Hub for more scalable and automated deletion tracking across your environment. Also if you want to notify specific users, consider integrating with services like Amazon SNS or SES. Conclusion This Lambda function provides a lightweight and effective way to track deletion events across your AWS account using CloudTrail logs. By automatically processing logs from S3 and printing relevant deletion actions to CloudWatch Logs, it helps improve visibility, audit ability, and security awareness — without needing any additional alerting or external systems. Happy coding
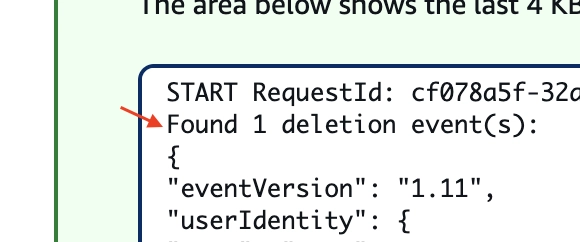
Imagine if you can automate an alerting system that whenever a user deletes a resource on the AWS cloud you want to send message to specific people.
Well, we are going to create one using two AWS services, AWS Lambda and CloudTrail.
The main parts of this article:
1- About AWS Services
2- Technical Part (Python code)
3- Conclusion
About AWS Services
1- AWS Lambda: AWS Lambda is a compute service that runs your code in response to events and automatically manages the compute resources, making it the fastest way to turn an idea into a modern, production, serverless applications.
2- AWS CloudTrail: AWS CloudTrail is an AWS service that helps you enable operational and risk auditing, governance, and compliance of your AWS account. Actions taken by a user, role, or an AWS service are recorded as events in CloudTrail. Events include actions taken in the AWS Management Console, AWS Command Line Interface, and AWS SDKs and APIs.
Architecture overview
Before running the Lambda function, make sure CloudTrail logging is enabled. Go to the CloudTrail console, navigate to the Trails section, and create a trail if one doesn't already exist. Ensure that the trail is configured to deliver logs to an S3 bucket, as the Lambda function reads CloudTrail logs directly from S3.
Also make sure the Lambda function has the correct IAM role permissions to interact with S3 bucket.
import json
import boto3
import gzip
import io
def get_deleted_events(records):
deletion_events = []
for record in records:
if record.get("eventName") and "Delete" in record["eventName"]:
deletion_events.append(record)
return deletion_events
def lambda_handler(event, context):
s3_client = boto3.client("s3")
for record in event["Records"]:
bucket_name = record["s3"]["bucket"]["name"]
object_key = record["s3"]["object"]["key"]
response = s3_client.get_object(Bucket=bucket_name, Key=object_key)
with gzip.GzipFile(fileobj=io.BytesIO(response["Body"].read()), mode='rb') as file:
cloudtrail_logs = json.loads(file.read().decode("utf-8"))
deletion_events = get_deleted_events(cloudtrail_logs.get("Records", []))
if deletion_events:
print(f"Found {len(deletion_events)} deletion event(s):")
for event in deletion_events:
print(json.dumps(event, indent=2))
else:
print("No deletion events found in this log file.")
return {"statusCode": 200, "body": "Processed CloudTrail logs."}
Now in order to test this we need to input the following object:
{
"Records": [
{
"s3": {
"bucket": {
"name": ""
},
"object": {
"key": ""
}
}
}
]
}
Now in order to test, I will delete a Lambda function that I don't need it. And then take the latest Cloudtrail log from S3.
Great, the Lambda function successfully detected the deletion event.
To further enhance this solution, you can update the code to process multiple S3 objects in one run, or consider leveraging AWS-native services like AWS Config, AWS CloudTrail Lake, or AWS Security Hub for more scalable and automated deletion tracking across your environment.
Also if you want to notify specific users, consider integrating with services like Amazon SNS or SES.
Conclusion
This Lambda function provides a lightweight and effective way to track deletion events across your AWS account using CloudTrail logs. By automatically processing logs from S3 and printing relevant deletion actions to CloudWatch Logs, it helps improve visibility, audit ability, and security awareness — without needing any additional alerting or external systems.
Happy coding