Tower Of Hanoi | GeeksforGeeks Beginner's DSA Sheet | Recursion | With Source Code
hola coders! Welcome to my latest video, where I break down the Tower of Hanoi problem using the recursive approach in the simplest and most understandable way! what is the Tower of Hanoi? The Tower of Hanoi is a classic problem that involves moving a set of disks from one peg to another, following three simple rules: Only one disk can be moved at a time. A disk can only be placed on top of a larger disk. You can only move disks between the three pegs. Problem Link: https://www.geeksforgeeks.org/problems/tower-of-hanoi-1587115621/1 Source Code: https://github.com/debeshp6/Gfg-Tutorials/blob/main/TowerOfHanoi.java Code // User function Template for Java class Solution { public int towerOfHanoi(int n, int from, int to, int aux) { // Your code here if(n == 0) return 0; int move1 = towerOfHanoi(n-1, from, aux, to); // recursive call 1 int move2 = 1; int move3 = towerOfHanoi(n-1, aux, to, from); // recursive call 2 return move1 + move2 + move3; // returning the total number of movements } } This problem is an excellent example of recursion and divide-and-conquer techniques, making it essential for coding interviews and competitive programming. why Recursion? Instead of thinking about moving all disks at once, we break the problem into smaller subproblems, moving one disk at a time while following the rules. This recursive approach makes the solution clean, elegant, and easy to implement. If you're preparing for coding interviews or learning DSA, understanding recursion is a must! The Tower of Hanoi is one of the best problems to get a grip on recursive problem-solving techniques. Got any questions? Drop them in the comments below! I’ll be happy to help. happy coding!
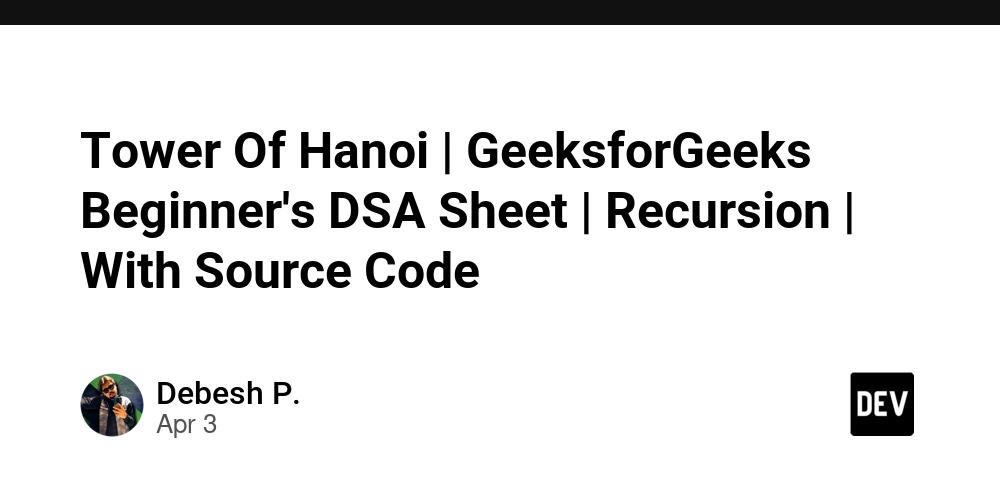
hola coders!
Welcome to my latest video, where I break down the Tower of Hanoi problem using the recursive approach in the simplest and most understandable way!
what is the Tower of Hanoi?
The Tower of Hanoi is a classic problem that involves moving a set of disks from one peg to another, following three simple rules:
- Only one disk can be moved at a time.
- A disk can only be placed on top of a larger disk.
- You can only move disks between the three pegs.
Problem Link: https://www.geeksforgeeks.org/problems/tower-of-hanoi-1587115621/1
Source Code: https://github.com/debeshp6/Gfg-Tutorials/blob/main/TowerOfHanoi.java
Code
// User function Template for Java
class Solution {
public int towerOfHanoi(int n, int from, int to, int aux) {
// Your code here
if(n == 0) return 0;
int move1 = towerOfHanoi(n-1, from, aux, to); // recursive call 1
int move2 = 1;
int move3 = towerOfHanoi(n-1, aux, to, from); // recursive call 2
return move1 + move2 + move3; // returning the total number of movements
}
}
This problem is an excellent example of recursion and divide-and-conquer techniques, making it essential for coding interviews and competitive programming.
why Recursion?
Instead of thinking about moving all disks at once, we break the problem into smaller subproblems, moving one disk at a time while following the rules. This recursive approach makes the solution clean, elegant, and easy to implement.
If you're preparing for coding interviews or learning DSA, understanding recursion is a must! The Tower of Hanoi is one of the best problems to get a grip on recursive problem-solving techniques.
Got any questions? Drop them in the comments below! I’ll be happy to help.
happy coding!