Top React Testing Libraries in 2025
React is a widely-used JavaScript library designed to create interactive and dynamic user interfaces. To ensure your React application is reliable and efficient, thorough testing is essential. React testing libraries help developers write automated tests to catch bugs early, validate functionality, and improve user experience. This guide provides an in-depth look into the top React testing libraries in 2025, their features, and how they can be utilized with practical examples. What is React Testing? React testing is the process of verifying that components and the application as a whole function correctly. It includes testing UI elements, interactions, and logic to ensure seamless performance. React applications benefit from testing in two major ways: Unit Testing: Focuses on individual components. Integration/End-to-End Testing: Validates the interaction between components or the entire application. Top React Testing Libraries 1. Mocha Mocha is a versatile JavaScript testing framework that integrates smoothly with both Node.js and web browsers. It is highly flexible and supports asynchronous testing, making it an excellent choice for applications that require extensive control over the testing environment. Mocha doesn’t include an assertion library but integrates well with popular libraries like Chai and Sinon for assertions and mocks. Developers appreciate its clean syntax, event-driven approach, and adaptability for various project setups, including React. This flexibility makes it one of the top choices for developers looking for granular control. Why Choose Mocha? Highly customizable with plugins Works with other assertion and mocking libraries like Chai and Sinon Example: const assert = require('chai').assert; describe('Array operations', function () { it('should return -1 for a non-existent element', function () { assert.equal([1, 2, 3].indexOf(4), -1); }); }); Use Mocha for detailed control over your testing environment and when dealing with asynchronous code. 2. Jest Jest is a comprehensive testing framework developed by Facebook and is the default choice for testing React applications. It includes built-in capabilities for mocking, spying, and snapshot testing, ensuring minimal setup for testing even the most complex applications. Jest’s extensive support for parallel test execution and interactive features like coverage reporting and watch mode makes it highly efficient. Key Features: Snapshot testing captures the component’s rendered output for comparison. Fast and efficient, with parallel test execution. import { render } from '@testing-library/react'; import App from './App'; test('displays the correct heading', () => { const { getByText } = render(); expect(getByText(/welcome to react/i)).toBeInTheDocument(); }); Jest is ideal for most React projects because of its simplicity and integration capabilities. 3. React Testing Library React Testing Library tests React components by simulating how users interact with them, rather than focusing on the implementation details. This library prioritizes the principle of testing components as users interact with them, making tests more resilient to code changes. By avoiding reliance on the internals of the components, it ensures that your tests remain focused on functionality and user experience. It integrates well with Jest and provides utilities to query, simulate interactions, and validate DOM updates effectively. Advantages: Encourages best practices by avoiding implementation details. Compatible with other testing libraries like Jest. import { render, fireEvent } from '@testing-library/react'; import Counter from './Counter'; test('increments the counter', () => { const { getByText } = render(); const button = getByText('Increment'); fireEvent.click(button); expect(getByText('Count: 1')).toBeInTheDocument(); }); React Testing Library is perfect for developers focusing on user-centered testing. 4. Enzyme Enzyme is a widely-used testing utility that provides robust tools for interacting with and inspecting React components. Its API supports shallow, full, and static rendering, enabling developers to test components in isolation or with their child components. Enzyme also allows testing lifecycle methods, making it ideal for applications with complex state and props interactions. Strengths: Access to component lifecycle methods Fine-grained control over component rendering import { shallow } from 'enzyme'; import Header from './Header'; test('renders the header title', () => { const wrapper = shallow() Use Enzyme for projects requiring detailed testing of React component internals. 5. Chai Chai is a versatile assertion library used for testing JavaScript applications, including React. Known for its expressive and readable syntax, Chai supports multiple assertion styles, such as BDD (Behav
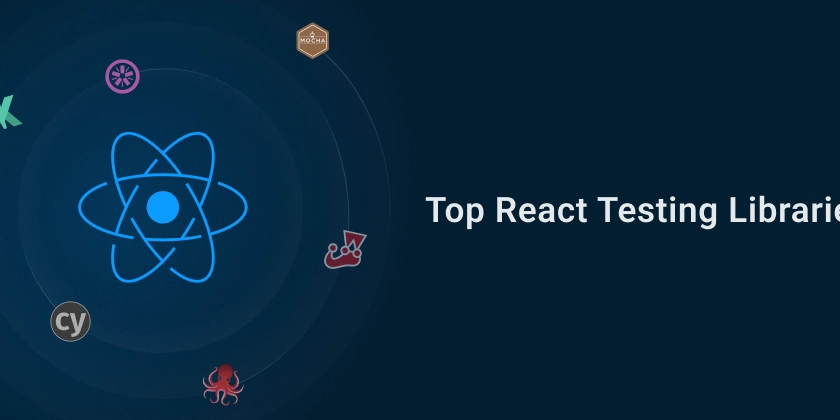
React is a widely-used JavaScript library designed to create interactive and dynamic user interfaces. To ensure your React application is reliable and efficient, thorough testing is essential. React testing libraries help developers write automated tests to catch bugs early, validate functionality, and improve user experience. This guide provides an in-depth look into the top React testing libraries in 2025, their features, and how they can be utilized with practical examples.
What is React Testing?
React testing is the process of verifying that components and the application as a whole function correctly. It includes testing UI elements, interactions, and logic to ensure seamless performance. React applications benefit from testing in two major ways:
- Unit Testing: Focuses on individual components.
- Integration/End-to-End Testing: Validates the interaction between components or the entire application.
Top React Testing Libraries
1. Mocha
Mocha is a versatile JavaScript testing framework that integrates smoothly with both Node.js and web browsers. It is highly flexible and supports asynchronous testing, making it an excellent choice for applications that require extensive control over the testing environment. Mocha doesn’t include an assertion library but integrates well with popular libraries like Chai and Sinon for assertions and mocks. Developers appreciate its clean syntax, event-driven approach, and adaptability for various project setups, including React. This flexibility makes it one of the top choices for developers looking for granular control.
Why Choose Mocha?
Highly customizable with plugins
Works with other assertion and mocking libraries like Chai and Sinon
Example:
const assert = require('chai').assert;
describe('Array operations', function () {
it('should return -1 for a non-existent element', function () {
assert.equal([1, 2, 3].indexOf(4), -1);
});
});
Use Mocha for detailed control over your testing environment and when dealing with asynchronous code.
2. Jest
Jest is a comprehensive testing framework developed by Facebook and is the default choice for testing React applications. It includes built-in capabilities for mocking, spying, and snapshot testing, ensuring minimal setup for testing even the most complex applications. Jest’s extensive support for parallel test execution and interactive features like coverage reporting and watch mode makes it highly efficient.
Key Features:
Snapshot testing captures the component’s rendered output for comparison.
Fast and efficient, with parallel test execution.
import { render } from '@testing-library/react';
import App from './App';
test('displays the correct heading', () => {
const { getByText } = render();
expect(getByText(/welcome to react/i)).toBeInTheDocument();
});
Jest is ideal for most React projects because of its simplicity and integration capabilities.
3. React Testing Library
React Testing Library tests React components by simulating how users interact with them, rather than focusing on the implementation details. This library prioritizes the principle of testing components as users interact with them, making tests more resilient to code changes. By avoiding reliance on the internals of the components, it ensures that your tests remain focused on functionality and user experience. It integrates well with Jest and provides utilities to query, simulate interactions, and validate DOM updates effectively.
Advantages:
Encourages best practices by avoiding implementation details.
Compatible with other testing libraries like Jest.
import { render, fireEvent } from '@testing-library/react';
import Counter from './Counter';
test('increments the counter', () => {
const { getByText } = render();
const button = getByText('Increment');
fireEvent.click(button);
expect(getByText('Count: 1')).toBeInTheDocument();
});
React Testing Library is perfect for developers focusing on user-centered testing.
4. Enzyme
Enzyme is a widely-used testing utility that provides robust tools for interacting with and inspecting React components. Its API supports shallow, full, and static rendering, enabling developers to test components in isolation or with their child components. Enzyme also allows testing lifecycle methods, making it ideal for applications with complex state and props interactions.
Strengths:
Access to component lifecycle methods
Fine-grained control over component rendering
import { shallow } from 'enzyme';
import Header from './Header';
test('renders the header title', () => {
const wrapper = shallow( )
Use Enzyme for projects requiring detailed testing of React component internals.
5. Chai
Chai is a versatile assertion library used for testing JavaScript applications, including React. Known for its expressive and readable syntax, Chai supports multiple assertion styles, such as BDD (Behavior-Driven Development) and TDD (Test-Driven Development). When paired with Mocha, it provides a powerful framework for writing detailed and effective test cases. Chai is particularly useful for testing both synchronous and asynchronous code, making it a reliable choice for complex React applications requiring detailed test scenarios.
Features:
Highly readable assertions
Works seamlessly with testing frameworks
const expect = require('chai').expect;
describe('Math operations', function () {
it('should add two numbers correctly', function () {
expect(2 + 2).to.equal(4);
});
});
Chai pairs well with Mocha, providing a more seamless and efficient testing experience.
6. Jasmine
Jasmine is a standalone JavaScript testing framework that emphasizes simplicity and ease of use. It provides all necessary tools for writing tests without requiring additional libraries. Jasmine’s BDD syntax makes it easy to describe and structure tests in a way that’s easy to understand. While it doesn’t come with advanced features like mocking or spying, its lightweight nature and straightforward approach make it a good option for small to medium-sized React projects.
Benefits:
No dependencies or external libraries required
Simple syntax for writing test cases
Example:
describe('A test suite', function () {
it('contains a passing spec', function () {
expect(true).toBe(true);
});
});
Jasmine is perfect for developers who prefer a standalone testing solution.
7. Cypress
Cypress is a powerful end-to-end testing tool that enables developers to test complete workflows in React applications. With its real-time reloading and time-travel debugging features, Cypress allows developers to observe their tests step-by-step as they run. It provides a fast and interactive experience, making it a top choice for testing user interfaces, API calls, and application behavior in a single framework.
Key Highlights:
Real-time reloads for instant feedback
Built-in time-travel debugging
Example:
describe('Homepage tests', () => {
it('loads the homepage', () => {
cy.visit('/');
cy.contains('Welcome to React');
});
});
Cypress is essential for projects emphasizing comprehensive UI testing.
8. Puppeteer
Puppeteer is a headless browser automation tool built on Chromium. It allows developers to simulate user interactions, capture screenshots, and perform UI tests with a high degree of accuracy. Puppeteer is particularly useful for testing cross-browser compatibility and visual elements in React applications. Its robust API enables precise control over browser actions, making it a valuable tool for UI testing and debugging.
Features:
Control over browser rendering
Capture screenshots for debugging
Example:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
const title = await page.title();
console.log(title);
await browser.close();
})();
Puppeteer is best suited for UI and performance testing.
9. Karma
Karma is a flexible test runner that allows developers to execute tests across multiple real browsers. It’s commonly paired with frameworks like Jasmine and Mocha to provide a seamless testing environment for React applications. Karma is particularly beneficial for applications where cross-browser compatibility is a priority, as it enables developers to validate functionality on various platforms simultaneously.
Why Use Karma?
Runs tests across different browsers
Provides real-world testing scenarios
Example: Testing a React Component Using Karma with Jasmine
1.Install Dependencies
Install Karma and required plugins:
npm install --save-dev karma karma-jasmine jasmine-core karma-chrome-launcher karma-webpack react react-dom @babel/preset-env @babel/preset-react
2.Configure
karma.conf.js
Set up Karma with Webpack and Jasmine:
module.exports = function (config) {
config.set({
frameworks: ['jasmine'], // Use Jasmine framework
files: ['test/**/*.spec.js'], // Test files to include
preprocessors: {
'test/**/*.spec.js': ['webpack'], // Preprocess test files using Webpack
},
webpack: {
mode: 'development',
module: {
rules: [
{
test: /\.jsx?$/,
exclude: /node_modules/,
loader: 'babel-loader',
options: {
presets: ['@babel/preset-env', '@babel/preset-react'],
},
},
],
},
},
browsers: ['Chrome'], // Use Chrome for testing
reporters: ['progress'], // Show progress of tests
singleRun: true, // Run tests once
});
};
3.React Component to Test
Create a simple React component in src/Button.js
:
import React from 'react';
export default function Button({ onClick }) {
return ;
}
4.Test File
Write the test case in test/Button.spec.js
:
import React from 'react';
import { render, fireEvent, screen } from '@testing-library/react';
import Button from '../src/Button';
describe('Button Component', () => {
it('triggers onClick when clicked', () => {
const handleClick = jasmine.createSpy('onClick');
render();
fireEvent.click(screen.getByText('Click Me'));
expect(handleClick).toHaveBeenCalled();
});
});
5.Run Tests
Execute the tests using Karma:
npx karma start
Output
If the test passes, Karma outputs:
Button Component
✓ triggers onClick when clicked
Executed 1 of 1 SUCCESS (0.007 secs / 0.006 secs)
Karma, with its ability to run tests across real browsers, ensures that your React applications function correctly in diverse environments. By combining it with Jasmine, you can create robust, browser-agnostic tests that validate real-world scenarios effectively.
10. React Test Utils and Test Renderer
React’s native tools, TestUtils
and TestRenderer
, are lightweight solutions for testing components directly. While these tools are not as feature-rich as third-party libraries, they are highly effective for small projects or specific component-level tests. They allow developers to simulate events, validate DOM structure, and test component outputs without requiring additional dependencies.
Example:
import TestRenderer from 'react-test-renderer';
import Button from './Button';
const testRenderer = TestRenderer.create();
const testInstance = testRenderer.root;
expect(testInstance.findByType('button').props.children).toBe('Click Me');
These tools are ideal for small projects or component-level testing.
Conclusion
Every React testing library comes with its own advantages and specific use cases. From unit testing with Jest to full-stack testing with Cypress or Puppeteer, the right choice depends on your project needs. Start by integrating one into your workflow, and expand as you encounter more complex testing scenarios.