Top 3 PostgreSQL Performance Issues: Diagnosis and Proven Solutions
1. Slow Queries Due to Lack of Indexing The absence of appropriate indexes is a primary culprit for slow query execution in PostgreSQL. When a query requires filtering or sorting data, PostgreSQL might resort to a full table scan (also known as a sequential scan) if the relevant columns lack indexes. This involves examining every row in the table, which becomes increasingly time-consuming and resource-intensive as the table size grows. Bad Table Design Example (Continued): CREATE TABLE users ( user_id SERIAL PRIMARY KEY, username VARCHAR(50), email VARCHAR(255) ); -- Frequent query without an index: SELECT * FROM users WHERE email = 'test@example.com'; EXPLAIN Output (before adding index): QUERY PLAN ------------------------------------------------- Seq Scan on users (cost=0.00..16.50 rows=1 width=36) Filter: ((email)::text = 'test@example.com'::text) (2 rows) Diagnosis: The EXPLAIN output clearly indicates a Seq Scan (Sequential Scan) on the users table. This signifies that PostgreSQL is examining every row in the table to find the matching email address. The cost estimate also provides an indication of the query's potential resource consumption. Proven Solution: Adding an Index To optimize the query, we can create an index on the email column. A B-tree index is the most common and generally effective type of index for equality and range comparisons. CREATE INDEX idx_users_email ON users (email); EXPLAIN Output (after adding index): QUERY PLAN ------------------------------------------------------------------- Index Scan using idx_users_email on users (cost=0.14..8.16 rows=1 width=36) Index Cond: ((email)::text = 'test@example.com'::text) (2 rows) Explanation: After adding the index, the EXPLAIN output now shows an Index Scan using the newly created idx_users_email index. The Index Cond indicates that the index is being used to directly locate the relevant rows based on the email address. Notice the significantly lower cost estimate, indicating a more efficient query plan. Further Considerations for Indexing: Index Types: PostgreSQL offers various index types (e.g., B-tree, Hash, GIN, GIST, BRIN) suitable for different data types and query patterns. Choose the appropriate index type based on your specific needs. For example, GIN indexes are excellent for full-text search or indexing array and JSONB data, while GIST indexes are often used for spatial data. Composite Indexes: If you frequently query on multiple columns together, consider creating a composite index that includes all those columns in the appropriate order. Over-indexing: While indexes improve read performance for certain queries, they can slow down write operations (INSERT, UPDATE, DELETE) as the index also needs to be updated. Avoid creating unnecessary indexes. Identifying Missing Indexes: Tools like pg_stat_statements (an extension that tracks query execution statistics) can help identify frequently executed queries that could benefit from indexing. Analyzing slow query logs can also pinpoint queries taking excessive time. 2. Bloat and Inefficient Vacuuming Over time, PostgreSQL tables and indexes can accumulate "bloat." Bloat refers to dead tuples (rows that have been logically deleted or updated but are still physically present in the database files). This unused space can lead to increased storage consumption, slower sequential scans, and less efficient index operations. Diagnosis: Monitoring Table and Index Sizes: Regularly monitor the size of your tables and indexes. A sudden or unexpected increase might indicate bloat. Using pg_stat_all_tables and pg_stat_all_indexes: These system views provide statistics about table and index activity, including the number of dead tuples. Specialized Tools and Queries: Several community-developed scripts and extensions (like pgstattuple) can provide more detailed information about bloat levels in tables and indexes. Proven Solutions: Regular VACUUM: The VACUUM command reclaims storage occupied by dead tuples. A regular VACUUM operation is essential for maintaining database performance. AUTOVACUUM: PostgreSQL has an autovacuum daemon that automatically performs VACUUM and ANALYZE operations in the background. Ensure that autovacuum is enabled and properly configured. Adjusting parameters like autovacuum_vacuum_threshold and autovacuum_vacuum_scale_factor might be necessary based on your workload. VACUUM FULL (Use with Caution): The VACUUM FULL command rewrites the entire table, reclaiming all dead space and potentially reducing the table size on disk. However, it requires an exclusive lock on the table, making it unavailable for other operations. It should be used sparingly and during maintenance windows. pg_repack: This is an online, non-blocking full table rewrite solution that can be used to eliminate bloat without requiring exclusive locks. It's a more advanced option for critical production environments. 3. I
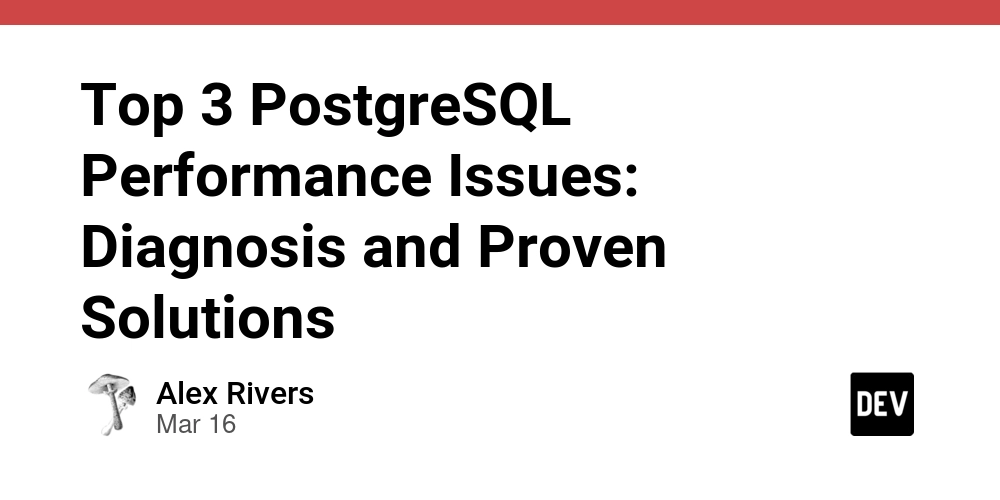
1. Slow Queries Due to Lack of Indexing
The absence of appropriate indexes is a primary culprit for slow query execution in PostgreSQL. When a query requires filtering or sorting data, PostgreSQL might resort to a full table scan (also known as a sequential scan) if the relevant columns lack indexes. This involves examining every row in the table, which becomes increasingly time-consuming and resource-intensive as the table size grows.
Bad Table Design Example (Continued):
CREATE TABLE users (
user_id SERIAL PRIMARY KEY,
username VARCHAR(50),
email VARCHAR(255)
);
-- Frequent query without an index:
SELECT * FROM users WHERE email = 'test@example.com';
EXPLAIN Output (before adding index):
QUERY PLAN
-------------------------------------------------
Seq Scan on users (cost=0.00..16.50 rows=1 width=36)
Filter: ((email)::text = 'test@example.com'::text)
(2 rows)
Diagnosis: The EXPLAIN
output clearly indicates a Seq Scan
(Sequential Scan) on the users
table. This signifies that PostgreSQL is examining every row in the table to find the matching email address. The cost
estimate also provides an indication of the query's potential resource consumption.
Proven Solution: Adding an Index
To optimize the query, we can create an index on the email
column. A B-tree index is the most common and generally effective type of index for equality and range comparisons.
CREATE INDEX idx_users_email ON users (email);
EXPLAIN Output (after adding index):
QUERY PLAN
-------------------------------------------------------------------
Index Scan using idx_users_email on users (cost=0.14..8.16 rows=1 width=36)
Index Cond: ((email)::text = 'test@example.com'::text)
(2 rows)
Explanation: After adding the index, the EXPLAIN
output now shows an Index Scan
using the newly created idx_users_email
index. The Index Cond
indicates that the index is being used to directly locate the relevant rows based on the email address. Notice the significantly lower cost
estimate, indicating a more efficient query plan.
Further Considerations for Indexing:
- Index Types: PostgreSQL offers various index types (e.g., B-tree, Hash, GIN, GIST, BRIN) suitable for different data types and query patterns. Choose the appropriate index type based on your specific needs. For example, GIN indexes are excellent for full-text search or indexing array and JSONB data, while GIST indexes are often used for spatial data.
- Composite Indexes: If you frequently query on multiple columns together, consider creating a composite index that includes all those columns in the appropriate order.
- Over-indexing: While indexes improve read performance for certain queries, they can slow down write operations (INSERT, UPDATE, DELETE) as the index also needs to be updated. Avoid creating unnecessary indexes.
-
Identifying Missing Indexes: Tools like
pg_stat_statements
(an extension that tracks query execution statistics) can help identify frequently executed queries that could benefit from indexing. Analyzing slow query logs can also pinpoint queries taking excessive time.
2. Bloat and Inefficient Vacuuming
Over time, PostgreSQL tables and indexes can accumulate "bloat." Bloat refers to dead tuples (rows that have been logically deleted or updated but are still physically present in the database files). This unused space can lead to increased storage consumption, slower sequential scans, and less efficient index operations.
Diagnosis:
- Monitoring Table and Index Sizes: Regularly monitor the size of your tables and indexes. A sudden or unexpected increase might indicate bloat.
-
Using
pg_stat_all_tables
andpg_stat_all_indexes
: These system views provide statistics about table and index activity, including the number of dead tuples. -
Specialized Tools and Queries: Several community-developed scripts and extensions (like
pgstattuple
) can provide more detailed information about bloat levels in tables and indexes.
Proven Solutions:
-
Regular
VACUUM
: TheVACUUM
command reclaims storage occupied by dead tuples. A regularVACUUM
operation is essential for maintaining database performance. -
AUTOVACUUM
: PostgreSQL has anautovacuum
daemon that automatically performsVACUUM
andANALYZE
operations in the background. Ensure thatautovacuum
is enabled and properly configured. Adjusting parameters likeautovacuum_vacuum_threshold
andautovacuum_vacuum_scale_factor
might be necessary based on your workload. -
VACUUM FULL
(Use with Caution): TheVACUUM FULL
command rewrites the entire table, reclaiming all dead space and potentially reducing the table size on disk. However, it requires an exclusive lock on the table, making it unavailable for other operations. It should be used sparingly and during maintenance windows. -
pg_repack
: This is an online, non-blocking full table rewrite solution that can be used to eliminate bloat without requiring exclusive locks. It's a more advanced option for critical production environments.
3. Inefficient Queries Beyond Indexing
Even with proper indexing, some queries can still perform poorly due to their structure and the operations they perform. Inefficient query patterns can put unnecessary load on the database server.
Diagnosis:
-
EXPLAIN ANALYZE
: UnlikeEXPLAIN
,EXPLAIN ANALYZE
actually executes the query and provides detailed information about the execution time of each step in the query plan. This is invaluable for identifying bottlenecks. - Slow Query Logs: Configure PostgreSQL to log queries that take longer than a specified threshold. Analyzing these logs can reveal patterns of inefficient queries.
-
pg_stat_statements
: This extension provides statistics on the execution time and resource usage of all executed SQL statements, helping to identify the most time-consuming queries.
Proven Solutions:
-
Rewriting Queries: Often, a poorly performing query can be significantly improved by rewriting it. This might involve:
-
Avoiding
SELECT *
: Only select the columns you actually need. Retrieving unnecessary data increases I/O and network traffic. -
Optimizing
WHERE
clauses: Ensure that yourWHERE
clauses are selective and use indexed columns effectively. Avoid using functions on indexed columns in theWHERE
clause (e.g.,WHERE lower(email) = ...
) as it might prevent index usage. - Efficient Joins: Understand the different types of joins (INNER, LEFT, RIGHT, FULL) and choose the most appropriate one for your needs. Ensure that join conditions use indexed columns.
-
Limiting Results: Use
LIMIT
andOFFSET
clauses when fetching only a subset of rows. - Using Appropriate Functions: Leverage built-in PostgreSQL functions for specific tasks instead of performing complex logic in your application.
- Considering Materialized Views: For frequently executed complex queries with relatively static underlying data, consider using materialized views to pre-compute and store the results.
-
Avoiding
-
Analyzing Query Plans: Carefully examine the output of
EXPLAIN
andEXPLAIN ANALYZE
to understand how PostgreSQL is executing your queries and identify potential areas for optimization. Look for operations likeSeq Scan
on large tables when an index scan is expected, or inefficient join algorithms likeMerge Join
when aHash Join
might be more appropriate. - Partitioning: For very large tables, consider partitioning them into smaller, more manageable chunks. This can improve query performance by allowing PostgreSQL to only scan the relevant partitions.
Want to learn more? Check out these resources:
https://www.somethingsblog.com/2025/03/16/top-9-postgresql-performance-issues-and-how-to-fix-them/