Synchronous vs. Asynchronous in Python: Understanding the Key Differences
Introduction When building applications, it’s important to know how tasks are handled. Python allows both synchronous and asynchronous programming, and each has its benefits and drawbacks. Choosing the right approach can make your application faster and more efficient. In this article, we will explain the difference between these two methods and look at the frameworks that support them in Python. What is Synchronous Programming? Synchronous programming means tasks run one after another. Each task must finish before the next one starts. This makes it simple to follow, but it can be slow when dealing with multiple operations. Real-Life Example Imagine you are at a bank, and there is only one cashier. Each customer must wait in line and can only be served after the previous customer is done. This is how synchronous programming works—one task at a time, in order. Pros and Cons of Synchronous Programming ✅ Easy to understand and debug ✅ Simple to implement ❌ Can be slow when handling multiple tasks ❌ Blocks execution, causing delays in performance Python Frameworks for Synchronous Programming Flask – A simple web framework that processes requests one at a time. Django (Default Mode) – Django processes requests synchronously unless modified. Requests – A popular library for making HTTP requests, but it runs synchronously. What is Asynchronous Programming? Asynchronous programming allows multiple tasks to run at the same time. This is useful for operations that involve waiting, such as database queries or API requests. Instead of blocking the whole program, asynchronous programming continues running other tasks while waiting for a response. Real-Life Example Imagine you are downloading multiple files from the internet. Instead of waiting for one file to finish before starting the next, your computer can download several files at the same time. You can even browse the internet or work on something else while the downloads continue in the background. This is how asynchronous programming works—multiple tasks can run independently without blocking each other. Pros and Cons of Asynchronous Programming ✅ Ideal for tasks that involve waiting (e.g., API calls, database queries) ✅ Improves performance for applications with multiple users ✅ Helps build real-time applications like chat apps ❌ More complex to understand and debug ❌ Requires knowledge of event loops and coroutines Python Frameworks for Asynchronous Programming FastAPI – A high-performance web framework that runs fully asynchronously. Django (With ASGI and Django Channels) – Supports async processing when configured with ASGI. Asyncio – Python’s built-in library for handling asynchronous tasks. When to Use Synchronous vs. Asynchronous Programming? Use Synchronous Programming When: Your application is simple and does not need to handle many tasks at once. You are working with CPU-heavy tasks rather than waiting for responses. You prefer a straightforward approach that is easy to debug. Use Asynchronous Programming When: Your application involves making lots of API calls or database queries. You need to handle many users at the same time without slowing down. You are building real-time applications like chat apps or live notifications. Conclusion Choosing between synchronous and asynchronous programming depends on your project. Synchronous programming is easier to use, but asynchronous programming is better for handling multiple tasks at once. Frameworks like Flask and Django (default) work well for simple applications, while FastAPI, Django with ASGI are better for handling multiple users at once. Understanding these two approaches will help you build better applications that perform well. If you have experience with synchronous or asynchronous frameworks, feel free to share your thoughts in the comments!
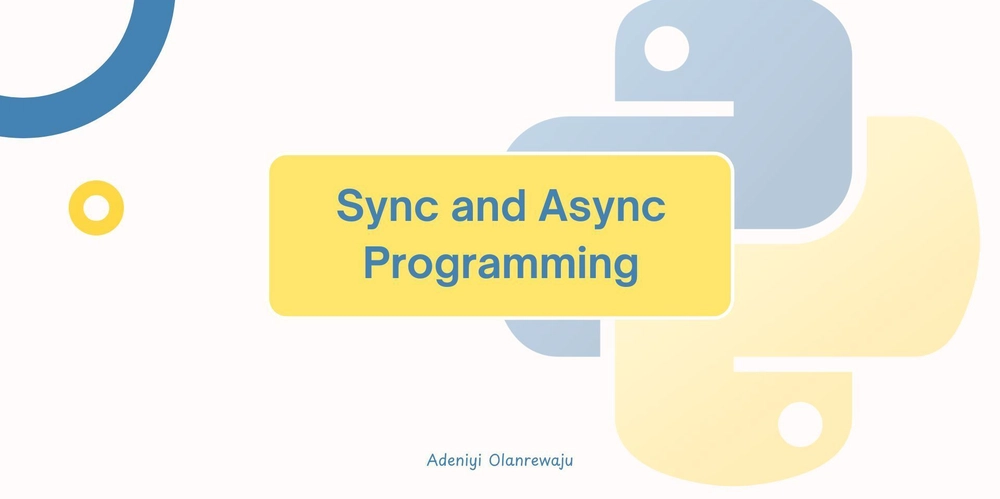
Introduction
When building applications, it’s important to know how tasks are handled. Python allows both synchronous and asynchronous programming, and each has its benefits and drawbacks. Choosing the right approach can make your application faster and more efficient. In this article, we will explain the difference between these two methods and look at the frameworks that support them in Python.
What is Synchronous Programming?
Synchronous programming means tasks run one after another. Each task must finish before the next one starts. This makes it simple to follow, but it can be slow when dealing with multiple operations.
Real-Life Example
Imagine you are at a bank, and there is only one cashier. Each customer must wait in line and can only be served after the previous customer is done. This is how synchronous programming works—one task at a time, in order.
Pros and Cons of Synchronous Programming
✅ Easy to understand and debug
✅ Simple to implement
❌ Can be slow when handling multiple tasks
❌ Blocks execution, causing delays in performance
Python Frameworks for Synchronous Programming
Flask – A simple web framework that processes requests one at a time.
Django (Default Mode) – Django processes requests synchronously unless modified.
Requests – A popular library for making HTTP requests, but it runs synchronously.
What is Asynchronous Programming?
Asynchronous programming allows multiple tasks to run at the same time. This is useful for operations that involve waiting, such as database queries or API requests. Instead of blocking the whole program, asynchronous programming continues running other tasks while waiting for a response.
Real-Life Example
Imagine you are downloading multiple files from the internet. Instead of waiting for one file to finish before starting the next, your computer can download several files at the same time. You can even browse the internet or work on something else while the downloads continue in the background. This is how asynchronous programming works—multiple tasks can run independently without blocking each other.
Pros and Cons of Asynchronous Programming
✅ Ideal for tasks that involve waiting (e.g., API calls, database queries)
✅ Improves performance for applications with multiple users
✅ Helps build real-time applications like chat apps
❌ More complex to understand and debug
❌ Requires knowledge of event loops and coroutines
Python Frameworks for Asynchronous Programming
FastAPI – A high-performance web framework that runs fully asynchronously.
Django (With ASGI and Django Channels) – Supports async processing when configured with ASGI.
Asyncio – Python’s built-in library for handling asynchronous tasks.
When to Use Synchronous vs. Asynchronous Programming?
Use Synchronous Programming When:
Your application is simple and does not need to handle many tasks at once.
You are working with CPU-heavy tasks rather than waiting for responses.
You prefer a straightforward approach that is easy to debug.
Use Asynchronous Programming When:
Your application involves making lots of API calls or database queries.
You need to handle many users at the same time without slowing down.
You are building real-time applications like chat apps or live notifications.
Conclusion
Choosing between synchronous and asynchronous programming depends on your project. Synchronous programming is easier to use, but asynchronous programming is better for handling multiple tasks at once.
Frameworks like Flask and Django (default) work well for simple applications, while FastAPI, Django with ASGI are better for handling multiple users at once.
Understanding these two approaches will help you build better applications that perform well. If you have experience with synchronous or asynchronous frameworks, feel free to share your thoughts in the comments!