Mastering Next.js API Caching: Improve Performance with Middleware and Headers
In the previous post, we explored Incremental Static Regeneration (ISR) in Next.js, learning how to achieve a perfect balance between static site performance and dynamic data freshness. While ISR provides a powerful way to manage page-level caching, it's just one piece of the larger caching puzzle. When building performant web applications, it's crucial to not overlook caching your API routes. API routes often serve dynamic data fetched from databases, CMS platforms, or third-party services. Without effective caching, every API request triggers fresh database queries or external calls, leading to unnecessary latency, increased server load, and potentially higher operational costs. By implementing proper caching strategies for your API routes, you can dramatically improve your app's performance, reduce server strain, and deliver content faster to your users. In this post, we'll cover: How to effectively cache API responses using Cache-Control headers. How Next.js Middleware can be leveraged to manage caching at scale. Real-world examples and best practices to implement caching smartly and efficiently. Let's dive in and learn how to harness the power of Next.js API caching for optimized performance. Why Cache API Routes? Before jumping into the how-to, it’s essential to understand the importance of caching API routes in Next.js. Every time a client requests data from an API route, the server executes a series of potentially expensive operations, such as database queries, external API calls, or computation-heavy logic. Without caching, each request leads to repeated execution of these operations—even if the data rarely changes. This pattern can quickly result in increased latency, unnecessary server load, and higher operational expenses, particularly for popular routes with frequent traffic. Benefits of Caching API Responses: Reduced Latency: Caching significantly cuts down response times since previously retrieved data can be served immediately, bypassing time-consuming operations. Lower Server Load: By serving cached responses, your server can handle more concurrent requests without becoming overwhelmed. Optimized External API Usage: APIs often come with rate limits or costs per call. Caching responses helps reduce the frequency of external calls, saving costs and minimizing rate limit issues. Real-life Scenario: Imagine an e-commerce platform fetching product data from a database every single time a user visits the product listing page. If product information changes infrequently (perhaps only once per hour), repeatedly hitting the database with every request is inefficient. Instead, caching API responses allows visitors to receive the data instantly, while the server fetches updated information in the background at defined intervals. By intelligently caching your API responses, you ensure a smoother, faster, and more reliable user experience while keeping your infrastructure costs manageable. Next, let’s dive into implementing caching using Cache-Control headers in your Next.js API routes. Setting Cache-Control Headers in API Routes One of the simplest and most effective ways to cache API responses in Next.js is through HTTP headers—particularly the Cache-Control header. This powerful header instructs both browsers and Content Delivery Networks (CDNs) on how to cache and serve your API responses. Basic Setup Here's a fundamental example of setting the Cache-Control header in a Next.js API route: export default async function handler(req, res) { const data = await fetchDataFromDB(); res.setHeader('Cache-Control', 'public, s-maxage=60, stale-while-revalidate=120'); res.status(200).json(data); } Understanding the Headers: Let’s break down what each directive means in detail: public Indicates the response can safely be cached by browsers and CDNs. Use this for general, non-user-specific data. s-maxage=60 Sets the cache lifetime specifically for CDN caches (such as Vercel's Edge Network or Cloudflare). In this example, CDNs will cache responses for 60 seconds. stale-while-revalidate=120 This directive instructs the CDN to serve cached responses (even if expired) for an additional 120 seconds while fetching and updating the cache in the background. Users receive cached data immediately, resulting in consistently fast response times. How it Works with CDNs: When deployed on platforms like Vercel or other CDN-enabled infrastructures, this setup operates as follows: First request: The API fetches data directly from your server or database and caches it at the CDN edge. Subsequent requests (within 60 seconds): Users immediately receive the response cached by the CDN—no server query required. Request after cache expiry (after 60 seconds but within the 120-second stale period): The CDN still serves the cached (now stale) data instantly while simultaneously fetching fresh data from your AP
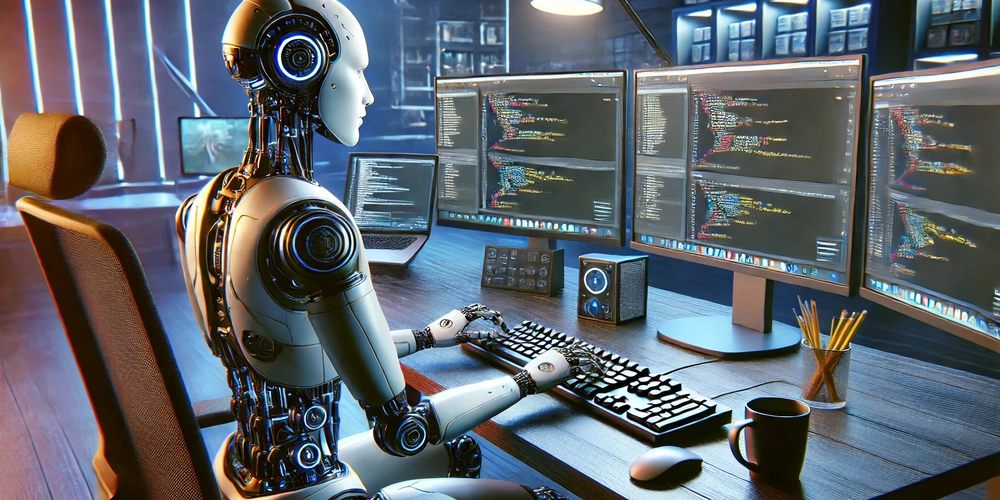
In the previous post, we explored Incremental Static Regeneration (ISR) in Next.js, learning how to achieve a perfect balance between static site performance and dynamic data freshness. While ISR provides a powerful way to manage page-level caching, it's just one piece of the larger caching puzzle.
When building performant web applications, it's crucial to not overlook caching your API routes. API routes often serve dynamic data fetched from databases, CMS platforms, or third-party services. Without effective caching, every API request triggers fresh database queries or external calls, leading to unnecessary latency, increased server load, and potentially higher operational costs.
By implementing proper caching strategies for your API routes, you can dramatically improve your app's performance, reduce server strain, and deliver content faster to your users.
In this post, we'll cover:
- How to effectively cache API responses using
Cache-Control
headers. - How Next.js Middleware can be leveraged to manage caching at scale.
- Real-world examples and best practices to implement caching smartly and efficiently.
Let's dive in and learn how to harness the power of Next.js API caching for optimized performance.
Why Cache API Routes?
Before jumping into the how-to, it’s essential to understand the importance of caching API routes in Next.js.
Every time a client requests data from an API route, the server executes a series of potentially expensive operations, such as database queries, external API calls, or computation-heavy logic. Without caching, each request leads to repeated execution of these operations—even if the data rarely changes. This pattern can quickly result in increased latency, unnecessary server load, and higher operational expenses, particularly for popular routes with frequent traffic.
Benefits of Caching API Responses:
-
Reduced Latency:
Caching significantly cuts down response times since previously retrieved data can be served immediately, bypassing time-consuming operations.
-
Lower Server Load:
By serving cached responses, your server can handle more concurrent requests without becoming overwhelmed.
-
Optimized External API Usage:
APIs often come with rate limits or costs per call. Caching responses helps reduce the frequency of external calls, saving costs and minimizing rate limit issues.
Real-life Scenario:
Imagine an e-commerce platform fetching product data from a database every single time a user visits the product listing page. If product information changes infrequently (perhaps only once per hour), repeatedly hitting the database with every request is inefficient. Instead, caching API responses allows visitors to receive the data instantly, while the server fetches updated information in the background at defined intervals.
By intelligently caching your API responses, you ensure a smoother, faster, and more reliable user experience while keeping your infrastructure costs manageable.
Next, let’s dive into implementing caching using Cache-Control
headers in your Next.js API routes.
Setting Cache-Control
Headers in API Routes
One of the simplest and most effective ways to cache API responses in Next.js is through HTTP headers—particularly the Cache-Control
header. This powerful header instructs both browsers and Content Delivery Networks (CDNs) on how to cache and serve your API responses.
Basic Setup
Here's a fundamental example of setting the Cache-Control
header in a Next.js API route:
export default async function handler(req, res) {
const data = await fetchDataFromDB();
res.setHeader('Cache-Control', 'public, s-maxage=60, stale-while-revalidate=120');
res.status(200).json(data);
}
Understanding the Headers:
Let’s break down what each directive means in detail:
-
public
Indicates the response can safely be cached by browsers and CDNs. Use this for general, non-user-specific data.
-
s-maxage=60
Sets the cache lifetime specifically for CDN caches (such as Vercel's Edge Network or Cloudflare). In this example, CDNs will cache responses for 60 seconds.
-
stale-while-revalidate=120
This directive instructs the CDN to serve cached responses (even if expired) for an additional 120 seconds while fetching and updating the cache in the background. Users receive cached data immediately, resulting in consistently fast response times.
How it Works with CDNs:
When deployed on platforms like Vercel or other CDN-enabled infrastructures, this setup operates as follows:
-
First request:
The API fetches data directly from your server or database and caches it at the CDN edge.
-
Subsequent requests (within 60 seconds):
Users immediately receive the response cached by the CDN—no server query required.
-
Request after cache expiry (after 60 seconds but within the 120-second stale period):
The CDN still serves the cached (now stale) data instantly while simultaneously fetching fresh data from your API route in the background.
Using this method effectively reduces latency and server load, ensuring your application remains swift and scalable even during traffic spikes.
Next, we'll apply these concepts practically by looking at an example use case: caching a products API route.
Example Use Case: Caching a Products API
To truly understand the power of API caching, let’s explore a practical, real-world example. Suppose you’re building an e-commerce website. Your product list changes infrequently, perhaps every 5–10 minutes. Without caching, each visitor to your site would trigger a fresh database call, leading to slower responses and higher server load.
Let’s fix this inefficiency by implementing a smart caching strategy with Next.js API routes using the Cache-Control
header.
Practical Implementation
Here's how you might set up caching for your /api/products
route:
// pages/api/products.js
export default async function handler(req, res) {
const products = await fetchProductsFromDatabase();
res.setHeader(
'Cache-Control',
'public, s-maxage=300, stale-while-revalidate=600'
);
res.status(200).json(products);
}
Breaking Down the Caching Strategy
-
public
Indicates responses are safe for caching by both browsers and CDNs.
-
s-maxage=300
(5 minutes)The CDN caches responses for 5 minutes. Any visitor accessing
/api/products
within this period receives a cached version instantly. -
stale-while-revalidate=600
(10 minutes)After the initial 5-minute cache expiry, the CDN serves the cached (but stale) data instantly for an additional 10 minutes. Simultaneously, it fetches fresh data in the background. Once fetched, the CDN cache is updated seamlessly.
Result and Benefits
With this setup, here's what your users experience:
-
Initial request:
Data is fetched directly from your database and cached at the CDN level.
-
Subsequent requests (within 5 minutes):
Visitors receive lightning-fast responses from the CDN cache, significantly reducing load times and backend requests.
-
After cache expiry (5–15 minutes period):
Visitors still experience instant responses (cached stale data), while fresh product data updates silently behind the scenes. This ensures visitors always see content quickly, while also maintaining data freshness.
By implementing caching this way, your application becomes highly performant and responsive, improving user experience and reducing infrastructure costs.
In the next section, we'll take caching efficiency even further by exploring how Next.js Middleware can help you apply caching strategies more systematically across your entire API surface.
Leveraging Middleware for Global API Caching Rules
Manually setting caching headers for each individual API route can quickly become tedious and error-prone, especially as your application scales. Next.js Middleware solves this elegantly, allowing you to apply caching policies globally or selectively across your API routes in a clean, centralized manner.
Middleware intercepts requests to your routes, enabling you to modify headers, responses, or even rewrite requests before they reach your actual API handlers.
Practical Middleware Example: Global API Caching
Imagine you have several public API endpoints under /api/public/*
that you want to cache consistently. Instead of repeating caching logic across each route, use middleware to centralize this:
Step-by-step Middleware Setup:
1. Create a Middleware file (middleware.js
or middleware.ts
at the root of your project):
// middleware.js
import { NextResponse } from 'next/server';
export function middleware(request) {
const response = NextResponse.next();
if (request.nextUrl.pathname.startsWith('/api/public/')) {
response.headers.set(
'Cache-Control',
'public, s-maxage=120, stale-while-revalidate=240'
);
}
return response;
}
2. Explanation of Middleware Logic:
-
Condition:
Checks whether the incoming request path starts with
/api/public/
. -
Caching Headers:
-
public
: Safe for CDN and browser caching. -
s-maxage=120
: Cache responses at the CDN edge for 2 minutes. -
stale-while-revalidate=240
: After expiry, serves stale cached data for an additional 4 minutes while fetching fresh data in the background.
-
Benefits of Middleware for Caching:
-
Centralized Management:
Modify caching rules easily from a single location, avoiding repetition and potential inconsistencies.
-
Flexible Scalability:
Easily extend your caching strategy to new API endpoints or change existing ones without touching individual routes.
-
Cleaner Code:
Separating concerns means your actual API route handlers remain clean and focused solely on their primary logic.
Using Middleware this way ensures your API caching logic remains clean, maintainable, and scalable. It significantly simplifies managing caching policies, especially for larger projects.
In the next section, we'll cover the differences between various caching directives (Cache-Control
, no-cache
, private
) and understand exactly when to use each to maximize performance and security.
Cache-Control vs No-Cache vs Private: Know When to Use What
To truly master API caching in Next.js, it’s crucial to clearly understand the different caching headers available. Choosing the right header directives ensures data freshness, security, and optimal performance.
Below is a breakdown of the primary directives you’ll encounter:
Cache-Control Directives Explained:
-
public
- Meaning: Response can be cached by CDNs and browsers.
- Use Case: Suitable for general, non-sensitive data like blogs, products, or public info.
-
private
- Meaning: Response should be cached only by the client’s browser, never by shared caches (CDNs).
- Use Case: Ideal for user-specific responses like dashboards, profiles, or user preferences.
-
no-store
- Meaning: Completely disables caching; responses must always be freshly fetched.
- Use Case: Sensitive, dynamic, or frequently changing data (authentication routes, payments, real-time data).
-
no-cache
- Meaning: Allows caching, but cached data must be validated with the server each time.
- Use Case: Data needing freshness checks each time (useful but less common in API routes).
-
s-maxage
- Meaning: Sets cache expiration explicitly for CDN caches (e.g., Vercel Edge, Cloudflare).
- Use Case: Critical for controlling CDN-level caching separately from browser caching.
-
stale-while-revalidate
- Meaning: Allows serving stale cached data while revalidating in the background.
- Use Case: Ensures users always get quick responses, even during cache regeneration.
Quick Reference Table
Directive | Suitable Scenario | Example Routes |
---|---|---|
public |
General public data, safe for caching |
/api/blog , /api/products
|
private |
User-specific data | /api/user/profile |
no-store |
Highly sensitive or dynamic data |
/api/auth , /api/payment
|
no-cache |
Needs freshness checks every request | Rarely used in API context |
s-maxage |
CDN-level caching, separate from browsers | /api/public/* |
stale-while-revalidate |
Background cache updates | Commonly combined with s-maxage
|
Best Practices:
- Always use
private
orno-store
for sensitive or user-specific data. - Combine
public
withs-maxage
andstale-while-revalidate
to serve general data efficiently through CDNs. - Clearly define caching strategies based on the specific nature and sensitivity of each endpoint.
- Regularly audit your caching headers—misconfiguration can lead to stale data, security vulnerabilities, or performance degradation.
Understanding and correctly implementing these cache headers is essential for achieving a performant and secure Next.js application.
In the upcoming section, we'll explore how combining API caching with ISR and SSG strategies can further enhance your application's performance and scalability.
Combining API Caching with ISR and SSG
Up until now, we've primarily focused on caching API responses using headers and middleware. However, Next.js's full potential shines when you combine API caching with page-level caching strategies like Incremental Static Regeneration (ISR) and Static Site Generation (SSG).
By layering these caching methods, you achieve blazing-fast performance and optimal resource management.
Practical Implementation: Layered Caching Strategy
Imagine you're building a blog platform where your frontend uses ISR to keep blog posts fresh and fast. Instead of directly fetching from your database within getStaticProps
, you fetch data from your cached API routes. Here's how you might set this up practically:
Step 1: Cache your API route with Headers
// pages/api/posts.js
export default async function handler(req, res) {
const posts = await fetchPostsFromDatabase();
res.setHeader(
'Cache-Control',
'public, s-maxage=300, stale-while-revalidate=600'
);
res.status(200).json(posts);
}
Step 2: Consume the Cached API in ISR (or SSG)
Now, utilize your cached API route within the ISR-enabled page:
// pages/blog.js
export async function getStaticProps() {
const res = await fetch('https://yourdomain.com/api/posts');
const posts = await res.json();
return {
props: { posts },
revalidate: 300, // ISR revalidates every 5 minutes
};
}
Why Layered Caching Works So Well:
-
API Layer Caching:
Reduces database hits, improving API responsiveness and decreasing server load.
-
ISR or SSG Layer Caching:
Generates pages statically at build-time or incrementally, minimizing runtime computation.
-
Double Performance Gains:
Your static pages load instantly from CDN caches, while your API responses are served rapidly from the CDN or browser cache. This combination dramatically enhances the overall speed of your application.
Real-world Benefits:
- Significantly reduces infrastructure costs by decreasing database load.
- Provides faster initial load times and improved SEO benefits due to fully static pages.
- Allows for easy scalability, gracefully handling traffic spikes without degrading performance.
By strategically combining these caching layers, you ensure your Next.js applications deliver exceptional performance and scalability, setting your project apart from traditional dynamic applications.
Next, let's explore common pitfalls around cache invalidation and how to avoid them to maintain data consistency and user trust.
Cache Invalidation Gotchas
While API caching offers tremendous performance gains, incorrect handling of cache invalidation can introduce frustrating problems like serving outdated data or unintentionally revealing sensitive information. Let's explore common pitfalls and how to avoid them.
Common Cache Invalidation Pitfalls:
1. Serving Stale Data for Too Long
When using directives like stale-while-revalidate
, cached data continues to serve during regeneration. If misconfigured (setting excessively long stale durations), users might see outdated content longer than intended.
Solution:
Carefully choose appropriate caching durations based on data update frequency. Regularly monitor and adjust as needed.
2. Misconfigured CDN and Browser Caches
Sometimes developers mistakenly set caching headers that lead to unexpected behavior in browsers or CDNs, causing confusing inconsistencies in content freshness.
Solution:
- Explicitly use
s-maxage
to control CDN cache duration. - Use browser-specific directives like
max-age
separately if needed. - Always test header behavior thoroughly during development (tools like
curl -I
, Postman, or browser DevTools are invaluable here).
3. Forgetting to Version API Routes
When you change your API schema or data structure significantly, cached responses might still deliver outdated payloads to users.
Solution:
- Implement API route versioning (e.g.,
/api/v1/products
,/api/v2/products
). - Clearly define cache durations per version to seamlessly transition users to new data.
4. Accidentally Caching Sensitive Data
Applying caching headers incorrectly can accidentally expose user-specific or private data via CDN caches.
Solution:
- Always mark user-specific responses as
private
orno-store
. - Regularly audit routes handling sensitive information.
5. Incorrect Middleware Usage
Misconfiguring middleware can inadvertently apply caching globally, including routes intended to remain dynamic or private.
Solution:
- Carefully define middleware conditions (
pathname.startsWith('/api/public/')
) to ensure caching rules apply only to intended routes. - Clearly document middleware logic for team clarity.
Best Practices to Avoid Invalidation Issues:
- Monitor production caching behavior with logging tools, CDNs analytics, or observability solutions to quickly detect caching anomalies.
- Regularly audit caching headers and middleware logic during code reviews and before deployments.
-
Test extensively using browser tools or HTTP request tools (
curl -I
, Postman) to verify expected caching behaviors.
By understanding and carefully addressing these cache invalidation challenges, you'll maintain trust with your users, deliver consistently fresh data, and ensure your Next.js app remains both performant and reliable.
In the next section, we'll wrap up with essential pro tips and best practices to further sharpen your Next.js API caching skills.
Pro Tips & Best Practices
To wrap up your mastery of API caching in Next.js, let's consolidate key tips and proven best practices that experienced developers use to achieve consistent performance, scalability, and maintainability in their applications.
Key Tips for API Caching Excellence:
1. Combine s-maxage
and stale-while-revalidate
Strategically
- Always pair these two directives for optimal caching.
-
Example for general use-cases:
res.setHeader('Cache-Control', 'public, s-maxage=120, stale-while-revalidate=240');
2. Centralize Caching Logic with Middleware
- Middleware simplifies caching policy management and scales effortlessly as your app grows.
- Use middleware selectively to avoid accidentally caching private endpoints.
3. Monitor and Audit Your Caches
- Regularly inspect caching headers in production to ensure they behave as expected.
- Tools like CDN dashboards, logging services, or observability platforms (e.g., Datadog, New Relic) are invaluable.
4. Separate Public and Private APIs Clearly
- Clearly differentiate between public, cacheable routes and private, non-cacheable routes.
-
Always mark private endpoints with:
res.setHeader('Cache-Control', 'private, no-store');
5. Leverage Versioning for API Stability
- Version your API routes (e.g.,
/api/v1/...
,/api/v2/...
) to safely roll out breaking changes without causing cache invalidation issues.
6. Combine API Caching with ISR and SSG for Maximum Performance
- Cache API responses at the CDN layer.
- Fetch from cached APIs during ISR or SSG, doubling performance benefits.
7. Explicitly Control Browser and CDN Caches Separately
-
Use
max-age
for browser caches ands-maxage
for CDN caches when you need distinct caching behaviors for browsers and CDNs:
res.setHeader('Cache-Control', 'public, max-age=60, s-maxage=120, stale-while-revalidate=240');
Things to Avoid:
-
❌ Avoid overly aggressive caching:
Long cache durations may lead to stale data being served longer than users expect.
-
❌ Never cache sensitive or personalized data:
This includes authentication responses, user data, or sensitive transactional information.
-
❌ Avoid blindly applying global middleware:
Carefully apply middleware conditions to prevent caching unintended routes.
Following these best practices ensures your Next.js apps will deliver consistently fast experiences, optimized infrastructure costs, and reliable caching behavior.
In our concluding section, we'll summarize everything we've covered and preview what's coming next in our caching series.
Conclusion
In this post, we've taken an in-depth look at how mastering API caching in Next.js can significantly boost your application's performance and scalability. By thoughtfully implementing caching strategies with headers and middleware, you can ensure fast response times, reduce unnecessary server load, and enhance user experience dramatically.
Key Takeaways:
- API caching is essential for reducing latency and optimizing performance.
- Using
Cache-Control
headers correctly can drastically enhance how your API responds to traffic. - Next.js Middleware offers a scalable, maintainable way to manage caching logic across multiple routes.
- Clearly understanding cache invalidation pitfalls and applying best practices helps maintain data freshness and consistency.
- Combining API caching with ISR and SSG provides powerful, layered caching strategies, maximizing both performance and reliability.
What's Next?
In our next post, we'll delve deeper into enhancing API caching performance by integrating Redis into your Next.js application. You'll learn how to achieve lightning-fast response times for frequently accessed data through Redis caching.
Stay tuned for:
Post 5: Using Redis with Next.js for Lightning-Fast API Responses.
Catch Up on Previous Posts:
- Post 1: Understanding Caching in Next.js
- Post 2: Static vs Dynamic Caching in Next.js
- Post 3: Implementing Revalidation with ISR
Stay Connected:
Feel free to reach out with feedback or questions as we continue this caching series.