Supercharging Your Observability: Integrating Logrus with Grafana Loki
In the world of microservices and distributed systems, effective logging is not just a convenience—it's a necessity. Today, I'll walk you through integrating Grafana Loki with the popular Go logging library logrus to create a powerful, searchable logging system that will dramatically improve your observability capabilities. The Two Paths to Loki Integration When it comes to sending logs to a Grafana dashboard using Loki, there are two primary approaches: Using Promtail: This agent collects container logs and forwards them to Loki Using a direct hook with logrus: This captures log messages and sends them directly to the Grafana dashboard over HTTP In this guide, we'll focus on the second approach for its simplicity and direct control over what gets logged. Understanding Logrus Hooks: The Magic Behind the Scenes If you are already familiar with logrus hooks you can skip to Finding the Right Hook Implementation Before diving into the implementation, let's understand what hooks are in Logrus and why they're so powerful. What is a Hook in Logrus? A hook in Logrus is essentially an extension point that lets you execute additional actions whenever a log entry is created. Hooks implement a simple interface that includes methods like Fire() (which is called when a log entry is created) and Levels() (which defines which log levels this hook should be triggered for). type Hook interface { Levels() []Level Fire(*Entry) error } This simple but powerful interface allows Logrus to remain focused on core logging functionality while enabling virtually unlimited extensibility. In our specific case with Loki, a hook allows us to intercept log entries as they're created and immediately send them to Loki via its HTTP API, without changing how we interact with the logger in our application code. Finding the Right Hook Implementation After extensive research (and quite a few cups of coffee), I found that while there's no official Golang package specifically for Loki-logrus integration, there are several community options. The most robust and trusted appears to be YuKitsune/lokirus, which provides a reliable hook implementation that's widely used in production environments. Understanding Loki's Label-Based Log Organization Before diving into code, it's important to understand that Loki organizes logs using labels, similar to how Prometheus handles metrics. This approach makes logs highly searchable and filterable, allowing you to quickly drill down to what matters. For our implementation, we'll include these critical labels in our logs: Log level (info, warning, error, critical) Request path HTTP method Message content Request body (when needed for debugging) Request duration A unique identifier for request tracing Implementation: The Complete Solution Let's build a robust logging system step by step. Step 1: Creating a Request Tracer Middleware First, we'll create a middleware for our Gin-based API that captures important request details and adds them to the context: func RequestTracerMiddleware() gin.HandlerFunc { return func(c *gin.Context) { // Capture request start time for duration calculation startTime := time.Now() // Extract basic request information method := c.Request.Method path := c.Request.URL.Path // Capture request body while preserving it for further use bodyBytes, _ := io.ReadAll(c.Request.Body) c.Request.Body = io.NopCloser(bytes.NewBuffer(bodyBytes)) // Store all captured information in the context c.Set("requestBody", string(bodyBytes)) c.Set("path", path) c.Set("method", method) c.Set("start_time", startTime.UnixMilli()) // Continue request processing c.Next() } } This middleware captures HTTP method, path, request body, and the start time of the request. By storing these in the Gin context, we can access them later in our logging code. Step 2: Creating a Logger Interface Next, we'll define a clean logging interface that abstracts away the implementation details: package logging import ( "context" "github.com/sirupsen/logrus" "github.com/yukitsune/lokirus" ) // Logger defines the standard logging interface. type Logger interface { Debug(ctx context.Context, format string, args ...interface{}) Info(ctx context.Context, format string, args ...interface{}) Warn(ctx context.Context, format string, args ...interface{}) Error(ctx context.Context, format string, args ...interface{}) } This interface provides a clean abstraction that will be easy to use throughout your application. Step 3: Initializing the Logger with Loki Hook Now, let's create a function to initialize our logger with the Loki hook: func New(logtag string) Logger { // Configure the Loki hook with appropriate options opts := lokirus.NewLokiHookOptions().
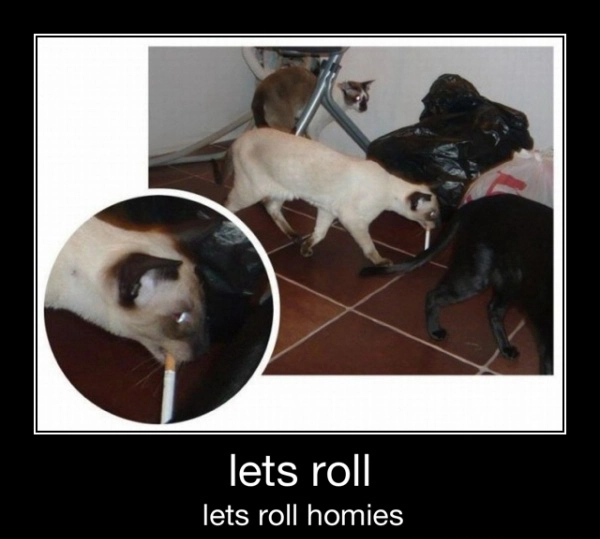
In the world of microservices and distributed systems, effective logging is not just a convenience—it's a necessity. Today, I'll walk you through integrating Grafana Loki with the popular Go logging library logrus to create a powerful, searchable logging system that will dramatically improve your observability capabilities.
The Two Paths to Loki Integration
When it comes to sending logs to a Grafana dashboard using Loki, there are two primary approaches:
- Using Promtail: This agent collects container logs and forwards them to Loki
- Using a direct hook with logrus: This captures log messages and sends them directly to the Grafana dashboard over HTTP
In this guide, we'll focus on the second approach for its simplicity and direct control over what gets logged.
Understanding Logrus Hooks: The Magic Behind the Scenes
If you are already familiar with logrus hooks you can skip to Finding the Right Hook Implementation Before diving into the implementation, let's understand what hooks are in Logrus and why they're so powerful.
What is a Hook in Logrus?
A hook in Logrus is essentially an extension point that lets you execute additional actions whenever a log entry is created. Hooks implement a simple interface that includes methods like Fire()
(which is called when a log entry is created) and Levels()
(which defines which log levels this hook should be triggered for).
type Hook interface {
Levels() []Level
Fire(*Entry) error
}
This simple but powerful interface allows Logrus to remain focused on core logging functionality while enabling virtually unlimited extensibility.
In our specific case with Loki, a hook allows us to intercept log entries as they're created and immediately send them to Loki via its HTTP API, without changing how we interact with the logger in our application code.
Finding the Right Hook Implementation
After extensive research (and quite a few cups of coffee), I found that while there's no official Golang package specifically for Loki-logrus integration, there are several community options. The most robust and trusted appears to be YuKitsune/lokirus, which provides a reliable hook implementation that's widely used in production environments.
Understanding Loki's Label-Based Log Organization
Before diving into code, it's important to understand that Loki organizes logs using labels, similar to how Prometheus handles metrics. This approach makes logs highly searchable and filterable, allowing you to quickly drill down to what matters.
For our implementation, we'll include these critical labels in our logs:
- Log level (info, warning, error, critical)
- Request path
- HTTP method
- Message content
- Request body (when needed for debugging)
- Request duration
- A unique identifier for request tracing
Implementation: The Complete Solution
Let's build a robust logging system step by step.
Step 1: Creating a Request Tracer Middleware
First, we'll create a middleware for our Gin-based API that captures important request details and adds them to the context:
func RequestTracerMiddleware() gin.HandlerFunc {
return func(c *gin.Context) {
// Capture request start time for duration calculation
startTime := time.Now()
// Extract basic request information
method := c.Request.Method
path := c.Request.URL.Path
// Capture request body while preserving it for further use
bodyBytes, _ := io.ReadAll(c.Request.Body)
c.Request.Body = io.NopCloser(bytes.NewBuffer(bodyBytes))
// Store all captured information in the context
c.Set("requestBody", string(bodyBytes))
c.Set("path", path)
c.Set("method", method)
c.Set("start_time", startTime.UnixMilli())
// Continue request processing
c.Next()
}
}
This middleware captures HTTP method, path, request body, and the start time of the request. By storing these in the Gin context, we can access them later in our logging code.
Step 2: Creating a Logger Interface
Next, we'll define a clean logging interface that abstracts away the implementation details:
package logging
import (
"context"
"github.com/sirupsen/logrus"
"github.com/yukitsune/lokirus"
)
// Logger defines the standard logging interface.
type Logger interface {
Debug(ctx context.Context, format string, args ...interface{})
Info(ctx context.Context, format string, args ...interface{})
Warn(ctx context.Context, format string, args ...interface{})
Error(ctx context.Context, format string, args ...interface{})
}
This interface provides a clean abstraction that will be easy to use throughout your application.
Step 3: Initializing the Logger with Loki Hook
Now, let's create a function to initialize our logger with the Loki hook:
func New(logtag string) Logger {
// Configure the Loki hook with appropriate options
opts := lokirus.NewLokiHookOptions().
// Map logrus panic level to Grafana's critical level
// See: https://grafana.com/docs/grafana/latest/explore/logs-integration/
WithLevelMap(lokirus.LevelMap{logrus.PanicLevel: "critical"}).
WithFormatter(&logrus.JSONFormatter{}).
WithStaticLabels(lokirus.Labels{
"job": "BE_SERVER1", // Service identifier
"tag": logtag, // Custom tag for grouping related logs
})
// Create the Loki hook with specified log levels
hook := lokirus.NewLokiHookWithOpts(
"http://127.0.0.1:3100", // Loki endpoint
opts,
logrus.InfoLevel,
logrus.WarnLevel,
logrus.ErrorLevel,
logrus.FatalLevel)
// Create and configure the logrus logger
l := logrus.New()
l.AddHook(hook)
l.SetFormatter(&logrus.JSONFormatter{})
// Return our implementation
return &LogrusLogger{
logger: l,
}
}
In this initialization:
- We configure a Loki hook that will intercept log entries
- We specify which log levels should trigger the hook (info, warn, error, fatal)
- We set up static labels that will be attached to every log entry
- We properly map logrus levels to Grafana levels
- We attach the hook to our logger instance
The beauty of this approach is that our logger will continue to function normally, writing logs to its default output (usually stdout), and it will also send those logs to Loki. If Loki is temporarily unavailable, our application will continue logging locally without issues.
Step 4: Implementing the Logger Methods
Finally, let's implement the actual logging methods that will extract context information and format our logs:
package logging
import (
"context"
"fmt"
"time"
"github.com/sirupsen/logrus"
)
// LogrusLogger implements the Logger interface using Logrus.
type LogrusLogger struct {
logger *logrus.Logger
fields logrus.Fields
}
// Debug logs at debug level.
func (l *LogrusLogger) Debug(ctx context.Context, format string, args ...interface{}) {
entry := l.createEntry(ctx)
entry.Debugf(format, args...)
}
// Info logs at info level.
func (l *LogrusLogger) Info(ctx context.Context, format string, args ...interface{}) {
entry := l.createEntry(ctx)
entry.Infof(format, args...)
}
// Warn logs at warn level.
func (l *LogrusLogger) Warn(ctx context.Context, format string, args ...interface{}) {
entry := l.createEntry(ctx)
entry.Warnf(format, args...)
}
// Error logs at error level.
func (l *LogrusLogger) Error(ctx context.Context, format string, args ...interface{}) {
entry := l.createEntry(ctx)
entry.Errorf(format, args...)
}
// createEntry creates a logrus entry with fields from context.
func (l *LogrusLogger) createEntry(ctx context.Context) *logrus.Entry {
fields := copyFields(l.fields)
// Add request ID for tracing
if requestID, ok := ctx.Value("requestID").(string); ok {
fields["request_id"] = requestID
}
// Add HTTP request body for detailed debugging
if requestBody, ok := ctx.Value("requestBody").(string); ok {
fields["request_body"] = requestBody
}
// Add path for request identification
if path, ok := ctx.Value("path").(string); ok {
fields["path"] = path
}
// Add HTTP method for request type
if method, ok := ctx.Value("method").(string); ok {
fields["method"] = method
}
// Calculate request duration for performance monitoring
if startTime, ok := ctx.Value("start_time").(int64); ok {
duration := time.Now().UnixMilli() - startTime
fields["duration"] = fmt.Sprintf("%dms", duration)
}
return l.logger.WithFields(fields)
}
// copyFields creates a copy of the fields map to avoid modifying the original.
func copyFields(fields logrus.Fields) logrus.Fields {
newFields := logrus.Fields{}
for k, v := range fields {
newFields[k] = v
}
return newFields
}
The implementation:
- Provides methods for different log levels
- Extracts useful information from the context
- Formats it all with appropriate labels
- Calculates request duration for performance monitoring
When any of these logging methods are called, our Loki hook will intercept the log entry and send it to Grafana Loki, complete with all the fields and context we've added.
Using the Logger in Your Application
With our implementation complete, using it in your application is straightforward:
// Initialize at application startup
logger := logging.New("api-service")
// Use in your request handlers
func HandleRequest(c *gin.Context) {
// Create a context from the Gin context
ctx := c.Request.Context()
// Log request information
logger.Info(ctx, "Processing request")
// Your business logic here
// Log completion
logger.Info(ctx, "Request completed successfully")
}
Configuring Grafana to Display Your Logs
Once your application is sending logs to Loki, you'll need to:
- Add Loki as a data source in Grafana
- Create a dashboard with a Logs panel
- Configure the query to filter by your service labels
Here's a simple Loki query example to get you started:
{job="BE_SERVER1"} | json
This will pull all logs from your service and parse them as JSON. You can then add filters:
{job="BE_SERVER1"} | json | path=~"/api/v1/.*" | duration > "100ms"
This query finds all requests to API v1 endpoints that took longer than 100ms to process—perfect for identifying slow endpoints!
Advanced Tips and Tricks
1. Custom Hook Logic for Special Cases
You can create your own custom hooks when you need special handling:
type CustomHook struct {
// Configuration fields here
}
func (h *CustomHook) Fire(entry *logrus.Entry) error {
// Custom processing logic
return nil
}
func (h *CustomHook) Levels() []logrus.Level {
return []logrus.Level{logrus.ErrorLevel, logrus.FatalLevel}
}
This pattern is particularly useful for integrating with internal systems or implementing specialized processing for certain types of logs.
2. Log Aggregation with Logical Operators
Loki supports logical operators for complex queries:
{job="BE_SERVER1"} | json | level="error" or level="warn"
3. Smart Sampling for High-Volume Services
For high-traffic services, consider sampling your logs:
if rand.Float64() < 0.1 { // Log 10% of requests
logger.Info(ctx, "Sampled request details", "full_details", true)
}
4. Structured Logging for Enhanced Searchability
Always prefer structured logging over string concatenation:
// Good
logger.Info(ctx, "User authentication", "user_id", userID, "result", "success")
// Avoid
logger.Info(ctx, fmt.Sprintf("User %s authenticated successfully", userID))
The structured approach makes it much easier to filter and search logs later.
Conclusion
With this implementation, you now have a powerful, searchable logging system that integrates seamlessly with Grafana and Loki. By leveraging Logrus hooks, we've established a clean separation between application logic and logging infrastructure, making our system more maintainable and flexible.
The hook-based approach provides:
- Centralized log storage and visualization
- Powerful querying capabilities
- Rich context for each log entry
- Performance metrics embedded in logs
- Minimal impact on application performance
Remember to adjust your Grafana dashboard's IP address and service name based on your specific environment. Happy logging!