Static method and Non Static method
`A static method is one that belongs to a class instead of an instance of a class A static method is a part of the class itself rather than an instance of a class. The method is available to each instance of the class. Static methods can be accessed directly in non-static and static methods Static methods are accessed through the class name, rather than an object of the class ` public class Student{ //static keyword static int studentRollNo=1234; String studentName="jeyalakshmi"; public static void main(String args[]){ System.out.println(studentRollNo); Student name=new Student(); System.out.println(name.studentName); System.out.println(name.studentRollNo); } } public class BankAccount{ int accountNo=1000; static String accountName="jeyaLakshmi"; void deposit(){ System.out.println("deposits Succesfully"); } static void creditCartd(){ System.out.println("CreditCardSucessfully"); } public static void main(String args[]){ BankAccount bc=new BankAccount(); bc.creditCartd(); bc.deposit(); System.out.println(bc.accountNo); System.out.println(accountName); } } public class Library{ //staic variable static String name="jeya"; int price=1000; //Static methods static void libraryPlace(){ System.out.println("libraryPlace"); } void booksCount(){ System.out.println("BooksCount"); } public static void main(String args[]){ Library lib=new Library(); //varible call System.out.println(lib.price); System.out.println(lib.name); libraryPlace(); lib.booksCount(); } } `
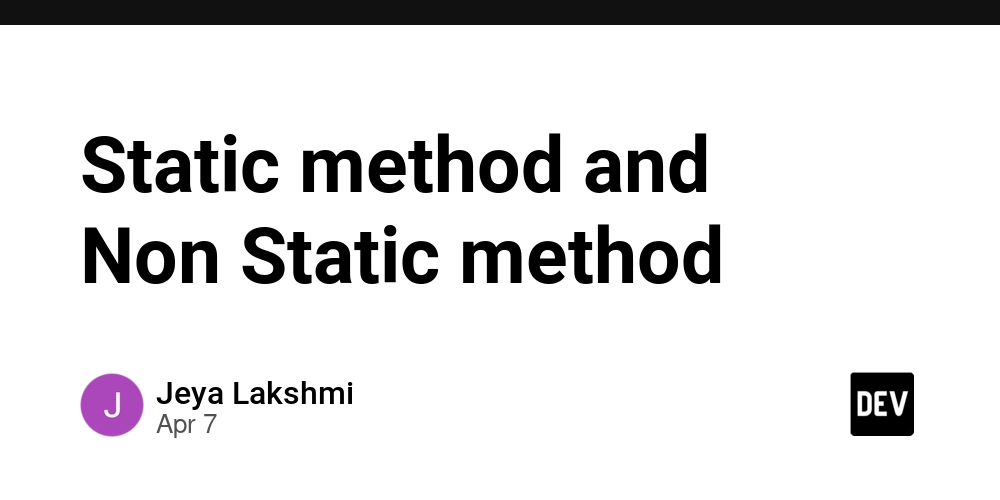
`A static method is one that belongs to a class instead of an instance of a class
A static method is a part of the class itself rather than an instance of a class.
The method is available to each instance of the class.
Static methods can be accessed directly in non-static and static methods
Static methods are accessed through the class name, rather than an object of the class
`
public class Student{
//static keyword
static int studentRollNo=1234;
String studentName="jeyalakshmi";
public static void main(String args[]){
System.out.println(studentRollNo);
Student name=new Student();
System.out.println(name.studentName);
System.out.println(name.studentRollNo);
}
}
public class BankAccount{
int accountNo=1000;
static String accountName="jeyaLakshmi";
void deposit(){
System.out.println("deposits Succesfully");
}
static void creditCartd(){
System.out.println("CreditCardSucessfully");
}
public static void main(String args[]){
BankAccount bc=new BankAccount();
bc.creditCartd();
bc.deposit();
System.out.println(bc.accountNo);
System.out.println(accountName);
}
}
public class Library{
//staic variable
static String name="jeya";
int price=1000;
//Static methods
static void libraryPlace(){
System.out.println("libraryPlace");
}
void booksCount(){
System.out.println("BooksCount");
}
public static void main(String args[]){
Library lib=new Library();
//varible call
System.out.println(lib.price);
System.out.println(lib.name);
libraryPlace();
lib.booksCount();
}
}
`