Spring Security’s Silent Rules That Break Your App
If you’ve ever added Spring Security to your project and immediately thought: "Why is nothing working?!" …you’re not alone. Spring Security is powerful, but its default behavior can be confusing. After debugging countless issues (and reading one too many Stack Overflow threads), I’ve compiled some of the basic mistakes I made — the kind many beginners are likely to make as well. The Silent Lockdown: Why All Your Endpoints Return 401 Problem: You add spring-boot-starter-security, and suddenly every request gets blocked. Why? Spring Security defaults to securing all endpoints. Fix: (Gradually tighten security starting with permitAll()) @Bean SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception { http.authorizeHttpRequests(auth -> auth.anyRequest().permitAll()); return http.build(); } CSRF & Stateless APIs: The Hidden 403 Forbidden Problem: Your POST requests fail even with valid tokens. Why? CSRF protection is enabled by default (good for traditional apps, bad for APIs). Fix: http.csrf(csrf -> csrf.disable()); When to keep CSRF? Only for session-based apps. CORS Errors: Why @CrossOrigin Isn’t Enough Problem: Your frontend gets blocked despite using @CrossOrigin. Why? Spring Security overrides CORS settings. Fix: Configure it in SecurityFilterChain http.cors(cors -> cors.configurationSource(request -> { CorsConfiguration config = new CorsConfiguration(); config.setAllowedOrigins(List.of("http://localhost:3000")); config.setAllowedMethods(List.of("*")); return config; })); Final Thoughts Spring Security’s defaults are secure—but that also means they’re restrictive. The key is understanding why things break before changing them. What’s your biggest Spring Security struggle? Did I miss any common pitfalls? Let’s discuss in the comments!
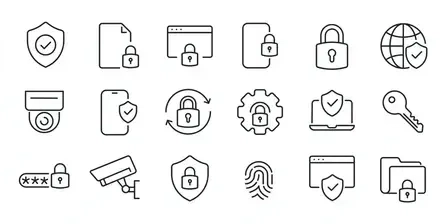
If you’ve ever added Spring Security to your project and immediately thought:
"Why is nothing working?!"
…you’re not alone.
Spring Security is powerful, but its default behavior can be confusing. After debugging countless issues (and reading one too many Stack Overflow threads), I’ve compiled some of the basic mistakes I made — the kind many beginners are likely to make as well.
The Silent Lockdown: Why All Your Endpoints Return 401
Problem: You add spring-boot-starter-security, and suddenly every request gets blocked.
Why? Spring Security defaults to securing all endpoints.
Fix: (Gradually tighten security starting with permitAll())
@Bean
SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http.authorizeHttpRequests(auth -> auth.anyRequest().permitAll());
return http.build();
}
CSRF & Stateless APIs: The Hidden 403 Forbidden
Problem: Your POST requests fail even with valid tokens.
Why? CSRF protection is enabled by default (good for traditional apps, bad for APIs).
Fix:
http.csrf(csrf -> csrf.disable());
When to keep CSRF? Only for session-based apps.
CORS Errors: Why @CrossOrigin Isn’t Enough
Problem: Your frontend gets blocked despite using @CrossOrigin.
Why? Spring Security overrides CORS settings.
Fix: Configure it in SecurityFilterChain
http.cors(cors -> cors.configurationSource(request -> {
CorsConfiguration config = new CorsConfiguration();
config.setAllowedOrigins(List.of("http://localhost:3000"));
config.setAllowedMethods(List.of("*"));
return config;
}));
Final Thoughts
Spring Security’s defaults are secure—but that also means they’re restrictive. The key is understanding why things break before changing them.
What’s your biggest Spring Security struggle? Did I miss any common pitfalls? Let’s discuss in the comments!