SKT: Types, Numbers, Booleans, and Strings
In the modern world of app and web development, three languages are making waves: Swift, Kotlin, and TypeScript (SKT). While each has its own domain (iOS, Android, and Web, respectively), they share fundamental concepts, especially when it comes to basic data types. Let's take a quick tour through numbers, booleans, and strings in these powerful languages. Types: The Foundation All three languages are statically typed, meaning you explicitly (or implicitly) define the type of a variable. This helps catch errors early and improves code readability. Swift: Known for its type safety, Swift strongly encourages explicit type declarations. Kotlin: Like Swift, Kotlin is statically typed and offers type inference, reducing boilerplate. TypeScript: Built on JavaScript, TypeScript adds static typing, making large JavaScript projects more manageable. Numbers: Handling Numerical Data Numbers are essential for calculations and data manipulation. Swift: Swift provides various numeric types: Int (integers), Double (floating-point numbers), and Float. let integerValue: Int = 10 let doubleValue: Double = 3.14 Kotlin: Kotlin's numeric types are similar to Java's: Int, Double, Float, Long, Short, and Byte. val integerValue: Int = 10 val doubleValue: Double = 3.14 TypeScript: TypeScript has a single numeric type: number, which can represent both integers and floating-point values. let numericValue: number = 10; let floatValue: number = 3.14; Booleans: Representing Truth Booleans represent true or false values, crucial for conditional logic. Swift: Swift uses Bool for boolean values. let isTrue: Bool = true let isFalse: Bool = false Kotlin: Kotlin uses Boolean. val isTrue: Boolean = true val isFalse: Boolean = false TypeScript: TypeScript uses boolean. let isTrue: boolean = true; let isFalse: boolean = false; Strings: Working with Text Strings represent sequences of characters. Swift: Swift uses String for text. let message: String = "Hello, Swift!" Kotlin: Kotlin uses String. val message: String = "Hello, Kotlin!" TypeScript: TypeScript uses string. let message: string = "Hello, TypeScript!"; Common Ground, Different Paths While Swift, Kotlin, and TypeScript cater to different platforms, their handling of basic data types reflects a shared understanding of fundamental programming concepts. Understanding these similarities makes it easier to transition between these powerful languages.
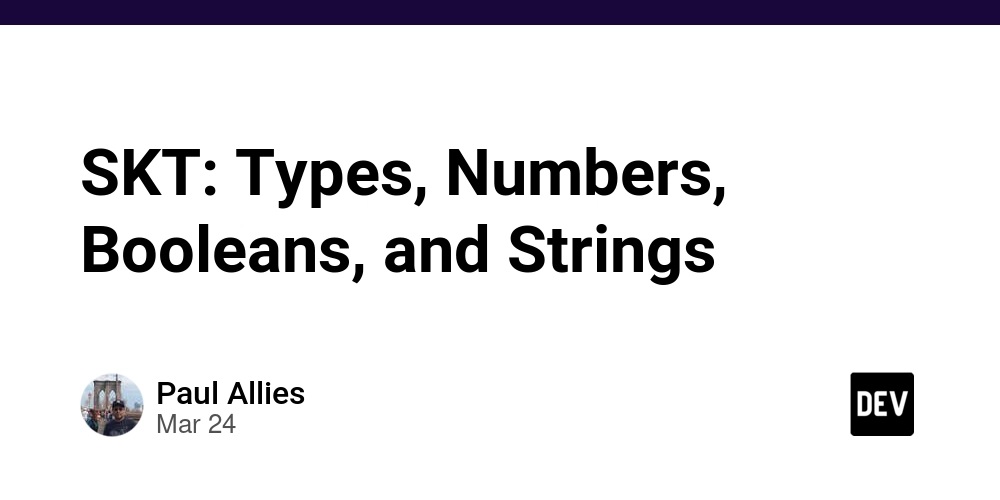
In the modern world of app and web development, three languages are making waves: Swift, Kotlin, and TypeScript (SKT). While each has its own domain (iOS, Android, and Web, respectively), they share fundamental concepts, especially when it comes to basic data types. Let's take a quick tour through numbers, booleans, and strings in these powerful languages.
Types: The Foundation
All three languages are statically typed, meaning you explicitly (or implicitly) define the type of a variable. This helps catch errors early and improves code readability.
Swift: Known for its type safety, Swift strongly encourages explicit type declarations.
Kotlin: Like Swift, Kotlin is statically typed and offers type inference, reducing boilerplate.
TypeScript: Built on JavaScript, TypeScript adds static typing, making large JavaScript projects more manageable.
Numbers: Handling Numerical Data
Numbers are essential for calculations and data manipulation.
Swift:
Swift provides various numeric types: Int
(integers), Double
(floating-point numbers), and Float
.
let integerValue: Int = 10
let doubleValue: Double = 3.14
Kotlin:
Kotlin's numeric types are similar to Java's: Int
, Double
, Float
, Long
, Short
, and Byte
.
val integerValue: Int = 10
val doubleValue: Double = 3.14
TypeScript:
TypeScript has a single numeric type: number
, which can represent both integers and floating-point values.
let numericValue: number = 10;
let floatValue: number = 3.14;
Booleans: Representing Truth
Booleans represent true or false values, crucial for conditional logic.
Swift:
Swift uses Bool
for boolean values.
let isTrue: Bool = true
let isFalse: Bool = false
Kotlin:
Kotlin uses Boolean
.
val isTrue: Boolean = true
val isFalse: Boolean = false
TypeScript:
TypeScript uses boolean
.
let isTrue: boolean = true;
let isFalse: boolean = false;
Strings: Working with Text
Strings represent sequences of characters.
Swift:
Swift uses String
for text.
let message: String = "Hello, Swift!"
Kotlin:
Kotlin uses String
.
val message: String = "Hello, Kotlin!"
TypeScript:
TypeScript uses string
.
let message: string = "Hello, TypeScript!";
Common Ground, Different Paths
While Swift, Kotlin, and TypeScript cater to different platforms, their handling of basic data types reflects a shared understanding of fundamental programming concepts. Understanding these similarities makes it easier to transition between these powerful languages.