Shell Scripting Back to Basics
Shell Scripting 1. Variables naming conventions recommended file="filename.txt" no spaces allowed between '=' house_color, source_file constants written in UpperCase Example: read only FILE="filename.txt" varialbe outside of scope to be used then use exports ### 2. Functions: functions can start with function keyword or without it. calculate_area() { } clone_repo() { } Expanding Variables The below both versions works well. #!/bin/bash var="value of var" echo ${var} #!/bin/bash var="value of var" echo $var The difference come when appending additional string in the end. Expanding variables with {} should be used based on need. #!/bin/bash height=70 echo "Your height is = $heightcm" # throws error echo "Your height is = ${height}cm" # works good Without the quotes the variable string will split by field seperators here space. #!/bin/bash string="One Two Three" for element in ${string}; do echo ${element} # or echo $element done O/P: One Two Three Now try enclosing with the double quotes. The string will not split out and will be act as entire string as one element. #!/bin/bash string="One Two Three" for element in "${string}"; do echo ${element} # or echo $element done O/P: One Two Three For the below scenario we want server names seperately. #!/bin/bash readonly SERVERS="server1 server2 server3" for server in ${SERVERS}; do echo "${server}.worker-node" done O/P: server1.worker-node server2.worker-node server3.worker-node Recommended to use "" expanding variables: Directory paths, filenames Assigning URLs to variables When in doubt, use double quotes :) Let revisit basic concepts of linux commands, loops, performing tasks. Shell scripting is much more powerfull. It follows the top down approach and only when we can it gets executed ( calling functions). # list items ls pwd cd mkdir Lets say there is some command will throws the errors to break the script over there we have declare "set -x" in the top of script. Usage of [[ ]] for conditional controls. #!/bin/bash if [[ 3 > 4 ]]; then echo " three greater than four"; fi Shell script has 4 loop types While for until similar to do-while loop While loop #!/bin/bash i=1 while [[ $i -le 3 ]]; do echo "Iteration $i" i=$((i+1)) done For loop for i in {1..3}; do # for i in $(seq 1 3); do using the sequence as sub-shell the output from it is treated input to i echo "Iteration $i" done Until Loop #!/bin/bash i=3 until [[ $i -eq 0 ]]; do echo "Iteration $i" i=$((i-1)) done To import the other script file There were sometimes we need to import variables from other files(.sh) # conf file name="Ubuntu" #!/bin/bas source .conf # sourcing the conf file echo "${name}" what if the conf file doesnt exists. We need to checkpoint in the script #!/bin/bash readonly CONF_FILE=".conf" if [[ -f ${CONF_FILE} ]]; then source "${CONF_FILE}" else name="Bob" fi echo "${name}" exit 0
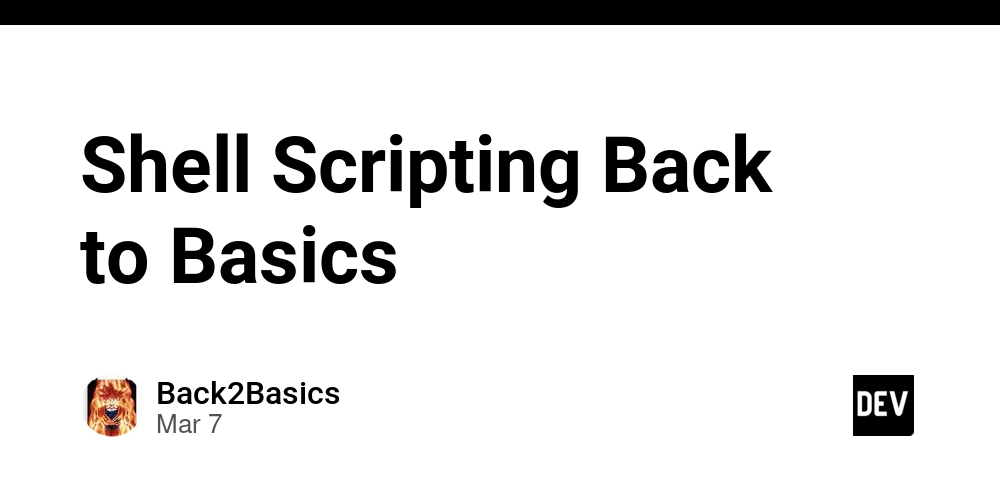
Shell Scripting
1. Variables naming conventions recommended
- file="filename.txt" no spaces allowed between '='
- house_color, source_file
- constants written in UpperCase Example: read only FILE="filename.txt"
- varialbe outside of scope to be used then use exports ### 2. Functions: functions can start with function keyword or without it.
calculate_area() {
}
clone_repo() {
}
Expanding Variables
The below both versions works well.
#!/bin/bash
var="value of var"
echo ${var}
#!/bin/bash
var="value of var"
echo $var
The difference come when appending additional string in the end. Expanding variables with {} should be used based on need.
#!/bin/bash
height=70
echo "Your height is = $heightcm" # throws error
echo "Your height is = ${height}cm" # works good
Without the quotes the variable string will split by field seperators here space.
#!/bin/bash
string="One Two Three"
for element in ${string}; do
echo ${element} # or echo $element
done
O/P:
One
Two
Three
Now try enclosing with the double quotes. The string will not split out and will be act as entire string as one element.
#!/bin/bash
string="One Two Three"
for element in "${string}"; do
echo ${element} # or echo $element
done
O/P:
One Two Three
For the below scenario we want server names seperately.
#!/bin/bash
readonly SERVERS="server1 server2 server3"
for server in ${SERVERS}; do
echo "${server}.worker-node"
done
O/P:
server1.worker-node
server2.worker-node
server3.worker-node
Recommended to use "" expanding variables:
- Directory paths, filenames
- Assigning URLs to variables
- When in doubt, use double quotes :)
Let revisit basic concepts of linux commands, loops, performing tasks. Shell scripting is much more powerfull. It follows the top down approach and only when we can it gets executed ( calling functions).
# list items
ls
pwd
cd
mkdir
Lets say there is some command will throws the errors to break the script over there we have declare "set -x" in the top of script.
Usage of [[ ]] for conditional controls.
#!/bin/bash
if [[ 3 > 4 ]]; then
echo " three greater than four";
fi
Shell script has 4 loop types
- While
- for
- until similar to do-while loop
While loop
#!/bin/bash
i=1
while [[ $i -le 3 ]]; do
echo "Iteration $i"
i=$((i+1))
done
For loop
for i in {1..3}; do
# for i in $(seq 1 3); do using the sequence as sub-shell the output from it is treated input to i
echo "Iteration $i"
done
Until Loop
#!/bin/bash
i=3
until [[ $i -eq 0 ]]; do
echo "Iteration $i"
i=$((i-1))
done
To import the other script file
There were sometimes we need to import variables from other files(.sh)
# conf file
name="Ubuntu"
#!/bin/bas
source .conf # sourcing the conf file
echo "${name}"
what if the conf file doesnt exists. We need to checkpoint in the script
#!/bin/bash
readonly CONF_FILE=".conf"
if [[ -f ${CONF_FILE} ]]; then
source "${CONF_FILE}"
else
name="Bob"
fi
echo "${name}"
exit 0