SharedArrayBuffer and Atomics
SharedArrayBuffer and Atomics: A Comprehensive Exploration In the ever-evolving landscape of JavaScript and web technologies, managing concurrent operations has been an area of significant interest, particularly as web applications become increasingly complex and resource-heavy. Central to this pursuit is the SharedArrayBuffer and Atomics APIs, introduced in ECMAScript 2017 and designed to facilitate multi-threading in JavaScript using Web Workers. This article aims to provide an in-depth exploration of these two constructs, analyzing their history, technical mechanisms, applications, performance implications, and common pitfalls. Historical Context Before diving into SharedArrayBuffer and Atomics, it’s essential to understanding the evolution of concurrency in JavaScript. JavaScript, by design, is single-threaded; it executes code in a single sequence while using an event loop to handle asynchronous operations. However, with the introduction of Web Workers in HTML5, developers gained the ability to run scripts in background threads, cleverly sidestepping the single-threaded nature of JavaScript. Yet, up to the introduction of SharedArrayBuffer, communication between such workers was primarily achieved via postMessage, which utilized a copy of the data being sent — inherently limiting performance and efficiency. Introduction of SharedArrayBuffer SharedArrayBuffer, proposed as a solution, allows binary data buffers to be shared among multiple threads without requiring copies, which enables high-performance applications. This primitive allows for direct memory access, fostering efficient inter-thread communication. However, issues related to security, such as the Spectre vulnerabilities identified in 2018, led to the re-evaluation and, temporarily, the disabling of SharedArrayBuffer in various browsers. Nonetheless, with advancements in security measures, its usage has been cautiously re-enabled in certain contexts, particularly when combined with appropriate Cross-Origin-Opener-Policy (COOP) and Cross-Origin-Embedder-Policy (COEP) headers. Atomics – The Synchronization Backbone Accompanying SharedArrayBuffer is the Atomics object, providing a set of atomic operations that ensure data integrity when multiple threads access the same shared memory. The atomic operations facilitated by this object include operations for loading, storing, adding, subtracting, and comparing values without the risk of race conditions. Technical Overview Creating a SharedArrayBuffer At its core, a SharedArrayBuffer can be instantiated similarly to a regular ArrayBuffer. The primary difference lies in the ability to share this buffer across multiple execution contexts, such as different web workers. const sab = new SharedArrayBuffer(16); // 16 bytes const uint8View = new Uint8Array(sab); Enhancing with Atomics The Atomics module provides methods that perform atomic operations on SharedArrayBuffer views. These include: Atomics.add() Atomics.sub() Atomics.or() Atomics.and() Atomics.xor() Atomics.compareExchange() Atomics.exchange() Atomics.load() Atomics.store() Atomics.wait() Atomics.wake() Example Scenario: A Counter Implementation Consider a situation where multiple workers increment a shared counter. Below is an illustrative example. // worker.js const sab = new SharedArrayBuffer(4); const counter = new Int32Array(sab); function incrementCounter() { for (let i = 0; i
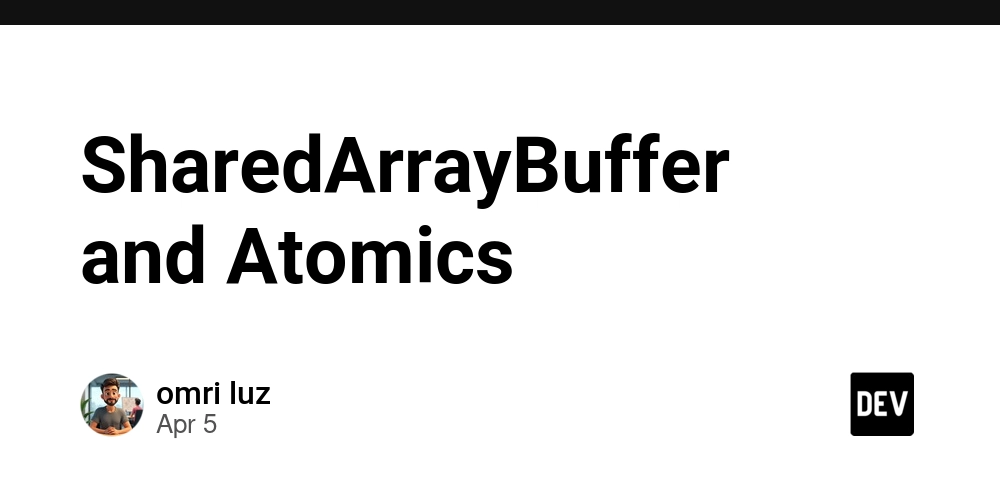
SharedArrayBuffer and Atomics: A Comprehensive Exploration
In the ever-evolving landscape of JavaScript and web technologies, managing concurrent operations has been an area of significant interest, particularly as web applications become increasingly complex and resource-heavy. Central to this pursuit is the SharedArrayBuffer
and Atomics
APIs, introduced in ECMAScript 2017 and designed to facilitate multi-threading in JavaScript using Web Workers. This article aims to provide an in-depth exploration of these two constructs, analyzing their history, technical mechanisms, applications, performance implications, and common pitfalls.
Historical Context
Before diving into SharedArrayBuffer
and Atomics
, it’s essential to understanding the evolution of concurrency in JavaScript. JavaScript, by design, is single-threaded; it executes code in a single sequence while using an event loop to handle asynchronous operations. However, with the introduction of Web Workers in HTML5, developers gained the ability to run scripts in background threads, cleverly sidestepping the single-threaded nature of JavaScript. Yet, up to the introduction of SharedArrayBuffer
, communication between such workers was primarily achieved via postMessage
, which utilized a copy of the data being sent — inherently limiting performance and efficiency.
Introduction of SharedArrayBuffer
SharedArrayBuffer
, proposed as a solution, allows binary data buffers to be shared among multiple threads without requiring copies, which enables high-performance applications. This primitive allows for direct memory access, fostering efficient inter-thread communication. However, issues related to security, such as the Spectre vulnerabilities identified in 2018, led to the re-evaluation and, temporarily, the disabling of SharedArrayBuffer
in various browsers. Nonetheless, with advancements in security measures, its usage has been cautiously re-enabled in certain contexts, particularly when combined with appropriate Cross-Origin-Opener-Policy (COOP) and Cross-Origin-Embedder-Policy (COEP) headers.
Atomics – The Synchronization Backbone
Accompanying SharedArrayBuffer
is the Atomics
object, providing a set of atomic operations that ensure data integrity when multiple threads access the same shared memory. The atomic operations facilitated by this object include operations for loading, storing, adding, subtracting, and comparing values without the risk of race conditions.
Technical Overview
Creating a SharedArrayBuffer
At its core, a SharedArrayBuffer
can be instantiated similarly to a regular ArrayBuffer
. The primary difference lies in the ability to share this buffer across multiple execution contexts, such as different web workers.
const sab = new SharedArrayBuffer(16); // 16 bytes
const uint8View = new Uint8Array(sab);
Enhancing with Atomics
The Atomics
module provides methods that perform atomic operations on SharedArrayBuffer
views. These include:
Atomics.add()
Atomics.sub()
Atomics.or()
Atomics.and()
Atomics.xor()
Atomics.compareExchange()
Atomics.exchange()
Atomics.load()
Atomics.store()
Atomics.wait()
Atomics.wake()
Example Scenario: A Counter Implementation
Consider a situation where multiple workers increment a shared counter. Below is an illustrative example.
// worker.js
const sab = new SharedArrayBuffer(4);
const counter = new Int32Array(sab);
function incrementCounter() {
for (let i = 0; i < 1000; i++) {
Atomics.add(counter, 0, 1); // Atomic increment
}
}
// Spawning multiple workers
for (let i = 0; i < navigator.hardwareConcurrency; i++) {
new Worker('worker.js');
}
// Main thread can read the value
console.log(Atomics.load(counter, 0)); // Outputs the final count after workers are finished
In this example, every worker increments the counter without risk of race conditions, thanks to atomicity.
Advanced Usage Patterns
While the preceding example demonstrates basic usage, advanced scenarios warrant deeper exploration, especially concerning the use of wait()
and wake()
methods which allows threads to sleep and be awakened, facilitating a robust producer-consumer model.
Example: Producer-Consumer Pattern
In a scenario where one thread produces data and another consumes it, we can utilize wait
and wake
.
const sab = new SharedArrayBuffer(4);
const buffer = new Int32Array(sab);
let index = 0;
let producer = new Worker('./producer.js');
let consumer = new Worker('./consumer.js');
// producer.js
function produce() {
while (true) {
Atomics.store(buffer, 0, index);
index++;
Atomics.wake(buffer, 0, 1); // Notifies the consumer
}
}
// consumer.js
function consume() {
while (true) {
Atomics.wait(buffer, 0, 0); // Wait for producer to update
const value = Atomics.load(buffer, 0);
console.log(`Consumed: ${value}`);
}
}
In this code, the producer updates the shared buffer while the consumer waits for new data, minimizing busy waiting.
Edge Cases and Advanced Techniques
Handling Race Conditions and Deadlocks
While atomic operations mitigate many typical threading issues, understanding potential race conditions and deadlocks is crucial. For instance, if a shared resource requires a combination of operations — such as first checking a condition before performing an operation, this may lead to undesirable states unless correctly managed. As a best practice, avoid holding multiple locks and defer locking until necessary.
Synchronization Patterns
Synchronization is critical in multi-threaded programming. Consider the examples of barriers or mutexes that allow threads to wait for each other to reach a certain point before proceeding. Implementing such mechanisms using Atomics
requires careful design, especially concerning memory visibility.
Performance Considerations
While SharedArrayBuffer
and Atomics
promise performance improvements, there are trade-offs. Key performance considerations include:
- Overhead of Atomic Operations: Atomic operations may introduce latency compared to non-atomic operations due to the need for memory synchronization.
-
Memory Management: Using
SharedArrayBuffer
efficiently requires a careful consideration of the size of the buffer to avoid excessive memory consumption, especially when scaling applications.
Optimization Strategies
To mitigate performance pitfalls:
- Minimize Contention: Design a system that minimizes shared states or reduces the number of atomic operations by using local copies where possible.
- Use Appropriate Views: Utilize typed arrays that align with the data types necessary for the application to reduce casting overhead.
Real-World Use Cases
The use of SharedArrayBuffer
and Atomics
spans across various industries. A prevalent use case lies in real-time applications such as online gaming, where multiple clients maintain a shared game state. Another example can be found in video processing or compression, where threads work together to handle large chunks of data in parallel for improved performance.
Debugging Tips
Debugging multi-threaded JavaScript can be challenging due to non-deterministic behavior. Consider the following strategies:
-
Log Notifications: Add extensive logging around
wait()
andwake()
calls to trace states and transitions. - Use Browser Debugger Tools: Modern browsers provide debugging tools for Web Workers allowing the inspection of performance bottlenecks and memory use.
- Simulate Concurrency Issues: Utilize testing frameworks to simulate race conditions under controlled scenarios to validate behavioral expectations.
Conclusion
The advent of SharedArrayBuffer
and Atomics
marks a significant evolution in the realm of JavaScript, enabling multi-threaded capabilities in a language historically bound to single-threaded environments. This complexity introduces new possibilities, encouraging developers to push the envelope of what's achievable within web applications while requiring a deeper understanding of concurrent programming principles.
As JavaScript continues to evolve, mastering SharedArrayBuffer
and Atomics
will be crucial for developers seeking to build efficient, high-performance applications that leverage the full potential of modern hardware.
References
- MDN Web Docs: SharedArrayBuffer
- MDN Web Docs: Atomics
- HTML Living Standard: SharedArrayBuffer
- Web Workers: Using the worker API
- Concurrency with Web Workers
- Concurrency Considerations
By engaging deeply with SharedArrayBuffer
and Atomics
, you sharpen your understanding of modern JavaScript's multi-threading capabilities, directly enhancing your ability to create cutting-edge applications.