Send Emails from Next.js with Resend and React Email
In this tutorial, we'll explore how to send emails from a Next.js application using Resend, including custom email templates, domain verification, scheduling, and file attachments. What are Resend and React Email? Resend is an email API service that allows developers to send emails from their applications with minimal setup. React Email provides components for building responsive email templates using React. You can check the video tutorial on the Cules Coding channel here: Project Setup Let's start with a basic Next.js application. Our project structure looks like this: src/ ├── actions/ │ └── sendEmail.js // Server action to handle email sending ├── app/ │ ├── page.jsx // Simple form to collect user input │ ├── layout.js // Root layout │ └── globals.css └── emails/ └── MyEmail.jsx // Email template component This structure separates our concerns nicely — server actions for backend logic, the app directory for Next.js pages, and a dedicated emails directory for our email templates. First, install the required packages: npm install resend @react-email/components react-email We're installing three packages: resend for the email API. @react-email/components for pre-built email components. react-email for the environment to preview our emails. Creating Email Templates with React Email React Email provides components for building responsive email templates. Let's create a template in src/emails/MyEmail.jsx: import { Tailwind, Body, Container, Head, Heading, Html, Img, Link, Preview, Section, Text, Font, } from '@react-email/components' const MyEmail = ({ name = 'Anjan' }) => { const previewText = 'Your free guide is here! Download now.' const downloadUrl = 'https://reallygreatsite.com/download' return ( Your Free Guide Is Here {previewText} {/* Email content here */} YOUR FREE GUIDE IS HERE {name.toUpperCase()}
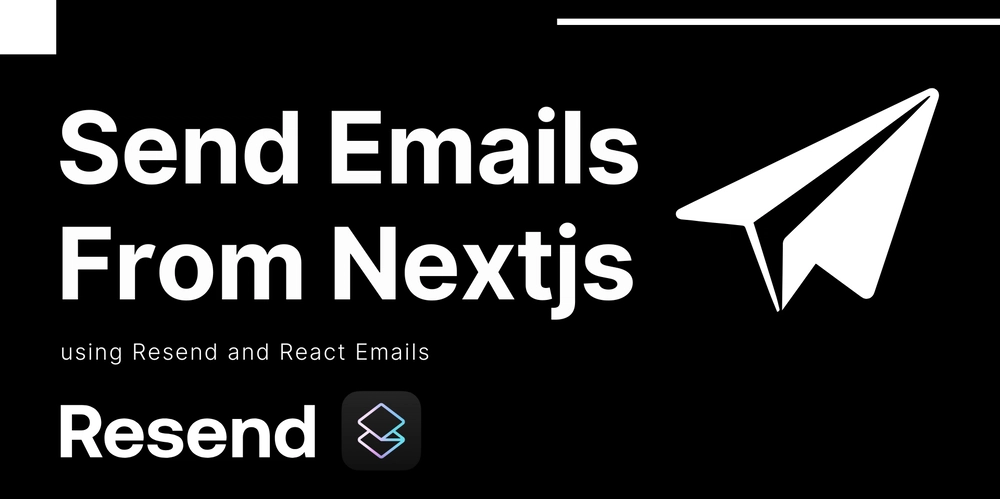
In this tutorial, we'll explore how to send emails from a Next.js application using Resend, including custom email templates, domain verification, scheduling, and file attachments.
What are Resend and React Email?
Resend is an email API service that allows developers to send emails from their applications with minimal setup. React Email provides components for building responsive email templates using React.
You can check the video tutorial on the Cules Coding channel here:
Project Setup
Let's start with a basic Next.js application. Our project structure looks like this:
src/
├── actions/
│ └── sendEmail.js // Server action to handle email sending
├── app/
│ ├── page.jsx // Simple form to collect user input
│ ├── layout.js // Root layout
│ └── globals.css
└── emails/
└── MyEmail.jsx // Email template component
This structure separates our concerns nicely — server actions for backend logic, the app directory for Next.js pages, and a dedicated emails directory for our email templates.
First, install the required packages:
npm install resend @react-email/components react-email
We're installing three packages:
- resend for the email API.
- @react-email/components for pre-built email components.
- react-email for the environment to preview our emails.
Creating Email Templates with React Email
React Email provides components for building responsive email templates. Let's create a template in src/emails/MyEmail.jsx
:
import {
Tailwind,
Body,
Container,
Head,
Heading,
Html,
Img,
Link,
Preview,
Section,
Text,
Font,
} from '@react-email/components'
const MyEmail = ({ name = 'Anjan' }) => {
const previewText = 'Your free guide is here! Download now.'
const downloadUrl = 'https://reallygreatsite.com/download'
return (
<Html>
<Tailwind>
<Head>
<title>Your Free Guide Is Heretitle>
<Font
fontFamily='Inter'
fallbackFontFamily='Arial'
webFont={{
url: 'https://fonts.googleapis.com/css2?family=Inter:wght@400;500;600;700;800&display=swap',
format: 'woff2',
}}
fontWeight={400}
fontStyle='normal'
/>
Head>
<Preview>{previewText}Preview>
<Body className="bg-[#e5e5e3] font-['Inter',Arial,sans-serif] py-10">
<Container className='mx-auto max-w-[600px] text-center'>
{/* Email content here */}
<Heading className="text-5xl leading-tight font-extrabold text-[#333333] my-8 font-['Inter',Arial,sans-serif] uppercase tracking-tight">
YOUR FREE GUIDE IS HERE {name.toUpperCase()}