Recursion is not a function calling itself!
I noticed the common definition of recursion that "It is a function calling itself" is wrong. Wait! Don't react yet. Hear me out first; is the function below a loop or a recursion? Note: If you want a video version of this explanation, watch What is recursion. function countdown(count) { console.log(count); if(count > 1) { count = count - 1; countdown(count); } else { return; }; }; // access function countdown(5); I know you're probably going to say it is a recursion because of the common definition but it is not — it is a loop. If you check the console, nothing will be repeated but the output of a recursion must be a repetition of a structure. Is recursion really a case of a function calling itself? I don't think so. I came to this realization when I was building koras.jsx that uses Constant Reactivity and it is implemented in a way that looks like it is recursive but it is not. I will get back to that later but let's focus on recursion first. Then, what is recursion if it is not a function calling itself? Before I define what a recursion is, it is important I define what a loop is for clarification. What is a loop? A loop is an operation that applies a process to a series or sequence of elements once until the last element (if any) or continue till infinity. That means a loop doesn't go back to reapply the same process to already processed elements. Now, let go back to the previous function so that you realize it is a loop: function countdown(count) { console.log(count); if(count > 1) { count = count - 1; countdown(count); } else { return; }; }; // access function countdown(5); You will notice that, even though the function calls itself to moves to the next line of action, it doesn't reapply the same process to the same element(s). If you log the count in the console, nothing will be recursive, that is, repeat the same index, structure or item(s). That means, you can implement a loop with a function. So a function calling itself is not a criterion to determine a recursion. Let's compare the function above with a while loop for clarification. let depth = 5; while(depth > 0){ console.log(depth) depth--; } The countdown function is very similar to the above while loop because they get similar results with similar structures. Can you see it is a loop? Okay. Did you say "How do you define and identify a recursion?"? Keep on reading. What is a recursion? It is a repetition of an operation on the same element(s) by always starting from the beginning to the end and then starting again, again and again until the stopping point (if any); if not, it continues till infinity. A recursion always repeats the same process on the same elements several times until the stopping point is reached or it continues till infinity. We have some examples of recursion in the real world. A good example are the days of the week or February 14. A week always starts from Monday; continues to Sunday and goes backs to Monday again, again and again. Here we are again; it is VAL
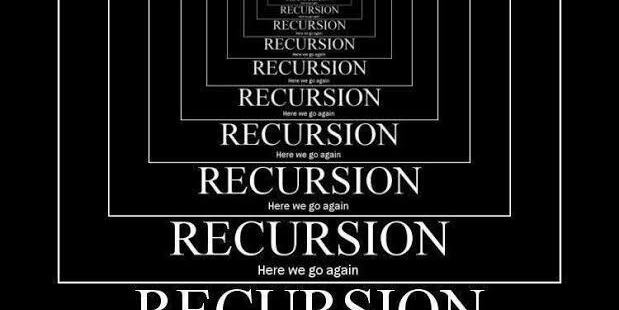
I noticed the common definition of recursion that "It is a function calling itself" is wrong.
Wait! Don't react yet. Hear me out first; is the function below a loop or a recursion?
Note: If you want a video version of this explanation, watch What is recursion.
function countdown(count) {
console.log(count);
if(count > 1) {
count = count - 1;
countdown(count);
} else {
return;
};
};
// access function
countdown(5);
I know you're probably going to say it is a recursion because of the common definition but it is not — it is a loop.
If you check the console, nothing will be repeated but the output of a recursion must be a repetition of a structure.
Is recursion really a case of a function calling itself? I don't think so.
I came to this realization when I was building koras.jsx that uses Constant Reactivity and it is implemented in a way that looks like it is recursive but it is not.
I will get back to that later but let's focus on recursion first. Then, what is recursion if it is not a function calling itself?
Before I define what a recursion is, it is important I define what a loop is for clarification.
What is a loop?
A loop is an operation that applies a process to a series or sequence of elements once until the last element (if any) or continue till infinity.
That means a loop doesn't go back to reapply the same process to already processed elements.
Now, let go back to the previous function so that you realize it is a loop:
function countdown(count) {
console.log(count);
if(count > 1) {
count = count - 1;
countdown(count);
} else {
return;
};
};
// access function
countdown(5);
You will notice that, even though the function calls itself to moves to the next line of action, it doesn't reapply the same process to the same element(s).
If you log the count in the console, nothing will be recursive, that is, repeat the same index, structure or item(s).
That means, you can implement a loop with a function. So a function calling itself is not a criterion to determine a recursion.
Let's compare the function above with a while loop for clarification.
let depth = 5;
while(depth > 0){
console.log(depth)
depth--;
}
The countdown function
is very similar to the above while loop because they get similar results with similar structures. Can you see it is a loop? Okay.
Did you say "How do you define and identify a recursion?"? Keep on reading.
What is a recursion?
It is a repetition of an operation on the same element(s) by always starting from the beginning to the end and then starting again, again and again until the stopping point (if any); if not, it continues till infinity.
A recursion always repeats the same process on the same elements several times until the stopping point is reached or it continues till infinity.
We have some examples of recursion in the real world. A good example are the days of the week or February 14.
A week always starts from Monday; continues to Sunday and goes backs to Monday again, again and again.
Here we are again; it is VAL