My Blackjack Terminal Game
Hello everyone, my name is Aaron! As a part of my Codecademy Portfolio Project: Python Terminal Game, I made a simple Blackjack game coded in python. I picked Blackjack because it's a fun game that I enjoy playing. It also helped me practice Object-Oriented Programming (OOP) in python. How It Works: Asks for the players name. You have $100 as your starting balance. Asks the player to place a bet. Deals the cards to you and the dealer. Lets the player hit or stand. Once the player stands, the dealer goes. Whoever is closer to 21 and doesn't go over, wins. If tied with dealer, you get your money back. What I've Learned: How to use classes and objects in Python. Working with lists and randomization. Writing clean and readable code. Creating a Github Repo and how to push my code. You can check the code on Github here: https://github.com/AaronASB/Blackjack-Terminal-Game/blob/main/Blackjack-Game.py *Here's a sample: * Code Snippet: Player Class Here's a look at the Player class that manages the user’s betting, and wins: python import random # The player class represents the player and the dealer. class Player: def __init__(self, name, balance): self.name = name self.balance = balance self.wins = 0 self.hand = [] #Placing a Bet def bet(self, amount): if amount > self.balance: return False #Not enough money to bet. self.balance -= amount return True #Win counter and adds to your balance when you win. def win(self, amount): self.balance += amount self.wins += 1 return "{name} wins ${amount}. New balance: ${balance}.".format( name=self.name, amount=amount, balance=self.balance )
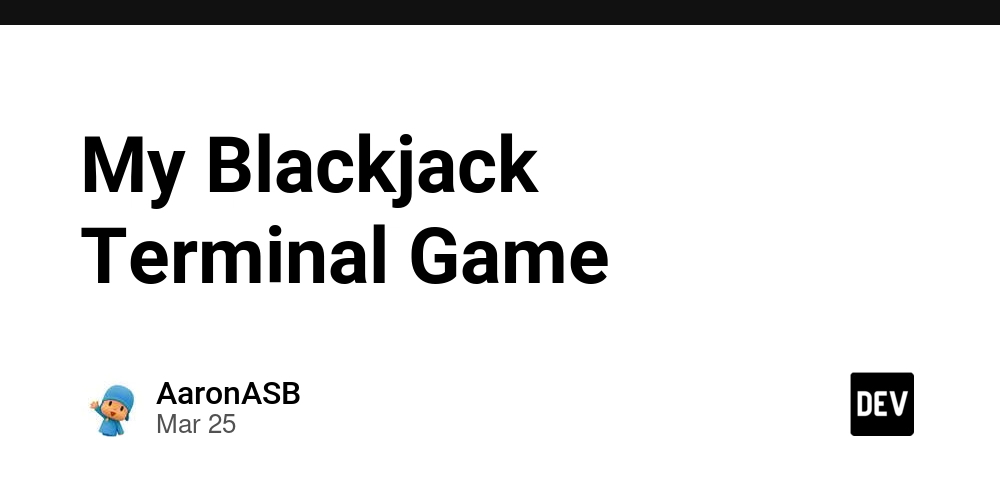
Hello everyone, my name is Aaron!
As a part of my Codecademy Portfolio Project: Python Terminal Game, I made a simple Blackjack game coded in python. I picked Blackjack because it's a fun game that I enjoy playing. It also helped me practice Object-Oriented Programming (OOP) in python.
How It Works:
- Asks for the players name.
- You have $100 as your starting balance.
- Asks the player to place a bet.
- Deals the cards to you and the dealer.
- Lets the player hit or stand.
- Once the player stands, the dealer goes.
- Whoever is closer to 21 and doesn't go over, wins.
- If tied with dealer, you get your money back.
What I've Learned:
- How to use classes and objects in Python.
- Working with lists and randomization.
- Writing clean and readable code.
- Creating a Github Repo and how to push my code.
You can check the code on Github here:
https://github.com/AaronASB/Blackjack-Terminal-Game/blob/main/Blackjack-Game.py
*Here's a sample: *
Code Snippet: Player Class
Here's a look at the Player
class that manages the user’s betting, and wins:
python
import random
# The player class represents the player and the dealer.
class Player:
def __init__(self, name, balance):
self.name = name
self.balance = balance
self.wins = 0
self.hand = []
#Placing a Bet
def bet(self, amount):
if amount > self.balance:
return False #Not enough money to bet.
self.balance -= amount
return True
#Win counter and adds to your balance when you win.
def win(self, amount):
self.balance += amount
self.wins += 1
return "{name} wins ${amount}. New balance: ${balance}.".format(
name=self.name,
amount=amount,
balance=self.balance
)