Realtime Collaboration App with WebSockets & Node.js
Realtime Collaboration App with WebSockets & Node.js Real-time collaboration tools have become a core part of our digital workspace. Whether it's working on a shared document, editing a whiteboard, or coding in sync, real-time communication is essential. In this blog, we will explore how to build a Realtime Collaboration App using WebSockets with Node.js, focusing on a shared whiteboard or document editor powered by Socket.IO. What is Real-Time Collaboration? Real-time collaboration enables multiple users to interact with the same digital resource simultaneously. This could mean co-editing text, drawing on a shared canvas, or viewing changes live as they happen. Key features of real-time collaboration include: Low latency updates Synchronization across clients Event handling and broadcasting Conflict resolution Why Use WebSockets and Socket.IO? WebSockets WebSockets provide a full-duplex communication channel over a single TCP connection, ideal for low-latency, real-time apps. Socket.IO Socket.IO is a library built on top of WebSockets that simplifies real-time communication and includes: Auto-reconnection Room support Event-based communication Cross-browser support System Architecture Overview Frontend (Web): Users interact with a whiteboard or document editor Backend (Node.js + Socket.IO): Manages WebSocket connections Broadcasts updates to all clients in the same room Optional Database: Save history or states of the whiteboard or document Use Case Example: Shared Whiteboard We will build a collaborative whiteboard where multiple users can draw in real-time and see each other's input live. Step-by-Step Implementation 1. Initialize Node.js Project mkdir collaborative-whiteboard cd collaborative-whiteboard npm init -y npm install express socket.io 2. Setup the Backend (index.js) const express = require('express'); const http = require('http'); const { Server } = require('socket.io'); const app = express(); const server = http.createServer(app); const io = new Server(server); app.use(express.static('public')); io.on('connection', (socket) => { console.log('User connected:', socket.id); socket.on('draw', (data) => { socket.broadcast.emit('draw', data); // Send to all except sender }); socket.on('clear', () => { io.emit('clear'); // Send to all clients }); }); server.listen(3000, () => console.log('Server running on http://localhost:3000')); 3. Frontend (public/index.html) Collaborative Whiteboard canvas { border: 1px solid #000; } Clear const socket = io(); const canvas = document.getElementById('board'); const ctx = canvas.getContext('2d'); let drawing = false; canvas.addEventListener('mousedown', () => drawing = true); canvas.addEventListener('mouseup', () => drawing = false); canvas.addEventListener('mousemove', draw); function draw(e) { if (!drawing) return; const x = e.offsetX; const y = e.offsetY; ctx.lineTo(x, y); ctx.stroke(); socket.emit('draw', { x, y }); } socket.on('draw', (data) => { ctx.lineTo(data.x, data.y); ctx.stroke(); }); function clearCanvas() { ctx.clearRect(0, 0, canvas.width, canvas.height); socket.emit('clear'); } socket.on('clear', () => ctx.clearRect(0, 0, canvas.width, canvas.height)); Extending to a Document Editor To build a collaborative document editor: Replace the canvas with a or contenteditable Emit input or keydown events with content On the backend, broadcast content to all clients Use versioning to manage concurrent changes (CRDTs or OT) Example event handling: socket.on('text-update', (text) => { socket.broadcast.emit('text-update', text); }); And on the frontend: textarea.addEventListener('input', () => { socket.emit('text-update', textarea.value); }); socket.on('text-update', (text) => { textarea.value = text; }); Bonus: Room Support Rooms allow you to isolate users into separate collaborative sessions. socket.on('join-room', (roomId) => { socket.join(roomId); socket.on('draw', (data) => { socket.to(roomId).emit('draw', data); }); }); Deployment Tips Use HTTPS (WebSockets require secure connections in production) Use a WebSocket-compatible server (like NGINX with WS support) Scale using Redis or socket.io-redis for multi-node setups Conclusion With WebSockets and Node.js, building real-time collaborative applications like a shared whiteboard or document editor is approachable and powerful. Socket.IO simplifies many of the tricky aspects, allowing developers to focus on UX and functionality. From classrooms to remote teams, these tools create interactive, synchronous environments where ideas can flow instantly.
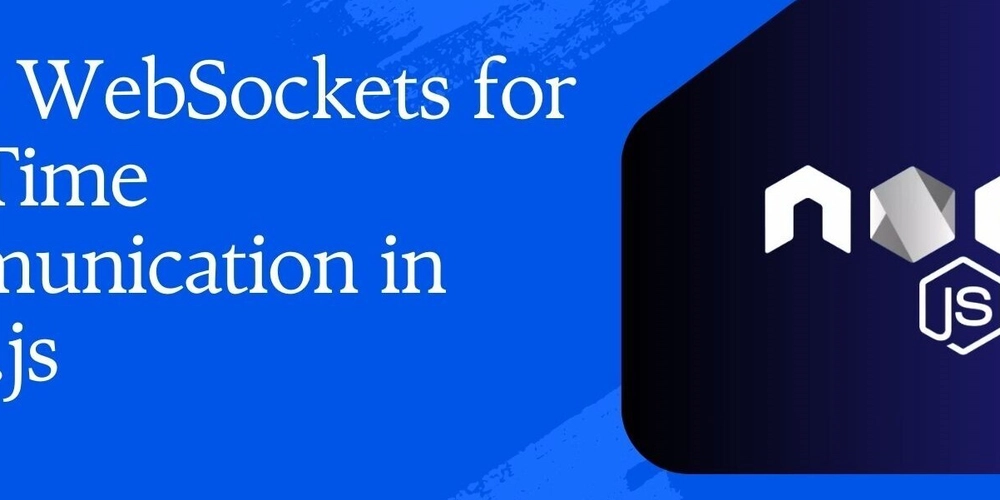
Realtime Collaboration App with WebSockets & Node.js
Real-time collaboration tools have become a core part of our digital workspace. Whether it's working on a shared document, editing a whiteboard, or coding in sync, real-time communication is essential. In this blog, we will explore how to build a Realtime Collaboration App using WebSockets with Node.js, focusing on a shared whiteboard or document editor powered by Socket.IO.
What is Real-Time Collaboration?
Real-time collaboration enables multiple users to interact with the same digital resource simultaneously. This could mean co-editing text, drawing on a shared canvas, or viewing changes live as they happen.
Key features of real-time collaboration include:
- Low latency updates
- Synchronization across clients
- Event handling and broadcasting
- Conflict resolution
Why Use WebSockets and Socket.IO?
WebSockets
WebSockets provide a full-duplex communication channel over a single TCP connection, ideal for low-latency, real-time apps.
Socket.IO
Socket.IO is a library built on top of WebSockets that simplifies real-time communication and includes:
- Auto-reconnection
- Room support
- Event-based communication
- Cross-browser support
System Architecture Overview
- Frontend (Web): Users interact with a whiteboard or document editor
-
Backend (Node.js + Socket.IO):
- Manages WebSocket connections
- Broadcasts updates to all clients in the same room
- Optional Database: Save history or states of the whiteboard or document
Use Case Example: Shared Whiteboard
We will build a collaborative whiteboard where multiple users can draw in real-time and see each other's input live.
Step-by-Step Implementation
1. Initialize Node.js Project
mkdir collaborative-whiteboard
cd collaborative-whiteboard
npm init -y
npm install express socket.io
2. Setup the Backend (index.js)
const express = require('express');
const http = require('http');
const { Server } = require('socket.io');
const app = express();
const server = http.createServer(app);
const io = new Server(server);
app.use(express.static('public'));
io.on('connection', (socket) => {
console.log('User connected:', socket.id);
socket.on('draw', (data) => {
socket.broadcast.emit('draw', data); // Send to all except sender
});
socket.on('clear', () => {
io.emit('clear'); // Send to all clients
});
});
server.listen(3000, () => console.log('Server running on http://localhost:3000'));
3. Frontend (public/index.html)
lang="en">
charset="UTF-8">
Collaborative Whiteboard
canvas { border: 1px solid #000; }
const socket = io();
const canvas = document.getElementById('board');
const ctx = canvas.getContext('2d');
let drawing = false;
canvas.addEventListener('mousedown', () => drawing = true);
canvas.addEventListener('mouseup', () => drawing = false);
canvas.addEventListener('mousemove', draw);
function draw(e) {
if (!drawing) return;
const x = e.offsetX;
const y = e.offsetY;
ctx.lineTo(x, y);
ctx.stroke();
socket.emit('draw', { x, y });
}
socket.on('draw', (data) => {
ctx.lineTo(data.x, data.y);
ctx.stroke();
});
function clearCanvas() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
socket.emit('clear');
}
socket.on('clear', () => ctx.clearRect(0, 0, canvas.width, canvas.height));
Extending to a Document Editor
To build a collaborative document editor:
- Replace the canvas with a
or contenteditable
- Emit
input
orkeydown
events with content- On the backend, broadcast content to all clients
- Use versioning to manage concurrent changes (CRDTs or OT)
Example event handling:
socket.on('text-update', (text) => { socket.broadcast.emit('text-update', text); });
And on the frontend:
textarea.addEventListener('input', () => { socket.emit('text-update', textarea.value); }); socket.on('text-update', (text) => { textarea.value = text; });
Bonus: Room Support
Rooms allow you to isolate users into separate collaborative sessions.
socket.on('join-room', (roomId) => { socket.join(roomId); socket.on('draw', (data) => { socket.to(roomId).emit('draw', data); }); });
Deployment Tips
- Use HTTPS (WebSockets require secure connections in production)
- Use a WebSocket-compatible server (like NGINX with WS support)
- Scale using Redis or socket.io-redis for multi-node setups
Conclusion
With WebSockets and Node.js, building real-time collaborative applications like a shared whiteboard or document editor is approachable and powerful. Socket.IO simplifies many of the tricky aspects, allowing developers to focus on UX and functionality. From classrooms to remote teams, these tools create interactive, synchronous environments where ideas can flow instantly.
Collaboration isn’t just about sharing—it's about creating in real-time. Let your app be the space where collaboration comes alive.
- Emit