Read Excel and PDF in Java
Read Excel in Java To read Excel file in java you need to create Maven project: To create:file--->New-->Maven project If You Want to Download JARs Manually Go to Maven Repository and search for: 1.poi 2.poi-ooxml 3.log4j-core 4.log4j-api Download the .jar files for latest version POM.xlm org.apache.poi poi 5.2.3 org.apache.poi poi-ooxml 5.2.3 org.apache.logging.log4j log4j-core 2.17.1 org.apache.logging.log4j log4j-api 2.17.1 Place them in your project's libs folder.Open the pom.xml file and add your inside the tag. package pdf_reader; import java.io.File;//Represents the Excel file. import java.io.FileInputStream;//Reads bytes from the file. import java.io.IOException;// import org.apache.poi.hssf.usermodel.HSSFWorkbook;//Used to read .xls files (Excel 97-2003 format). import org.apache.poi.ss.usermodel.*;//Handles Workbook, Sheet, Row, and Cell. public class ExcelReader { public static void main(String[] args) { String filePath = "/home/neelakandan/Downloads/Movie 1.xls"; // Path to your file try (FileInputStream fis = new FileInputStream(new File(filePath));//Opening and Reading the Excel File Workbook workbook = new HSSFWorkbook(fis)) { Sheet sheet = workbook.getSheetAt(0); // Get the first sheet for (Row row : sheet) { for (Cell cell : row) { switch (cell.getCellType()) //Reading Cell Values { case STRING://Reads and prints values based on their data type System.out.print(cell.getStringCellValue() + "\t"); break; case NUMERIC: System.out.print(cell.getNumericCellValue() + "\t"); break; case BOOLEAN: System.out.print(cell.getBooleanCellValue() + "\t"); break; default: System.out.print("UNKNOWN\t"); } } System.out.println(); } } catch (IOException e)//Catches IOException (e.g., file not found, read error) and prints the error stack trace. { e.printStackTrace(); } } } OutPut: Leo Vijay Trisha 623 Crore 7.2 Vidaamuyarchi Ajith Kumar Trisha 139.73 Crore 6.5 Valimai Ajith Kumar Huma Qureshi 234 Crore 6.7 End game Tony Natasha 2.8 Billion 8.4 Annabelle John Mia 257 Million 5.5 PDF Reader You can get the Apache PDFBox dependency from the Maven Repository (Maven Central). Steps to Download Visit the Maven Repository:--> Apache PDFBox in Maven Repository Download the JAR File: Click on "Download jar" under Files. If Using Maven: Add the following dependency to your pom.xml: POM.xlm org.apache.pdfbox pdfbox 2.0.27 Place them in your project's libs folder.Open the pom.xml file and add your inside the tag package pdf_reader; import java.io.File; import java.io.IOException; import org.apache.pdfbox.pdmodel.PDDocument; import org.apache.pdfbox.text.PDFTextStripper; public class PDFReader { public static void main(String[] args) { String filePath = "/home/neelakandan/Downloads/The Story of Gandhi.pdf"; // Update the path if needed File file = new File(filePath); if (!file.exists()) { System.out.println("File not found!"); return; } try (PDDocument document = PDDocument.load(file)) { // Auto-closing resource System.out.println("PDF Content:\n" + new PDFTextStripper().getText(document)); } catch (IOException e) { e.printStackTrace(); } } } ``
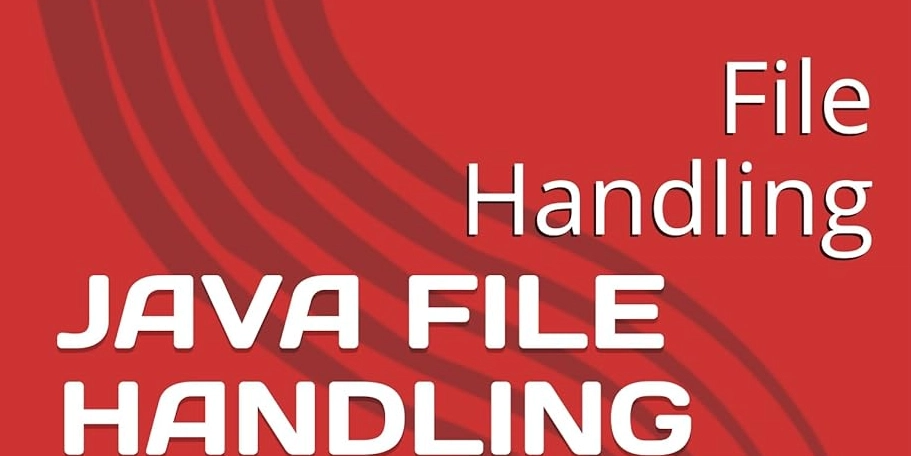
Read Excel in Java
To read Excel file in java you need to create Maven project:
To create:file--->New-->Maven project
If You Want to Download JARs Manually
Go to Maven Repository and search for:
1.poi
2.poi-ooxml
3.log4j-core
4.log4j-api
Download the .jar files for latest version
POM.xlm
org.apache.poi
poi
5.2.3
org.apache.poi
poi-ooxml
5.2.3
org.apache.logging.log4j
log4j-core
2.17.1
org.apache.logging.log4j
log4j-api
2.17.1
Place them in your project's libs folder.Open the pom.xml file and add your inside the tag.
package pdf_reader;
import java.io.File;//Represents the Excel file.
import java.io.FileInputStream;//Reads bytes from the file.
import java.io.IOException;//
import org.apache.poi.hssf.usermodel.HSSFWorkbook;//Used to read .xls files (Excel 97-2003 format).
import org.apache.poi.ss.usermodel.*;//Handles Workbook, Sheet, Row, and Cell.
public class ExcelReader {
public static void main(String[] args) {
String filePath = "/home/neelakandan/Downloads/Movie 1.xls"; // Path to your file
try (FileInputStream fis = new FileInputStream(new File(filePath));//Opening and Reading the Excel File
Workbook workbook = new HSSFWorkbook(fis)) {
Sheet sheet = workbook.getSheetAt(0); // Get the first sheet
for (Row row : sheet) {
for (Cell cell : row) {
switch (cell.getCellType()) //Reading Cell Values
{
case STRING://Reads and prints values based on their data type
System.out.print(cell.getStringCellValue() + "\t");
break;
case NUMERIC:
System.out.print(cell.getNumericCellValue() + "\t");
break;
case BOOLEAN:
System.out.print(cell.getBooleanCellValue() + "\t");
break;
default:
System.out.print("UNKNOWN\t");
}
}
System.out.println();
}
} catch (IOException e)//Catches IOException (e.g., file not found, read error) and prints the error stack trace.
{
e.printStackTrace();
}
}
}
OutPut:
Leo Vijay Trisha 623 Crore 7.2
Vidaamuyarchi Ajith Kumar Trisha 139.73 Crore 6.5
Valimai Ajith Kumar Huma Qureshi 234 Crore 6.7
End game Tony Natasha 2.8 Billion 8.4
Annabelle John Mia 257 Million 5.5
PDF Reader
You can get the Apache PDFBox dependency from the Maven Repository (Maven Central).
Steps to Download
Visit the Maven Repository:--> Apache PDFBox in Maven Repository
Download the JAR File:
Click on "Download jar" under Files.
If Using Maven:
Add the following dependency to your pom.xml:
POM.xlm
org.apache.pdfbox
pdfbox
2.0.27
Place them in your project's libs folder.Open the pom.xml file and add your inside the tag
package pdf_reader;
import java.io.File;
import java.io.IOException;
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.text.PDFTextStripper;
public class PDFReader {
public static void main(String[] args) {
String filePath = "/home/neelakandan/Downloads/The Story of Gandhi.pdf"; // Update the path if needed
File file = new File(filePath);
if (!file.exists()) {
System.out.println("File not found!");
return;
}
try (PDDocument document = PDDocument.load(file)) { // Auto-closing resource
System.out.println("PDF Content:\n" + new PDFTextStripper().getText(document));
} catch (IOException e) {
e.printStackTrace();
}
}
}
``