Q.1 How do you check if a variable is an array?
Using Array.isArray() let arr = [1, 2, 3]; let notArray = "Hello"; console.log(Array.isArray(arr)); // true console.log(Array.isArray(notArray)); // false Using instanceof Operator The instanceof operator can be used to check if a variable is an instance of Array. let arr = [1, 2, 3]; let notArray = "Hello"; console.log(arr instanceof Array); // true console.log(notArray instanceof Array); // false Using constructor Property You can check the constructor property of an object, but this method is not as reliable because the constructor property can be modified. let arr = [1, 2, 3]; let notArray = "Hello"; console.log(arr.constructor === Array); // true console.log(notArray.constructor === Array); // false While this works in most cases, it's generally not recommended due to potential issues with modifying the constructor property. Recommended Method: The best and most reliable way to check if a variable is an array in JavaScript is by using Array.isArray() because it is simple, clear, and works in all scenarios.
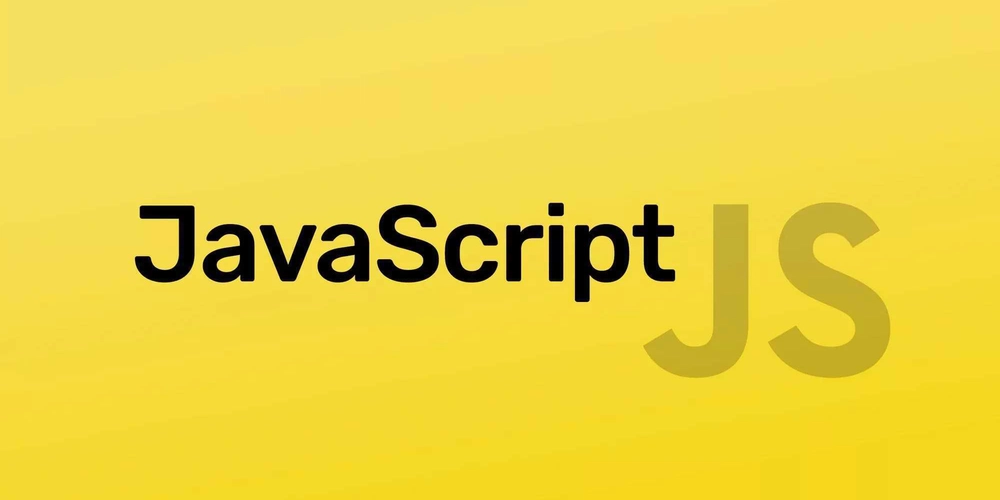
Using Array.isArray()
let arr = [1, 2, 3];
let notArray = "Hello";
console.log(Array.isArray(arr)); // true
console.log(Array.isArray(notArray)); // false
Using instanceof Operator
The instanceof operator can be used to check if a variable is an instance of Array.
let arr = [1, 2, 3];
let notArray = "Hello";
console.log(arr instanceof Array); // true
console.log(notArray instanceof Array); // false
Using constructor Property
You can check the constructor property of an object, but this method is not as reliable because the constructor property can be modified.
let arr = [1, 2, 3];
let notArray = "Hello";
console.log(arr.constructor === Array); // true
console.log(notArray.constructor === Array); // false
While this works in most cases, it's generally not recommended due to potential issues with modifying the constructor property.
Recommended Method:
The best and most reliable way to check if a variable is an array in JavaScript is by using Array.isArray() because it is simple, clear, and works in all scenarios.