PerformanceObserver API for Resource Tracking
PerformanceObserver API for Resource Tracking: A Comprehensive Guide Table of Contents Historical and Technical Context Understanding the PerformanceObserver API In-Depth Code Examples Basic Usage Complex Scenarios Edge Cases and Advanced Implementation Techniques Comparison with Alternative Approaches Real-World Use Cases Performance Considerations and Optimization Strategies Potential Pitfalls and Advanced Debugging Techniques Conclusion Further Reading and Resources Historical and Technical Context The PerformanceObserver API is rooted in a broader endeavor to improve web performance measurement techniques, encapsulated by the High Resolution Time API and the Navigation Timing API. As web applications became increasingly complex, the significance of precise performance tracking grew. Standard metrics like page load time were inadequate for dissecting the performance bottlenecks in increasingly modular and interactive applications. Introduced with High Resolution Time Level 2 Specification, PerformanceObserver allows developers to asynchronously observe performance metrics of various kinds, such as resource timing, mark, measure, and user timing. This API defines an interface for collecting and processing performance entries from the browser's performance metrics—empowering developers to monitor the resource performance in real-time. Understanding the PerformanceObserver API The PerformanceObserver API allows you to set up an observer that listens for specific performance-related entries. The entries that can be observed include: Resource Timing: Collects timing data for performance entries for all resources fetched by the document (e.g., images, scripts, styles). Mark/Measure: Allows for custom timing data, where you can define your own performance marks and measure them against one another. Key Components of the API PerformanceObserver Constructor: Used to create an observer instance. observe() method: Starts observing specific entry types. disconnect() method: Stops observing the entries. takeRecords() method: Retrieves any performance entries that have been recorded but not yet delivered to the observer's callback. In-Depth Code Examples Basic Usage Using PerformanceObserver for resource timing can help you identify when specific resources are loaded. Below is a concise example demonstrating its basic structure. // Create a new PerformanceObserver instance const observer = new PerformanceObserver((list) => { list.getEntries().forEach((entry) => { console.log(`${entry.name} - Load Time: ${entry.duration} ms`); }); }); // Start observing resource timing entries observer.observe({ entryTypes: ['resource'] }); // Example of loading a resource const script = document.createElement('script'); script.src = 'https://example.com/script.js'; document.head.appendChild(script); Complex Scenarios Let's consider a more complex scenario where you might want to handle different entry types and debounce the processing of performance entries. const debounce = (func, delay) => { let timeout; return function(...args) { clearTimeout(timeout); timeout = setTimeout(() => func.apply(this, args), delay); }; }; const observer = new PerformanceObserver(debounce((list) => { list.getEntries().forEach((entry) => { if (entry.entryType === "resource") { console.log(`Resource loaded: ${entry.name} - Duration: ${entry.duration} ms`); } else if (entry.entryType === "mark") { console.log(`Mark identified: ${entry.name}`); } }); }, 100)); observer.observe({ entryTypes: ['resource', 'mark'] }); // Marking a specific point in time performance.mark("startLoading"); // Simulate some loading to trigger the observer setTimeout(() => { performance.mark("endLoading"); performance.measure("Loading Duration", "startLoading", "endLoading"); }, 200); Edge Cases and Advanced Implementation Techniques Handling Overlapping Observations One of the edge cases in using PerformanceObserver is when multiple observers can observe the same entry type. If you're tracking multiple resources or measures, you should ensure that your logic to track these is robust. const observers = []; const createObserver = (type) => { const observer = new PerformanceObserver((list) => { list.getEntries().forEach((entry) => { console.log(`Type: ${type}, Entry: ${entry.name}, Duration: ${entry.duration}`); }); }); observer.observe({ entryTypes: [type] }); observers.push(observer); }; createObserver('resource'); createObserver('mark'); Comparison with Alternative Approaches Traditional Performance Measurement Before the introduction of the PerformanceObserver API, developers would typically rely on older methods of performance measurement, such as: Manual time tracking using Date.now() or performance.now() to measur
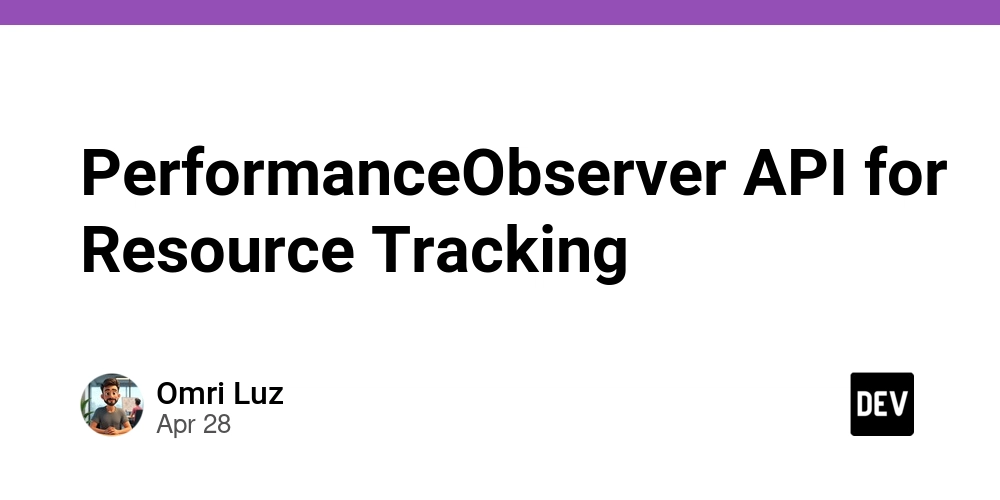
PerformanceObserver API for Resource Tracking: A Comprehensive Guide
Table of Contents
- Historical and Technical Context
- Understanding the PerformanceObserver API
-
In-Depth Code Examples
- Basic Usage
- Complex Scenarios
- Edge Cases and Advanced Implementation Techniques
- Comparison with Alternative Approaches
- Real-World Use Cases
- Performance Considerations and Optimization Strategies
- Potential Pitfalls and Advanced Debugging Techniques
- Conclusion
- Further Reading and Resources
Historical and Technical Context
The PerformanceObserver
API is rooted in a broader endeavor to improve web performance measurement techniques, encapsulated by the High Resolution Time API and the Navigation Timing API. As web applications became increasingly complex, the significance of precise performance tracking grew. Standard metrics like page load time were inadequate for dissecting the performance bottlenecks in increasingly modular and interactive applications.
Introduced with High Resolution Time Level 2 Specification, PerformanceObserver
allows developers to asynchronously observe performance metrics of various kinds, such as resource timing, mark, measure, and user timing. This API defines an interface for collecting and processing performance entries from the browser's performance metrics—empowering developers to monitor the resource performance in real-time.
Understanding the PerformanceObserver API
The PerformanceObserver
API allows you to set up an observer that listens for specific performance-related entries. The entries that can be observed include:
- Resource Timing: Collects timing data for performance entries for all resources fetched by the document (e.g., images, scripts, styles).
- Mark/Measure: Allows for custom timing data, where you can define your own performance marks and measure them against one another.
Key Components of the API
- PerformanceObserver Constructor: Used to create an observer instance.
- observe() method: Starts observing specific entry types.
- disconnect() method: Stops observing the entries.
- takeRecords() method: Retrieves any performance entries that have been recorded but not yet delivered to the observer's callback.
In-Depth Code Examples
Basic Usage
Using PerformanceObserver
for resource timing can help you identify when specific resources are loaded. Below is a concise example demonstrating its basic structure.
// Create a new PerformanceObserver instance
const observer = new PerformanceObserver((list) => {
list.getEntries().forEach((entry) => {
console.log(`${entry.name} - Load Time: ${entry.duration} ms`);
});
});
// Start observing resource timing entries
observer.observe({ entryTypes: ['resource'] });
// Example of loading a resource
const script = document.createElement('script');
script.src = 'https://example.com/script.js';
document.head.appendChild(script);
Complex Scenarios
Let's consider a more complex scenario where you might want to handle different entry types and debounce the processing of performance entries.
const debounce = (func, delay) => {
let timeout;
return function(...args) {
clearTimeout(timeout);
timeout = setTimeout(() => func.apply(this, args), delay);
};
};
const observer = new PerformanceObserver(debounce((list) => {
list.getEntries().forEach((entry) => {
if (entry.entryType === "resource") {
console.log(`Resource loaded: ${entry.name} - Duration: ${entry.duration} ms`);
} else if (entry.entryType === "mark") {
console.log(`Mark identified: ${entry.name}`);
}
});
}, 100));
observer.observe({ entryTypes: ['resource', 'mark'] });
// Marking a specific point in time
performance.mark("startLoading");
// Simulate some loading to trigger the observer
setTimeout(() => {
performance.mark("endLoading");
performance.measure("Loading Duration", "startLoading", "endLoading");
}, 200);
Edge Cases and Advanced Implementation Techniques
Handling Overlapping Observations
One of the edge cases in using PerformanceObserver
is when multiple observers can observe the same entry type. If you're tracking multiple resources or measures, you should ensure that your logic to track these is robust.
const observers = [];
const createObserver = (type) => {
const observer = new PerformanceObserver((list) => {
list.getEntries().forEach((entry) => {
console.log(`Type: ${type}, Entry: ${entry.name}, Duration: ${entry.duration}`);
});
});
observer.observe({ entryTypes: [type] });
observers.push(observer);
};
createObserver('resource');
createObserver('mark');
Comparison with Alternative Approaches
Traditional Performance Measurement
Before the introduction of the PerformanceObserver
API, developers would typically rely on older methods of performance measurement, such as:
- Manual time tracking using
Date.now()
orperformance.now()
to measure time spans between synchronous code execution. - Network panel tools available in browser developer tools to assess loading times.
Advantages of PerformanceObserver
- Granularity: Observers can capture detailed performance entries including custom marks and vital user timing.
- Asynchronous Notifications: Unlike traditional synchronous measures, the API is designed to offload collection and reporting, thus not blocking the main thread.
Limitations of Alternatives
- Limited to synchronous measures: Older methods do not support capturing detailed metrics on resource load timing.
- Manual data management: Handling performance entries requires manual coding overhead to push metrics to external systems.
Real-World Use Cases
Application Performance Monitoring (APM)
Using the PerformanceObserver
API, major APM solutions like New Relic or Datadog integrate real-time performance data to provide insights into application performance, helping identify bottlenecks dynamically.
User Experience Optimization
PayPal uses the PerformanceObserver
API to track loading times for various touches of the application. Using performance metrics enables adaptive loading strategies (degradation, optimization of resource-serving mechanisms) that are pivotal in enhancing user experience.
Client-Side A/B Testing
Companies like Shopify implement client-side A/B testing solutions that leverage real-time performance tracking. This allows them to assess user interactions with different versions of their site, driving iterative design and performance improvements.
Performance Considerations and Optimization Strategies
Minimize Observer Count
While you can have multiple PerformanceObserver
instances, creating too many can lead to increased overhead. It’s optimal to consolidate observers when possible.
Throttle Data Processing
Implementing debouncing or throttling mechanisms (examples shown earlier) can significantly limit performance impacts, especially under high-frequency resource loading scenarios.
Resource Warming
Before measuring performance metrics on critical resources like fonts or styles, you might pre-load them to avoid skewed results in your performance assessments.
Potential Pitfalls and Advanced Debugging Techniques
Overhead Concerns
Using PerformanceObserver
adds a non-negligible overhead, especially in scenarios where performance capture is frequent. Developers should be conscious of how many observers are active concurrently.
Losing Metrics
If an observer is disconnected before its callback is processed, relevant metrics might be lost. It's prudent to ensure observers remain active for as long as the application can expect resource load events.
Flags for Debugging
Leverage the browser’s DevTools to monitor performance entries. The Performance
tab often provides deep insights into painted frames, painting timing, and resource timing that can complement the output of PerformanceObserver
.
Introduce Custom Loggers
In advanced debugging, consider integrating frameworks such as Sentry, which can help log performance metrics to a centralized location for further analysis.
Conclusion
The PerformanceObserver
API is a powerful tool that allows developers to implement resource tracking in dynamic web applications effectively. Its integration offers a granular view of resource timing, performance metrics, and overall application efficiency.
Transient bottlenecks and performance degradation might not be easily caught without a robust implementation of performance monitoring. Understanding how to utilize PerformanceObserver
can elevate a web application’s stability and user experience significantly.
Further Reading and Resources
- MDN Documentation on PerformanceObserver
- W3C High Resolution Time Specification
- Google Web Fundamentals: Performance APIs
- Web Performance Working Group
- Resource Timing API Specification
This article serves as a comprehensive exploration of the PerformanceObserver
API, providing insights and methodologies that will be invaluable to senior developers looking to optimize web performance rigorously.