Passing info between different branches of a class hierarchy in C++?
So, I have a class hierarchy that looks like this: It is an embedded project, so the entire code will be run over and over. I have several algorithm classes, that compute some result given some inputs. The results are written to some structs (that contain the necessary members). class Algorithm1 { Algo1Output out; }; class Algorithm2 { Algo2Output out; }; class Algorithm3 { Algo3Output out; }; These algorithm classes are orchestrated by an Algorithms class. This class gathers the outputs of the algorithms in an aggregated output (that is just references to the individual outputs). class Algorithms { Algorithm1 a1{}; Algorithm2 a2{}; Algorithm3 a3{}; AlgosOutput out{ a1.out, a2.out, a3.out }; }; class AlgosOutput { Algo1Output& a1out; Algo2Output& a2out; Algo3Output& a3out; }; The Algorithms class is in turn managed by a Manager class, that also manages a state machine and has an output of its own that is just a reference to the outputs of Algorithms and StateMachine: class Manager { StateMachine sm; Algorithms a; ManagerOutput out{ sm.out, a.out }; }; class StateMachine { SmOutput out; }; All of this hierarchy gets its inputs from a common source, that is simply passed down at initialization. The constructors basically all look almost the same: Manager(InputData& inData) : sm(inData), a(inData) {} StateMachine(InputData& inData) : inData(inData) {} // holds a reference to inData for actual use Algorithms(InputData& inData) : a1(inData), a2(inData), a3(inData) {} Algorithm1(InputData& inData) : inData(inData) {} Algorithm2(InputData& inData) : inData(inData) {} Algorithm3(InputData& inData) : inData(inData) {} So all the components have a reference to inData (read-only access, but I tried to keep code fragments brief), and inData is updated with new info every run cycle. And each class in the hierarchy has access to the output of the classes immediately below it (which in turn contain the outptus of the classes immediately below them etc.). When execution is done, the state machine and the algorithms are all executed using inData. After execution, according to the new state that StateMachine is in, the result of one of the 3 algorithm classes will be used. This logic is in Manager: void Algorithms::execute() { a1.execute(); a2.execute(); a3.execute(); } void Manager::execute() { sm.execute(); a.execute(); switch (out.stateMachineOut.currentState) { // select one of the 3 algo results for further use } } Problem is that now, Algorithm2 needs the current state of the state machine to function. So I need a way to pass along that info. Ideally an elegant way that does not involve a complex design pattern like Mediator. Option 1: One way would be to basically make inData non-const in the state machine and add a member to that, since everyone has a reference to inData, but inData only contains input data from outside this system so I have a feeling it would be bad practice to pollute it with temporary values that pertain to the internals of the system. Option 2: Another way would be to have Algorithms also get (as a ctor argument) a reference to sm.out and pass that along to Algorhithm 1/2/3 so now the algorithm classes maintain a reference to sm.out and have instant read-only access to the output of state machine. However because of how C++ initialization works, this would mean that inside Manager, StateMachine should always remain declared above Algorithms so it is initilized before Algorithms, meaning I would have to put a big comment with "LEAVE THE DECLARATION OF STATE MACHINE ABOVE ALGORITHMS" and hope other developers bother to read it. Option 3: And another way would be to pass the current state of StateMachine as an argument to Algorithms::execute() (which will in turn pass it to the 3 algorithm classes). This method also gives me a feeling that itmay be bad practice since all classes basically only use inData as input, and if I were to add one single argument to them, the situation of the Algorithm classes would change to the input being split, with about 95% of the input values inside inData and one input value being passed as an argument. Which way would be the "best practice" here and why? Is there something I did not think of? My gut says to go with option 2, but that would create a new piece of coupling between StateMachine and Algorithms, and quite a big piece of coupling at that. Or is a degree of coupling inevitable since I basically need to bring the output of StateMachine into Algorithms anyways?
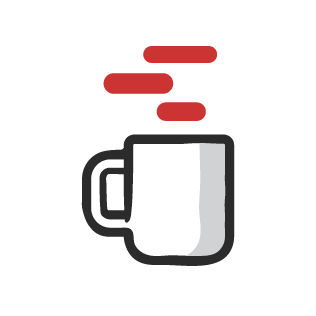
So, I have a class hierarchy that looks like this:
It is an embedded project, so the entire code will be run over and over.
I have several algorithm classes, that compute some result given some inputs. The results are written to some structs (that contain the necessary members).
class Algorithm1 { Algo1Output out; };
class Algorithm2 { Algo2Output out; };
class Algorithm3 { Algo3Output out; };
These algorithm classes are orchestrated by an Algorithms
class. This class gathers the outputs of the algorithms in an aggregated output (that is just references to the individual outputs).
class Algorithms {
Algorithm1 a1{};
Algorithm2 a2{};
Algorithm3 a3{};
AlgosOutput out{ a1.out, a2.out, a3.out };
};
class AlgosOutput {
Algo1Output& a1out;
Algo2Output& a2out;
Algo3Output& a3out;
};
The Algorithms
class is in turn managed by a Manager
class, that also manages a state machine and has an output of its own that is just a reference to the outputs of Algorithms
and StateMachine
:
class Manager {
StateMachine sm;
Algorithms a;
ManagerOutput out{ sm.out, a.out };
};
class StateMachine {
SmOutput out;
};
All of this hierarchy gets its inputs from a common source, that is simply passed down at initialization. The constructors basically all look almost the same:
Manager(InputData& inData) : sm(inData), a(inData) {}
StateMachine(InputData& inData) : inData(inData) {} // holds a reference to inData for actual use
Algorithms(InputData& inData) : a1(inData), a2(inData), a3(inData) {}
Algorithm1(InputData& inData) : inData(inData) {}
Algorithm2(InputData& inData) : inData(inData) {}
Algorithm3(InputData& inData) : inData(inData) {}
So all the components have a reference to inData
(read-only access, but I tried to keep code fragments brief), and inData
is updated with new info every run cycle. And each class in the hierarchy has access to the output of the classes immediately below it (which in turn contain the outptus of the classes immediately below them etc.).
When execution is done, the state machine and the algorithms are all executed using inData
. After execution, according to the new state that StateMachine
is in, the result of one of the 3 algorithm classes will be used. This logic is in Manager
:
void Algorithms::execute() {
a1.execute();
a2.execute();
a3.execute();
}
void Manager::execute() {
sm.execute();
a.execute();
switch (out.stateMachineOut.currentState) {
// select one of the 3 algo results for further use
}
}
Problem is that now, Algorithm2
needs the current state of the state machine to function. So I need a way to pass along that info. Ideally an elegant way that does not involve a complex design pattern like Mediator.
Option 1: One way would be to basically make inData
non-const in the state machine and add a member to that, since everyone has a reference to inData
, but inData
only contains input data from outside this system so I have a feeling it would be bad practice to pollute it with temporary values that pertain to the internals of the system.
Option 2: Another way would be to have Algorithms
also get (as a ctor argument) a reference to sm.out
and pass that along to Algorhithm 1/2/3
so now the algorithm classes maintain a reference to sm.out
and have instant read-only access to the output of state machine. However because of how C++ initialization works, this would mean that inside Manager
, StateMachine
should always remain declared above Algorithms
so it is initilized before Algorithms
, meaning I would have to put a big comment with "LEAVE THE DECLARATION OF STATE MACHINE ABOVE ALGORITHMS" and hope other developers bother to read it.
Option 3: And another way would be to pass the current state of StateMachine
as an argument to Algorithms::execute()
(which will in turn pass it to the 3 algorithm classes). This method also gives me a feeling that itmay be bad practice since all classes basically only use inData
as input, and if I were to add one single argument to them, the situation of the Algorithm
classes would change to the input being split, with about 95% of the input values inside inData
and one input value being passed as an argument.
Which way would be the "best practice" here and why? Is there something I did not think of?
My gut says to go with option 2, but that would create a new piece of coupling between StateMachine
and Algorithms
, and quite a big piece of coupling at that. Or is a degree of coupling inevitable since I basically need to bring the output of StateMachine
into Algorithms
anyways?