Nextjs with tailwind and typescript
next js with tailwind and typescript Next.js is an innovative React framework that simplifies the process of building modern web applications with features like server-side rendering, static site generation, and automatic code splitting. It provides a comprehensive solution that allows developers to create dynamic and high-performance applications with ease, all while leveraging the powerful capabilities of React. Understanding the difference between frameworks and libraries is essential when diving into Next.js. In the world of web development, libraries are collections of pre-written code that developers can invoke or utilize as needed. They provide specific functionalities that can be used to build applications but do not dictate the structure of your code. On the other hand, a framework is a more opinionated set of tools or libraries that provides a foundation for building applications, imposing a certain structure and set of conventions to help guide developers. Next.js falls into the category of a framework. It not only includes React but also provides essential features for routing, data fetching, and rendering strategies, making it easier to create robust applications. As we explore Next.js, we will also integrate Tailwind CSS, a utility-first CSS framework that allows for rapid styling and customization of applications without leaving your markup. Coupling Next.js with TypeScript, a statically typed superset of JavaScript, enhances the development experience, providing type safety and improved code quality. In this article, we will take a deep dive into how Next.js works, the app folder approach introduced in Next.js 13 and onward, and how you can utilize Tailwind CSS and TypeScript to create visually appealing and type-safe web applications. You'll also learn about key files in Next.js, such as page.tsx, layout.tsx, and route.ts, which play crucial roles in the routing and structure of your app. If you want to advance your knowledge in Next.js or explore AI tools like gpteach to learn how to code effectively, consider subscribing, following, or joining our blog for more updates and tutorials. What Makes Next.js Stand Out? Next.js enhances the React experience by introducing several powerful features: File-based Routing: This allows developers to create routes by simply adding files to the pages directory. Each file automatically becomes a route, simplifying the process of setting up navigation in your application. Static Site Generation (SSG) and Server-side Rendering (SSR): Next.js supports hybrid applications, meaning you can choose to statically generate some pages while rendering others on the server to optimize performance and SEO. API Routes: You can create serverless functions using the api folder, making it easy to manage backend logic without needing a separate server. Built-in CSS and Sass Support: Using Tailwind CSS with Next.js is seamless because the framework supports global styles via special CSS files. Incremental Static Regeneration (ISR): It allows you to update static content without having to rebuild the entire site, offering the best of both worlds between static and dynamic content. Setting Up Next.js with Tailwind and TypeScript To get started with Next.js using Tailwind CSS and TypeScript, follow these steps: Step 1: Create a Next.js Application You can create a new Next.js project with TypeScript using npx: npx create-next-app@latest my-next-app --typescript Step 2: Install Tailwind CSS Next, navigate to your project and install Tailwind CSS: cd my-next-app npm install -D tailwindcss postcss autoprefixer npx tailwindcss init -p This command will generate a tailwind.config.js file along with a postcss.config.js file. Step 3: Configure Tailwind CSS In your tailwind.config.js, set up the paths to your template files: module.exports = { content: [ "./pages/**/*.{js,ts,jsx,tsx}", "./components/**/*.{js,ts,jsx,tsx}" ], theme: { extend: {}, }, plugins: [], } Next, update your CSS file (usually located at styles/globals.css) to include the Tailwind directives: @tailwind base; @tailwind components; @tailwind utilities; Step 4: Create Your First Page In the pages directory, create a new file called index.tsx and set up a simple component: const Home = () => { return ( Welcome to Next.js with Tailwind CSS and TypeScript! ); }; export default Home; Exploring Key Files in Next.js In a Next.js application, you will encounter several key files, each serving a critical purpose: page.tsx: This file represents a route in your application. Each .tsx file within the pages directory corresponds to a separate route, helping to establish a clear and organized structure for your application. layout.tsx: This allows you to define the layout for your pages. You can use this file to share common components like h
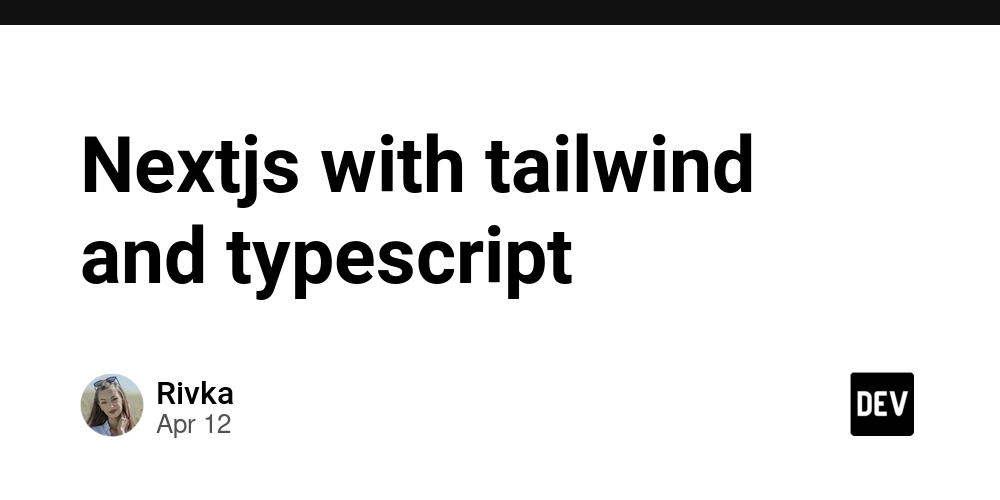
next js with tailwind and typescript
Next.js is an innovative React framework that simplifies the process of building modern web applications with features like server-side rendering, static site generation, and automatic code splitting. It provides a comprehensive solution that allows developers to create dynamic and high-performance applications with ease, all while leveraging the powerful capabilities of React. Understanding the difference between frameworks and libraries is essential when diving into Next.js.
In the world of web development, libraries are collections of pre-written code that developers can invoke or utilize as needed. They provide specific functionalities that can be used to build applications but do not dictate the structure of your code. On the other hand, a framework is a more opinionated set of tools or libraries that provides a foundation for building applications, imposing a certain structure and set of conventions to help guide developers. Next.js falls into the category of a framework. It not only includes React but also provides essential features for routing, data fetching, and rendering strategies, making it easier to create robust applications.
As we explore Next.js, we will also integrate Tailwind CSS, a utility-first CSS framework that allows for rapid styling and customization of applications without leaving your markup. Coupling Next.js with TypeScript, a statically typed superset of JavaScript, enhances the development experience, providing type safety and improved code quality.
In this article, we will take a deep dive into how Next.js works, the app folder approach introduced in Next.js 13 and onward, and how you can utilize Tailwind CSS and TypeScript to create visually appealing and type-safe web applications. You'll also learn about key files in Next.js, such as page.tsx
, layout.tsx
, and route.ts
, which play crucial roles in the routing and structure of your app.
If you want to advance your knowledge in Next.js or explore AI tools like gpteach to learn how to code effectively, consider subscribing, following, or joining our blog for more updates and tutorials.
What Makes Next.js Stand Out?
Next.js enhances the React experience by introducing several powerful features:
File-based Routing: This allows developers to create routes by simply adding files to the
pages
directory. Each file automatically becomes a route, simplifying the process of setting up navigation in your application.Static Site Generation (SSG) and Server-side Rendering (SSR): Next.js supports hybrid applications, meaning you can choose to statically generate some pages while rendering others on the server to optimize performance and SEO.
API Routes: You can create serverless functions using the
api
folder, making it easy to manage backend logic without needing a separate server.Built-in CSS and Sass Support: Using Tailwind CSS with Next.js is seamless because the framework supports global styles via special CSS files.
Incremental Static Regeneration (ISR): It allows you to update static content without having to rebuild the entire site, offering the best of both worlds between static and dynamic content.
Setting Up Next.js with Tailwind and TypeScript
To get started with Next.js using Tailwind CSS and TypeScript, follow these steps:
Step 1: Create a Next.js Application
You can create a new Next.js project with TypeScript using npx:
npx create-next-app@latest my-next-app --typescript
Step 2: Install Tailwind CSS
Next, navigate to your project and install Tailwind CSS:
cd my-next-app
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init -p
This command will generate a tailwind.config.js
file along with a postcss.config.js
file.
Step 3: Configure Tailwind CSS
In your tailwind.config.js
, set up the paths to your template files:
module.exports = {
content: [
"./pages/**/*.{js,ts,jsx,tsx}",
"./components/**/*.{js,ts,jsx,tsx}"
],
theme: {
extend: {},
},
plugins: [],
}
Next, update your CSS file (usually located at styles/globals.css
) to include the Tailwind directives:
@tailwind base;
@tailwind components;
@tailwind utilities;
Step 4: Create Your First Page
In the pages
directory, create a new file called index.tsx
and set up a simple component:
const Home = () => {
return (
<div className="flex justify-center items-center h-screen bg-gray-100">
<h1 className="text-3xl font-bold text-blue-600">Welcome to Next.js with Tailwind CSS and TypeScript!h1>
div>
);
};
export default Home;
Exploring Key Files in Next.js
In a Next.js application, you will encounter several key files, each serving a critical purpose:
page.tsx
: This file represents a route in your application. Each.tsx
file within thepages
directory corresponds to a separate route, helping to establish a clear and organized structure for your application.layout.tsx
: This allows you to define the layout for your pages. You can use this file to share common components like headers, footers, or sidebars across different pages, promoting consistent design.route.ts
: With Next.js’s routing capabilities, theroute.ts
file allows you to define custom routes and access parameters within your application, giving you finer control over navigation.
Conclusion
Next.js is a powerful framework for building React applications, with robust features that streamline the development process. By integrating Tailwind CSS and TypeScript, developers can create visually appealing and type-safe applications effectively. Explore the capabilities of Next.js, follow best practices, and don’t hesitate to experiment with your projects. If you want to learn more about Next.js or use AI tools like gpteach to enhance your coding skills, consider subscribing to our blog for insightful articles and tutorials. Happy coding!