Mobile App with Expo & Firebase Auth - Part 1: Setup
In this first post, I'll show you how to set up your Expo project and configure Firebase for authentication. Step 1: Create Your Expo Project Open your terminal and run: expo init MyAuthApp cd MyAuthApp expo start This initializes your project and starts the Expo development server. Step 2: Set Up Firebase Go to the Firebase Console and create a new project. In the Authentication section, enable Email/Password and Google sign-in. Install Firebase in your project: npm install firebase Create a file named firebaseConfig.js with your Firebase credentials: import firebase from 'firebase/app'; import 'firebase/auth'; const firebaseConfig = { apiKey: "YOUR_API_KEY", authDomain: "YOUR_PROJECT_ID.firebaseapp.com", // ...other settings }; if (!firebase.apps.length) { firebase.initializeApp(firebaseConfig); } export { firebase }; Step 3: Create an Auth Context Make a new file called AuthContext.js inside a context folder: import React, { createContext, useState, useEffect } from 'react'; import { firebase } from '../firebaseConfig'; export const AuthContext = createContext(); export const AuthProvider = ({ children }) => { const [user, setUser] = useState(null); const [loading, setLoading] = useState(true); useEffect(() => { const unsubscribe = firebase.auth().onAuthStateChanged(currentUser => { setUser(currentUser); setLoading(false); }); return unsubscribe; }, []); return ( {children} ); }; Then, wrap your main component with the Auth Provider in App.js: import React from 'react'; import { AuthProvider } from './context/AuthContext'; import Routes from './Routes'; export default function App() { return ( ); } Conclusion In this post, we set up an Expo project, configured Firebase for authentication, and created a simple Auth Context to handle user sessions. Next time, I'll cover building the login and sign-up screens. Happy coding!
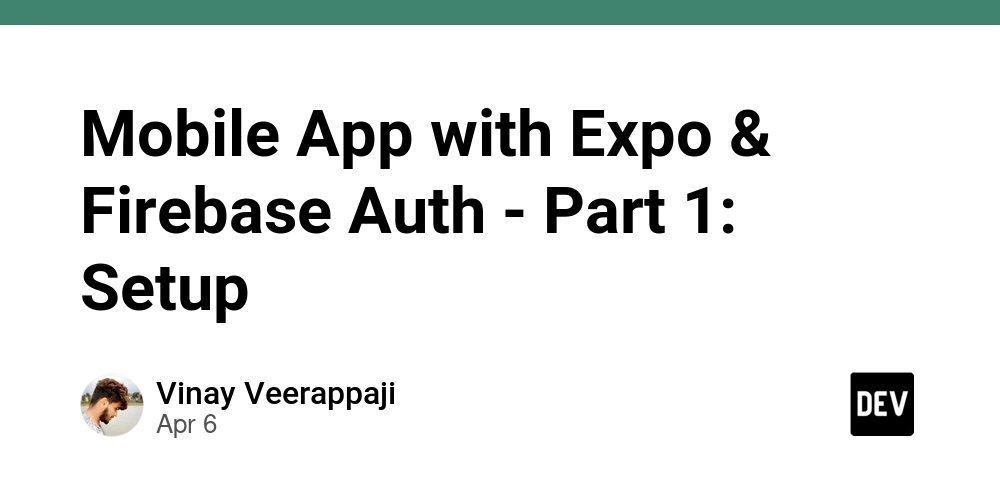
In this first post, I'll show you how to set up your Expo project and configure Firebase for authentication.
Step 1: Create Your Expo Project
Open your terminal and run:
expo init MyAuthApp
cd MyAuthApp
expo start
This initializes your project and starts the Expo development server.
Step 2: Set Up Firebase
- Go to the Firebase Console and create a new project.
- In the Authentication section, enable Email/Password and Google sign-in.
Install Firebase in your project:
npm install firebase
Create a file named firebaseConfig.js with your Firebase credentials:
import firebase from 'firebase/app';
import 'firebase/auth';
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_PROJECT_ID.firebaseapp.com",
// ...other settings
};
if (!firebase.apps.length) {
firebase.initializeApp(firebaseConfig);
}
export { firebase };
Step 3: Create an Auth Context
Make a new file called AuthContext.js inside a context folder:
import React, { createContext, useState, useEffect } from 'react';
import { firebase } from '../firebaseConfig';
export const AuthContext = createContext();
export const AuthProvider = ({ children }) => {
const [user, setUser] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
const unsubscribe = firebase.auth().onAuthStateChanged(currentUser => {
setUser(currentUser);
setLoading(false);
});
return unsubscribe;
}, []);
return (
{children}
);
};
Then, wrap your main component with the Auth Provider in App.js:
import React from 'react';
import { AuthProvider } from './context/AuthContext';
import Routes from './Routes';
export default function App() {
return (
);
}
Conclusion
In this post, we set up an Expo project, configured Firebase for authentication, and created a simple Auth Context to handle user sessions. Next time, I'll cover building the login and sign-up screens.
Happy coding!