Mastering JavaScript Closures in Simple Terms
Title: Mastering JavaScript Closures in Simple Terms Introduction JavaScript, one of the most popular scripting languages in today's web development, fascinates developers with its power, flexibility, and versatility. But to master this language, one has to understand its fundamental concepts thoroughly. One important concept that often confuses new JavaScript developers is closures. So, let's demystify this concept and help you become a JavaScript maestro. What is a Closure? In JavaScript, a closure is a function that has access to its own scope, the outer function's scope, and the global scope. It is the combination of a function bundled together (enclosed) with references to its surrounding state (the lexical environment). Simply put, a closure gives you access to an outer function’s scope from an inner function. Lexical Environment To understand closures, it's crucial to comprehend what the lexical environment is. In JavaScript, a lexical environment is a data structure that holds variable and function declarations. It's used by the JavaScript engine to look up variables and functions, and it consists of two main components: the environment record and a reference to the outer environment. When a function is invoked, a new lexical environment is created to hold the local variables of that function. If that function contains nested functions, those functions hold a reference to their parent function’s environment, as well as the global environment. This is the basis of how closures work. Practical Examples Let's take a look at a practical example to understand closures better. function outerFunction() { let outerVariable = 'I am outside!'; function innerFunction() { console.log(outerVariable); } return innerFunction; } let myNewFunction = outerFunction(); myNewFunction(); // Logs: 'I am outside!' In this example, myNewFunction is a closure that consists of the innerFunction and the scope in which innerFunction was declared. When we invoke myNewFunction, it can still access outerVariable from its parent scope, even though outerFunction has finished executing. This is the power of closures - they remember the environment in which they were created. Interview Tricky Questions Closures are a common topic in JavaScript interviews. Here are a few tricky questions you might encounter: a) What will be the output of the following code? for (var i = 1; i
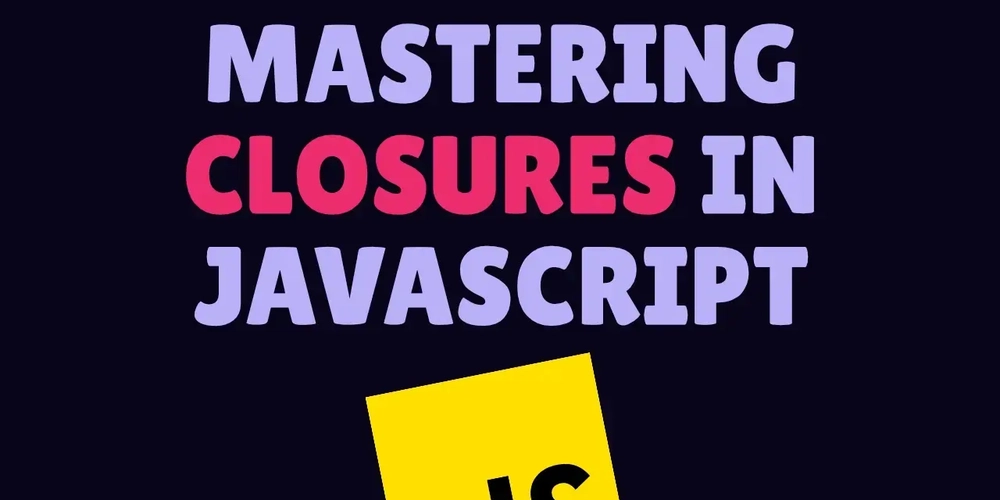
Title: Mastering JavaScript Closures in Simple Terms
Introduction
JavaScript, one of the most popular scripting languages in today's web development, fascinates developers with its power, flexibility, and versatility. But to master this language, one has to understand its fundamental concepts thoroughly. One important concept that often confuses new JavaScript developers is closures. So, let's demystify this concept and help you become a JavaScript maestro.
- What is a Closure?
In JavaScript, a closure is a function that has access to its own scope, the outer function's scope, and the global scope. It is the combination of a function bundled together (enclosed) with references to its surrounding state (the lexical environment). Simply put, a closure gives you access to an outer function’s scope from an inner function.
- Lexical Environment
To understand closures, it's crucial to comprehend what the lexical environment is. In JavaScript, a lexical environment is a data structure that holds variable and function declarations. It's used by the JavaScript engine to look up variables and functions, and it consists of two main components: the environment record and a reference to the outer environment.
When a function is invoked, a new lexical environment is created to hold the local variables of that function. If that function contains nested functions, those functions hold a reference to their parent function’s environment, as well as the global environment. This is the basis of how closures work.
- Practical Examples
Let's take a look at a practical example to understand closures better.
function outerFunction() {
let outerVariable = 'I am outside!';
function innerFunction() {
console.log(outerVariable);
}
return innerFunction;
}
let myNewFunction = outerFunction();
myNewFunction(); // Logs: 'I am outside!'
In this example, myNewFunction
is a closure that consists of the innerFunction
and the scope in which innerFunction
was declared. When we invoke myNewFunction
, it can still access outerVariable
from its parent scope, even though outerFunction
has finished executing. This is the power of closures - they remember the environment in which they were created.
- Interview Tricky Questions
Closures are a common topic in JavaScript interviews. Here are a few tricky questions you might encounter:
a) What will be the output of the following code?
for (var i = 1; i <= 5; i++) {
setTimeout(function() {
console.log(i);
}, i * 1000);
}
Many would expect this code to log the numbers 1 through 5, each after one second. However, it will actually log the number 6 five times, each after one second. This is because var
does not have block scope, and the callbacks capture the same single global scope.
b) How would you make this code log the numbers 1 through 5?
The answer is to use a closure. By creating an IIFE (Immediately Invoked Function Expression) inside the loop, we can create a new scope for each iteration, capturing the current value of i
.
for (var i = 1; i <= 5; i++) {
(function(i) {
setTimeout(function() {
console.log(i);
}, i * 1000);
})(i);
}
In this version, each IIFE creates a new scope, capturing the current value of i
. The setTimeout
callback then references this scope, logging the expected values.
Wrapping Up
Understanding closures is a crucial step on the path to mastering JavaScript. They offer powerful capabilities for managing scope and preserving state over time. Keep practicing and experimenting with closures, and you'll find them becoming second nature in no time.
Remember, as with all things JavaScript, it is practice and exploration that makes perfect. Happy coding!