Mastering Browser Dimension Properties (clientWidth, offsetWidth etc): Never to look back again
Introduction When working with web development, understanding how to measure and manipulate elements in the browser can be confusing. Properties like innerHeight, clientHeight, scrollHeight, and others have subtle but important differences. In this article, we'll demystify these properties and provide clear examples of when to use each one. 1. Window Properties Let's start with the properties of the window object that help us understand the browser viewport. window.innerHeight and window.innerWidth These properties give you the height and width of the browser's viewport (the visible area where your content displays), including scrollbars: window.innerHeight: The interior height of the browser window in pixels window.innerWidth: The interior width of the browser window in pixels // Get the viewport dimensions const viewportHeight = window.innerHeight; const viewportWidth = window.innerWidth; console.log(`Viewport: ${viewportWidth}px × ${viewportHeight}px`); window.outerHeight and window.outerWidth These measure the entire browser window, including toolbars, scrollbars, and other browser UI elements: window.outerHeight: The total height of the browser window window.outerWidth: The total width of the browser window // Get the dimensions of the entire browser window const windowHeight = window.outerHeight; const windowWidth = window.outerWidth; console.log(`Browser window: ${windowWidth}px × ${windowHeight}px`); window.scrollX and window.scrollY These properties tell you how many pixels the document has been scrolled: window.scrollX: Horizontal scroll position (pixels scrolled from the left) window.scrollY: Vertical scroll position (pixels scrolled from the top) // Track scroll position window.addEventListener('scroll', () => { console.log(`Scrolled: ${window.scrollX}px horizontally, ${window.scrollY}px vertically`); }); window.screen Properties The screen object provides information about the user's screen (not just the browser): screen.width and screen.height: Total screen dimensions screen.availWidth and screen.availHeight: Available screen space excluding system UI elements console.log(`Screen: ${screen.width}px × ${screen.height}px`); console.log(`Available screen: ${screen.availWidth}px × ${screen.availHeight}px`); 2. Element Properties Now let's look at the properties that can be used to measure HTML elements. element.clientHeight and element.clientWidth These properties measure the inner dimensions of an element: Include: padding Exclude: borders, margins, and scrollbars const box = document.getElementById('myBox'); console.log(`Inner content area: ${box.clientWidth}px × ${box.clientHeight}px`); element.offsetHeight and element.offsetWidth These properties measure the outer dimensions of an element: Include: padding, borders, and scrollbars Exclude: margins const box = document.getElementById('myBox'); console.log(`Element with borders: ${box.offsetWidth}px × ${box.offsetHeight}px`); element.scrollHeight and element.scrollWidth These properties measure the total size of an element's content, including parts that are not visible due to overflow: element.scrollHeight: The minimum height the element would need to fit all content without using a vertical scrollbar element.scrollWidth: The minimum width the element would need to fit all content without using a horizontal scrollbar const container = document.getElementById('scrollableContainer'); console.log(`Total content size: ${container.scrollWidth}px × ${container.scrollHeight}px`); element.scrollLeft and element.scrollTop These properties tell you how much of an element's content is scrolled: element.scrollLeft: Pixels scrolled from the left of the content element.scrollTop: Pixels scrolled from the top of the content const container = document.getElementById('scrollableContainer'); container.addEventListener('scroll', () => { console.log(`Element scrolled: ${container.scrollLeft}px from left, ${container.scrollTop}px from top`); }); element.getBoundingClientRect() This method returns a DOMRect object with properties like top, right, bottom, left, width, height, x, and y that describe the element's size and position relative to the viewport: const box = document.getElementById('myBox'); const rect = box.getBoundingClientRect(); console.log(`Position relative to viewport: top: ${rect.top}px, right: ${rect.right}px, bottom: ${rect.bottom}px, left: ${rect.left}px, width: ${rect.width}px, height: ${rect.height}px`); Practical Examples Example 1: Detecting if an Element is in the Viewport function isInViewport(element) { const rect = element.getBoundingClientRect(); return ( rect.top >= 0 && rect.left >= 0 && rect.bottom { // Calcu
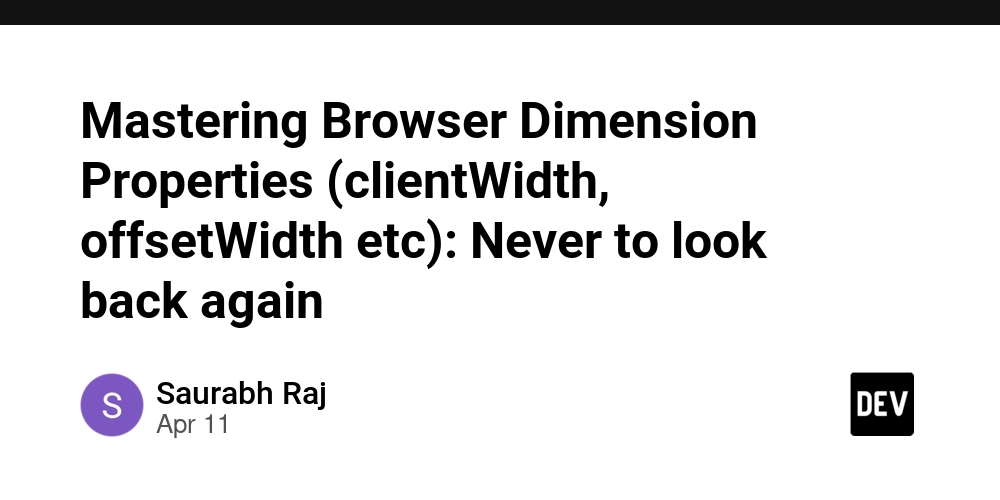
Introduction
When working with web development, understanding how to measure and manipulate elements in the browser can be confusing. Properties like innerHeight
, clientHeight
, scrollHeight
, and others have subtle but important differences. In this article, we'll demystify these properties and provide clear examples of when to use each one.
1. Window Properties
Let's start with the properties of the window
object that help us understand the browser viewport.
window.innerHeight
and window.innerWidth
These properties give you the height and width of the browser's viewport (the visible area where your content displays), including scrollbars:
-
window.innerHeight
: The interior height of the browser window in pixels -
window.innerWidth
: The interior width of the browser window in pixels
// Get the viewport dimensions
const viewportHeight = window.innerHeight;
const viewportWidth = window.innerWidth;
console.log(`Viewport: ${viewportWidth}px × ${viewportHeight}px`);
window.outerHeight
and window.outerWidth
These measure the entire browser window, including toolbars, scrollbars, and other browser UI elements:
-
window.outerHeight
: The total height of the browser window -
window.outerWidth
: The total width of the browser window
// Get the dimensions of the entire browser window
const windowHeight = window.outerHeight;
const windowWidth = window.outerWidth;
console.log(`Browser window: ${windowWidth}px × ${windowHeight}px`);
window.scrollX
and window.scrollY
These properties tell you how many pixels the document has been scrolled:
-
window.scrollX
: Horizontal scroll position (pixels scrolled from the left) -
window.scrollY
: Vertical scroll position (pixels scrolled from the top)
// Track scroll position
window.addEventListener('scroll', () => {
console.log(`Scrolled: ${window.scrollX}px horizontally, ${window.scrollY}px vertically`);
});
window.screen
Properties
The screen
object provides information about the user's screen (not just the browser):
-
screen.width
andscreen.height
: Total screen dimensions -
screen.availWidth
andscreen.availHeight
: Available screen space excluding system UI elements
console.log(`Screen: ${screen.width}px × ${screen.height}px`);
console.log(`Available screen: ${screen.availWidth}px × ${screen.availHeight}px`);
2. Element Properties
Now let's look at the properties that can be used to measure HTML elements.
element.clientHeight
and element.clientWidth
These properties measure the inner dimensions of an element:
- Include: padding
- Exclude: borders, margins, and scrollbars
const box = document.getElementById('myBox');
console.log(`Inner content area: ${box.clientWidth}px × ${box.clientHeight}px`);
element.offsetHeight
and element.offsetWidth
These properties measure the outer dimensions of an element:
- Include: padding, borders, and scrollbars
- Exclude: margins
const box = document.getElementById('myBox');
console.log(`Element with borders: ${box.offsetWidth}px × ${box.offsetHeight}px`);
element.scrollHeight
and element.scrollWidth
These properties measure the total size of an element's content, including parts that are not visible due to overflow:
-
element.scrollHeight
: The minimum height the element would need to fit all content without using a vertical scrollbar -
element.scrollWidth
: The minimum width the element would need to fit all content without using a horizontal scrollbar
const container = document.getElementById('scrollableContainer');
console.log(`Total content size: ${container.scrollWidth}px × ${container.scrollHeight}px`);
element.scrollLeft
and element.scrollTop
These properties tell you how much of an element's content is scrolled:
-
element.scrollLeft
: Pixels scrolled from the left of the content -
element.scrollTop
: Pixels scrolled from the top of the content
const container = document.getElementById('scrollableContainer');
container.addEventListener('scroll', () => {
console.log(`Element scrolled: ${container.scrollLeft}px from left, ${container.scrollTop}px from top`);
});
element.getBoundingClientRect()
This method returns a DOMRect
object with properties like top
, right
, bottom
, left
, width
, height
, x
, and y
that describe the element's size and position relative to the viewport:
const box = document.getElementById('myBox');
const rect = box.getBoundingClientRect();
console.log(`Position relative to viewport:
top: ${rect.top}px,
right: ${rect.right}px,
bottom: ${rect.bottom}px,
left: ${rect.left}px,
width: ${rect.width}px,
height: ${rect.height}px`);
Practical Examples
Example 1: Detecting if an Element is in the Viewport
function isInViewport(element) {
const rect = element.getBoundingClientRect();
return (
rect.top >= 0 &&
rect.left >= 0 &&
rect.bottom <= window.innerHeight &&
rect.right <= window.innerWidth
);
}
// Usage
const element = document.getElementById('targetElement');
if (isInViewport(element)) {
console.log('Element is fully visible in the viewport!');
}
Example 2: Creating an Infinite Scroll
window.addEventListener('scroll', () => {
// Calculate how far we've scrolled
const scrolled = window.scrollY;
// Calculate the total height of the page
const totalHeight = document.body.scrollHeight;
// Check if we're near the bottom (within 200px)
if (scrolled + window.innerHeight >= totalHeight - 200) {
// Load more content
loadMoreContent();
}
});
Example 3: Creating a Custom Scrollbar
function updateCustomScrollbar() {
const content = document.getElementById('content');
const scrollTrack = document.getElementById('scrollTrack');
const scrollThumb = document.getElementById('scrollThumb');
// Calculate the ratio of visible content to total content
const visibleRatio = content.clientHeight / content.scrollHeight;
// Set the scrollThumb height proportionally
scrollThumb.style.height = `${visibleRatio * 100}%`;
// Position the thumb based on scroll position
const scrollPercentage = content.scrollTop / (content.scrollHeight - content.clientHeight);
const thumbPosition = scrollPercentage * (scrollTrack.clientHeight - scrollThumb.clientHeight);
scrollThumb.style.top = `${thumbPosition}px`;
}
// Update on scroll and window resize
document.getElementById('content').addEventListener('scroll', updateCustomScrollbar);
window.addEventListener('resize', updateCustomScrollbar);
Example 4: Creating a Sticky Header
const header = document.getElementById('header');
const headerOffset = header.offsetTop;
window.addEventListener('scroll', () => {
if (window.scrollY >= headerOffset) {
header.classList.add('sticky');
} else {
header.classList.remove('sticky');
}
});
Example 5: Calculating Percentage Scrolled
function getScrollPercentage() {
// Calculate the maximum scrollable distance
const maxScroll = document.documentElement.scrollHeight - window.innerHeight;
// Calculate current scroll position as percentage
const percentScrolled = (window.scrollY / maxScroll) * 100;
return Math.min(100, Math.max(0, percentScrolled));
}
window.addEventListener('scroll', () => {
const progress = document.getElementById('progressBar');
progress.style.width = `${getScrollPercentage()}%`;
console.log(`Page scrolled: ${getScrollPercentage().toFixed(1)}%`);
});
Visual Representation
Here's how these various properties relate to each other for an element:
┌─────────────────────────────── ELEMENT ────────────────────────────────┐
│ │
│ margin │
│ ┌───────────────────────── offsetWidth/Height ──────────────────────┐ │
│ │ │ │
│ │ border │ │
│ │ ┌───────────────────── clientWidth/Height ────────────────────┐ │ │
│ │ │ │ │ │
│ │ │ padding │ │ │
│ │ │ ┌─────────────────── content area ──────────────────────┐ │ │ │
│ │ │ │ │ │ │ │
│ │ │ │ │ │ │ │
│ │ │ │ scrollWidth/Height │ │ │ │
│ │ │ │ (if content overflows) │ │ │ │
│ │ │ │ │ │ │ │
│ │ │ └────────────────────────────────────────────────────────┘ │ │ │
│ │ │ │ │ │
│ │ └──────────────────────────────────────────────────────────────┘ │ │
│ │ │ │
│ └────────────────────────────────────────────────────────────────────┘ │
│ │
└─────────────────────────────────────────────────────────────────────────┘
Test Your Understanding
-
Question: If an element has padding of 20px, a border of 5px, and content that's 200px × 150px, what would its
clientHeight
andoffsetHeight
be?-
Answer:
clientHeight
would be 190px (150px content + 40px padding), andoffsetHeight
would be 200px (150px content + 40px padding + 10px border).
-
Answer:
-
Question: What's the difference between
window.innerHeight
andelement.clientHeight
?-
Answer:
window.innerHeight
measures the viewport's height including scrollbars, whileelement.clientHeight
measures an element's content area plus padding, excluding borders and scrollbars.
-
Answer:
-
Question: How would you determine if a user has scrolled to the bottom of a page?
-
Answer: Compare
window.scrollY + window.innerHeight
withdocument.documentElement.scrollHeight
. If they're equal or very close, the user is at the bottom.
-
Answer: Compare
-
Question: If an element's
scrollHeight
is greater than itsclientHeight
, what does that indicate?- Answer: It indicates that the element has more content than can be displayed in its visible area, so it's scrollable.
-
Question: How would you find the absolute position of an element on the page (not just relative to the viewport)?
-
Answer: Combine
getBoundingClientRect()
with the current scroll position:const rect = element.getBoundingClientRect(); const absoluteTop = rect.top + window.scrollY; const absoluteLeft = rect.left + window.scrollX;
-
Answer: Combine
Conclusion
Understanding browser dimension properties is crucial for creating responsive, dynamic web applications. Each property serves a specific purpose:
-
Window properties (
innerHeight
,innerWidth
, etc.) help you understand the viewport. -
Element size properties (
clientHeight
,offsetHeight
,scrollHeight
, etc.) help you measure different aspects of elements. -
Position properties (
getBoundingClientRect()
,scrollTop
, etc.) help you determine where elements are located.
By mastering these properties, you can create more sophisticated user interfaces, implement features like infinite scrolling, sticky elements, and progress indicators, and solve common layout challenges.
Remember that these properties are read-only (except for scrollLeft
and scrollTop
), so they're used primarily for measurements rather than directly modifying sizes. Use CSS for styling and layout, and these JavaScript properties for measurement and calculations.
Happy coding!