Keep It Tight: Using Locally Scoped Helper Functions in Kotlin Use Cases
In modern Android and Kotlin development, especially when following clean architecture, we aim for focused, testable, and readable use cases. One subtle yet powerful technique to help us achieve that is placing small, tightly-bound helper functions inside a function like invoke() — rather than elevating them to the class level. Here’s how and why that matters. A Real Example Let’s say you have a feature that displays business hours for some sort of business like a dental clinic. You’ve got a BusinessSchedule proto model or API response and you want to display: "Open Today: 9:00 AM - 5:00 PM" if today’s hours are valid "Open Tomorrow: ..." if today is closed but tomorrow is open Or the next available day like "Open Thursday:..." Or a fallback like "Contact us for available business hours" You might implement a use case like this: class GetBusinessAvailabilityScheduleUseCase { operator fun invoke(businessSchedule: List): String { val dayNames = listOf("Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday") val today = LocalDate.now().dayOfWeek.value % 7 val tomorrow = (today + 1) % 7 fun hasValidHours(index: Int): Boolean { val schedule = businessSchedule.getOrNull(index) val open = schedule?.open?.value.orEmpty() val close = schedule?.close?.value.orEmpty() return open.isNotBlank() && close.isNotBlank() } fun formatSchedule(prefix: String, index: Int): String { val schedule = businessSchedule[index] val open = schedule.open?.value.orEmpty() val close = schedule.close?.value.orEmpty() return "$prefix: $open - $close" } return when { hasValidHours(today) -> formatSchedule("Open Today", today) hasValidHours(tomorrow) -> formatSchedule("Open Tomorrow", tomorrow) else -> { for (i in 0..6) { val index = (today + i + 1) % 7 if (hasValidHours(index)) { val dayName = dayNames[index] return formatSchedule("Open $dayName", index) } } "Contact us for available business hours" } } } } Why Use Local Helper Functions? 1. Encapsulation Helpers like hasValidHours() and formatSchedule() are implementation details. They don’t belong to the class's public API and aren’t useful anywhere else. Keeping them inside the invoke() keeps the rest of your class clean and focused. 2. Readability Keeping helper logic near the place it’s used creates a narrative flow in your code. You don’t have to scroll around to find where something like hasValidHours() is defined — it’s right there, scoped to the logic it supports. 3. Refactor Safety When helpers are class-level, other developers might start reusing them in unintended ways. If they’re scoped locally, the compiler ensures they only exist where they're needed, which makes future refactors less risky. 4. Access to Local Variables These functions can "close over" values like today, dayNames, or businessSchedule, without requiring additional parameters. This reduces parameter clutter and makes your logic more concise. When to Extract Them There’s a tipping point. If a helper: Is reused across multiple functions Becomes complex enough to deserve testing Or starts to feel like a domain concept on its own ... then it’s time to extract it to a private method or even a standalone utility. For example, hasValidHours() could be extracted to a shared ScheduleUtils if it's reused elsewhere. Final Thoughts This pattern — defining small, single-use helpers inside invoke() — is a clean, idiomatic way to write Kotlin use cases. It encourages encapsulation, local reasoning, and less API pollution, making your code more maintainable and testable over time. It’s a small trick — but when applied consistently, it has a big payoff. TL;DR Define helper functions inside invoke() if they’re only used there. It improves readability, encapsulation, and refactorability. Extract them only if they become reusable or complex.
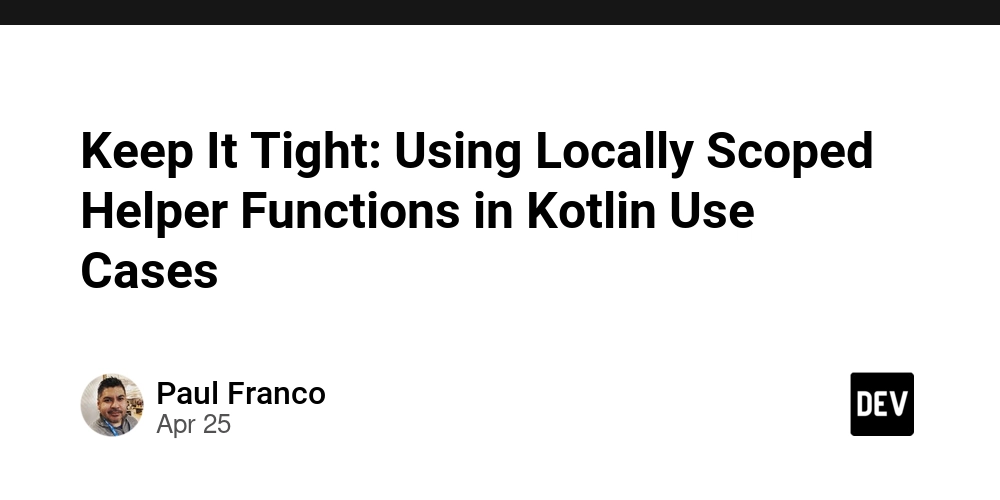
In modern Android and Kotlin development, especially when following clean architecture, we aim for focused, testable, and readable use cases. One subtle yet powerful technique to help us achieve that is placing small, tightly-bound helper functions inside a function like invoke()
— rather than elevating them to the class level.
Here’s how and why that matters.
A Real Example
Let’s say you have a feature that displays business hours for some sort of business like a dental clinic. You’ve got a BusinessSchedule
proto model or API response and you want to display:
"Open Today: 9:00 AM - 5:00 PM"
if today’s hours are valid"Open Tomorrow: ..."
if today is closed but tomorrow is openOr the next available day like
"Open Thursday:..."
Or a fallback like
"Contact us for available business hours"
You might implement a use case like this:
class GetBusinessAvailabilityScheduleUseCase {
operator fun invoke(businessSchedule: List): String {
val dayNames = listOf("Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday")
val today = LocalDate.now().dayOfWeek.value % 7
val tomorrow = (today + 1) % 7
fun hasValidHours(index: Int): Boolean {
val schedule = businessSchedule.getOrNull(index)
val open = schedule?.open?.value.orEmpty()
val close = schedule?.close?.value.orEmpty()
return open.isNotBlank() && close.isNotBlank()
}
fun formatSchedule(prefix: String, index: Int): String {
val schedule = businessSchedule[index]
val open = schedule.open?.value.orEmpty()
val close = schedule.close?.value.orEmpty()
return "$prefix: $open - $close"
}
return when {
hasValidHours(today) -> formatSchedule("Open Today", today)
hasValidHours(tomorrow) -> formatSchedule("Open Tomorrow", tomorrow)
else -> {
for (i in 0..6) {
val index = (today + i + 1) % 7
if (hasValidHours(index)) {
val dayName = dayNames[index]
return formatSchedule("Open $dayName", index)
}
}
"Contact us for available business hours"
}
}
}
}
Why Use Local Helper Functions?
1. Encapsulation
Helpers like hasValidHours()
and formatSchedule()
are implementation details. They don’t belong to the class's public API and aren’t useful anywhere else. Keeping them inside the invoke() keeps the rest of your class clean and focused.
2. Readability
Keeping helper logic near the place it’s used creates a narrative flow in your code. You don’t have to scroll around to find where something like hasValidHours()
is defined — it’s right there, scoped to the logic it supports.
3. Refactor Safety
When helpers are class-level, other developers might start reusing them in unintended ways. If they’re scoped locally, the compiler ensures they only exist where they're needed, which makes future refactors less risky.
4. Access to Local Variables
These functions can "close over" values like today
, dayNames
, or businessSchedule
, without requiring additional parameters. This reduces parameter clutter and makes your logic more concise.
When to Extract Them
There’s a tipping point. If a helper:
Is reused across multiple functions
Becomes complex enough to deserve testing
Or starts to feel like a domain concept on its own
... then it’s time to extract it to a private method or even a standalone utility.
For example, hasValidHours()
could be extracted to a shared ScheduleUtils
if it's reused elsewhere.
Final Thoughts
This pattern — defining small, single-use helpers inside invoke()
— is a clean, idiomatic way to write Kotlin use cases. It encourages encapsulation, local reasoning, and less API pollution, making your code more maintainable and testable over time.
It’s a small trick — but when applied consistently, it has a big payoff.
TL;DR
Define helper functions inside
invoke()
if they’re only used there.It improves readability, encapsulation, and refactorability.
Extract them only if they become reusable or complex.