JavaScript Memory Leaks: The Silent Killers and How to Fix Them
Memory leaks in JavaScript are like slow poison—they creep up unnoticed, degrade performance, and eventually crash your app. If your web app gets slower over time, consumes too much RAM, or crashes unexpectedly, you might be dealing with memory leaks. The worst part? They’re often invisible until it’s too late. In this post, we’ll uncover: ✔ What causes memory leaks in JS? ✔ How to detect them using Chrome DevTools ✔ Common leak patterns (and how to fix them) ✔ Best practices to prevent leaks Let’s dive in! What is a Memory Leak? A memory leak happens when your app unintentionally retains objects that are no longer needed, preventing garbage collection. Over time, this fills up memory, slowing down (or crashing) your app. Top 4 Memory Leak Culprits in JavaScript 1. Forgotten Timers & Intervals // Leak! setInterval keeps running even if component unmounts function startTimer() { setInterval(() => { console.log("Still running..."); }, 1000); } // Fix: Always clear intervals let intervalId; function startTimer() { intervalId = setInterval(() => { console.log("Running..."); }, 1000); } function stopTimer() { clearInterval(intervalId); }
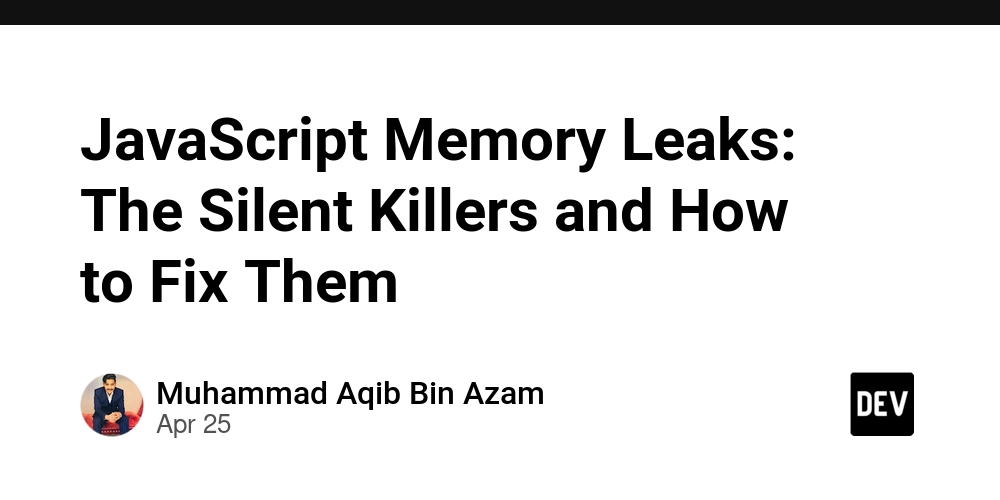
Memory leaks in JavaScript are like slow poison—they creep up unnoticed, degrade performance, and eventually crash your app.
If your web app gets slower over time, consumes too much RAM, or crashes unexpectedly, you might be dealing with memory leaks. The worst part? They’re often invisible until it’s too late.
In this post, we’ll uncover:
✔ What causes memory leaks in JS?
✔ How to detect them using Chrome DevTools
✔ Common leak patterns (and how to fix them)
✔ Best practices to prevent leaks
Let’s dive in!
What is a Memory Leak?
A memory leak happens when your app unintentionally retains objects that are no longer needed, preventing garbage collection. Over time, this fills up memory, slowing down (or crashing) your app.
Top 4 Memory Leak Culprits in JavaScript
1. Forgotten Timers & Intervals
// Leak! setInterval keeps running even if component unmounts
function startTimer() {
setInterval(() => {
console.log("Still running...");
}, 1000);
}
// Fix: Always clear intervals
let intervalId;
function startTimer() {
intervalId = setInterval(() => {
console.log("Running...");
}, 1000);
}
function stopTimer() {
clearInterval(intervalId);
}