JavaScript Mastery Showdown: Precision, Power, and Performance
Prologue: A Rivalry Ignited In the legendary halls of CodeBreak, two champions stand opposed. Chandan—Razör Sharp—crafts code with surgical precision. Avinash—Force Aggressor—overwhelms bugs with relentless power. Their rivalry is unspoken: each believes only they can truly master JavaScript’s deepest secrets. 1. Clash at Hoisting Highlands As dawn’s mist clears, both arrive at the fabled Hoisting Highlands. Razör Sharp smirks: console.log(ally); // undefined let ally = 'Companion'; Chandan (taunting): “Let vs. var—my let-bound variable keeps you guessing!” Force Aggressor grits his teeth: console.log(enemy); // ReferenceError var enemy = 'Nemesis'; Avinash (growling): “Var hoists fully—never underestimate brute legacy!” Visualization (Hoisting Flow): timeline title Variable and Function Hoisting 1900 : Declaration phase 2000 : Initialization phase Code Challenge: What will the output be if you replace let ally with var ally and why? 2. Duel in Closure Caverns Deep beneath the earth, they race to trap closures. Chandan fires first: function scout(name) { let stealth = true; return () => stealth && console.log(`${name} moves unseen`); } const ninja = scout('Razör'); ninja(); Chandan: “My private scope is untouchable!” Avinash counters: let counter = (function() { let count = 0; return () => ++count; })(); console.log(counter()); // 1 Avinash: “Stateful closures—powers I wield again and again!” Diagram (Closure Scope): graph LR A[forgeBlade invocation] B[(stealth variable)] A --> B --> C[strike function] Code Challenge: Write a closure that tracks and logs timestamps of each invocation. 3. Prototypes & Inheritance Arena On the high cliffs, prototypes shape-shift. Razör Sharp crafts class-based elegance: class Knight { constructor(rank) { this.rank = rank; } attack() { return this.rank * 12; } } Force Aggressor roars with raw prototype chaining: function Berserker(rage) { this.rage = rage; } Berserker.prototype.fury = function() { return this.rage + 50; }; Avinash: “Legacy chains endure where classes falter!” Diagram (Prototype Chain): classDiagram class Knight class Berserker Knight >WebAPI: setTimeout() WebAPI-->>CallbackQueue: callback CallbackQueue-->>Main: execute callback Code Challenge: Implement a Promise.allSettled() polyfill using only Promise and then. 5. Metaprogramming & Reflects They reach Metaprogramming Mountain. Razör Sharp wields Proxies: const sentinel = new Proxy({}, { get(target, prop) { console.log(`Accessing ${String(prop)}`); return Reflect.get(target, prop); } }); sentinel.secret; Chandan: “Every operation under my watch!” Force Aggressor calls upon Symbols and Reflect: const UNIQUE = Symbol('uniq'); const arsenal = { [UNIQUE]: 'Power' }; console.log(Reflect.get(arsenal, UNIQUE)); Avinash: “Symbols grant hidden strength!” Diagram (Proxy Trap): graph TD Proxy -->|get| Handler Handler -->|Reflect.get| Target Code Challenge: Build a Proxy that validates property assignments against a schema object. 6. Generators, Observables & Streams Showdown In the final amphitheater, they unleash advanced flows. Razör Sharp spins Generators: function* sequence() { yield 1; yield* [2,3]; } for (let num of sequence()) console.log(num); Avinash channels RxJS Observables: import { of } from 'rxjs'; of(1,2,3).subscribe(console.log); Avinash (triumphant): “Reactive power flows without pause!” Diagram (Generator vs Observable): gantt title Data Flow Comparison dateFormat YYYY-MM-DD section Generator Yield :a1, 2025-01-01, 1d section Observable Subscribe :a2, 2025-01-02, 1d Code Challenge: Create your own iterable using a Generator that yields Fibonacci numbers up to n. 7. The WebAssembly Warfront Beyond high-level script lies the domain of low-level performance. Razör Sharp and Force Aggressor summon WebAssembly modules: // example.wat (text format) (module (func $add (param $a i32) (param $b i32) (result i32) local.get $a local.get $b i32.add) (export "add" (func $add)) ) // in JavaScript fetch('example.wasm') .then(res => res.arrayBuffer()) .then(bytes => WebAssembly.instantiate(bytes)) .then(({ instance }) => console.log(instance.exports.add(2, 3))); // 5 Chandan: “Compile speed demons into the browser!” Avinash: “My force meets near-native power!” Diagram (JS ↔️ WASM Interop): flowchart LR JS -- arrayBuffer() --> WASM WASM -- exports --> JS Code Challenge: Write a small C function, compile to WASM, and integrate it to perform a computationally heavy task (e.g., matrix multiplication). Epilogue: A Respect Earned After countless clashes, they descend from CodeBreak’s summit, bruised but enlightened. Respect forged in rivalry, they part ways—each empowered by the
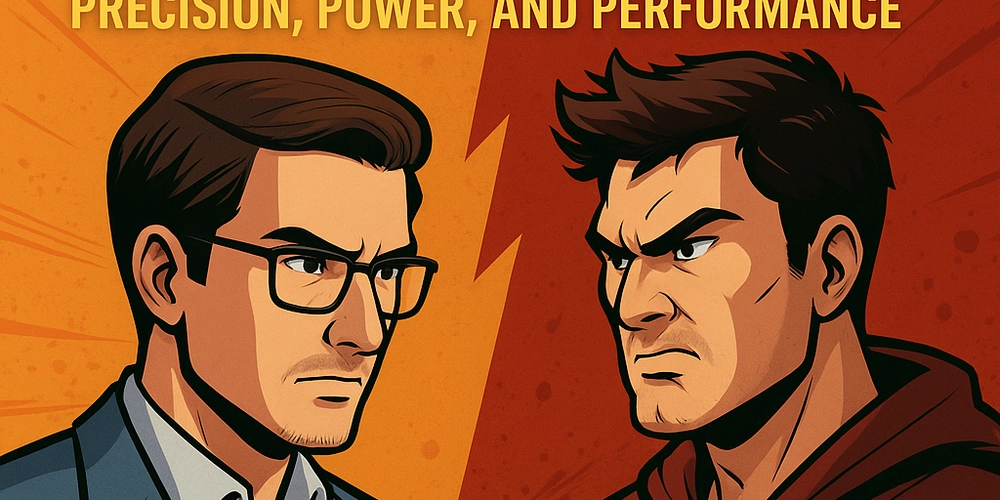
Prologue: A Rivalry Ignited
In the legendary halls of CodeBreak, two champions stand opposed. Chandan—Razör Sharp—crafts code with surgical precision. Avinash—Force Aggressor—overwhelms bugs with relentless power. Their rivalry is unspoken: each believes only they can truly master JavaScript’s deepest secrets.
1. Clash at Hoisting Highlands
As dawn’s mist clears, both arrive at the fabled Hoisting Highlands. Razör Sharp smirks:
console.log(ally); // undefined
let ally = 'Companion';
Chandan (taunting): “Let vs. var—my let-bound variable keeps you guessing!”
Force Aggressor grits his teeth:
console.log(enemy); // ReferenceError
var enemy = 'Nemesis';
Avinash (growling): “Var hoists fully—never underestimate brute legacy!”
Visualization (Hoisting Flow):
timeline
title Variable and Function Hoisting
1900 : Declaration phase
2000 : Initialization phase
Code Challenge: What will the output be if you replace let ally
with var ally
and why?
2. Duel in Closure Caverns
Deep beneath the earth, they race to trap closures. Chandan fires first:
function scout(name) {
let stealth = true;
return () => stealth && console.log(`${name} moves unseen`);
}
const ninja = scout('Razör');
ninja();
Chandan: “My private scope is untouchable!”
Avinash counters:
let counter = (function() {
let count = 0;
return () => ++count;
})();
console.log(counter()); // 1
Avinash: “Stateful closures—powers I wield again and again!”
Diagram (Closure Scope):
graph LR
A[forgeBlade invocation]
B[(stealth variable)]
A --> B --> C[strike function]
Code Challenge: Write a closure that tracks and logs timestamps of each invocation.
3. Prototypes & Inheritance Arena
On the high cliffs, prototypes shape-shift. Razör Sharp crafts class-based elegance:
class Knight {
constructor(rank) { this.rank = rank; }
attack() { return this.rank * 12; }
}
Force Aggressor roars with raw prototype chaining:
function Berserker(rage) { this.rage = rage; }
Berserker.prototype.fury = function() { return this.rage + 50; };
Avinash: “Legacy chains endure where classes falter!”
Diagram (Prototype Chain):
classDiagram
class Knight
class Berserker
Knight <|-- Berserker
Code Challenge: Extend both Knight and Berserker to share a defend()
method without duplicating code.
4. The Async Abyss & Concurrency Coliseum
Time warps beneath the event loop. Razör Sharp conjures async/await:
async function duel() {
await delay(1000);
console.log('Strike!');
}
duel();
Avinash strikes with Promises and race:
Promise.race([
fetch('/speed'),
timeout(500)
]).then(console.log);
Chandan: “My code reads like prophecy.”
Avinash: “My race finishes first!”
Diagram (Event Loop):
sequenceDiagram
participant Main
participant WebAPI
participant CallbackQueue
Main->>WebAPI: setTimeout()
WebAPI-->>CallbackQueue: callback
CallbackQueue-->>Main: execute callback
Code Challenge: Implement a Promise.allSettled()
polyfill using only Promise
and then
.
5. Metaprogramming & Reflects
They reach Metaprogramming Mountain. Razör Sharp wields Proxies:
const sentinel = new Proxy({}, {
get(target, prop) { console.log(`Accessing ${String(prop)}`); return Reflect.get(target, prop); }
});
sentinel.secret;
Chandan: “Every operation under my watch!”
Force Aggressor calls upon Symbols and Reflect:
const UNIQUE = Symbol('uniq');
const arsenal = { [UNIQUE]: 'Power' };
console.log(Reflect.get(arsenal, UNIQUE));
Avinash: “Symbols grant hidden strength!”
Diagram (Proxy Trap):
graph TD
Proxy -->|get| Handler
Handler -->|Reflect.get| Target
Code Challenge: Build a Proxy that validates property assignments against a schema object.
6. Generators, Observables & Streams Showdown
In the final amphitheater, they unleash advanced flows. Razör Sharp spins Generators:
function* sequence() { yield 1; yield* [2,3]; }
for (let num of sequence()) console.log(num);
Avinash channels RxJS Observables:
import { of } from 'rxjs';
of(1,2,3).subscribe(console.log);
Avinash (triumphant): “Reactive power flows without pause!”
Diagram (Generator vs Observable):
gantt
title Data Flow Comparison
dateFormat YYYY-MM-DD
section Generator
Yield :a1, 2025-01-01, 1d
section Observable
Subscribe :a2, 2025-01-02, 1d
Code Challenge: Create your own iterable using a Generator that yields Fibonacci numbers up to n
.
7. The WebAssembly Warfront
Beyond high-level script lies the domain of low-level performance. Razör Sharp and Force Aggressor summon WebAssembly modules:
// example.wat (text format)
(module
(func $add (param $a i32) (param $b i32) (result i32)
local.get $a
local.get $b
i32.add)
(export "add" (func $add))
)
// in JavaScript
fetch('example.wasm')
.then(res => res.arrayBuffer())
.then(bytes => WebAssembly.instantiate(bytes))
.then(({ instance }) => console.log(instance.exports.add(2, 3))); // 5
Chandan: “Compile speed demons into the browser!”
Avinash: “My force meets near-native power!”
Diagram (JS ↔️ WASM Interop):
flowchart LR
JS -- arrayBuffer() --> WASM
WASM -- exports --> JS
Code Challenge: Write a small C function, compile to WASM, and integrate it to perform a computationally heavy task (e.g., matrix multiplication).
Epilogue: A Respect Earned
After countless clashes, they descend from CodeBreak’s summit, bruised but enlightened. Respect forged in rivalry, they part ways—each empowered by the other’s strengths. Their duel echoes through every line of JavaScript written: precision tempered by force.
Which champion guides your code? Share your triumphs and trials below!