How to Write Better Commits and Pull Requests for Clean and Scalable Projects
When a team’s Git history is chaotic, collaboration becomes painful, code reviews get slower, and releases are harder to manage. On the other hand, clear commits and well-structured pull requests build trust, speed up teamwork, and prepare your project for real scalability. This article explains how to write better commits and pull requests — with concrete examples, professional practices, and real reasoning behind every rule. If you want your projects to be clean, scalable, and contributor-friendly, this guide is for you. Why It Matters: Beyond “Just Following Rules” Understandable history: Good commits act as a living documentation. Efficient teamwork: Pull requests are easier to review and merge. Reliable automation: Changelogs, releases, and CI/CD pipelines depend on well-structured commits and PRs. Professional image: Whether you work in open-source, freelancing, startups, or corporations, a clean workflow reflects your engineering standards. Good commits and pull requests are not bureaucracy. They are an investment in the long-term quality and speed of your project. 1. How to Write Effective Commit Messages The Structure You Should Use Follow the Conventional Commits format: type(scope): [module] short description Where: type: The type of change (feat, fix, docs, etc.). (scope): The part of the project (optional but recommended) like api, web, auth, dashboard. [module]: The feature or topic touched by the change. short description: A simple, imperative sentence starting with a verb ("add", "fix", "improve", "refactor", …) — no period at the end. Good Examples: feat(web): [authentication] add login form validation fix(api): [user-service] resolve user timezone conversion Bad Examples (too vague and useless for your future self or teammates): update code fix working on something Standard Types You Should Use Type Meaning feat Adding a new feature fix Fixing a bug docs Documentation changes only style Formatting changes (no logic change) refactor Code restructuring without behavior change test Adding or updating tests chore Maintenance tasks (builds, tooling) ci Changes to CI/CD pipelines build Build system or dependency changes perf Code changes that improve performance revert Revert a previous commit deps Updating dependencies These types make your Git history easier to filter, automate, and understand. Practical Commit Writing Tips Start with a verb: “add”, “fix”, “improve”, “refactor”, “remove”, “update”. Make each commit focused: One change = One commit. No WIP commits in main branches (main, develop). Explain why if necessary: Small clarification in the body of the commit is okay. Use clear, neutral English — avoid slang or jokes. Example with a body: refactor(api): [user-service] simplify timezone handling Remove duplicated timezone conversion logic. Use built-in Date API instead of manual parsing. 2. How to Write Effective Pull Requests A pull request is not just code. It is a conversation and a story. Treat it seriously, and you will speed up reviews, avoid misunderstandings, and improve team velocity. Pull Request Title Structure Follow this format: [Type]-APP-#Issue short description [Type]: Type of change (capitalized) [Feat], [Fix], [Docs], etc. APP: Major part of the project: WEB, API, MOBILE, DESKTOP, etc. #Issue: The issue number related to this PR. short description: Simple, clear, action-driven sentence. Examples: [Feat]-WEB-#1234 add user profile editing page [Fix]-API-#1250 fix incorrect password reset tokens Pull Request Description Template Always fill your PR description properly: ### What was done - Implemented user profile editing page - Added form validation and error handling ### Related issue Closes #1234 ### How to test - Go to `/profile/edit` - Try updating your name and profile picture - Submit invalid data to see validation errors ### Additional notes - No database migrations needed 3. Best Practices for Commits and PRs 1. Small, Focused Commits Each commit should represent one logical change. Split your work if necessary. This makes reviewing, reverting, or debugging much easier. 2. Small and Clear Pull Requests Ideal pull request size: ~300–500 lines changed. Big PRs are harder to review and more prone to bugs. If your PR is getting too big: Split into smaller PRs. Clearly explain why if you must keep it big. 3. Link Related Issues Always link your PR to its related issues using keywords like Closes #1234 or Fixes #1250. This keeps tracking automatic in GitHub, GitLab, Bitbucket, etc. 4. Keep Descriptions Professional and Practical No jokes or vague comments in commit or PR descriptions. Assume that someone you don’t know — your future teammates or even future you — will read these commits
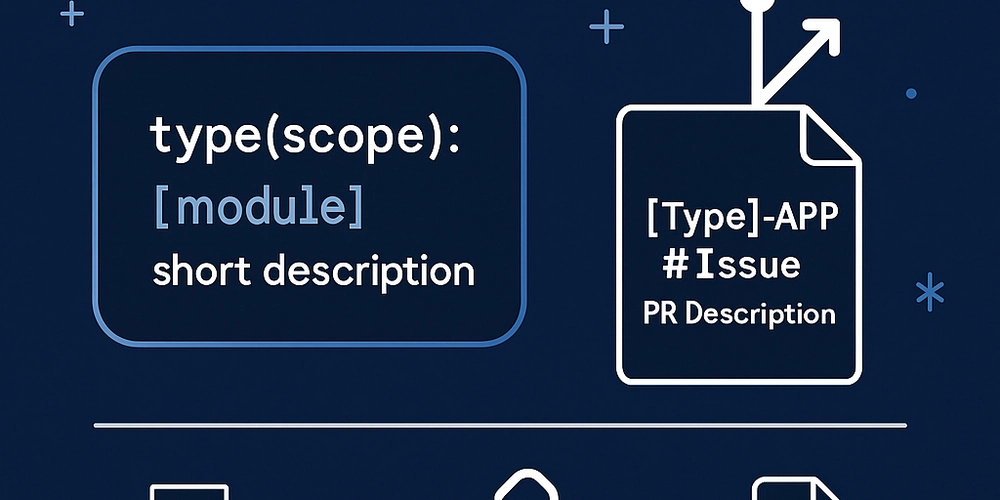
When a team’s Git history is chaotic, collaboration becomes painful, code reviews get slower, and releases are harder to manage.
On the other hand, clear commits and well-structured pull requests build trust, speed up teamwork, and prepare your project for real scalability.
This article explains how to write better commits and pull requests — with concrete examples, professional practices, and real reasoning behind every rule.
If you want your projects to be clean, scalable, and contributor-friendly, this guide is for you.
Why It Matters: Beyond “Just Following Rules”
- Understandable history: Good commits act as a living documentation.
- Efficient teamwork: Pull requests are easier to review and merge.
- Reliable automation: Changelogs, releases, and CI/CD pipelines depend on well-structured commits and PRs.
- Professional image: Whether you work in open-source, freelancing, startups, or corporations, a clean workflow reflects your engineering standards.
Good commits and pull requests are not bureaucracy.
They are an investment in the long-term quality and speed of your project.
1. How to Write Effective Commit Messages
The Structure You Should Use
Follow the Conventional Commits format:
type(scope): [module] short description
Where:
-
type
: The type of change (feat
,fix
,docs
, etc.). -
(scope)
: The part of the project (optional but recommended) likeapi
,web
,auth
,dashboard
. -
[module]
: The feature or topic touched by the change. -
short description
: A simple, imperative sentence starting with a verb ("add", "fix", "improve", "refactor", …) — no period at the end.
Good Examples:
feat(web): [authentication] add login form validation
fix(api): [user-service] resolve user timezone conversion
Bad Examples (too vague and useless for your future self or teammates):
update code
fix
working on something
Standard Types You Should Use
Type | Meaning |
---|---|
feat |
Adding a new feature |
fix |
Fixing a bug |
docs |
Documentation changes only |
style |
Formatting changes (no logic change) |
refactor |
Code restructuring without behavior change |
test |
Adding or updating tests |
chore |
Maintenance tasks (builds, tooling) |
ci |
Changes to CI/CD pipelines |
build |
Build system or dependency changes |
perf |
Code changes that improve performance |
revert |
Revert a previous commit |
deps |
Updating dependencies |
These types make your Git history easier to filter, automate, and understand.
Practical Commit Writing Tips
- Start with a verb: “add”, “fix”, “improve”, “refactor”, “remove”, “update”.
- Make each commit focused: One change = One commit.
-
No WIP commits in main branches (
main
,develop
). - Explain why if necessary: Small clarification in the body of the commit is okay.
- Use clear, neutral English — avoid slang or jokes.
Example with a body:
refactor(api): [user-service] simplify timezone handling
Remove duplicated timezone conversion logic.
Use built-in Date API instead of manual parsing.
2. How to Write Effective Pull Requests
A pull request is not just code. It is a conversation and a story.
Treat it seriously, and you will speed up reviews, avoid misunderstandings, and improve team velocity.
Pull Request Title Structure
Follow this format:
[Type]-APP-#Issue short description
-
[Type]
: Type of change (capitalized)[Feat]
,[Fix]
,[Docs]
, etc. -
APP
: Major part of the project:WEB
,API
,MOBILE
,DESKTOP
, etc. -
#Issue
: The issue number related to this PR. -
short description
: Simple, clear, action-driven sentence.
Examples:
[Feat]-WEB-#1234 add user profile editing page
[Fix]-API-#1250 fix incorrect password reset tokens
Pull Request Description Template
Always fill your PR description properly:
### What was done
- Implemented user profile editing page
- Added form validation and error handling
### Related issue
Closes #1234
### How to test
- Go to `/profile/edit`
- Try updating your name and profile picture
- Submit invalid data to see validation errors
### Additional notes
- No database migrations needed
3. Best Practices for Commits and PRs
1. Small, Focused Commits
Each commit should represent one logical change.
Split your work if necessary. This makes reviewing, reverting, or debugging much easier.
2. Small and Clear Pull Requests
Ideal pull request size: ~300–500 lines changed.
Big PRs are harder to review and more prone to bugs.
If your PR is getting too big:
- Split into smaller PRs.
- Clearly explain why if you must keep it big.
3. Link Related Issues
Always link your PR to its related issues using keywords like Closes #1234
or Fixes #1250
.
This keeps tracking automatic in GitHub, GitLab, Bitbucket, etc.
4. Keep Descriptions Professional and Practical
No jokes or vague comments in commit or PR descriptions.
Assume that someone you don’t know — your future teammates or even future you — will read these commits and PRs.
Stay clear, neutral, and professional.
5. Use Tools That Enforce Good Practices
- Commitlint + Husky: Force commit message conventions.
- Conventional Commits: Standardize commit types.
- Semantic Release: Automate versioning based on commits.
- Prettier, ESLint: Keep code clean before even pushing it.
Good tools make good practices automatic.
Discipline = Speed + Quality
Writing good commits and pull requests is not a waste of time —
it’s what makes professional software development faster, safer, and more scalable.
If you want to:
- Be a developer people enjoy working with
- Maintain clean codebases without fear
- Ship faster with fewer bugs
- Contribute easily to serious open-source projects
Start today: Write every commit and pull request as if you were reviewing it 6 months from now.
References and Further Reading
- Conventional Commits Specification
- Clean Code by Robert C. Martin
- How to Make a Git Commit Message Good
- GitHub Documentation on Closing Issues via PRs
If you found this helpful, share it with your team.
Clean projects build better futures.