Introduction to set theory with Python. Part II.
Topics: Set difference, complement of a set. On this post, we are talking about some other topics we didn´t covered on the previous one. https://dev.to/eaarroyo/introduction-to-set-theory-with-python-3jol Let's start with set difference. Set Difference The difference of two sets is writter as: A \ B or A - B. If A = {1, 2, 3} and B = {3, 4, 5}, the difference is a set of those objects of A that are not in B. e.g. A - B = {1, 2} In builder notation this would be: A\B = {x : x ∈ A and x ∉ B }; #Python Script A = {1, 2, 3} B = {3, 4, 5} A.difference(B) # Output is {1, 2} Complement of a set The complement of a set are all those elements of the universal set that are not on the other set. So, for this, we need to define a Universal set such as: U = {1, 2, 3, 4} A = {2, 3} A' = {1, 4} A' is the complement of A. Being U the universal set. It can be written in builder notation like this: A' = {x ∈ U and x ∉ A }; This present to us something really interesting: Being: U = {1, 2, 3, 4} A = {2, 3} U - A = {x : x ∈ U and x ∉ A }; U - A = {1, 4} And, A' = {x ∈ U and x ∉ A }; A' = {1, 4} So, A' = U - A; In python there is not a "Complement of a set" method, per se. So this would need to be done with the difference method. #Python script U = {1, 2, 3, 4} A = {2, 3} U.difference(A) # Output: {1, 4} Written by E. A. Arroyo
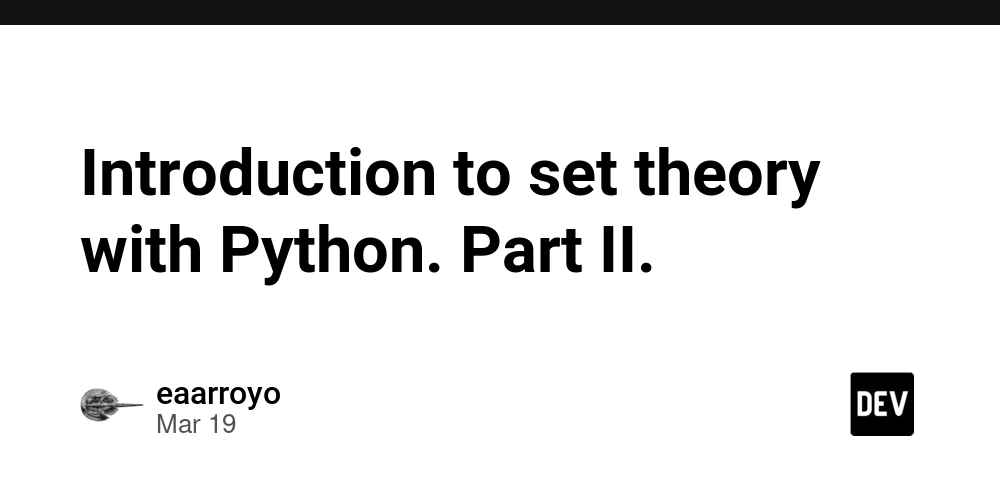
Topics: Set difference, complement of a set.
On this post, we are talking about some other topics we didn´t covered on the previous one.
https://dev.to/eaarroyo/introduction-to-set-theory-with-python-3jol
Let's start with set difference.
Set Difference
The difference of two sets is writter as:
A \ B or A - B.
If A = {1, 2, 3} and B = {3, 4, 5}, the difference is a set of those objects of A that are not in B.
e.g. A - B = {1, 2}
In builder notation this would be:
A\B = {x : x ∈ A and x ∉ B };
#Python Script
A = {1, 2, 3}
B = {3, 4, 5}
A.difference(B) # Output is {1, 2}
Complement of a set
The complement of a set are all those elements of the universal set that are not on the other set.
So, for this, we need to define a Universal set such as:
U = {1, 2, 3, 4}
A = {2, 3}
A' = {1, 4}
A' is the complement of A. Being U the universal set.
It can be written in builder notation like this:
A' = {x ∈ U and x ∉ A };
This present to us something really interesting:
Being:
U = {1, 2, 3, 4}
A = {2, 3}
U - A = {x : x ∈ U and x ∉ A };
U - A = {1, 4}
And,
A' = {x ∈ U and x ∉ A };
A' = {1, 4}
So, A' = U - A;
In python there is not a "Complement of a set" method, per se. So this would need to be done with the difference method.
#Python script
U = {1, 2, 3, 4}
A = {2, 3}
U.difference(A) # Output: {1, 4}
Written by E. A. Arroyo