Improving Eloquent: BuilderExtensions versus Scopes
Because of my comment on the Eloquent Builder post I wanted to start the Eloquent improvement with scopes. The scopes functionality Eloquent has two types of scopes, global and local. The global scopes allow developers to add Builder options to the model, to make them execute on every model query. There are two ways to add global scopes, by using the ScopedBy attribute or by using the addGlobalScope method in the booted method of the model. Beside using classes that implement the Scope interface, it is also possible to use a closure. protected static function booted(): void { // Scope interface class static::addGlobalScope(new AncientScope); // Scope closure static::addGlobalScope('ancient', function (Builder $builder) { $builder->where('created_at', 'identifiers = $identifiers; } } Because I want to use it as a class attribute and a method attribute both flags are added. To identify the different types of scopes I added a BuilderExtensionType enum. namespace Illuminate\Database\Eloquent; enum BuilderExtensionType : int { case REGULAR = 0; case REQUIRED = 1; case LOCALE = 2; } Now that I am set up, the changes of the Model and Builder classes can start. I added a builderExtensions property to the Model and an extensions property to the Builder. Next task is to get the BuilderExtension attributes that are added to a model. I discovered the best way was to add a bootBuilderExtensions method to the boot method. protected static function bootBuilderExtensions() { static::$builderExtensions = []; $reflectionClass = new ReflectionClass(static::class); $classBuilderExtensions = $reflectionClass->getAttributes(BuilderExtension::class); foreach ($classBuilderExtensions as $classBuilderExtension) { $arguments = $classBuilderExtension->getArguments(); $argumentsCount = count($arguments); if($argumentsCount == 0){ throw new LogicException('Builder Extension must have at least one argument'); } if($argumentsCount > 2) { throw new LogicException('Builder Extension has maximun two arguments'); } if($argumentsCount == 1){ $argument = $arguments[0]; if(is_string($argument)){ static::$builderExtensions[$argument] = BuilderExtensionType::REGULAR; } elseif(is_array($argument)){ if(is_string(array_key_first($argument))){ foreach($argument as $extension => $extensionType){ static::$builderExtensions[$extension] = $extensionType; } } else { foreach($argument as $extension){ static::$builderExtensions[$extension] = BuilderExtensionType::REGULAR; } } } } if($argumentsCount == 2){ static::$builderExtensions[$arguments[0]] = $arguments[1]; } } $methods = $reflectionClass->getMethods(\ReflectionMethod::IS_PUBLIC); foreach ($methods as $method) { $attributes = $method->getAttributes(BuilderExtension::class); if(count($attributes) > 1){ throw new LogicException('a method can have only one BuilderExtension attribute'); } if(count($attributes) == 1 && count($attributes[0]->getArguments()) > 0){ throw new LogicException('a method BuilderExtension attribute as no arguments'); } if(count($attributes) == 1) { static::$builderExtensions[$method->getName()] = BuilderExtensionType::LOCALE; } } } This will get every extension from the following example. #[BuilderExtension(ActiveBuilderExtension::class, BuilderExtensionType::REQUIRED)] #[BuilderExtension(OrderBuilderExtension::class)] // regular type is default // Or as an array. When the types are mixed the type is mandatory. #[BuilderExtension([ ActiveBuilderExtension::class => BuilderExtensionType::REQUIRED, OrderBuilderExtension::class => BuilderExtensionType::REGULAR ] )] class Test extends Model { protected $table = 'table'; #[BuilderExtension] public function active(Builder &$builder) { $builder->where('active', true); } } To move the required and regular scopes to the Builder class I had to add the change the newQuery method and add the registerBuilderExtensions method. public function newQuery() { return $this->registerBuilderExtensions($this->registerGlobalScopes($this->newQueryWithoutScopes())); } public function registerBuilderExtensions(Builder $builder) { $builderExtensions = array_filter(static::$builderExtensions, function($builderExtensionType, $builderExtens
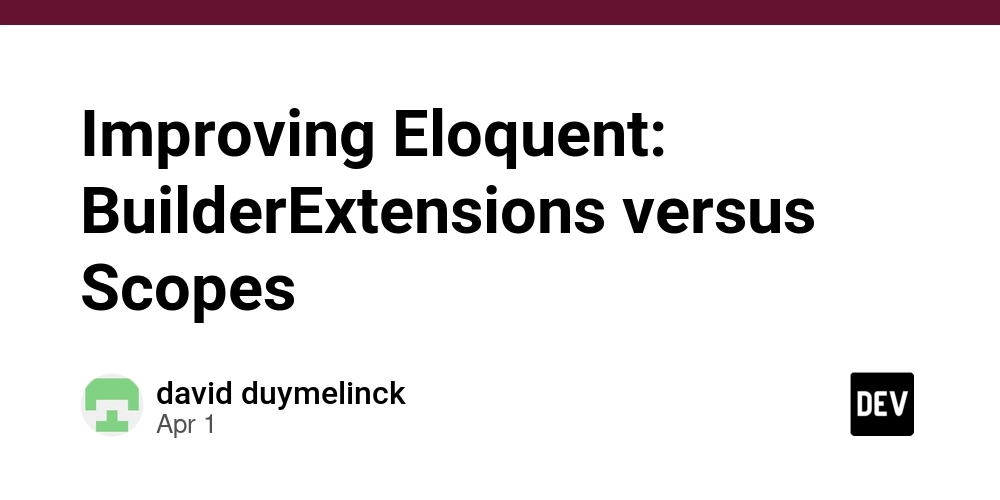
Because of my comment on the Eloquent Builder post I wanted to start the Eloquent improvement with scopes.
The scopes functionality
Eloquent has two types of scopes, global and local.
The global scopes allow developers to add Builder options to the model, to make them execute on every model query.
There are two ways to add global scopes, by using the ScopedBy
attribute or by using the addGlobalScope
method in the booted
method of the model.
Beside using classes that implement the Scope
interface, it is also possible to use a closure.
protected static function booted(): void
{
// Scope interface class
static::addGlobalScope(new AncientScope);
// Scope closure
static::addGlobalScope('ancient', function (Builder $builder) {
$builder->where('created_at', '<', now()->subYears(2000));
});
}
The local scopes are only executed when they are added as a method in the model fluent API chain. In this examplePost(['title' => 'Test'])->isDraft()->save()
is the isDraft
method the local scope.
There are two ways of adding local scopes. Using a method that is prefixed with scope or with a Scope
attribute. The latter is probably a lesser known method because it is not in the documentation. There is not even a test for this option in the codebase.
The problem I see with scopes
The main problem I see with scopes is that local scopes can't be reused by other models without using traits. The Scope
interface exists to make global scopes reusable, why not use that to make local scopes reusable?
I'm not a big fan of prefixing methods to identify them as a special method, that is the job of attributes. When I started the changes I wasn't aware of the Scope
attribute, that makes it slightly better.
The BuilderExtensions POC
At first I wanted to remove the scopes and replace it with my changes. But because that would break a lot of functionality I left it as it is and add my changes.
The first thing I had to decide after that was the name. Because the scopes are mainly handled by the Eloquent\Builder class it felt logical to choose BuilderExtension as the name. Maybe it exposes too much information from the inner workings of the Model
class, but I think the Builder pattern is generic enough to use as a name.
The first task is to implement the global scopes. So I needed a BuilderExtension interface.
namespace Illuminate\Database\Eloquent;
interface BuilderExtension
{
public function apply(Builder &$builder);
}
The global scope does something weird by using a callScope
method with a closure that executes the apply
method of the scope.
I though it would be less code to pass the Builder
class by reference.
The function of the global scope way might become clear when I make further changes.
Next on the list is creating a BuilderExtension
attribute
namespace Illuminate\Database\Eloquent\Attributes;
use Attribute;
#[Attribute(Attribute::TARGET_METHOD | Attribute::TARGET_CLASS | Attribute::IS_REPEATABLE)]
class BuilderExtension
{
public mixed $identifiers;
public function __construct(mixed ...$identifiers)
{
$this->identifiers = $identifiers;
}
}
Because I want to use it as a class attribute and a method attribute both flags are added.
To identify the different types of scopes I added a BuilderExtensionType enum.
namespace Illuminate\Database\Eloquent;
enum BuilderExtensionType : int
{
case REGULAR = 0;
case REQUIRED = 1;
case LOCALE = 2;
}
Now that I am set up, the changes of the Model
and Builder
classes can start.
I added a builderExtensions
property to the Model
and an extensions
property to the Builder
.
Next task is to get the BuilderExtension
attributes that are added to a model.
I discovered the best way was to add a bootBuilderExtensions
method to the boot
method.
protected static function bootBuilderExtensions()
{
static::$builderExtensions = [];
$reflectionClass = new ReflectionClass(static::class);
$classBuilderExtensions = $reflectionClass->getAttributes(BuilderExtension::class);
foreach ($classBuilderExtensions as $classBuilderExtension) {
$arguments = $classBuilderExtension->getArguments();
$argumentsCount = count($arguments);
if($argumentsCount == 0){
throw new LogicException('Builder Extension must have at least one argument');
}
if($argumentsCount > 2) {
throw new LogicException('Builder Extension has maximun two arguments');
}
if($argumentsCount == 1){
$argument = $arguments[0];
if(is_string($argument)){
static::$builderExtensions[$argument] = BuilderExtensionType::REGULAR;
} elseif(is_array($argument)){
if(is_string(array_key_first($argument))){
foreach($argument as $extension => $extensionType){
static::$builderExtensions[$extension] = $extensionType;
}
} else {
foreach($argument as $extension){
static::$builderExtensions[$extension] = BuilderExtensionType::REGULAR;
}
}
}
}
if($argumentsCount == 2){
static::$builderExtensions[$arguments[0]] = $arguments[1];
}
}
$methods = $reflectionClass->getMethods(\ReflectionMethod::IS_PUBLIC);
foreach ($methods as $method) {
$attributes = $method->getAttributes(BuilderExtension::class);
if(count($attributes) > 1){
throw new LogicException('a method can have only one BuilderExtension attribute');
}
if(count($attributes) == 1 && count($attributes[0]->getArguments()) > 0){
throw new LogicException('a method BuilderExtension attribute as no arguments');
}
if(count($attributes) == 1) {
static::$builderExtensions[$method->getName()] = BuilderExtensionType::LOCALE;
}
}
}
This will get every extension from the following example.
#[BuilderExtension(ActiveBuilderExtension::class, BuilderExtensionType::REQUIRED)]
#[BuilderExtension(OrderBuilderExtension::class)] // regular type is default
// Or as an array. When the types are mixed the type is mandatory.
#[BuilderExtension([
ActiveBuilderExtension::class => BuilderExtensionType::REQUIRED,
OrderBuilderExtension::class => BuilderExtensionType::REGULAR
]
)]
class Test extends Model
{
protected $table = 'table';
#[BuilderExtension]
public function active(Builder &$builder)
{
$builder->where('active', true);
}
}
To move the required and regular scopes to the Builder
class I had to add the change the newQuery
method and add the registerBuilderExtensions
method.
public function newQuery()
{
return $this->registerBuilderExtensions($this->registerGlobalScopes($this->newQueryWithoutScopes()));
}
public function registerBuilderExtensions(Builder $builder)
{
$builderExtensions = array_filter(static::$builderExtensions, function($builderExtensionType, $builderExtension) {
return in_array($builderExtensionType, [BuilderExtensionType::REGULAR, BuilderExtensionType::REQUIRED]);
}, ARRAY_FILTER_USE_BOTH);
foreach ($builderExtensions as $builderExtension => $builderExtensionType) {
$builder->addBuilderExtension($builderExtension, $builderExtensionType);
}
return $builder;
}
In the Builder
class the applyScopes
method is called when a query is being build. So i added my extensions. And that is it to mimic the global scopes.
public function applyScopes()
{
$builder = clone $this;
$requiredBuilderExtensions = array_filter($this->extensions, function($extensionType, $extension) {
return $extensionType == BuilderExtensionType::REQUIRED;
}, ARRAY_FILTER_USE_BOTH);
if(count($requiredBuilderExtensions) > 0) {
foreach ($requiredBuilderExtensions as $extension => $extensionType) {
new $extension()->apply($builder);
}
}
// original code from here
if (! $this->scopes) {
return $builder;
}
}
Local scopes functionality
By using the regular and local extension types there are two different paths to execute them.
In the __call
method of the Builder
I added;
if($this->hasLocalBuilderExtension($method)) {
return $this->callLocalBuilderExtension($method, $parameters);
}
if($this->hasRegularBuilderExtension($method)) {
return $this->callRegularBuilderExtension($method, $parameters);
}
And the methods are as follows.
public function hasLocalBuilderExtension($builderExtension) : bool
{
return $this->model && $this->model->hasLocalBuilderExtension($builderExtension);
}
public function hasRegularBuilderExtension($builderExtension) : bool
{
$regularBuilderExtensions = array_filter($this->extensions, function ($extensionType, $extension){
return $extensionType == BuilderExtensionType::REGULAR;
}, ARRAY_FILTER_USE_BOTH);
foreach ($regularBuilderExtensions as $extension => $extensionType) {
if(str_contains(strtolower($extension), strtolower($builderExtension))) {
return true;
}
}
return false;
}
protected function callLocalBuilderExtension($builderExtension, array $parameters = []) : Builder
{
$this->model->$builderExtension($this, $parameters);
return $this;
}
protected function callRegularBuilderExtension($builderExtension, array $parameters = []) : Builder
{
$regularBuilderExtensions = array_filter($this->extensions, function ($extensionType, $extension) use($builderExtension) {
return $extensionType == BuilderExtensionType::REGULAR &&
str_contains(strtolower($extension), strtolower($builderExtension));
}, ARRAY_FILTER_USE_BOTH);
if(count($regularBuilderExtensions) == 0) {
throw new \LogicException('callRegularBuilderExtension method called without extension exist chack.');
}
$extension = array_key_first($regularBuilderExtensions);
new $extension()->apply($this);
return $this;
}
Because the local method is in the model I needed the hasLocalBuilderExtension
method.
public function hasLocalBuilderExtension($name) : bool
{
return isset(static::$builderExtensions[$name]) && static::$builderExtensions[$name] == BuilderExtensionType::LOCALE;
}
And that is it for the local scopes functionality.
Conclusion
For the people who want to see it working, check the Github commit. I also added tests.
This is just scraping the surface of the scopes functionality. The main thing I wanted to achieve for now is a more straight forward way to work with scopes.