IIFE in Javascript
Have you ever come across a function in JavaScript that's wrapped in parentheses and immediately executed? It might look strange at first, but it’s actually one of the most elegant patterns in JavaScript called as IIFE. In this blog post, we'll break down what IIFE means, why it's useful, and how you can use it in your codebase. What is an IIFE? IIFE stands for Immediately Invoked Function Expression. It’s a function that runs immediately after it is defined. Basic Syntax (function() { console.log("IIFE runs!"); })(); You can also use an arrow function: (() => { console.log("Arrow IIFE runs!"); })(); Why Use an IIFE? 1. Avoid Global Scope Pollution Variables declared inside an IIFE can’t be accessed from the outside, keeping the global namespace clean. 2. Encapsulation You can hide variables and functions from the outside world, which is great for creating private scopes. 3. Safe Initialization Code IIFEs are often used for code that needs to run once and not leave any trace in the global scope. Real-World Example Here’s a simple counter module using an IIFE: const counter = (function() { let count = 0; return { increment: () => ++count, getCount: () => count }; })(); console.log(counter.increment()); // 1 console.log(counter.getCount()); // 1 In this example, the count variable is private. Only the returned methods can interact with it. Types of IIFE Traditional Function IIFE (function() { console.log("Traditional IIFE"); })(); Arrow Function IIFE (() => { console.log("Arrow IIFE"); })(); IIFE in a React Component You might not use IIFE very often in React, but here’s a useful scenario: rendering logic that should execute immediately inside JSX. JSX Example import React from 'react'; function UserGreeting({ user }) { return ( {(() => { if (user?.isLoggedIn) { return Welcome back, {user.name}!; } else { return Please sign in.; } })()} ); } export default UserGreeting; Here, the IIFE is used inside JSX to conditionally render different outputs using more complex logic than what a ternary operator might allow. Async IIFE Inside useEffect A common React pattern is making API calls inside useEffect. Since useEffect doesn't accept async functions directly, developers often define an inner async function and then call it. Instead, you can use an IIFE: Traditional Approach useEffect(() => { const fetchApiCall = async () => { try { const { data } = await axios.get('apiurl'); setData(data); } catch (error) { setError(error.message); } }; fetchApiCall(); }, []); Using IIFE useEffect(() => { (async () => { try { const { data } = await axios.get('apiurl'); setData(data); } catch (error) { setError(error.message); } })(); }, []); ✅ Why Use an IIFE Here? Avoids having to separately define and call an inner function Keeps the logic compact and scoped inside useEffect Looks cleaner, especially for short one-off fetch operations Common Use Cases Wrapping library or plugin code Avoiding variable conflicts Creating isolated scope in loops Polyfills Async functions in useEffect (React) IIFE vs Modern Alternatives With the introduction of ES6, we now have better ways to manage scope: Modules: ES6 modules have their own scope. Block Scope: let and const allow block-level scoping. Closures: More powerful and flexible for state management. Even though these alternatives exist, understanding IIFEs is essential for reading legacy code and building a solid foundation in JavaScript. Conclusion IIFE is a classic pattern in JavaScript that solves real problems like variable scope management and safe initialization. Even in the modern JS ecosystem, it remains a valuable concept to understand and appreciate. Next time you see a function wrapped in parentheses and immediately called, you’ll know exactly what's going on—and why it’s such a neat trick. Happy coding! Bonus Tip: Try converting some of your global-scope-heavy scripts into IIFEs and see the difference it makes in structure and readability. Feel free to share your thoughts or use-cases for IIFE in the comments below!
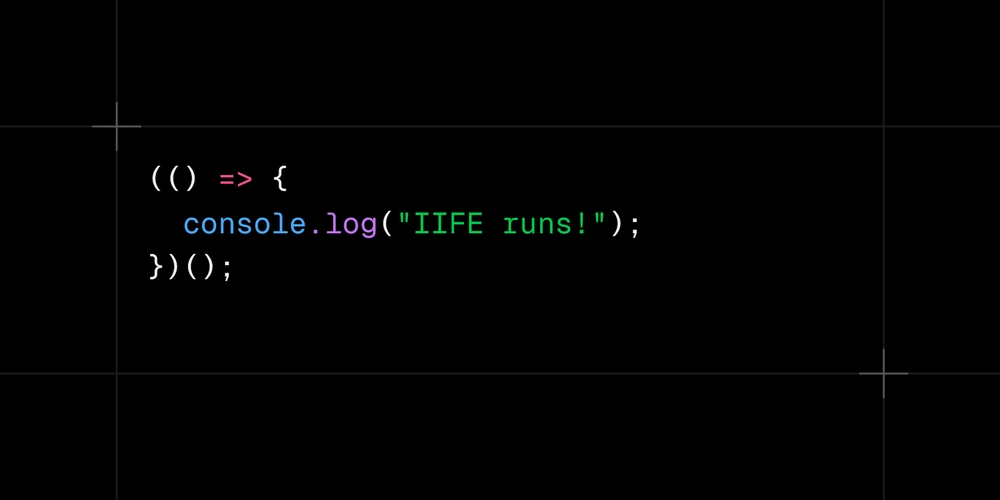
Have you ever come across a function in JavaScript that's wrapped in parentheses and immediately executed? It might look strange at first, but it’s actually one of the most elegant patterns in JavaScript called as IIFE.
In this blog post, we'll break down what IIFE means, why it's useful, and how you can use it in your codebase.
What is an IIFE?
IIFE stands for Immediately Invoked Function Expression. It’s a function that runs immediately after it is defined.
Basic Syntax
(function() {
console.log("IIFE runs!");
})();
You can also use an arrow function:
(() => {
console.log("Arrow IIFE runs!");
})();
Why Use an IIFE?
1. Avoid Global Scope Pollution
Variables declared inside an IIFE can’t be accessed from the outside, keeping the global namespace clean.
2. Encapsulation
You can hide variables and functions from the outside world, which is great for creating private scopes.
3. Safe Initialization Code
IIFEs are often used for code that needs to run once and not leave any trace in the global scope.
Real-World Example
Here’s a simple counter module using an IIFE:
const counter = (function() {
let count = 0;
return {
increment: () => ++count,
getCount: () => count
};
})();
console.log(counter.increment()); // 1
console.log(counter.getCount()); // 1
In this example, the count
variable is private. Only the returned methods can interact with it.
Types of IIFE
Traditional Function IIFE
(function() {
console.log("Traditional IIFE");
})();
Arrow Function IIFE
(() => {
console.log("Arrow IIFE");
})();
IIFE in a React Component
You might not use IIFE very often in React, but here’s a useful scenario: rendering logic that should execute immediately inside JSX.
JSX Example
import React from 'react';
function UserGreeting({ user }) {
return (
<div>
{(() => {
if (user?.isLoggedIn) {
return <h2>Welcome back, {user.name}!h2>;
} else {
return <h2>Please sign in.h2>;
}
})()}
div>
);
}
export default UserGreeting;
Here, the IIFE is used inside JSX to conditionally render different outputs using more complex logic than what a ternary operator might allow.
Async IIFE Inside useEffect
A common React pattern is making API calls inside useEffect
. Since useEffect
doesn't accept async functions directly, developers often define an inner async function and then call it. Instead, you can use an IIFE:
Traditional Approach
useEffect(() => {
const fetchApiCall = async () => {
try {
const { data } = await axios.get('apiurl');
setData(data);
} catch (error) {
setError(error.message);
}
};
fetchApiCall();
}, []);
Using IIFE
useEffect(() => {
(async () => {
try {
const { data } = await axios.get('apiurl');
setData(data);
} catch (error) {
setError(error.message);
}
})();
}, []);
✅ Why Use an IIFE Here?
- Avoids having to separately define and call an inner function
- Keeps the logic compact and scoped inside
useEffect
- Looks cleaner, especially for short one-off fetch operations
Common Use Cases
- Wrapping library or plugin code
- Avoiding variable conflicts
- Creating isolated scope in loops
- Polyfills
- Async functions in
useEffect
(React)
IIFE vs Modern Alternatives
With the introduction of ES6, we now have better ways to manage scope:
- Modules: ES6 modules have their own scope.
-
Block Scope:
let
andconst
allow block-level scoping. - Closures: More powerful and flexible for state management.
Even though these alternatives exist, understanding IIFEs is essential for reading legacy code and building a solid foundation in JavaScript.
Conclusion
IIFE is a classic pattern in JavaScript that solves real problems like variable scope management and safe initialization. Even in the modern JS ecosystem, it remains a valuable concept to understand and appreciate.
Next time you see a function wrapped in parentheses and immediately called, you’ll know exactly what's going on—and why it’s such a neat trick.
Happy coding!
Bonus Tip: Try converting some of your global-scope-heavy scripts into IIFEs and see the difference it makes in structure and readability.
Feel free to share your thoughts or use-cases for IIFE in the comments below!