How to Use Reference and Output Parameters in Java
Introduction In programming, passing parameters to functions is crucial for manipulating data effectively. Java, unlike some languages such as C#, does not support reference parameters in the same way. Instead, Java uses pass-by-value semantics, which means that when you pass a variable to a method, a copy of that variable is sent. However, there are ways to achieve similar functionality using mutable objects or wrapper classes. In this article, we'll explore how to simulate reference-like behavior in Java using simple examples and provide a clear understanding of handling output parameters in functions. Understanding Reference Parameters in Java When you define a method parameter in Java, it typically receives a copy of the variable's value. If you want to modify the value of an object referenced by a parameter, you must use a mutable object. For example, consider a scenario where you want to change a string inside a method: Example of a Simulated Reference Parameter Here’s how you might attempt to simulate a reference parameter behavior with a mutable structure: class StringWrapper { String value; StringWrapper(String value) { this.value = value; } } void changeString(StringWrapper str) { str.value = "def"; } void main() { StringWrapper abc = new StringWrapper("abc"); changeString(abc); System.out.println(abc.value); // prints "def" } In this example, we created a StringWrapper class to encapsulate the string. By passing an instance of StringWrapper to the changeString method, modifications made within the method are reflected outside of it, similar to a reference parameter. How to Use Output Parameters in Java While Java does not have a built-in mechanism for output parameters as seen in some other languages like C#, we can achieve the same result using a return statement or using mutable objects. Here's an example: Example of an Output Parameter Simulation Suppose you want to return multiple values or an altered variable from a method without using return: class StringWrapper { String value; StringWrapper(String value) { this.value = value; } } void changeString(StringWrapper str) { str.value = "def"; } void main() { StringWrapper abc = new StringWrapper(null); changeString(abc); System.out.println(abc.value); // prints "def" } Alternatively, you can simply return a value: String changeString() { return "def"; } void main() { String abc = changeString(); System.out.println(abc); // prints "def" } This approach of explicitly returning a value can be clearer and less error-prone as your logic indicates the purpose of returning the new value directly. Advantages of Using Mutable Objects Utilizing mutable objects for simulating reference and output parameters can help keep your code clean and logical. However, care should be taken to avoid unintended side effects, as it can lead to less manageable code. Always aim for clear data flow in your code, making it easier for others (and you) to read and maintain. Frequently Asked Questions Can Java support true reference parameters? No, Java does not support true reference parameters like some languages do, but you can emulate it using mutable objects. Why should I avoid using mutable shared state? Mutable shared state can lead to issues such as race conditions and bugs in a multi-threaded environment. Try to minimize mutable shared state wherever possible for cleaner, easier maintenance. Is using wrapper classes a good practice? Using wrapper classes can be a good practice if you need to modify data within a function. It provides a clear structure and reduces confusion compared to using primitive types directly. Conclusion While Java does not support reference and output parameters natively as seen in other languages, understanding how to work with mutable objects allows you to mimic these behaviors. By implementing the outlined strategies, you can effectively manage data flow within your applications and accommodate scenarios that require similar functionality. Remember to opt for clear and maintainable patterns to ease future modifications and debugging.
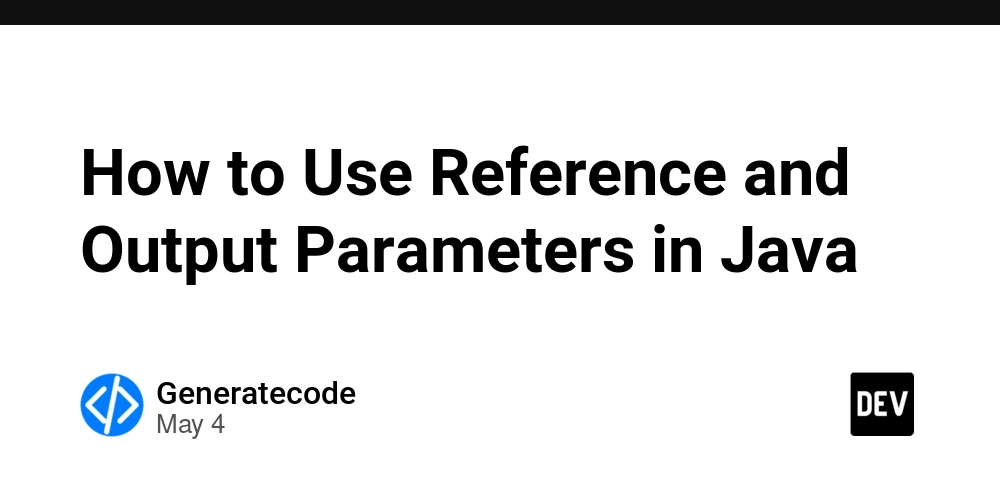
Introduction
In programming, passing parameters to functions is crucial for manipulating data effectively. Java, unlike some languages such as C#, does not support reference parameters in the same way. Instead, Java uses pass-by-value semantics, which means that when you pass a variable to a method, a copy of that variable is sent. However, there are ways to achieve similar functionality using mutable objects or wrapper classes. In this article, we'll explore how to simulate reference-like behavior in Java using simple examples and provide a clear understanding of handling output parameters in functions.
Understanding Reference Parameters in Java
When you define a method parameter in Java, it typically receives a copy of the variable's value. If you want to modify the value of an object referenced by a parameter, you must use a mutable object. For example, consider a scenario where you want to change a string inside a method:
Example of a Simulated Reference Parameter
Here’s how you might attempt to simulate a reference parameter behavior with a mutable structure:
class StringWrapper {
String value;
StringWrapper(String value) { this.value = value; }
}
void changeString(StringWrapper str) {
str.value = "def";
}
void main() {
StringWrapper abc = new StringWrapper("abc");
changeString(abc);
System.out.println(abc.value); // prints "def"
}
In this example, we created a StringWrapper
class to encapsulate the string. By passing an instance of StringWrapper
to the changeString
method, modifications made within the method are reflected outside of it, similar to a reference parameter.
How to Use Output Parameters in Java
While Java does not have a built-in mechanism for output parameters as seen in some other languages like C#, we can achieve the same result using a return statement or using mutable objects. Here's an example:
Example of an Output Parameter Simulation
Suppose you want to return multiple values or an altered variable from a method without using return:
class StringWrapper {
String value;
StringWrapper(String value) { this.value = value; }
}
void changeString(StringWrapper str) {
str.value = "def";
}
void main() {
StringWrapper abc = new StringWrapper(null);
changeString(abc);
System.out.println(abc.value); // prints "def"
}
Alternatively, you can simply return a value:
String changeString() {
return "def";
}
void main() {
String abc = changeString();
System.out.println(abc); // prints "def"
}
This approach of explicitly returning a value can be clearer and less error-prone as your logic indicates the purpose of returning the new value directly.
Advantages of Using Mutable Objects
Utilizing mutable objects for simulating reference and output parameters can help keep your code clean and logical. However, care should be taken to avoid unintended side effects, as it can lead to less manageable code. Always aim for clear data flow in your code, making it easier for others (and you) to read and maintain.
Frequently Asked Questions
Can Java support true reference parameters?
No, Java does not support true reference parameters like some languages do, but you can emulate it using mutable objects.
Why should I avoid using mutable shared state?
Mutable shared state can lead to issues such as race conditions and bugs in a multi-threaded environment. Try to minimize mutable shared state wherever possible for cleaner, easier maintenance.
Is using wrapper classes a good practice?
Using wrapper classes can be a good practice if you need to modify data within a function. It provides a clear structure and reduces confusion compared to using primitive types directly.
Conclusion
While Java does not support reference and output parameters natively as seen in other languages, understanding how to work with mutable objects allows you to mimic these behaviors. By implementing the outlined strategies, you can effectively manage data flow within your applications and accommodate scenarios that require similar functionality. Remember to opt for clear and maintainable patterns to ease future modifications and debugging.