How to Use Multer with React.js and Node.js for File Uploads
Handling file uploads is a common feature in modern web applications. Whether you're building a profile system, a document portal, or an image gallery, you'll likely need to upload files to a server. In this blog, you’ll learn how to use Multer (a popular Node.js middleware) to handle file uploads from a React.js frontend. Let’s build a simple file upload app with React.js on the frontend and Node.js/Express on the backend using Multer. Tools & Technologies Used React.js (Frontend) Node.js + Express (Backend) Multer (Middleware for file uploads) Axios (For HTTP requests) Render or Vercel (for deployment, optional) What is Multer? Multer is a middleware for handling multipart/form-data, which is used to upload files. It makes handling file uploads in Express simple and customizable. Project Structure file-upload-app/ ├── backend/ │ ├── uploads/ │ ├── index.js │ └── package.json └── frontend/ ├── src/ │ ├── App.js │ └── ... └── package.json Backend Setup (Node.js + Express + Multer) 1. Initialize Backend mkdir backend cd backend npm init -y npm install express multer cors 2. Create index.js const express = require('express'); const cors = require('cors'); const multer = require('multer'); const path = require('path'); const app = express(); app.use(cors()); app.use('/uploads', express.static('uploads')); // Storage setup const storage = multer.diskStorage({ destination: function (req, file, cb) { cb(null, 'uploads/') }, filename: function (req, file, cb) { cb(null, Date.now() + path.extname(file.originalname)); } }); const upload = multer({ storage: storage }); // Route to handle file upload app.post('/upload', upload.single('file'), (req, res) => { res.json({ filePath: `/uploads/${req.file.filename}` }); }); app.listen(5000, () => { console.log('Server is running on http://localhost:5000'); }); Frontend Setup (React.js + Axios) 1. Initialize Frontend npx create-react-app frontend cd frontend npm install axios 2. Update App.js import React, { useState } from 'react'; import axios from 'axios'; function App() { const [file, setFile] = useState(null); const [preview, setPreview] = useState(""); const handleFileChange = (e) => { setFile(e.target.files[0]); setPreview(URL.createObjectURL(e.target.files[0])); }; const handleUpload = async () => { const formData = new FormData(); formData.append("file", file); try { const res = await axios.post("http://localhost:5000/upload", formData, { headers: { "Content-Type": "multipart/form-data", }, }); alert("File uploaded successfully: " + res.data.filePath); } catch (err) { console.error(err); alert("Upload failed."); } }; return ( Upload a File {preview && } Upload ); } export default App; Run Your App 1. Start Backend cd backend node index.js 2. Start Frontend cd frontend npm start Final Thoughts You now have a working file upload system using React and Node.js with Multer. This simple setup can be extended to support: Multiple file uploads File validation (e.g., only images or PDFs) Cloud storage (e.g., AWS S3, Cloudinary) Authentication and user-specific folders
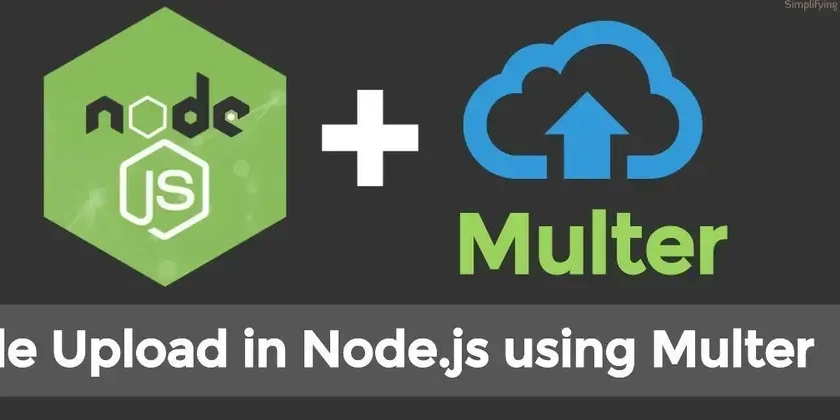
Handling file uploads is a common feature in modern web applications. Whether you're building a profile system, a document portal, or an image gallery, you'll likely need to upload files to a server. In this blog, you’ll learn how to use Multer (a popular Node.js middleware) to handle file uploads from a React.js frontend.
Let’s build a simple file upload app with React.js on the frontend and Node.js/Express on the backend using Multer.
Tools & Technologies Used
- React.js (Frontend)
- Node.js + Express (Backend)
- Multer (Middleware for file uploads)
- Axios (For HTTP requests)
- Render or Vercel (for deployment, optional)
What is Multer?
Multer is a middleware for handling multipart/form-data
, which is used to upload files. It makes handling file uploads in Express simple and customizable.
Project Structure
file-upload-app/
├── backend/
│ ├── uploads/
│ ├── index.js
│ └── package.json
└── frontend/
├── src/
│ ├── App.js
│ └── ...
└── package.json
Backend Setup (Node.js + Express + Multer)
1. Initialize Backend
mkdir backend
cd backend
npm init -y
npm install express multer cors
2. Create index.js
const express = require('express');
const cors = require('cors');
const multer = require('multer');
const path = require('path');
const app = express();
app.use(cors());
app.use('/uploads', express.static('uploads'));
// Storage setup
const storage = multer.diskStorage({
destination: function (req, file, cb) {
cb(null, 'uploads/')
},
filename: function (req, file, cb) {
cb(null, Date.now() + path.extname(file.originalname));
}
});
const upload = multer({ storage: storage });
// Route to handle file upload
app.post('/upload', upload.single('file'), (req, res) => {
res.json({ filePath: `/uploads/${req.file.filename}` });
});
app.listen(5000, () => {
console.log('Server is running on http://localhost:5000');
});
Frontend Setup (React.js + Axios)
1. Initialize Frontend
npx create-react-app frontend
cd frontend
npm install axios
2. Update App.js
import React, { useState } from 'react';
import axios from 'axios';
function App() {
const [file, setFile] = useState(null);
const [preview, setPreview] = useState("");
const handleFileChange = (e) => {
setFile(e.target.files[0]);
setPreview(URL.createObjectURL(e.target.files[0]));
};
const handleUpload = async () => {
const formData = new FormData();
formData.append("file", file);
try {
const res = await axios.post("http://localhost:5000/upload", formData, {
headers: {
"Content-Type": "multipart/form-data",
},
});
alert("File uploaded successfully: " + res.data.filePath);
} catch (err) {
console.error(err);
alert("Upload failed.");
}
};
return (
<div style={{ padding: 20 }}>
<h2>Upload a Fileh2>
<input type="file" onChange={handleFileChange} />
{preview && <img src={preview} alt="preview" width="200" />}
<br />
<button onClick={handleUpload}>Uploadbutton>
div>
);
}
export default App;
Run Your App
1. Start Backend
cd backend
node index.js
2. Start Frontend
cd frontend
npm start
Final Thoughts
You now have a working file upload system using React and Node.js with Multer. This simple setup can be extended to support:
- Multiple file uploads
- File validation (e.g., only images or PDFs)
- Cloud storage (e.g., AWS S3, Cloudinary)
- Authentication and user-specific folders