How to Pass Parameters to TypeScript Functions in ASP.NET MVC?
Introduction Passing parameters from ASP.NET MVC page models to TypeScript functions can be tricky, especially when incorporating dynamic values such as token, url, and redirectUrl. In this guide, we will explore how to accomplish this effectively, ensuring that we maintain optimal coding practices. Understanding the Basics When working with TypeScript and ASP.NET MVC, it is crucial to understand how data flows between the server-side (C# code) and client-side (TypeScript/VBScript/JavaScript code). The Link.ts you provided defines an interface LinkConfig and an asynchronous function postData. This function needs to be called with correctly structured parameters that are passed from your Razor pages. Step 1: Setting Up the Razor Page Model In your Razor pages, extract the token, URL, and redirect URL that you intend to pass to the TypeScript function. Here's an example of how you might do this: // Example ASP.NET MVC page model using Microsoft.AspNetCore.Mvc.RazorPages; public class MyPageModel : PageModel { public string Token { get; set; } = "your_token_here"; public string Url { get; set; } = "https://example.com/api/link"; public string RedirectUrl { get; set; } = "https://example.com/success"; public void OnGet() { // Optionally set token, url, and redirectUrl dynamically } } This example defines properties for Token, Url, and RedirectUrl. You can set these values dynamically based on your application's logic. Step 2: Passing Data to JavaScript To pass these values from the Razor model to your TypeScript file, you need to insert them into your page's HTML. Here’s how to do it: @page @model MyPageModel My ASP.NET Page const linkConfig = { token: "@Model.Token", url: "@Model.Url", redirectUrl: "@Model.RedirectUrl" }; // Call the postData function from Link.ts postData(linkConfig); Explanation of the Code @Model Properties: Here, @Model.Token, @Model.Url, and @Model.RedirectUrl will render their respective values directly into the JavaScript code when the page is served. Creating an Object: The linkConfig object holds the values that match your LinkConfig interface in TypeScript. This will be passed to the postData function. Step 3: Function Execution Once the page loads, the JavaScript block will execute, creating a linkConfig object and immediately invoking the postData function: Asynchronous Call: This will call your TypeScript function which handles the API request as defined in your Link.ts file. Example of callRestEndpoint If you haven't defined the callRestEndpoint function yet, make sure to add it for making network requests. Here’s a simple example: async function callRestEndpoint(url: string, method: string, data: any): Promise { const response = await fetch(url, { method: method, headers: { 'Content-Type': 'application/json' }, body: JSON.stringify(data) }); return await response.json(); } Frequently Asked Questions How do I handle errors when calling postData? You can view errors in the console or display them on the webpage, as shown in your postData function. It already handles the error case and appends a message to the result element. Is it safe to expose tokens in JavaScript? Avoid sending sensitive tokens or keys to the client-side unless absolutely necessary. If the token is sensitive, consider using server-side logic to handle it securely. Conclusion By following these steps, you can successfully pass parameters from your ASP.NET MVC Razor page model into your TypeScript code, making your web application more interactive and dynamic. This setup seamlessly integrates server and client-side programming, enhancing user experiences significantly.
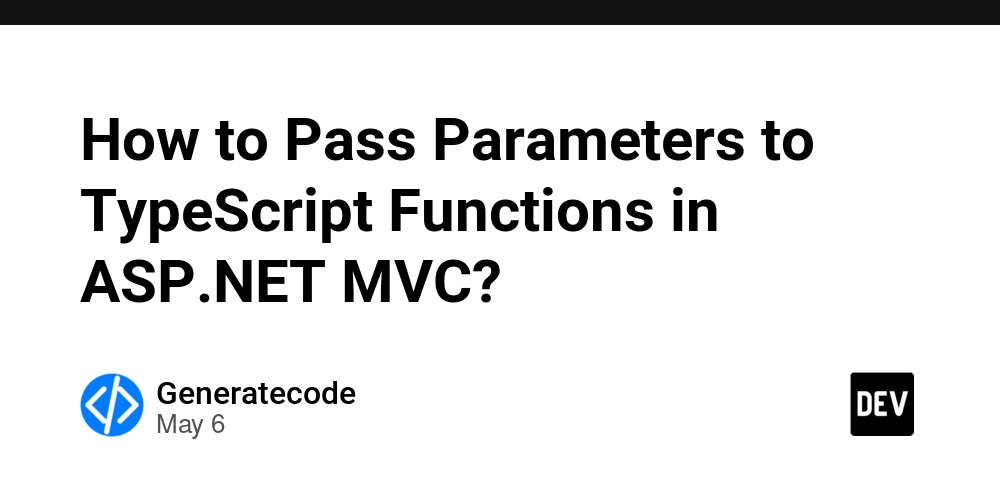
Introduction
Passing parameters from ASP.NET MVC page models to TypeScript functions can be tricky, especially when incorporating dynamic values such as token
, url
, and redirectUrl
. In this guide, we will explore how to accomplish this effectively, ensuring that we maintain optimal coding practices.
Understanding the Basics
When working with TypeScript and ASP.NET MVC, it is crucial to understand how data flows between the server-side (C# code) and client-side (TypeScript/VBScript/JavaScript code). The Link.ts
you provided defines an interface LinkConfig
and an asynchronous function postData
. This function needs to be called with correctly structured parameters that are passed from your Razor pages.
Step 1: Setting Up the Razor Page Model
In your Razor pages, extract the token, URL, and redirect URL that you intend to pass to the TypeScript function. Here's an example of how you might do this:
// Example ASP.NET MVC page model
using Microsoft.AspNetCore.Mvc.RazorPages;
public class MyPageModel : PageModel
{
public string Token { get; set; } = "your_token_here";
public string Url { get; set; } = "https://example.com/api/link";
public string RedirectUrl { get; set; } = "https://example.com/success";
public void OnGet()
{
// Optionally set token, url, and redirectUrl dynamically
}
}
This example defines properties for Token
, Url
, and RedirectUrl
. You can set these values dynamically based on your application's logic.
Step 2: Passing Data to JavaScript
To pass these values from the Razor model to your TypeScript file, you need to insert them into your page's HTML. Here’s how to do it:
@page
@model MyPageModel
My ASP.NET Page
Explanation of the Code
-
@Model Properties: Here,
@Model.Token
,@Model.Url
, and@Model.RedirectUrl
will render their respective values directly into the JavaScript code when the page is served. -
Creating an Object: The
linkConfig
object holds the values that match yourLinkConfig
interface in TypeScript. This will be passed to thepostData
function.
Step 3: Function Execution
Once the page loads, the JavaScript block will execute, creating a linkConfig
object and immediately invoking the postData
function:
-
Asynchronous Call: This will call your TypeScript function which handles the API request as defined in your
Link.ts
file.
Example of callRestEndpoint
If you haven't defined the callRestEndpoint
function yet, make sure to add it for making network requests. Here’s a simple example:
async function callRestEndpoint(url: string, method: string, data: any): Promise {
const response = await fetch(url, {
method: method,
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(data)
});
return await response.json();
}
Frequently Asked Questions
How do I handle errors when calling postData?
You can view errors in the console or display them on the webpage, as shown in your postData
function. It already handles the error case and appends a message to the result element.
Is it safe to expose tokens in JavaScript?
Avoid sending sensitive tokens or keys to the client-side unless absolutely necessary. If the token is sensitive, consider using server-side logic to handle it securely.
Conclusion
By following these steps, you can successfully pass parameters from your ASP.NET MVC Razor page model into your TypeScript code, making your web application more interactive and dynamic. This setup seamlessly integrates server and client-side programming, enhancing user experiences significantly.