How to use HarmonyOS NEXT - @Provide and @Consume?
@Provide and @Consume are used for two-way data synchronization with future generations of components, and for scenarios where state data is transmitted between multiple levels. Different from the above-mentioned transfer between father and son components through named parameter mechanism, @Provide and @Consume get rid of the constraints of parameter transfer mechanism and realize cross-level transfer. Among them, the variable decorated by @Provide is in the ancestor component, which can be understood as a state variable that is "provided" to future generations. The variable decorated by @Consume is the variable provided by the ancestor component in the descendant component. @Provide/@Consume is a two-way synchronization across component levels. Before reading the @Provide and @Consume documents, it is recommended that developers have a basic understanding of the basic syntax and custom components of the UI paradigm. It is recommended to read in advance: basic syntax overview, declarative UI description, custom components-creating custom components. The status variable decorated by @Provide/@Consume has the following characteristics: The state variable decorated by-@Provide is automatically available to all its descendant components, that is, the variable is "provided" to his descendant components. Thus, the convenience of @Provide is that developers don't need to pass variables between components many times. Descendants use @Consume to obtain variables provided by @Provide, and establish two-way data synchronization between @Provide and @Consume. Unlike @State/@link, the former can be passed between multi-level parent-child components. @Provide and @Consume can be bound by the same variable name or the same variable alias, suggesting the same type, otherwise implicit type conversion will occur, resulting in abnormal application behavior. @Provide/@Consume must specify the type, and the variable types that allow decoration are as follows: Object, class, string, number, boolean, enum types, and arrays of these types. Date type is supported. API11 and above support Map and Set types. Support the union types defined by the ArkUI framework, such as Length, ResourceStr and ResourceColor. The type of @Consume variable of-@Provide variable must be the same. any is not supported. API11 and above support the union types of the above supported types, such as string | number, string | undefined or ClassA | null. For an example, see @Provide_and_Consume for an example of supporting union types. Code example // Bound by the same variable name @Provide a: number = 0; @Consume a: number; // Bound by the same variable alias @Provide('a') b: number = 0; @Consume('a') c: number; Code example ProvideConsumePage import { SonComponent } from './components/SonComponent'; @Entry @Component struct ProvideConsumePage { @State message: string = '@Provide and @Consume'; @Provide('count') stateCount: number = 0 build() { Column({space:10}) { Text(this.message) .fontSize(20) .fontWeight(FontWeight.Bold) Button('增加次数').onClick(()=>{ this.stateCount++ }) Text('stateCount='+this.stateCount) SonComponent() } .height('100%') .width('100%') } } SonComponent import { GrandsonComponent } from './GrandsonComponent' @Component export struct SonComponent { build() { Column({ space: 10 }) { Text('这是子组件') GrandsonComponent() } .width('100%') .padding(10) .backgroundColor(Color.Orange) } } GrandsonComponent @Component export struct GrandsonComponent { @Consume('count') grandsonCount: number build() { Column({space:10}){ Text('孙组件') Button('增加次数').onClick(()=>{ this.grandsonCount++ }) Text('grandsonCount='+this.grandsonCount) } .width('100%') .padding(10) .backgroundColor('#EEEEEE') } }
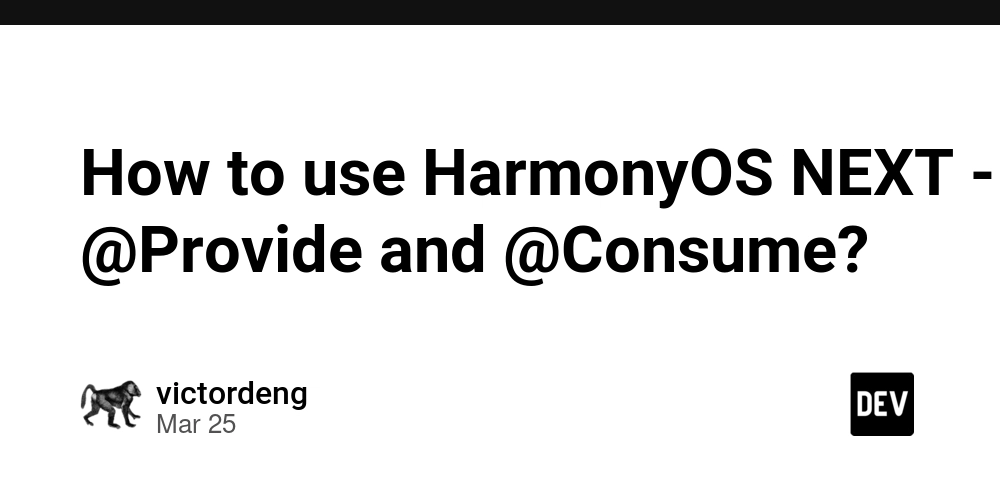
@Provide and @Consume are used for two-way data synchronization with future generations of components, and for scenarios where state data is transmitted between multiple levels. Different from the above-mentioned transfer between father and son components through named parameter mechanism, @Provide and @Consume get rid of the constraints of parameter transfer mechanism and realize cross-level transfer.
Among them, the variable decorated by @Provide is in the ancestor component, which can be understood as a state variable that is "provided" to future generations. The variable decorated by @Consume is the variable provided by the ancestor component in the descendant component.
@Provide/@Consume is a two-way synchronization across component levels. Before reading the @Provide and @Consume documents, it is recommended that developers have a basic understanding of the basic syntax and custom components of the UI paradigm. It is recommended to read in advance: basic syntax overview, declarative UI description, custom components-creating custom components.
The status variable decorated by @Provide/@Consume has the following characteristics:
- The state variable decorated by-@Provide is automatically available to all its descendant components, that is, the variable is "provided" to his descendant components. Thus, the convenience of @Provide is that developers don't need to pass variables between components many times.
- Descendants use @Consume to obtain variables provided by @Provide, and establish two-way data synchronization between @Provide and @Consume. Unlike @State/@link, the former can be passed between multi-level parent-child components.
- @Provide and @Consume can be bound by the same variable name or the same variable alias, suggesting the same type, otherwise implicit type conversion will occur, resulting in abnormal application behavior.
@Provide/@Consume must specify the type, and the variable types that allow decoration are as follows:
- Object, class, string, number, boolean, enum types, and arrays of these types.
- Date type is supported.
- API11 and above support Map and Set types.
- Support the union types defined by the ArkUI framework, such as Length, ResourceStr and ResourceColor.
- The type of @Consume variable of-@Provide variable must be the same.
- any is not supported.
- API11 and above support the union types of the above supported types, such as string | number, string | undefined or ClassA | null. For an example, see @Provide_and_Consume for an example of supporting union types.
Code example
// Bound by the same variable name
@Provide a: number = 0;
@Consume a: number;
// Bound by the same variable alias
@Provide('a') b: number = 0;
@Consume('a') c: number;
Code example
ProvideConsumePage
import { SonComponent } from './components/SonComponent';
@Entry
@Component
struct ProvideConsumePage {
@State message: string = '@Provide and @Consume';
@Provide('count') stateCount: number = 0
build() {
Column({space:10}) {
Text(this.message)
.fontSize(20)
.fontWeight(FontWeight.Bold)
Button('增加次数').onClick(()=>{
this.stateCount++
})
Text('stateCount='+this.stateCount)
SonComponent()
}
.height('100%')
.width('100%')
}
}
SonComponent
import { GrandsonComponent } from './GrandsonComponent'
@Component
export struct SonComponent {
build() {
Column({ space: 10 }) {
Text('这是子组件')
GrandsonComponent()
}
.width('100%')
.padding(10)
.backgroundColor(Color.Orange)
}
}
GrandsonComponent
@Component
export struct GrandsonComponent {
@Consume('count') grandsonCount: number
build() {
Column({space:10}){
Text('孙组件')
Button('增加次数').onClick(()=>{
this.grandsonCount++
})
Text('grandsonCount='+this.grandsonCount)
}
.width('100%')
.padding(10)
.backgroundColor('#EEEEEE')
}
}