How to Safely Delete All AWS S3 Buckets Using a Bash Script
Have you ever faced the situation where you realized you have too many S3 buckets in your account and you want to delete all? But AWS will not allow you to delete all at once in the console, especially if there is still content in them. The following Bash script automates and speeds up this process while ensuring that both object versions and delete markers are properly removed before bucket deletion. In this blog post, I will break down how this script works and the precautions it takes. The Purpose of the Script This script performs the following steps: 1. Lists all S3 buckets in your AWS account. 2. Asks for user confirmation before proceeding with deletion. 3. Iterates over each bucket and deletes all object versions and delete markers. Deletes the empty bucket from S3. Let's go through the script step by step. Script Breakdown The script starts with the shebang (#!/bin/bash), which ensures it runs in a Bash shell. #!/bin/bash Step 1: Listing All S3 Buckets This command lists all S3 buckets using aws s3 ls and extracts only the bucket names using awk '{print $3}'. buckets=$(aws s3 ls | awk '{print $3}') if [ -z "$buckets" ]; then echo "No S3 buckets found." exit 0 fi If no buckets exist, the script exits gracefully. Step 2: User Confirmation The script prompts the user for confirmation to prevent accidental deletions. If the user does not type yes, the operation is aborted. echo "WARNING: This will permanently delete all S3 buckets and their contents." echo "Do you want to proceed? (yes/no)" read confirmation if [ "$confirmation" != "yes" ]; then echo "Operation canceled." exit 1 fi Step 3: Deleting Object Versions Each bucket is processed in a loop: for bucket in $buckets; do echo "Deleting all object versions from bucket: $bucket" Object versions are retrieved using: versions=$(aws s3api list-object-versions --bucket "$bucket" --query 'Versions[*].[Key, VersionId]' --output text 2>/dev/null) If object versions exist, they are deleted in a loop: echo "$versions" | while read -r key versionId; do if [ -n "$key" ] && [ -n "$versionId" ]; then echo "Deleting: $key (version: $versionId)" aws s3api delete-object --bucket "$bucket" --key "$key" --version-id "$versionId" fi done Step 4: Deleting Delete Markers Similarly, delete markers (for versioned buckets) are retrieved and removed: markers=$(aws s3api list-object-versions --bucket "$bucket" --query 'DeleteMarkers[*].[Key, VersionId]' --output text 2>/dev/null) echo "$markers" | while read -r key versionId; do if [ -n "$key" ] && [ -n "$versionId" ]; then echo "Deleting marker: $key (version: $versionId)" aws s3api delete-object --bucket "$bucket" --key "$key" --version-id "$versionId" fi done Step 5: Deleting the Bucket After clearing all contents, the bucket itself is removed: echo "Deleting bucket: $bucket" aws s3 rb s3://$bucket --force This command forcefully removes the bucket from S3. Final Step: Script Completion Once all buckets are deleted, a success message is displayed. done echo "All S3 buckets deleted successfully." Conclusion This script provides a safe and efficient way to delete all S3 buckets while ensuring that all object versions and delete markers are removed. The user confirmation step helps prevent accidental deletions, making it a useful tool for cloud administrators. Additionally, the script can be further customized if you just want to delete specific buckets. If you plan to use this script, ensure you have the appropriate AWS permissions and double-check before running it to avoid unintended data loss! The script repository can be found here.
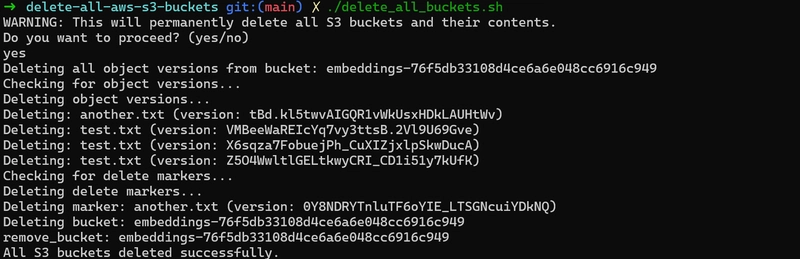
Have you ever faced the situation where you realized you have too many S3 buckets in your account and you want to delete all? But AWS will not allow you to delete all at once in the console, especially if there is still content in them.
The following Bash script automates and speeds up this process while ensuring that both object versions and delete markers are properly removed before bucket deletion. In this blog post, I will break down how this script works and the precautions it takes.
The Purpose of the Script
This script performs the following steps:
- 1. Lists all S3 buckets in your AWS account.
- 2. Asks for user confirmation before proceeding with deletion.
- 3. Iterates over each bucket and deletes all object versions and delete markers.
- Deletes the empty bucket from S3.
Let's go through the script step by step.
Script Breakdown
The script starts with the shebang (#!/bin/bash
), which ensures it runs in a Bash shell.
#!/bin/bash
Step 1: Listing All S3 Buckets
This command lists all S3 buckets using aws s3 ls
and extracts only the bucket names using awk '{print $3}'
.
buckets=$(aws s3 ls | awk '{print $3}')
if [ -z "$buckets" ]; then
echo "No S3 buckets found."
exit 0
fi
If no buckets exist, the script exits gracefully.
Step 2: User Confirmation
The script prompts the user for confirmation to prevent accidental deletions. If the user does not type yes
, the operation is aborted.
echo "WARNING: This will permanently delete all S3 buckets and their contents."
echo "Do you want to proceed? (yes/no)"
read confirmation
if [ "$confirmation" != "yes" ]; then
echo "Operation canceled."
exit 1
fi
Step 3: Deleting Object Versions
Each bucket is processed in a loop:
for bucket in $buckets; do
echo "Deleting all object versions from bucket: $bucket"
Object versions are retrieved using:
versions=$(aws s3api list-object-versions --bucket "$bucket" --query 'Versions[*].[Key, VersionId]' --output text 2>/dev/null)
If object versions exist, they are deleted in a loop:
echo "$versions" | while read -r key versionId; do
if [ -n "$key" ] && [ -n "$versionId" ]; then
echo "Deleting: $key (version: $versionId)"
aws s3api delete-object --bucket "$bucket" --key "$key" --version-id "$versionId"
fi
done
Step 4: Deleting Delete Markers
Similarly, delete markers (for versioned buckets) are retrieved and removed:
markers=$(aws s3api list-object-versions --bucket "$bucket" --query 'DeleteMarkers[*].[Key, VersionId]' --output text 2>/dev/null)
echo "$markers" | while read -r key versionId; do
if [ -n "$key" ] && [ -n "$versionId" ]; then
echo "Deleting marker: $key (version: $versionId)"
aws s3api delete-object --bucket "$bucket" --key "$key" --version-id "$versionId"
fi
done
Step 5: Deleting the Bucket
After clearing all contents, the bucket itself is removed:
echo "Deleting bucket: $bucket"
aws s3 rb s3://$bucket --force
This command forcefully removes the bucket from S3.
Final Step: Script Completion
Once all buckets are deleted, a success message is displayed.
done
echo "All S3 buckets deleted successfully."
Conclusion
This script provides a safe and efficient way to delete all S3 buckets while ensuring that all object versions and delete markers are removed. The user confirmation step helps prevent accidental deletions, making it a useful tool for cloud administrators. Additionally, the script can be further customized if you just want to delete specific buckets.
If you plan to use this script, ensure you have the appropriate AWS permissions and double-check before running it to avoid unintended data loss!
The script repository can be found here.