How to Implement Push Notifications Using JavaScript
1. Selecting the Button Element const notifyBtn = document.querySelector("#notify"); Selects the button with the id="notify" from the DOM. Stores it in the variable notifyBtn. 2. Notification and Interval Variables let interval; let notification; interval: Stores the ID of the interval function (used for clearing the interval later). notification: Stores the latest notification instance. 3. Click Event Listener on Button notifyBtn.addEventListener("click", () => { Notification.requestPermission().then((permission) => { if (permission == "granted") { notification = new Notification("Example", { body: "This is more text for example" + Math.random(), icon: "icon.png", tag: "Example", }); notification.addEventListener("error", (e) => { console.error(); }); } }); }); Explanation: When the button is clicked: Notification.requestPermission() asks for permission to display notifications. If the user grants permission (granted), a new notification is created: Title: "Example" Body: "This is more text for example" + random number. Icon: "icon.png" Tag: "Example" (helps to prevent duplicate notifications) Listens for error events on the notification and logs them. 4. Handling Page Visibility Changes document.addEventListener("visibilitychange", () => { if (document.visibilityState === "hidden") { const leaveDate = new Date(); interval = setInterval(() => { notification = new Notification("Come back", { body: `You have been gone for ${Math.round( (new Date() - leaveDate) / 1000 )} secs, come back!`, tag: "Come back", }); notification.addEventListener("error", (e) => { console.error(e); }); }, 100); } else { if (interval) { clearInterval(interval); } if (notification) { notification.close(); } } }); Explanation: visibilitychange event fires when the user switches tabs or minimizes the browser. If the page is hidden: Stores the time when the user left (leaveDate). Starts an interval that creates a new notification every 100ms. Notification says how long the user has been away in seconds. If the page becomes visible again: Stops the interval using clearInterval(interval). Closes any active notification. Summary of APIs Used API Description document.querySelector() Selects an element from the DOM. Notification.requestPermission() Requests permission for displaying notifications. new Notification(title, options) Creates a new browser notification. document.addEventListener("visibilitychange", callback) Fires when the page visibility changes. setInterval(callback, time) Repeats a function every time milliseconds. clearInterval(intervalID) Stops a running interval. notification.close() Closes the notification. Possible Improvements Prevent Spamming: The interval runs every 100ms, which is too frequent. Increase the delay to something like 5000 (5 seconds). Check for Notification Support: if (!("Notification" in window)) { alert("This browser does not support notifications."); } Improve Error Logging: notification.addEventListener("error", (e) => { console.error("Notification error:", e); });
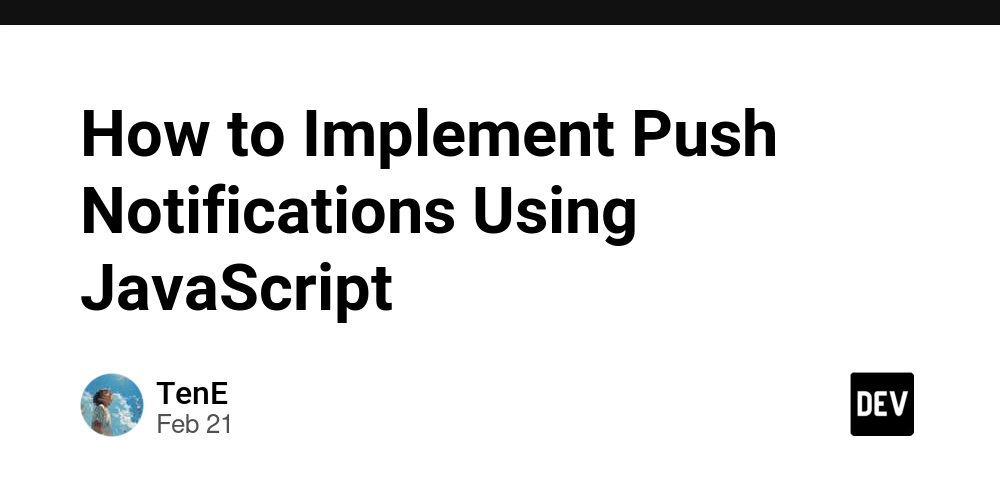
1. Selecting the Button Element
const notifyBtn = document.querySelector("#notify");
- Selects the button with the
id="notify"
from the DOM. - Stores it in the variable
notifyBtn
.
2. Notification and Interval Variables
let interval;
let notification;
-
interval
: Stores the ID of the interval function (used for clearing the interval later). -
notification
: Stores the latest notification instance.
3. Click Event Listener on Button
notifyBtn.addEventListener("click", () => {
Notification.requestPermission().then((permission) => {
if (permission == "granted") {
notification = new Notification("Example", {
body: "This is more text for example" + Math.random(),
icon: "icon.png",
tag: "Example",
});
notification.addEventListener("error", (e) => {
console.error();
});
}
});
});
Explanation:
- When the button is clicked:
-
Notification.requestPermission()
asks for permission to display notifications. - If the user grants permission (
granted
), a new notification is created: - Title:
"Example"
- Body:
"This is more text for example"
+ random number. - Icon:
"icon.png"
- Tag:
"Example"
(helps to prevent duplicate notifications)
-
- Listens for
error
events on the notification and logs them.
4. Handling Page Visibility Changes
document.addEventListener("visibilitychange", () => {
if (document.visibilityState === "hidden") {
const leaveDate = new Date();
interval = setInterval(() => {
notification = new Notification("Come back", {
body: `You have been gone for ${Math.round(
(new Date() - leaveDate) / 1000
)} secs, come back!`,
tag: "Come back",
});
notification.addEventListener("error", (e) => {
console.error(e);
});
}, 100);
} else {
if (interval) {
clearInterval(interval);
}
if (notification) {
notification.close();
}
}
});
Explanation:
-
visibilitychange
event fires when the user switches tabs or minimizes the browser. - If the page is hidden:
- Stores the time when the user left (
leaveDate
). - Starts an interval that creates a new notification every 100ms.
- Notification says how long the user has been away in seconds.
- Stores the time when the user left (
- If the page becomes visible again:
- Stops the interval using
clearInterval(interval)
. - Closes any active notification.
- Stops the interval using
Summary of APIs Used
API | Description |
---|---|
document.querySelector() |
Selects an element from the DOM. |
Notification.requestPermission() |
Requests permission for displaying notifications. |
new Notification(title, options) |
Creates a new browser notification. |
document.addEventListener("visibilitychange", callback) |
Fires when the page visibility changes. |
setInterval(callback, time) |
Repeats a function every time milliseconds. |
clearInterval(intervalID) |
Stops a running interval. |
notification.close() |
Closes the notification. |
Possible Improvements
-
Prevent Spamming: The interval runs every 100ms, which is too frequent. Increase the delay to something like
5000
(5 seconds). - Check for Notification Support:
if (!("Notification" in window)) {
alert("This browser does not support notifications.");
}
- Improve Error Logging:
notification.addEventListener("error", (e) => {
console.error("Notification error:", e);
});