How to Escape Callback Hell in JavaScript: A Developer’s Guide
Callback Hell, also known as the "Pyramid of Doom," is a common pain point in JavaScript when dealing with asynchronous operations. Nested callbacks lead to code that’s hard to read, debug, and maintain. In this guide, we’ll explore practical strategies to flatten your code and write cleaner, more maintainable JavaScript. What is Callback Hell? Callback Hell occurs when multiple asynchronous operations depend on each other, forcing you to nest callbacks. The result is deeply indented, pyramid-shaped code: getUser(userId, (user) => { getPosts(user.id, (posts) => { getComments(posts[0].id, (comments) => { renderDashboard(user, posts, comments, () => { // More nesting... }); }); }); }); This structure becomes unmanageable as complexity grows. Let’s fix it! Strategy 1: Use Promises to Flatten Code Promises provide a .then() chain to handle asynchronous tasks sequentially without nesting. Example: Refactoring Callbacks to Promises Before (Callback Hell): function fetchData(callback) { getUser(userId, (user) => { getPosts(user.id, (posts) => { getComments(posts[0].id, (comments) => { callback({ user, posts, comments }); }); }); }); } After (With Promises): function fetchData() { return getUser(userId) .then(user => getPosts(user.id)) .then(posts => getComments(posts[0].id)) .then(comments => ({ user, posts, comments })); } // Usage: fetchData() .then(data => renderDashboard(data)) .catch(error => console.error("Failed!", error)); Key Benefits: Chaining: Each .then() returns a new Promise. Error Handling: A single .catch() handles all errors. Strategy 2: Async/Await for Synchronous-Like Code async/await (ES7) simplifies Promise chains further, making asynchronous code look synchronous. Example: Refactoring to Async/Await async function fetchData() { try { const user = await getUser(userId); const posts = await getPosts(user.id); const comments = await getComments(posts[0].id); return { user, posts, comments }; } catch (error) { console.error("Failed!", error); } } // Usage: const data = await fetchData(); renderDashboard(data); Key Benefits: Readability: No more .then() chains. Error Handling: Use try/catch for synchronous-style error handling. Strategy 3: Modularize Your Code Break nested callbacks into smaller, reusable functions. Example: Modularization // 1. Split into focused functions const getUserData = async (userId) => await getUser(userId); const fetchUserPosts = async (user) => await getPosts(user.id); const fetchPostComments = async (posts) => await getComments(posts[0].id); // 2. Combine them async function buildDashboard() { const user = await getUserData(userId); const posts = await fetchUserPosts(user); const comments = await fetchPostComments(posts); renderDashboard({ user, posts, comments }); } Key Benefits: Reusability: Functions can be tested and reused. Clarity: Each function has a single responsibility. Strategy 4: Use Promise Utilities Handle complex flows with Promise.all(), Promise.race(), or libraries like async.js. Example: Parallel Execution with Promise.all() async function fetchAllData() { const [user, posts, comments] = await Promise.all([ getUser(userId), getPosts(userId), getComments(postId) ]); return { user, posts, comments }; } When to Use: Parallel Tasks: Fetch independent data simultaneously. Optimization: Reduce total execution time. Common Pitfalls & Best Practices Avoid Anonymous Functions: Name your functions for better stack traces. Always Handle Errors: Never omit .catch() or try/catch. Leverage Linters: Tools like ESLint detect nested callbacks. Use Modern Libraries: Replace callback-based APIs with Promise-based alternatives (e.g., fs.promises in Node.js). Conclusion: Choose the Right Tool Scenario Solution Simple async chains Promises or Async/Await Complex parallel tasks Promise.all() Legacy callback-based code Modularization By embracing Promises, async/await, and modular code, you’ll turn "Pyramids of Doom" into flat, readable workflows. Feel Free To Ask Questions, Happy Coding
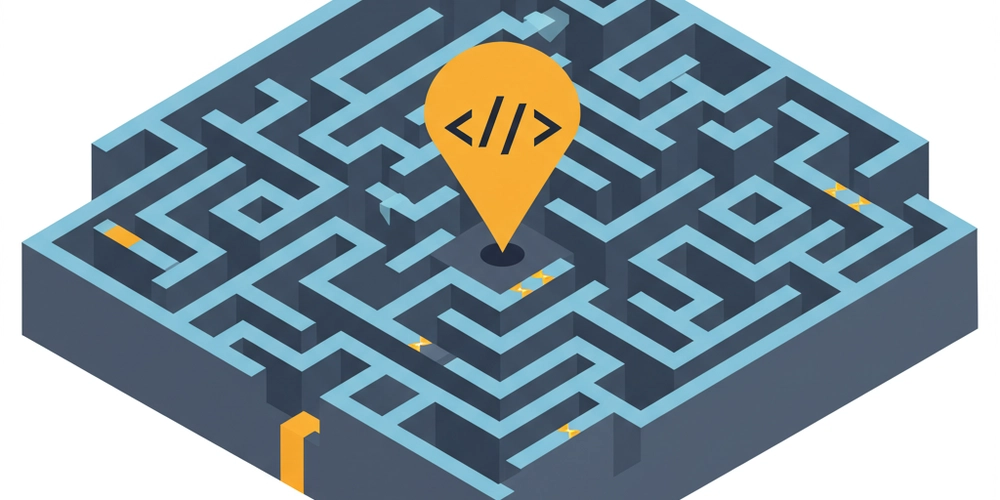
Callback Hell, also known as the "Pyramid of Doom," is a common pain point in JavaScript when dealing with asynchronous operations. Nested callbacks lead to code that’s hard to read, debug, and maintain. In this guide, we’ll explore practical strategies to flatten your code and write cleaner, more maintainable JavaScript.
What is Callback Hell?
Callback Hell occurs when multiple asynchronous operations depend on each other, forcing you to nest callbacks. The result is deeply indented, pyramid-shaped code:
getUser(userId, (user) => {
getPosts(user.id, (posts) => {
getComments(posts[0].id, (comments) => {
renderDashboard(user, posts, comments, () => {
// More nesting...
});
});
});
});
This structure becomes unmanageable as complexity grows. Let’s fix it!
Strategy 1: Use Promises to Flatten Code
Promises provide a .then()
chain to handle asynchronous tasks sequentially without nesting.
Example: Refactoring Callbacks to Promises
Before (Callback Hell):
function fetchData(callback) {
getUser(userId, (user) => {
getPosts(user.id, (posts) => {
getComments(posts[0].id, (comments) => {
callback({ user, posts, comments });
});
});
});
}
After (With Promises):
function fetchData() {
return getUser(userId)
.then(user => getPosts(user.id))
.then(posts => getComments(posts[0].id))
.then(comments => ({ user, posts, comments }));
}
// Usage:
fetchData()
.then(data => renderDashboard(data))
.catch(error => console.error("Failed!", error));
Key Benefits:
-
Chaining: Each
.then()
returns a new Promise. -
Error Handling: A single
.catch()
handles all errors.
Strategy 2: Async/Await for Synchronous-Like Code
async/await
(ES7) simplifies Promise chains further, making asynchronous code look synchronous.
Example: Refactoring to Async/Await
async function fetchData() {
try {
const user = await getUser(userId);
const posts = await getPosts(user.id);
const comments = await getComments(posts[0].id);
return { user, posts, comments };
} catch (error) {
console.error("Failed!", error);
}
}
// Usage:
const data = await fetchData();
renderDashboard(data);
Key Benefits:
-
Readability: No more
.then()
chains. -
Error Handling: Use
try/catch
for synchronous-style error handling.
Strategy 3: Modularize Your Code
Break nested callbacks into smaller, reusable functions.
Example: Modularization
// 1. Split into focused functions
const getUserData = async (userId) => await getUser(userId);
const fetchUserPosts = async (user) => await getPosts(user.id);
const fetchPostComments = async (posts) => await getComments(posts[0].id);
// 2. Combine them
async function buildDashboard() {
const user = await getUserData(userId);
const posts = await fetchUserPosts(user);
const comments = await fetchPostComments(posts);
renderDashboard({ user, posts, comments });
}
Key Benefits:
- Reusability: Functions can be tested and reused.
- Clarity: Each function has a single responsibility.
Strategy 4: Use Promise Utilities
Handle complex flows with Promise.all()
, Promise.race()
, or libraries like async.js
.
Example: Parallel Execution with Promise.all()
async function fetchAllData() {
const [user, posts, comments] = await Promise.all([
getUser(userId),
getPosts(userId),
getComments(postId)
]);
return { user, posts, comments };
}
When to Use:
- Parallel Tasks: Fetch independent data simultaneously.
- Optimization: Reduce total execution time.
Common Pitfalls & Best Practices
- Avoid Anonymous Functions: Name your functions for better stack traces.
-
Always Handle Errors: Never omit
.catch()
ortry/catch
. - Leverage Linters: Tools like ESLint detect nested callbacks.
-
Use Modern Libraries: Replace callback-based APIs with Promise-based alternatives (e.g.,
fs.promises
in Node.js).
Conclusion: Choose the Right Tool
Scenario | Solution |
---|---|
Simple async chains | Promises or Async/Await |
Complex parallel tasks | Promise.all() |
Legacy callback-based code | Modularization |
By embracing Promises, async/await
, and modular code, you’ll turn "Pyramids of Doom" into flat, readable workflows.
Feel Free To Ask Questions, Happy Coding