How to Become a Senior C# Developer: Action Steps
Introduction: Becoming a senior C# developer takes more than just time on the job. It requires growing your technical skills, developing professional qualities, and taking on bigger responsibilities. This guide offers practical steps to help you advance from a regular C# developer to a senior position. Master C# Technical Skills: Deepen your C# knowledge: Study advanced C# features like LINQ, async/await, and delegates. Practice using these in your daily work, not just reading about them. For example, try refactoring an existing synchronous method to use async/await patterns in your current project. Understand .NET ecosystem thoroughly: Get comfortable with the latest .NET versions and their features. Build small practice projects using new capabilities. If you're still working with .NET Framework, create a side project using .NET 6/7/8 to experience the differences firsthand. Learn design patterns: Start applying common patterns like Repository, Factory, and Dependency Injection in your code. When you build a new feature, think about which pattern would make your code more maintainable and explain your choice to colleagues. Master testing: Write unit tests for all new code you produce. Set a goal to achieve good test coverage in your projects. Learn to use testing frameworks like NUnit or xUnit and practice test-driven development on a small feature. Example: // Instead of this: public List GetActiveCustomers() { var customers = _database.GetAllCustomers(); return customers.Where(c => c.IsActive).ToList(); } // Write testable code like this: public List GetActiveCustomers(ICustomerRepository repository) { return repository.GetAll().Where(c => c.IsActive).ToList(); } Build System Architecture Knowledge: Learn database design: Practice creating efficient database schemas and writing optimized queries. Try to optimize an existing slow query in your project, measuring the performance before and after. Study system architecture: Understand how different parts of applications work together. Draw diagrams of systems you work on to visualize data flow and component interactions. Explore microservices: Build a small project using microservices architecture. Break down a monolithic application into smaller services that communicate with each other. Practice with cloud services: Set up a small application on Azure or AWS. Learn how to deploy, monitor, and scale applications in the cloud. Create a CI/CD pipeline for automatic deployment. Develop Professional Skills: Improve code reviews: Give helpful, specific feedback during reviews. Instead of saying "this could be better," suggest how: "Consider using a factory pattern here to make this more maintainable." Mentor others: Offer to help junior developers with their tasks. Schedule regular sessions to answer their questions and review their progress on projects. Communicate clearly: Practice explaining technical concepts in simple terms. Try explaining your current project to someone outside your team and refine your explanation based on their questions. Take ownership: Volunteer to lead a small project or feature. Take responsibility for its success, from planning to deployment and maintenance. Solving Complex Problems: Break down big issues: When facing a complex problem, divide it into smaller, manageable parts. Write out the steps needed to solve each part. Research efficiently: Develop a strategy for finding answers. Know which resources are reliable and relevant for C# development. Create a personal knowledge base of solutions you find. Debug systematically: Learn to use advanced debugging techniques in Visual Studio. Set conditional breakpoints, use watch windows effectively, and analyze memory usage to find performance issues. Example approach: // When debugging performance issues: 1. Use Stopwatch to measure execution time: var stopwatch = Stopwatch.StartNew(); // Code to measure stopwatch.Stop(); Console.WriteLine($"Execution time: {stopwatch.ElapsedMilliseconds}ms"); 2. Check database query performance with SQL Profiler 3. Look for unnecessary object creation in loops 4. Consider caching frequently accessed data Career Development Actions: Build a learning routine: Set aside time each week (even just 2-3 hours) for learning. Follow blogs like Scott Hanselman's or the official Microsoft Developer blogs. Contribute to open source: Find a C# open source project that interests you and fix a small bug or add a minor feature. This builds your portfolio and connects you with other developers. Track your growth: Keep a developer journal documenting challenges you've solved and skills you've learned. Review it monthly to see your progress. Seek feedback: Ask senior developers to review your code regularly. Accept criticism gracefully and apply what you learn to future work. Practical
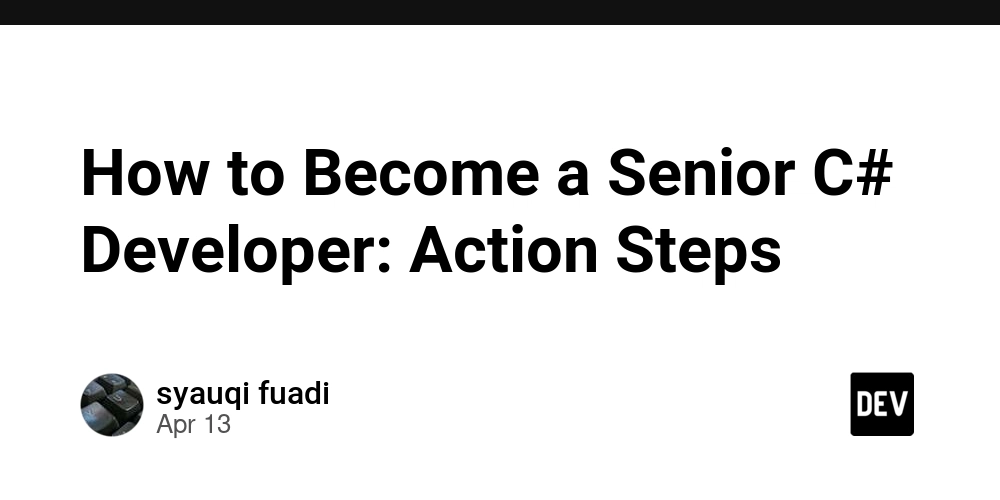
Introduction:
Becoming a senior C# developer takes more than just time on the job. It requires growing your technical skills, developing professional qualities, and taking on bigger responsibilities. This guide offers practical steps to help you advance from a regular C# developer to a senior position.
Master C# Technical Skills:
Deepen your C# knowledge:
- Study advanced C# features like LINQ, async/await, and delegates. Practice using these in your daily work, not just reading about them. For example, try refactoring an existing synchronous method to use async/await patterns in your current project.
Understand .NET ecosystem thoroughly:
- Get comfortable with the latest .NET versions and their features. Build small practice projects using new capabilities. If you're still working with .NET Framework, create a side project using .NET 6/7/8 to experience the differences firsthand.
Learn design patterns:
- Start applying common patterns like Repository, Factory, and Dependency Injection in your code. When you build a new feature, think about which pattern would make your code more maintainable and explain your choice to colleagues.
Master testing:
- Write unit tests for all new code you produce. Set a goal to achieve good test coverage in your projects. Learn to use testing frameworks like NUnit or xUnit and practice test-driven development on a small feature.
Example:
// Instead of this:
public List<Customer> GetActiveCustomers()
{
var customers = _database.GetAllCustomers();
return customers.Where(c => c.IsActive).ToList();
}
// Write testable code like this:
public List<Customer> GetActiveCustomers(ICustomerRepository repository)
{
return repository.GetAll().Where(c => c.IsActive).ToList();
}
Build System Architecture Knowledge:
Learn database design:
- Practice creating efficient database schemas and writing optimized queries. Try to optimize an existing slow query in your project, measuring the performance before and after.
Study system architecture:
- Understand how different parts of applications work together. Draw diagrams of systems you work on to visualize data flow and component interactions.
Explore microservices:
- Build a small project using microservices architecture. Break down a monolithic application into smaller services that communicate with each other.
Practice with cloud services:
- Set up a small application on Azure or AWS. Learn how to deploy, monitor, and scale applications in the cloud. Create a CI/CD pipeline for automatic deployment.
Develop Professional Skills:
Improve code reviews:
- Give helpful, specific feedback during reviews. Instead of saying "this could be better," suggest how: "Consider using a factory pattern here to make this more maintainable."
Mentor others:
- Offer to help junior developers with their tasks. Schedule regular sessions to answer their questions and review their progress on projects.
Communicate clearly:
- Practice explaining technical concepts in simple terms. Try explaining your current project to someone outside your team and refine your explanation based on their questions.
Take ownership:
- Volunteer to lead a small project or feature. Take responsibility for its success, from planning to deployment and maintenance.
Solving Complex Problems:
Break down big issues:
- When facing a complex problem, divide it into smaller, manageable parts. Write out the steps needed to solve each part.
Research efficiently:
- Develop a strategy for finding answers. Know which resources are reliable and relevant for C# development. Create a personal knowledge base of solutions you find.
Debug systematically:
- Learn to use advanced debugging techniques in Visual Studio. Set conditional breakpoints, use watch windows effectively, and analyze memory usage to find performance issues.
Example approach:
// When debugging performance issues:
1. Use Stopwatch to measure execution time:
var stopwatch = Stopwatch.StartNew();
// Code to measure
stopwatch.Stop();
Console.WriteLine($"Execution time: {stopwatch.ElapsedMilliseconds}ms");
2. Check database query performance with SQL Profiler
3. Look for unnecessary object creation in loops
4. Consider caching frequently accessed data
Career Development Actions:
Build a learning routine:
- Set aside time each week (even just 2-3 hours) for learning. Follow blogs like Scott Hanselman's or the official Microsoft Developer blogs.
Contribute to open source:
- Find a C# open source project that interests you and fix a small bug or add a minor feature. This builds your portfolio and connects you with other developers.
Track your growth:
- Keep a developer journal documenting challenges you've solved and skills you've learned. Review it monthly to see your progress.
Seek feedback:
- Ask senior developers to review your code regularly. Accept criticism gracefully and apply what you learn to future work.
Practical Next Steps:
- Choose one technical skill from the list to focus on this month
- Set up a small project to practice this skill
- Find a junior developer you can help mentor
- Join C# communities on Reddit, Stack Overflow, or Discord
- Schedule regular learning time on your calendar
Remember, becoming a senior developer happens gradually through consistent effort. Focus on continuous improvement rather than rushing to check off requirements. Each small step builds toward the expertise and perspective that defines a senior developer.