How I Learned to Talk About Stale Data in React – A Reflection
Recently, while chatting with a fellow developer, they asked me a question: "How do you handle stale data in your React apps?" My first instinct was to say, "I just use React Query and invalidate the data." It sounded straightforward at the time. They then asked the same question again. At first, I thought maybe I hadn’t explained myself clearly, so I repeated my answer: "Yeah, I invalidate the data when it's no longer fresh." That pause made me wonder, “Is there another approach they’re expecting? Am I missing something deeper here?” I wasn’t sure if they were looking for a more in-depth answer or exploring other methods I might use. Looking back, I realize that I could have provided a more detailed response. For instance, I could have mentioned strategies like prefetching or polling, which are useful when ensuring fresh data or managing updates that need to happen regularly. At that moment, I assumed these techniques were common knowledge, so I didn’t elaborate further. I now recognize that a deeper dive into these methods would have painted a more complete picture of how I manage stale data in React. For example, I could have explained how prefetching works by fetching data before it’s needed—so that when the component renders, the data is already available. I also could have touched on polling for real-time updates or discussed how caching works to reduce the need for frequent invalidation. This experience taught me an important lesson: while it’s easy to assume certain concepts are widely known, explaining the “why” and “how” behind each choice can lead to richer technical conversations. It’s a reminder that, in any discussion about architecture or strategy, digging deeper and showcasing a broader understanding is always beneficial. Digging Deeper: Strategies for Managing Stale Data Here are some strategies to consider: 1.Optimistic Updates With optimistic updates, you change the UI immediately as if everything went smoothly, even while the app syncs with the backend. This approach improves the user experience by making interactions feel snappier. const updateTodo = async (todoId, newTitle) => { await fetch(`/api/todos/${todoId}`, { method: 'PUT', body: JSON.stringify({ title: newTitle }), }); // Update local state immediately queryClient.setQueryData(['todos'], (oldTodos) => oldTodos.map((todo) => todo.id === todoId ? { ...todo, title: newTitle } : todo ) ); }; 2.Refetching Data Sometimes, simply refetching the data is the best way to ensure freshness. You can set up automatic refetch intervals or provide a manual refresh button. const { data, refetch } = useQuery('data', fetchData, { refetchInterval: 5000, // Refetch every 5 seconds }); return Refresh Data; 3.WebSockets for Real-Time Updates For live data scenarios, like chats or notifications, WebSockets enable you to receive updates instantly from the server. useEffect(() => { const socket = new WebSocket('wss://your-websocket-url'); socket.onmessage = (event) => { const updatedData = JSON.parse(event.data); queryClient.setQueryData('data', updatedData); }; return () => { socket.close(); }; }, []); 4.Polling If WebSockets aren’t an option, polling is a reliable alternative. This involves regularly sending requests to check for new data. Just be mindful of resource usage. const { data } = useQuery('data', fetchData, { refetchInterval: 10000, // Check for new data every 10 seconds }); 5.Cache Invalidation With React Query, you can invalidate queries when certain events occur, ensuring that the UI reflects the most recent data without the user needing to manually refresh. const mutation = useMutation(updateData, { onSuccess: () => { queryClient.invalidateQueries('data'); }, }); Key Considerations When Managing Stale Data User Experience: Aim for smooth interactions. Optimistic UI updates can help minimize perceived latency. Performance: Be mindful of resource consumption—refetching and polling can strain both the client and the server if overused. Staleness Control: Determine how long your data can remain outdated before it becomes problematic. Tools like TTL (Time-To-Live) can automate this process. Error Handling: Always include proper error management in case data fails to fetch or update. Consistency: Ensure that all parts of your application display consistent data, even when using multiple update strategies. Wrapping Up Reflecting on that conversation, I now appreciate the importance of thoroughly explaining the "why" and "how" behind each data management strategy. Whether it’s through prefetching, polling, or cache invalidation, sharing detailed insights not only deepens your own understanding but also fosters richer technical discussions with peers.
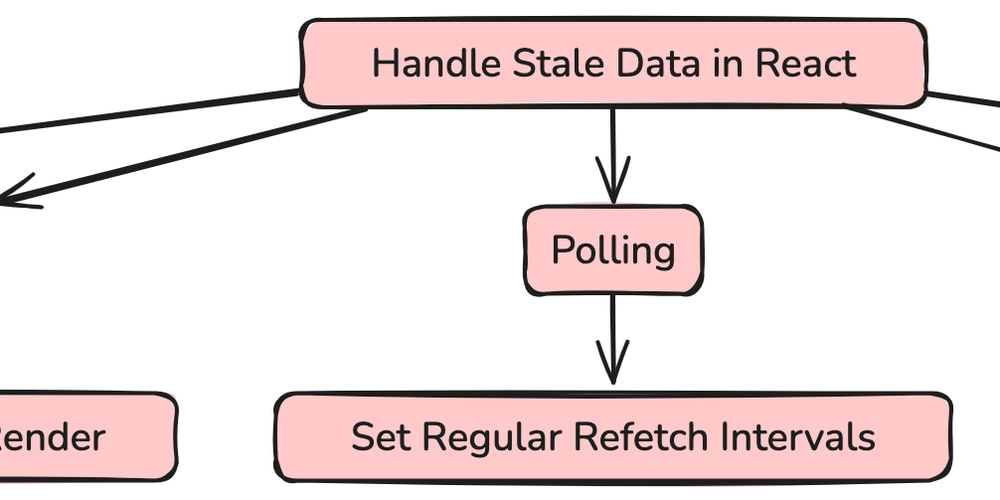
Recently, while chatting with a fellow developer, they asked me a question: "How do you handle stale data in your React apps?" My first instinct was to say, "I just use React Query and invalidate the data." It sounded straightforward at the time.
They then asked the same question again. At first, I thought maybe I hadn’t explained myself clearly, so I repeated my answer:
"Yeah, I invalidate the data when it's no longer fresh."
That pause made me wonder, “Is there another approach they’re expecting? Am I missing something deeper here?” I wasn’t sure if they were looking for a more in-depth answer or exploring other methods I might use.
Looking back, I realize that I could have provided a more detailed response. For instance, I could have mentioned strategies like prefetching or polling, which are useful when ensuring fresh data or managing updates that need to happen regularly. At that moment, I assumed these techniques were common knowledge, so I didn’t elaborate further.
I now recognize that a deeper dive into these methods would have painted a more complete picture of how I manage stale data in React. For example, I could have explained how prefetching works by fetching data before it’s needed—so that when the component renders, the data is already available. I also could have touched on polling for real-time updates or discussed how caching works to reduce the need for frequent invalidation.
This experience taught me an important lesson: while it’s easy to assume certain concepts are widely known, explaining the “why” and “how” behind each choice can lead to richer technical conversations. It’s a reminder that, in any discussion about architecture or strategy, digging deeper and showcasing a broader understanding is always beneficial.
Digging Deeper: Strategies for Managing Stale Data
Here are some strategies to consider:
1.Optimistic Updates
With optimistic updates, you change the UI immediately as if everything went smoothly, even while the app syncs with the backend. This approach improves the user experience by making interactions feel snappier.
const updateTodo = async (todoId, newTitle) => {
await fetch(`/api/todos/${todoId}`, {
method: 'PUT',
body: JSON.stringify({ title: newTitle }),
});
// Update local state immediately
queryClient.setQueryData(['todos'], (oldTodos) =>
oldTodos.map((todo) =>
todo.id === todoId ? { ...todo, title: newTitle } : todo
)
);
};
2.Refetching Data
Sometimes, simply refetching the data is the best way to ensure freshness. You can set up automatic refetch intervals or provide a manual refresh button.
const { data, refetch } = useQuery('data', fetchData, {
refetchInterval: 5000, // Refetch every 5 seconds
});
return <button onClick={refetch}>Refresh Data</button>;
3.WebSockets for Real-Time Updates
For live data scenarios, like chats or notifications, WebSockets enable you to receive updates instantly from the server.
useEffect(() => {
const socket = new WebSocket('wss://your-websocket-url');
socket.onmessage = (event) => {
const updatedData = JSON.parse(event.data);
queryClient.setQueryData('data', updatedData);
};
return () => {
socket.close();
};
}, []);
4.Polling
If WebSockets aren’t an option, polling is a reliable alternative. This involves regularly sending requests to check for new data. Just be mindful of resource usage.
const { data } = useQuery('data', fetchData, {
refetchInterval: 10000, // Check for new data every 10 seconds
});
5.Cache Invalidation
With React Query, you can invalidate queries when certain events occur, ensuring that the UI reflects the most recent data without the user needing to manually refresh.
const mutation = useMutation(updateData, {
onSuccess: () => {
queryClient.invalidateQueries('data');
},
});
Key Considerations When Managing Stale Data
User Experience: Aim for smooth interactions. Optimistic UI updates can help minimize perceived latency.
Performance: Be mindful of resource consumption—refetching and polling can strain both the client and the server if overused.
Staleness Control: Determine how long your data can remain outdated before it becomes problematic. Tools like TTL (Time-To-Live) can automate this process.
Error Handling: Always include proper error management in case data fails to fetch or update.
Consistency: Ensure that all parts of your application display consistent data, even when using multiple update strategies.
Wrapping Up
Reflecting on that conversation, I now appreciate the importance of thoroughly explaining the "why" and "how" behind each data management strategy. Whether it’s through prefetching, polling, or cache invalidation, sharing detailed insights not only deepens your own understanding but also fosters richer technical discussions with peers.