How I Built an Accurate and Ad-Free Age Calculator
Introduction As a developer, I often come across tools that are either cluttered with ads or lack precision. One such tool was an age calculator. Many existing options provided only a rough estimate or came with unnecessary distractions. That’s when I decided to build my own ad-free, accurate age calculator: DOB Calculator. In this article, I will walk you through the technical aspects of how I built it, the challenges I faced, and how you can create something similar. Planning the Age Calculator Before jumping into the code, I outlined my goals: Accuracy – The tool should calculate the exact number of years, months, and days between two dates. Ad-Free Experience – No intrusive ads, just a clean and simple UI. Fast and Responsive – Should work seamlessly on both desktop and mobile devices. User-Friendly Interface – A minimalistic design to keep things simple. Tech Stack To achieve these goals, I chose the following technologies: Frontend: HTML, CSS, JavaScript (Vanilla JS) Backend: Node.js (for future scalability) Hosting: Netlify (for fast and secure deployment) Building the Age Calculation Logic One of the key aspects of the calculator was accurately determining the difference between two dates. Here’s the JavaScript logic I used: `function calculateAge(dob) { let birthDate = new Date(dob); let today = new Date(); let years = today.getFullYear() - birthDate.getFullYear(); let months = today.getMonth() - birthDate.getMonth(); let days = today.getDate() - birthDate.getDate(); if (days < 0) { months--; days += new Date(today.getFullYear(), today.getMonth(), 0).getDate(); } if (months < 0) { years--; months += 12; } return { years, months, days }; } // Example Usage let age = calculateAge("1995-05-15"); console.log(You are ${age.years} years, ${age.months} months, and ${age.days} days old.);` This function correctly accounts for leap years and varying month lengths, ensuring precise calculations. Creating a Simple UI To make the calculator user-friendly, I designed a minimal UI using HTML and CSS: function showAge() { let dob = document.getElementById("dob").value; let age = calculateAge(dob); document.getElementById("result").innerText =You are ${age.years} years, ${age.months} months, and ${age.days} days old.; This keeps the interface clean and simple while ensuring a smooth user experience. Summary of my Experience Building this age calculator was a fun and educational experience. If you’re looking for a precise and ad-free tool, check out DOB Calculator. If you have any suggestions or feedback, feel free to share! What feature would you like to see next in the calculator? Let me know in the comments!
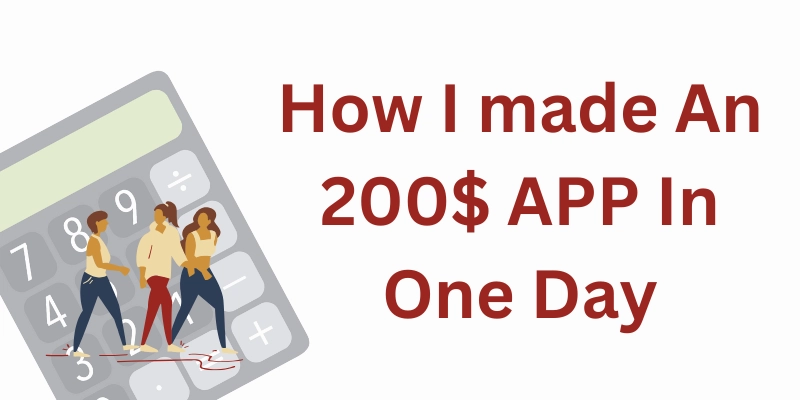
Introduction
As a developer, I often come across tools that are either cluttered with ads or lack precision. One such tool was an age calculator. Many existing options provided only a rough estimate or came with unnecessary distractions. That’s when I decided to build my own ad-free, accurate age calculator: DOB Calculator.
In this article, I will walk you through the technical aspects of how I built it, the challenges I faced, and how you can create something similar.
Planning the Age Calculator
Before jumping into the code, I outlined my goals:
Accuracy – The tool should calculate the exact number of years, months, and days between two dates.
Ad-Free Experience – No intrusive ads, just a clean and simple UI.
Fast and Responsive – Should work seamlessly on both desktop and mobile devices.
User-Friendly Interface – A minimalistic design to keep things simple.
Tech Stack
To achieve these goals, I chose the following technologies:
Frontend: HTML, CSS, JavaScript (Vanilla JS)
Backend: Node.js (for future scalability)
Hosting: Netlify (for fast and secure deployment)
Building the Age Calculation Logic
One of the key aspects of the calculator was accurately determining the difference between two dates. Here’s the JavaScript logic I used:
`function calculateAge(dob) {
let birthDate = new Date(dob);
let today = new Date();
let years = today.getFullYear() - birthDate.getFullYear();
let months = today.getMonth() - birthDate.getMonth();
let days = today.getDate() - birthDate.getDate();
if (days < 0) {
months--;
days += new Date(today.getFullYear(), today.getMonth(), 0).getDate();
}
if (months < 0) {
years--;
months += 12;
}
return { years, months, days };
}
// Example Usage
let age = calculateAge("1995-05-15");
console.log(You are ${age.years} years, ${age.months} months, and ${age.days} days old.
);`
This function correctly accounts for leap years and varying month lengths, ensuring precise calculations.
Creating a Simple UI
To make the calculator user-friendly, I designed a minimal UI using HTML and CSS:
function showAge() {
You are ${age.years} years, ${age.months} months, and ${age.days} days old.
let dob = document.getElementById("dob").value;
let age = calculateAge(dob);
document.getElementById("result").innerText =;
This keeps the interface clean and simple while ensuring a smooth user experience.
Summary of my Experience
Building this age calculator was a fun and educational experience. If you’re looking for a precise and ad-free tool, check out DOB Calculator. If you have any suggestions or feedback, feel free to share!
What feature would you like to see next in the calculator? Let me know in the comments!