How exactly should unit tests be written without mocking extensively?
As I understand, the point of unit tests is to test units of code in isolation. This means, that: They should not break by any unrelated code change elsewhere in the codebase. Only one unit test should break by a bug in the tested unit, as opposed to integration tests (which may break in heaps). All of this implies, that every outside dependency of a tested unit, should be mocked out. And I mean all the outside dependencies, not only the "outside layers" such as networking, filesystem, database, etc.. This leads to a logical conclusion, that virtually every unit test needs to mock. On the other hand, a quick Google search about mocking reveals tons of articles that claim that "mocking is a code smell" and should mostly (though not completely) be avoided. Now, to the question(s). How should unit tests be written properly? Where exactly does the line between them and the integration tests lie? Update 1 Please consider the following pseudo code: class Person { constructor(calculator) {} calculate(a, b) { const sum = this.calculator.add(a, b); // do some other stuff with the `sum` } } Can a test that tests the Person.calculate method without mocking the Calculator dependency (given, that the Calculator is a lightweight class that does not access "the outside world") be considered a unit test?
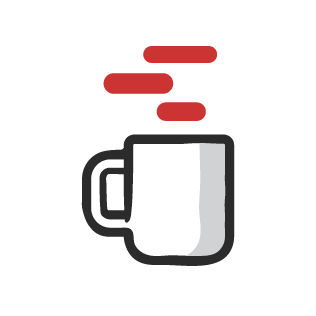
As I understand, the point of unit tests is to test units of code in isolation. This means, that:
- They should not break by any unrelated code change elsewhere in the codebase.
- Only one unit test should break by a bug in the tested unit, as opposed to integration tests (which may break in heaps).
All of this implies, that every outside dependency of a tested unit, should be mocked out. And I mean all the outside dependencies, not only the "outside layers" such as networking, filesystem, database, etc..
This leads to a logical conclusion, that virtually every unit test needs to mock. On the other hand, a quick Google search about mocking reveals tons of articles that claim that "mocking is a code smell" and should mostly (though not completely) be avoided.
Now, to the question(s).
- How should unit tests be written properly?
- Where exactly does the line between them and the integration tests lie?
Update 1
Please consider the following pseudo code:
class Person {
constructor(calculator) {}
calculate(a, b) {
const sum = this.calculator.add(a, b);
// do some other stuff with the `sum`
}
}
Can a test that tests the Person.calculate
method without mocking the Calculator
dependency (given, that the Calculator
is a lightweight class that does not access "the outside world") be considered a unit test?