How Do I Fix Performance Bottlenecks in Node.js?
Node.js is a powerful runtime that enables developers to build fast, scalable applications. However, as your application grows, you might encounter performance bottlenecks that slow it down, cause high memory usage, or even crash your server under load. If you've ever asked yourself, "Why is my Node.js application slow?" or "How can I improve its performance?"—this guide is for you. Understanding Performance Bottlenecks A performance bottleneck is any limitation that prevents your application from handling more load efficiently. In Node.js, bottlenecks can come from: Blocking operations (e.g., synchronous code) Poor database queries Excessive memory consumption (memory leaks) High CPU usage due to expensive computations Inefficient I/O operations Poor concurrency handling Let’s explore how to detect and fix these issues. 1. Identifying Bottlenecks in Node.js Before fixing performance issues, you need to identify the root cause. Here are the best ways to do that: 1.1 Use the Built-in Node.js Profiler Node.js has a built-in profiler that helps analyze CPU and memory usage. You can use it by running: node --prof app.js After running your app for some time, it will generate a log file (isolate-0x*.log). You can process this log using: node --prof-process isolate-0x*.log This gives insights into which functions are consuming the most CPU. 1.2 Use Chrome DevTools to Analyze Performance You can generate a V8 profiler log and analyze it in Chrome DevTools: node --inspect app.js Then, open Chrome and go to chrome://inspect. This allows you to analyze CPU profiles, memory snapshots, and heap allocations. 1.3 Use clinic.js for Deeper Analysis clinic.js is a powerful tool that provides a visual analysis of performance issues. Install it with: npm install -g clinic Then, analyze your app with: clinic doctor -- node app.js It generates an interactive report highlighting bottlenecks. 1.4 Monitor Memory Usage with Heap Snapshots If your app is consuming a lot of memory, take heap snapshots using: node --inspect app.js Then, open Chrome DevTools > Memory > Take Heap Snapshot. 2. Fixing Common Performance Bottlenecks Now that we've identified performance issues, let’s discuss solutions. 2.1 Avoid Blocking the Event Loop Node.js is single-threaded, meaning blocking operations can halt the entire application. Fix: Use Asynchronous Methods Avoid using synchronous functions like fs.readFileSync(), crypto.pbkdf2Sync(), and JSON.parse(largeString). Instead, use: fs.readFile('file.txt', 'utf8', (err, data) => { if (err) throw err; console.log(data); }); For CPU-heavy tasks, offload work to Worker Threads: const { Worker } = require('worker_threads'); const worker = new Worker('./worker.js'); worker.postMessage('Start'); worker.on('message', (message) => console.log(message)); 2.2 Optimize Database Queries Slow database queries can significantly affect performance. Fix: Use Indexing & Connection Pooling If using MongoDB, index frequently queried fields: db.users.createIndex({ email: 1 }); For PostgreSQL/MySQL, use connection pooling with pg-pool or mysql2: const { Pool } = require('pg'); const pool = new Pool({ max: 10 }); Also, avoid the N+1 query problem by using JOIN or batch queries. 2.3 Prevent Memory Leaks Memory leaks cause increased RAM usage, eventually crashing the server. Fix: Avoid Global Variables & Event Listeners Example of a memory leak: let cache = []; setInterval(() => { cache.push(new Array(1000000)); // Consumes memory indefinitely }, 1000); Solution: Use a caching mechanism like node-cache with expiration: const NodeCache = require('node-cache'); const cache = new NodeCache({ stdTTL: 100 }); cache.set('key', 'value'); console.log(cache.get('key')); 2.4 Optimize JSON Parsing & Large Data Handling Parsing large JSON objects blocks the event loop. Fix: Stream Large Files Instead of Reading Them at Once Instead of: const data = fs.readFileSync('large.json', 'utf8'); const json = JSON.parse(data); Use streaming: const readline = require('readline'); const stream = fs.createReadStream('large.json'); const rl = readline.createInterface({ input: stream }); rl.on('line', (line) => console.log(JSON.parse(line))); 2.5 Improve Concurrency with Cluster Mode By default, Node.js runs on one CPU core, which limits scalability. Fix: Use cluster to Utilize Multiple Cores const cluster = require('cluster'); const os = require('os'); if (cluster.isMaster) { os.cpus().forEach(() => cluster.fork()); } else { require('./server'); // Start server instance } This allows multiple in
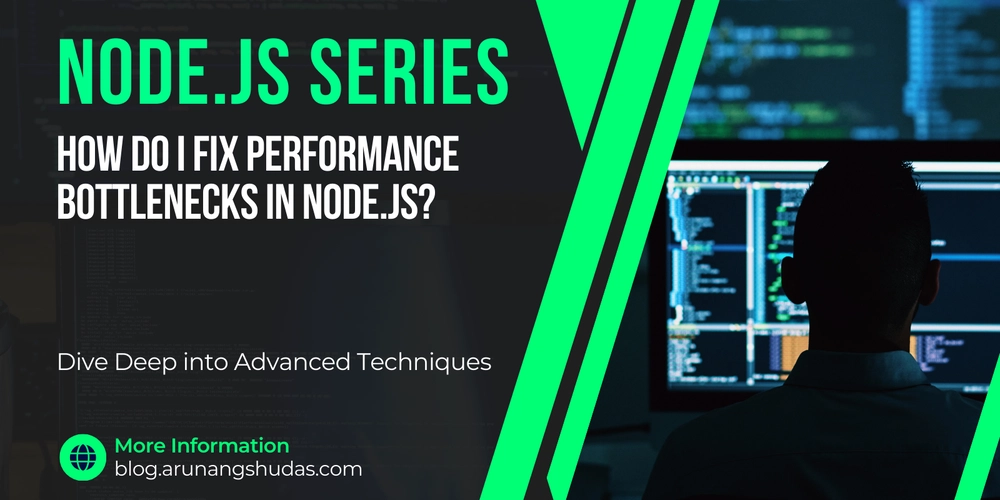
Node.js is a powerful runtime that enables developers to build fast, scalable applications. However, as your application grows, you might encounter performance bottlenecks that slow it down, cause high memory usage, or even crash your server under load.
If you've ever asked yourself, "Why is my Node.js application slow?" or "How can I improve its performance?"—this guide is for you.
Understanding Performance Bottlenecks
A performance bottleneck is any limitation that prevents your application from handling more load efficiently. In Node.js, bottlenecks can come from:
- Blocking operations (e.g., synchronous code)
- Poor database queries
- Excessive memory consumption (memory leaks)
- High CPU usage due to expensive computations
- Inefficient I/O operations
- Poor concurrency handling
Let’s explore how to detect and fix these issues.
1. Identifying Bottlenecks in Node.js
Before fixing performance issues, you need to identify the root cause. Here are the best ways to do that:
1.1 Use the Built-in Node.js Profiler
Node.js has a built-in profiler that helps analyze CPU and memory usage. You can use it by running:
node --prof app.js
After running your app for some time, it will generate a log file (isolate-0x*.log
). You can process this log using:
node --prof-process isolate-0x*.log
This gives insights into which functions are consuming the most CPU.
1.2 Use Chrome DevTools to Analyze Performance
You can generate a V8 profiler log and analyze it in Chrome DevTools:
node --inspect app.js
Then, open Chrome and go to chrome://inspect
. This allows you to analyze CPU profiles, memory snapshots, and heap allocations.
1.3 Use clinic.js
for Deeper Analysis
clinic.js
is a powerful tool that provides a visual analysis of performance issues. Install it with:
npm install -g clinic
Then, analyze your app with:
clinic doctor -- node app.js
It generates an interactive report highlighting bottlenecks.
1.4 Monitor Memory Usage with Heap Snapshots
If your app is consuming a lot of memory, take heap snapshots using:
node --inspect app.js
Then, open Chrome DevTools > Memory > Take Heap Snapshot.
2. Fixing Common Performance Bottlenecks
Now that we've identified performance issues, let’s discuss solutions.
2.1 Avoid Blocking the Event Loop
Node.js is single-threaded, meaning blocking operations can halt the entire application.
Fix: Use Asynchronous Methods
Avoid using synchronous functions like fs.readFileSync()
, crypto.pbkdf2Sync()
, and JSON.parse(largeString)
. Instead, use:
fs.readFile('file.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
For CPU-heavy tasks, offload work to Worker Threads:
const { Worker } = require('worker_threads');
const worker = new Worker('./worker.js');
worker.postMessage('Start');
worker.on('message', (message) => console.log(message));
2.2 Optimize Database Queries
Slow database queries can significantly affect performance.
Fix: Use Indexing & Connection Pooling
If using MongoDB, index frequently queried fields:
db.users.createIndex({ email: 1 });
For PostgreSQL/MySQL, use connection pooling with pg-pool
or mysql2
:
const { Pool } = require('pg');
const pool = new Pool({ max: 10 });
Also, avoid the N+1 query problem by using JOIN
or batch queries.
2.3 Prevent Memory Leaks
Memory leaks cause increased RAM usage, eventually crashing the server.
Fix: Avoid Global Variables & Event Listeners
Example of a memory leak:
let cache = [];
setInterval(() => {
cache.push(new Array(1000000)); // Consumes memory indefinitely
}, 1000);
Solution: Use a caching mechanism like node-cache
with expiration:
const NodeCache = require('node-cache');
const cache = new NodeCache({ stdTTL: 100 });
cache.set('key', 'value');
console.log(cache.get('key'));
2.4 Optimize JSON Parsing & Large Data Handling
Parsing large JSON objects blocks the event loop.
Fix: Stream Large Files Instead of Reading Them at Once
Instead of:
const data = fs.readFileSync('large.json', 'utf8');
const json = JSON.parse(data);
Use streaming:
const readline = require('readline');
const stream = fs.createReadStream('large.json');
const rl = readline.createInterface({ input: stream });
rl.on('line', (line) => console.log(JSON.parse(line)));
2.5 Improve Concurrency with Cluster Mode
By default, Node.js runs on one CPU core, which limits scalability.
Fix: Use cluster
to Utilize Multiple Cores
const cluster = require('cluster');
const os = require('os');
if (cluster.isMaster) {
os.cpus().forEach(() => cluster.fork());
} else {
require('./server'); // Start server instance
}
This allows multiple instances to run on different cores.
2.6 Use Caching to Reduce Load
Fetching the same data repeatedly from a database is inefficient.
Fix: Implement Redis Caching
const redis = require('redis');
const client = redis.createClient();
app.get('/data', async (req, res) => {
client.get('key', async (err, cachedData) => {
if (cachedData) return res.json(JSON.parse(cachedData));
const data = await fetchDataFromDB();
client.setex('key', 3600, JSON.stringify(data));
res.json(data);
});
});
2.7 Use Compression to Reduce Response Size
Sending large responses over the network increases latency.
Fix: Enable Gzip Compression
Install compression
:
npm install compression
Use it in your Express app:
const compression = require('compression');
app.use(compression());
Key Takeaways:
→ Use profiling tools like Chrome DevTools & clinic.js
→ Avoid blocking operations by using async code & worker threads
→ Optimize database queries with indexes & connection pooling
→ Prevent memory leaks by managing global objects & event listeners
→ Use clustering to utilize multiple CPU cores
→ Cache frequent queries with Redis
You may also like:
Read more blogs from Here
Share your experiences in the comments, and let’s discuss how to tackle them!
Follow me on Linkedin