Here's how Nue.js applies colors to the console output in the CLI.
In this article, we will review how Nue.js applies colors to the console logs in the CLI. I found this log function in the cli.js file. async function printVersion() { log(`Nue ${version} ${colors.green('•')} ${getEngine()}`) } log function is imported from packages/nuekit/src/util.js export function log(msg, extra = '') { console.log(colors.green('✓'), msg, extra) } What is colors.green here? colors function is defined in the same file and has the below code: // console colors export const colors = function() { const codes = { red: 31, green: 32, yellow: 33, blue: 34, magenta: 35, cyan: 36, gray: 90 } const fns = {} const noColor = process.env.NO_COLOR || !(process.env.TERM || process.platform == 'win32') for (const key in codes) { fns[key] = msg => noColor ? msg : `\u001b[${codes[key]}m${msg}\u001b[39m` } return fns }() I find this function really interesting because in the wild, OSS projects use Picocolors or chalk to apply colors to the console output in the CLI. There’s codes and noColor is set to true if there is an env, NO_COLOR, or when the platform is ‘win32’ and TERM is set (not sure what this TERM is). The trick is in the below code: for (const key in codes) { fns[key] = msg => noColor ? msg : `\u001b[${codes[key]}m${msg}\u001b[39m` } Here a \u001b[${codes[key]}m${msg}\u001b[39m is a template string commonly seen in JavaScript or TypeScript used to format console output with colors using ANSI escape codes. Interesting. About me: Hey, my name is Ramu Narasinga. I study large open-source projects and create content about their codebase architecture and best practices, sharing it through articles, videos. I am open to work on interesting projects. Send me an email at ramu.narasinga@gmail.com My Github — https://github.com/ramu-narasinga My website — https://ramunarasinga.com My Youtube channel — https://www.youtube.com/@ramu-narasinga Learning platform — https://thinkthroo.com Codebase Architecture — https://app.thinkthroo.com/architecture Best practices — https://app.thinkthroo.com/best-practices Production-grade projects — https://app.thinkthroo.com/production-grade-projects References: https://github.com/nuejs/nue/blob/master/packages/nuekit/src/util.js#L47 https://github.com/nuejs/nue/blob/master/packages/nuekit/src/util.js#L38 https://github.com/nuejs/nue/blob/master/packages/nuekit/src/cli.js#L82 https://www.npmjs.com/package/chalk https://www.npmjs.com/package/picocolors
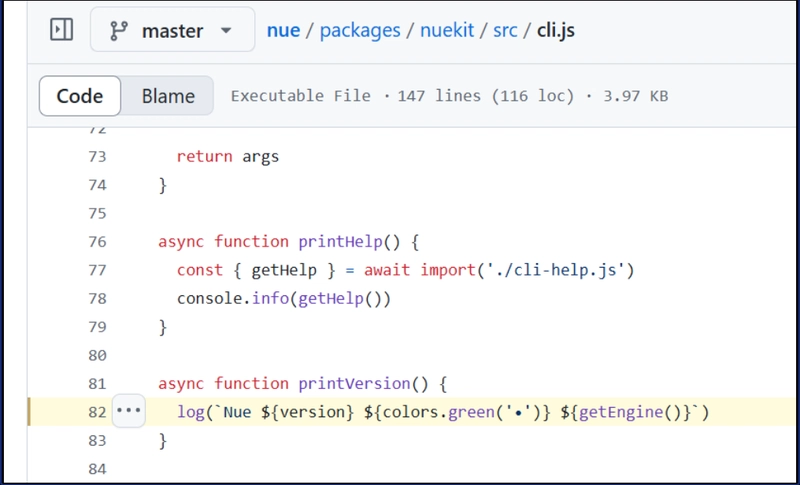
In this article, we will review how Nue.js applies colors to the console logs in the CLI.
I found this log function in the cli.js file.
async function printVersion() {
log(`Nue ${version} ${colors.green('•')} ${getEngine()}`)
}
log function is imported from packages/nuekit/src/util.js
export function log(msg, extra = '') {
console.log(colors.green('✓'), msg, extra)
}
What is colors.green here? colors function is defined in the same file and has the below code:
// console colors
export const colors = function() {
const codes = { red: 31, green: 32, yellow: 33, blue: 34, magenta: 35, cyan: 36, gray: 90 }
const fns = {}
const noColor = process.env.NO_COLOR || !(process.env.TERM || process.platform == 'win32')
for (const key in codes) {
fns[key] = msg => noColor ? msg : `\u001b[${codes[key]}m${msg}\u001b[39m`
}
return fns
}()
I find this function really interesting because in the wild, OSS projects use Picocolors or chalk to apply colors to the console output in the CLI.
There’s codes and noColor is set to true if there is an env, NO_COLOR, or when the platform is ‘win32’ and TERM is set (not sure what this TERM is).
The trick is in the below code:
for (const key in codes) {
fns[key] = msg => noColor ? msg : `\u001b[${codes[key]}m${msg}\u001b[39m`
}
Here a \u001b[${codes[key]}m${msg}\u001b[39m
is a template string commonly seen in JavaScript or TypeScript used to format console output with colors using ANSI escape codes. Interesting.
About me:
Hey, my name is Ramu Narasinga. I study large open-source projects and create content about their codebase architecture and best practices, sharing it through articles, videos.
I am open to work on interesting projects. Send me an email at ramu.narasinga@gmail.com
My Github — https://github.com/ramu-narasinga
My website — https://ramunarasinga.com
My Youtube channel — https://www.youtube.com/@ramu-narasinga
Learning platform — https://thinkthroo.com
Codebase Architecture — https://app.thinkthroo.com/architecture
Best practices — https://app.thinkthroo.com/best-practices
Production-grade projects — https://app.thinkthroo.com/production-grade-projects