GraphQL vs REST: A Technical Deep Dive into API Design
API architecture is a design framework that determines how an API is structured and built. It is the blueprint that defines how requests are made, data is exchanged, and functionality is delivered. Among several API architectures, such as SOAP, gRPC, and others, REST and GraphQL are two of the most popular choices. The former uses an architectural style similar to that of the web, while the latter is a query language for APIs. This article will break down both architectural styles and provide a technical comparison between them. Additionally, it'll go over how API security works in GraphQL vs REST and provide clarity on when best to use either of them. REST API: Architecture, strengths, and limitations Representational State Transfer (REST) is an architectural style that has become the backbone of many web-based APIs due to its simplicity and alignment with HTTP protocols. Introduced by Roy Fielding in his 2000 dissertation, REST utilizes the web's existing infrastructure to facilitate communication between clients and servers. Architecture REST architecture At its core, REST treats every piece of data or functionality as a resource, identified by a unique URL. These resources—such as users, posts, or orders—are manipulated using standard HTTP methods: GET to retrieve, POST to create, PUT to update, and DELETE to remove. For example, a GET request to api.example.com/users/123 might return a JSON object like: { "id": 123, "name": "Alice", "email": "alice@example.com" } REST is stateless, meaning each request contains all the information the server needs to process it—no session data is stored between calls. This statelessness, paired with a resource-based approach, makes REST intuitive: developers interact with APIs much like they navigate websites. Responses typically come in JSON (though XML is an option), and HTTP status codes signal success or failure. REST's reliance on HTTP also enables caching, where responses can be stored and reused to boost performance—a feature baked into web browsers and CDNs (Content Delivery Networks). To evolve, REST APIs often use versioning (e.g., api.example.com/v1/users), ensuring backward compatibility as requirements change. Strengths The following are some reasons why REST has become a go-to choice in API development for many developers: Simplicity and familiarity: Built on HTTP, REST is easy to grasp for developers who are already comfortable with web development. Its resource-based approach maps well to common data structures like databases. Scalability: Statelessness allows servers to handle requests independently, making it straightforward to scale horizontally by adding more machines. Caching further reduces server load. Broad support: REST enjoys mature tooling (e.g., Swagger/OpenAPI for documentation and platforms like Blackbird for end-to-end API development) and is natively supported by virtually all programming languages and frameworks. Flexibility: Thanks to its lightweight nature, it works for a wide range of applications, from public APIs (e.g., Twitter's API) to internal microservices. Limitations Despite its strengths, REST has notable drawbacks, especially as application complexity grows: Over-fetching and under-fetching: REST endpoints return fixed data structures. A call to /users/123 might include unwanted fields (over-fetching) or lack related data like posts (under-fetching), requiring additional requests. For instance, fetching a user's posts and comments might need multiple calls: /users/123, /users/123/posts, /posts/456/comments. Endpoint proliferation: Complex systems can lead to a sprawl of API endpoints. A social media app might need dozens of URLs to cover users, friends, posts, likes, and more, complicating maintenance. API versioning overhead: Changes to an API often require new versions (e.g., /v2/users), forcing developers to juggle multiple implementations or sunset old ones. Performance in complex scenarios: Multiple round trips for related data can slow down performance, especially on high-latency networks like mobile connections. These limitations don't render REST obsolete, but they highlight why alternatives like GraphQL have emerged. For example, a mobile app needing a user's profile, posts, and likes in one go might find REST's multi-request approach inefficient compared to a more tailored solution. GraphQL: Architecture, advantages, and trade-offs GraphQL, introduced by Facebook in 2015, is a query language for APIs that reexamines how clients and servers exchange data. Unlike REST's resource-centric model, GraphQL allows clients to request exactly the data they need in a single, flexible query. Architecture *GraphQL architecture * GraphQL revolves around a single endpoint—typically /graphql—through which all requests flow. Instead of predefined resource URLs, it uses a schema to define the data structure available on the server. This schema, written in a strongly typed language, outl
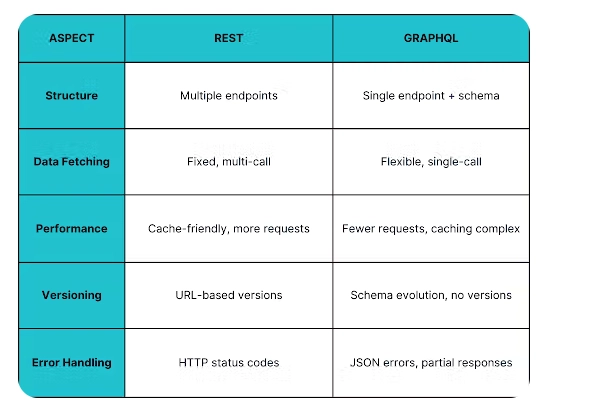
API architecture is a design framework that determines how an API is structured and built. It is the blueprint that defines how requests are made, data is exchanged, and functionality is delivered.
Among several API architectures, such as SOAP, gRPC, and others, REST and GraphQL are two of the most popular choices. The former uses an architectural style similar to that of the web, while the latter is a query language for APIs.
This article will break down both architectural styles and provide a technical comparison between them. Additionally, it'll go over how API security works in GraphQL vs REST and provide clarity on when best to use either of them.
REST API: Architecture, strengths, and limitations
Representational State Transfer (REST) is an architectural style that has become the backbone of many web-based APIs due to its simplicity and alignment with HTTP protocols. Introduced by Roy Fielding in his 2000 dissertation, REST utilizes the web's existing infrastructure to facilitate communication between clients and servers.
Architecture
REST architecture
At its core, REST treats every piece of data or functionality as a resource, identified by a unique URL. These resources—such as users, posts, or orders—are manipulated using standard HTTP methods: GET to retrieve, POST to create, PUT to update, and DELETE to remove.
For example, a GET request to api.example.com/users/123 might return a JSON object like:
{
"id": 123,
"name": "Alice",
"email": "alice@example.com"
}
REST is stateless, meaning each request contains all the information the server needs to process it—no session data is stored between calls. This statelessness, paired with a resource-based approach, makes REST intuitive: developers interact with APIs much like they navigate websites.
Responses typically come in JSON (though XML is an option), and HTTP status codes signal success or failure.
REST's reliance on HTTP also enables caching, where responses can be stored and reused to boost performance—a feature baked into web browsers and CDNs (Content Delivery Networks).
To evolve, REST APIs often use versioning (e.g., api.example.com/v1/users), ensuring backward compatibility as requirements change.
Strengths
The following are some reasons why REST has become a go-to choice in API development for many developers:
Simplicity and familiarity: Built on HTTP, REST is easy to grasp for developers who are already comfortable with web development. Its resource-based approach maps well to common data structures like databases.
Scalability: Statelessness allows servers to handle requests independently, making it straightforward to scale horizontally by adding more machines. Caching further reduces server load.
Broad support: REST enjoys mature tooling (e.g., Swagger/OpenAPI for documentation and platforms like Blackbird for end-to-end API development) and is natively supported by virtually all programming languages and frameworks.
Flexibility: Thanks to its lightweight nature, it works for a wide range of applications, from public APIs (e.g., Twitter's API) to internal microservices.
Limitations
Despite its strengths, REST has notable drawbacks, especially as application complexity grows:
Over-fetching and under-fetching: REST endpoints return fixed data structures. A call to /users/123 might include unwanted fields (over-fetching) or lack related data like posts (under-fetching), requiring additional requests. For instance, fetching a user's posts and comments might need multiple calls: /users/123, /users/123/posts, /posts/456/comments.
Endpoint proliferation: Complex systems can lead to a sprawl of API endpoints. A social media app might need dozens of URLs to cover users, friends, posts, likes, and more, complicating maintenance.
API versioning overhead: Changes to an API often require new versions (e.g., /v2/users), forcing developers to juggle multiple implementations or sunset old ones.
Performance in complex scenarios: Multiple round trips for related data can slow down performance, especially on high-latency networks like mobile connections.
These limitations don't render REST obsolete, but they highlight why alternatives like GraphQL have emerged. For example, a mobile app needing a user's profile, posts, and likes in one go might find REST's multi-request approach inefficient compared to a more tailored solution.
GraphQL: Architecture, advantages, and trade-offs
GraphQL, introduced by Facebook in 2015, is a query language for APIs that reexamines how clients and servers exchange data. Unlike REST's resource-centric model, GraphQL allows clients to request exactly the data they need in a single, flexible query.
Architecture
*GraphQL architecture
*
GraphQL revolves around a single endpoint—typically /graphql—through which all requests flow. Instead of predefined resource URLs, it uses a schema to define the data structure available on the server. This schema, written in a strongly typed language, outlines types (e.g., User, Post) and their fields (e.g., name, title).
For example:
type User {
id: ID!
name: String
posts: [Post]
}
type Post {
id: ID!
title: String
}
Clients send queries to this endpoint, specifying the exact data they want. For instance, a query like this:
query {
user(id: "123") {
name
posts {
title
}
}
}
Might return:
`
{
"data": {
"user": {
"name": "Alice",
"posts": [
{ "title": "Hello" },
{ "title": "World" }
]
}
}
}
`
GraphQL is still HTTP-based (usually), but it treats HTTP as a transport layer—queries are POSTed to the endpoint, and responses always return a 200 status, with errors nested in the JSON (e.g., {"errors": [{"message": "User not found"}] }).
The server resolves queries by mapping them to backend logic, often via resolvers—functions that fetch the requested data. Unlike REST, GraphQL avoids versioning; the schema evolves by adding new fields or deprecating old ones.
Advantages
GraphQL's design brings compelling benefits, especially for modern, data-intensive applications:
Precise data fetching: Clients avoid over- or under-fetching by requesting only what they need. For example, a mobile app can grab a user's name and recent posts in one call rather than hitting multiple REST endpoints.
Single-endpoint simplicity: One URL handles all requests, reducing the endpoint sprawl seen in REST. This streamlines API design as complexity grows.
**Flexibility and evolution: **The schema adapts to changing needs without versioning. Developers can add fields (e.g., email to User) while marking obsolete ones as deprecated, keeping the API lean.
Ecosystem and tooling: Tools like Apollo Client and GraphiQL enhance development, offering real-time query testing and client-side state management.
Trade-offs
GraphQL's power comes with challenges that can complicate its adoption. These include:
Learning curve: Its schema-based approach and query language require more upfront effort than REST's straightforward HTTP model. Developers must master concepts like resolvers and schema design.
Caching complexity: REST's URL-based caching (via HTTP headers) is simple; GraphQL's single endpoint makes caching trickier, often requiring custom solutions like persisted queries or third-party tools.
Server-side overhead: Flexible queries can strain servers if not optimized. A poorly written query (e.g., fetching deeply nested data) might trigger expensive database calls, necessitating rate limiting or query complexity analysis.
Less universal support: While growing, GraphQL's ecosystem isn't as ubiquitous as REST's. Legacy systems or simpler projects might not justify the switch.
For instance, a real-time dashboard pulling varied data might thrive with GraphQL, but a basic CRUD app could find its overhead unnecessary compared to REST's simplicity.
Technical comparison: GraphQL vs REST
REST and GraphQL represent two distinct paradigms for API design, each with its own approach to structure, data handling, and performance. While REST has long been the default for web APIs, GraphQL's rise reflects a shift toward flexibility and efficiency in modern applications.
The following points highlight how they differ and where each excels:
**1. Architectural approach
**REST is built on a resource-centric model which uses multiple endpoints to represent data or services. Each resource gets its own URL, like api.example.com/users/123 or api.example.com/posts/456.
It leverages HTTP's verbs (GET, POST, PUT, DELETE) to define actions, aligning closely with web standards. This distributed structure mimics how websites are navigated, with stateless requests driving interactions.
GraphL, on the other hand, centers on a single endpoint (e.g., api.example.com/graphql) backed by a strongly typed schema. Instead of predefined resources, clients query the schema to shape the response.
A query like user(id: "123") { name, posts { title } } pulls nested data in one go. This centralized approach shifts control to the client, abstracting resource-specific URLs into a unified interface.
**2. Data fetching and efficiency
**REST delivers fixed data structures per endpoint. A call to /users/123 might return { id: 123, name: "Alice", email: "alice@example.com"}, even if only the name is needed (over-fetching).
Fetching related data—like posts—requires another call (e.g., /users/123/posts), leading to under-fetching and multiple round trips.
GraphQL, on the other hand, lets clients specify their exact data needs in a single query. This, in turn, results in a tailored JSON response with just the requested fields, no excess. This reduces network calls and eliminates over/under-fetching.
**3. Performance and caching
**REST relies on HTTP/1.1 (or HTTP/2 where adopted) and benefits from native caching via headers like ETag or Cache-Control. Browsers or CDNs can cache a GET to /users/123, cutting server load. However, multiple requests for related data can bog down performance, especially on high-latency networks (e.g., mobile).
Typically, GraphQL uses HTTP POSTs to its single endpoint, fetching more data in fewer calls. However, caching is less straightforward; the dynamic nature of queries complicates HTTP-level caching. Solutions like persisted queries (predefined query IDs) or tools like Apollo Client add caching, but they require extra setup.
**4. Versioning and evolution
**REST handles changes via API versioning—e.g., api.example.com/v1/users becomes /v2/users when fields or logic shift. This ensures backward compatibility but can lead to maintaining multiple API versions, increasing overhead. Developers must carefully sunset old endpoints.
On the other hand, GraphQL avoids versioning by evolving the schema. New fields (e.g., email on User) are added, and outdated ones are deprecated with warnings (e.g., @deprecated). Clients adapt by updating queries, thereby keeping the API lean. However, this requires disciplined schema design to avoid breaking changes.
**5. Error handling
**RESTful APIs use HTTP status codes to communicate outcomes—200 OK for success, 404 Not Found for missing resources, and 500 for server errors. Error details vary by implementation, often tucked into the response body (e.g., {"error": "User not found"}). It's clear and standardized but lacks nuance for partial failures.
GraphQL always returns a 200 OK status, embedding errors in the response alongside data:
{
"data": { "user": null },
"errors": [{"message": "User not found", "path": ["user"] }]
}
This allows partial successes (e.g., some fields resolve despite errors), offering more granularity but diverging from HTTP conventions.
Below is a table summarizing the comparison:
GraphQL vs REST
API Security: GraphQL vs REST
You can't talk about APIs without mentioning security. It governs how data and functionality are protected from unauthorized access, misuse, or attacks.
While they differ in design, GraphQL and REST both operate over HTTP and face similar threats, such as authentication bypasses, injection attacks, or denial-of-service (DoS).
However, their architectural differences shape how security is implemented, and the challenges developers encounter.
REST API security
REST uses HTTP's ecosystem, making its security familiar and well-established. For authentication, it often relies on token-based systems like OAuth 2.0 or API keys. Clients send tokens in the Authorization header (e.g., Bearer ) with each request to an endpoint like /users/123. Servers validate the token and check permissions (e.g., "Can this user read this resource?") using middleware or backend logic.
Additionally, REST's URL-based structure makes it easy to apply rate limits per endpoint (e.g., 100 requests/hour to /posts). Tools like API gateways (e.g., Edge Stack) or reverse proxies often handle this, API throttling excessive traffic.
REST benefits from HTTP's mature security toolkit—HTTPS, OAuth, and caching controls (e.g., Cache-Control: private) are plug-and-play. Its statelessness simplifies session management, reducing attack surfaces like session hijacking.
GraphQL API security
GraphQL's single-endpoint, query-driven design shifts the security landscape, introducing unique protections and pitfalls.
Like REST, GraphQL uses OAuth, JWTs, or API keys, typically passed in headers for authentication. However, authorization happens at the field level within the schema. For example, a resolver for user.email might check if the requester has permission, even if user.name is public. This granularity requires careful implementation in resolvers.
Transport security mirrors REST—queries are POSTed to /graphql, so securing the endpoint is identical to REST's TLS setup.
GraphQL's schema offers fine-grained control—fields can be locked down individually, reducing accidental data leaks. A single endpoint simplifies securing transport (one HTTPS setup vs. many).
Best practices for API security
For both REST and GraphQL, the following practices enhance security:
REST: Enforce HTTPS, validate every endpoint, hide error details, and use API gateways.
GraphQL: Limit query depth/cost, sanitize inputs, mask errors, and enforce field-level authorization.
When to use GraphQL vs REST
REST is the ideal choice when simplicity and stability are priorities. It shines for straightforward CRUD operations, like a weather API (/weather/city), where fixed endpoints and HTTP's mature ecosystem—caching, OAuth, and versioning—make it easy to implement and scale.
It's perfect for public APIs or teams leveraging existing REST expertise. It offers predictable security (HTTPS, rate limiting) and performance via caching, though it struggles with complex data needs requiring multiple requests.
GraphQL excels when flexibility and efficiency take precedence, especially for complex, nested data—like a social media app fetching users, posts, and likes in one query (user { posts { title } }).
It's a fit for mobile apps, rapidly evolving projects, or client-driven development with a single, schema-driven endpoint. However, GraphQL demands more setup—query depth limits, resolver-level security—and a steeper learning curve, making it overkill for many use cases.
Choosing the right API architecture
GraphQL and REST each bring distinct strengths to API design, shaped by their architectures and trade-offs.
REST's resource-based simplicity, rooted in HTTP's conventions, offers a stable, cache-friendly foundation for straightforward applications—ideal for public APIs or legacy systems where predictability reigns.
On the other hand, GraphQL, with its schema-driven flexibility, redefines efficiency for complex, client-centric needs, cutting through REST's limitations with tailored data fetching and a single endpoint.
Ultimately, the choice between GraphQL and REST isn't about superiority but fit. REST powers simplicity and broad adoption; GraphQL fuels innovation in dynamic, data-intensive apps.
As your API demands evolve, understanding their mechanics, strengths, and challenges will equip you to pick the right tool for the job.