Go Lang Part - 02
In this blog post we will discuss a bit more on the Go lang. Functions Functions in Go can take zero or more arguments. To make Go code easier to read, the variable type comes after the variable name. Example: Accepts two integers and returns subtraction result. func sub(x int, y int) int { return x-y } Multiple parameters When multiple arguments are of the same type, the type only needs to be declared after the last one, assuming they are in order. If they are not in order they need to be defined seperately. Example: func add(x, y int) int { return x + y } func somename(firstname , lastname string, age int) int { } callbacks calling a function by passing another function as an argument to the function. f func(func(int,int) int), int) int Memory In Go variables are passed by value not by reference. The below code even though the increment function 5+1 but in the main() function has still 5. func main(){ x := 5 increment(x) fmt.Println(x) // 5 } func increment(x int){ x++ }
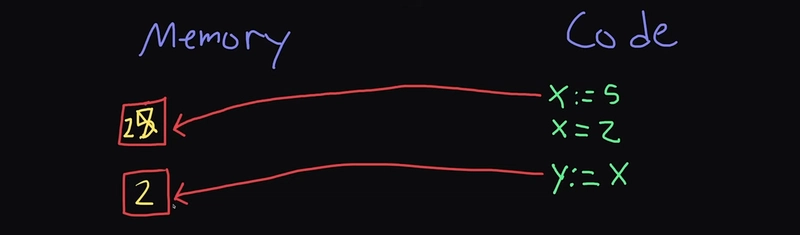
In this blog post we will discuss a bit more on the Go lang.
Functions
Functions in Go can take zero or more arguments.
To make Go code easier to read, the variable type comes after the variable name.
Example: Accepts two integers and returns subtraction result.
func sub(x int, y int) int {
return x-y
}
Multiple parameters
When multiple arguments are of the same type, the type only needs to be declared after the last one, assuming they are in order.
If they are not in order they need to be defined seperately.
Example:
func add(x, y int) int {
return x + y
}
func somename(firstname , lastname string, age int) int {
}
callbacks
calling a function by passing another function as an argument to the function.
f func(func(int,int) int), int) int
Memory
In Go variables are passed by value not by reference.
The below code even though the increment
function 5+1
but in the main()
function has still 5.
func main(){
x := 5
increment(x)
fmt.Println(x)
// 5
}
func increment(x int){
x++
}